+
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/devs/user/account-security.php b/devs/user/account-security.php
new file mode 100644
index 0000000..c535e7f
--- /dev/null
+++ b/devs/user/account-security.php
@@ -0,0 +1,490 @@
+prepare("SELECT * FROM users WHERE id = :user_id");
+ $stmt->bindParam(':user_id', $user_id);
+ $stmt->execute();
+ $user = $stmt->fetch(PDO::FETCH_ASSOC);
+ $profile_photo = $user['profile_photo'] ?: $default_profile_photo;
+} else {
+ $profile_photo = $default_profile_photo;
+}
+
+// Başarı ve hata mesajlarını tanımla
+$success_message = '';
+$error_message = '';
+
+// Form gönderildiyse
+if ($_SERVER['REQUEST_METHOD'] == 'POST' && isset($_POST['change_password'])) {
+ $current_password = $_POST['current_password'] ?? '';
+ $new_password = $_POST['password'] ?? '';
+ $confirm_password = $_POST['confirm_password'] ?? '';
+
+ // Şifre alanları boş mu kontrolü
+ if (empty($current_password) || empty($new_password) || empty($confirm_password)) {
+ $error_message = "Lütfen tüm alanları doldurunuz.";
+ }
+ // Yeni şifreler eşleşiyor mu kontrolü
+ elseif ($new_password !== $confirm_password) {
+ $error_message = "Yeni şifreler eşleşmiyor.";
+ }
+ // Yeni şifre minimum uzunluk kontrolü
+ elseif (strlen($new_password) < 6) {
+ $error_message = "Yeni şifre en az 6 karakter uzunluğunda olmalıdır.";
+ }
+ else {
+ // Mevcut şifreyi doğrula
+ $stmt = $db->prepare("SELECT password FROM users WHERE id = :user_id");
+ $stmt->bindParam(':user_id', $user_id);
+ $stmt->execute();
+ $row = $stmt->fetch(PDO::FETCH_ASSOC);
+
+ if (!$row || !password_verify($current_password, $row['password'])) {
+ $error_message = "Mevcut şifreniz hatalı.";
+ } else {
+ // Yeni şifre mevcut şifre ile aynı mı kontrolü
+ if (password_verify($new_password, $row['password'])) {
+ $error_message = "Yeni şifreniz mevcut şifrenizle aynı olamaz.";
+ } else {
+ // Yeni şifreyi şifrele
+ $hashed_password = password_hash($new_password, PASSWORD_DEFAULT);
+
+ // Veritabanında şifreyi güncelle
+ $stmt = $db->prepare("UPDATE users SET password = :password WHERE id = :user_id");
+ $stmt->bindParam(':password', $hashed_password);
+ $stmt->bindParam(':user_id', $user_id);
+
+ if ($stmt->execute()) {
+ $success_message = "Şifreniz başarıyla güncellendi.";
+ } else {
+ $error_message = "Şifre güncellenirken bir hata oluştu.";
+ }
+ }
+ }
+ }
+}
+
+// Mevcut kodun başına ekleyin
+$delete_error_message = '';
+$delete_success_message = '';
+
+// Hesap silme işlemi için form gönderildiyse
+if ($_SERVER['REQUEST_METHOD'] == 'POST' && isset($_POST['delete_account'])) {
+ $password = $_POST['delete_password'] ?? '';
+
+ if (empty($password)) {
+ $delete_error_message = "Hesabınızı silmek için şifrenizi girmelisiniz.";
+ } else {
+ // Şifreyi doğrula
+ $stmt = $db->prepare("SELECT password FROM users WHERE id = :user_id");
+ $stmt->bindParam(':user_id', $user_id);
+ $stmt->execute();
+ $row = $stmt->fetch(PDO::FETCH_ASSOC);
+
+ if (!$row || !password_verify($password, $row['password'])) {
+ $delete_error_message = "Girdiğiniz şifre hatalı.";
+ } else {
+ try {
+ // Kullanıcının tüm verilerini silme işlemleri
+ $db->beginTransaction();
+
+ // Kullanıcının projelerini sil
+ $stmt = $db->prepare("DELETE FROM projects WHERE user_id = :user_id");
+ $stmt->bindParam(':user_id', $user_id);
+ $stmt->execute();
+
+ // Kullanıcının hesabını sil
+ $stmt = $db->prepare("DELETE FROM users WHERE id = :user_id");
+ $stmt->bindParam(':user_id', $user_id);
+ $stmt->execute();
+
+ $db->commit();
+
+ // Oturumu sonlandır
+ session_destroy();
+
+ // Kullanıcıyı giriş sayfasına yönlendir
+ header("Location: login.php?message=account_deleted");
+ exit;
+ } catch (Exception $e) {
+ $db->rollBack();
+ $delete_error_message = "Hesap silinirken bir hata oluştu. Lütfen daha sonra tekrar deneyin.";
+ }
+ }
+ }
+}
+?>
+
+
+
+
+
+
+
Güvenlik Ayarları - Artado Developers
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
Hesap Ayarları
+
Hesabınızın güvenlik ayarlarını değiştirebilirsiniz.
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/devs/user/announcements.php b/devs/user/announcements.php
new file mode 100644
index 0000000..9cebbc3
--- /dev/null
+++ b/devs/user/announcements.php
@@ -0,0 +1,294 @@
+query("
+ SELECT a.*, u.username, u.profile_photo
+ FROM announcements a
+ LEFT JOIN users u ON a.user_id = u.id
+ ORDER BY a.created_at DESC
+");
+$announcements = $stmt->fetchAll(PDO::FETCH_ASSOC);
+?>
+
+
+
+
+
+
+
Duyurular - Artado Developers
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
Duyurular
+
Artado Developers duyurularını buradan takip edebilirsiniz.
+
+
+
+
+
+
+
+
+ prepare("SELECT username, profile_photo FROM users WHERE id = :user_id");
+ $stmt->bindParam(':user_id', $announcement['user_id']);
+ $stmt->execute();
+ $user = $stmt->fetch(PDO::FETCH_ASSOC);
+
+ // Eğer kullanıcı bulunamadıysa varsayılan değerleri kullan
+ $username = $user ? $user['username'] : 'Artado Developers';
+ $profile_photo = ($user && $user['profile_photo']) ? $user['profile_photo'] : $default_profile_photo;
+ ?>
+
+
+
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/devs/user/assets.zip b/devs/user/assets.zip
new file mode 100644
index 0000000..d628c15
Binary files /dev/null and b/devs/user/assets.zip differ
diff --git a/devs/user/auth-forgot-password.php b/devs/user/auth-forgot-password.php
new file mode 100644
index 0000000..eb3ffd8
--- /dev/null
+++ b/devs/user/auth-forgot-password.php
@@ -0,0 +1,53 @@
+
+
+
+
+
+
+
Şifremi Unuttum - Artado Developers
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+

+
+
Şifremi Unuttum
+
E-posta adresinizi girin, size şifre sıfırlama bağlantısı gönderelim.
+
+
+
+
Hesabınızı hatırladınız mı? Giriş Yap
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/devs/user/auth-login.php b/devs/user/auth-login.php
new file mode 100644
index 0000000..040cb9c
--- /dev/null
+++ b/devs/user/auth-login.php
@@ -0,0 +1,176 @@
+
+
+
+
+
+
+
+
+
Giriş Yap - Artado Developers
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+

+
+
Giriş Yap
+
Kayıt olurken kullandığınız bilgileriniz ile giriş yapın.
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
Hesabınız yok mu? Kayıt Ol
+
Şifremi Unuttum
+
+
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/devs/user/auth-register.php b/devs/user/auth-register.php
new file mode 100644
index 0000000..13e7d3f
--- /dev/null
+++ b/devs/user/auth-register.php
@@ -0,0 +1,188 @@
+prepare("INSERT INTO users (username, email, password, website) VALUES (:username, :email, :password, :website)");
+ $stmt->bindParam(':username', $username);
+ $stmt->bindParam(':email', $email);
+
+ $hashed_password = password_hash($password, PASSWORD_DEFAULT);
+ $stmt->bindParam(':password', $hashed_password);
+ $stmt->bindParam(':website', $website);
+
+ $stmt->execute();
+
+ $success_message = "Kayıt başarılı! Giriş yapabilirsiniz.";
+ header("Location: auth-login.php?registered=true");
+ exit();
+ } catch (PDOException $e) {
+ if ($e->getCode() == 23000) {
+ $error_message = "Bu kullanıcı adı veya e-posta adresi zaten kullanılıyor.";
+ } else {
+ // Hata mesajını detaylı göster
+ $error_message = "Kayıt sırasında bir hata oluştu: " . $e->getMessage();
+ // Geliştirme ortamında hata detaylarını görmek için:
+ error_log("Database Error: " . $e->getMessage());
+ }
+ }
+ }
+}
+?>
+
+
+
+
+
+
+
Register - Mazer Admin Dashboard
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+

+
+
Kayıt Ol
+
Hesap oluşturmak için bilgilerinizi giriniz.
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
Zaten hesabınız var mı? Giriş Yap
+
+
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/devs/user/create-eklenti.php b/devs/user/create-eklenti.php
new file mode 100644
index 0000000..f704525
--- /dev/null
+++ b/devs/user/create-eklenti.php
@@ -0,0 +1,373 @@
+prepare("INSERT INTO projects (user_id, title, description, version, features, file_path, category)
+ VALUES (:user_id, :title, :description, :version, :features, :file_path, :category)");
+ $stmt->bindParam(':user_id', $user_id);
+ $stmt->bindParam(':title', $title);
+ $stmt->bindParam(':description', $description);
+ $stmt->bindParam(':version', $version);
+ $stmt->bindParam(':features', $features);
+ $stmt->bindParam(':file_path', $target_file);
+ $stmt->bindParam(':category', $category);
+ $stmt->execute();
+
+ // Proje resmi yükleme
+ $project_id = $db->lastInsertId();
+ $image_upload_result = uploadProjectImage($project_id, $db);
+ if ($image_upload_result !== true) {
+ $error = $image_upload_result;
+ } else {
+ $_SESSION['success_message'] = "Eklenti başarıyla oluşturuldu.";
+ header("Location: projects.php?category=artado_eklenti");
+ exit();
+ }
+ } catch (PDOException $e) {
+ $error = "Veritabanı hatası: " . $e->getMessage();
+ }
+ } else {
+ $error = "Dosya taşınırken bir hata oluştu.";
+ }
+ } else {
+ switch ($fileError) {
+ case UPLOAD_ERR_INI_SIZE:
+ $error = "Dosya boyutu php.ini dosyasında belirlenen 'upload_max_filesize' değerini aşıyor.";
+ break;
+ case UPLOAD_ERR_FORM_SIZE:
+ $error = "Dosya boyutu HTML formunda belirlenen MAX_FILE_SIZE direktifini aşıyor.";
+ break;
+ case UPLOAD_ERR_PARTIAL:
+ $error = "Dosya yalnızca kısmen yüklendi.";
+ break;
+ case UPLOAD_ERR_NO_FILE:
+ $error = "Hiçbir dosya yüklenmedi.";
+ break;
+ case UPLOAD_ERR_NO_TMP_DIR:
+ $error = "Geçici klasör eksik.";
+ break;
+ case UPLOAD_ERR_CANT_WRITE:
+ $error = "Diske yazma başarısız oldu.";
+ break;
+ case UPLOAD_ERR_EXTENSION:
+ $error = "PHP uzantısı dosya yüklemeyi durdurdu.";
+ break;
+ default:
+ $error = "Bilinmeyen bir hata oluştu (Hata kodu: $fileError).";
+ }
+ }
+ } else {
+ $error = "Lütfen bir proje dosyası seçin.";
+ }
+}
+?>
+
+
+
+
+
+
+
+
Eklenti Oluştur - Artado Developers
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
Önizleme
+
+
+
+
+
+
+
+
+
+
+
+
Toplam Proje Sayısı
+
+
+
+
+
+
+
+
+
+
+
+
+
Toplam Kullanıcı Sayısı
+
+
+
+
+
+
+
+
+
+
+
+
+
+
Proje görüntülemem
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ query("SELECT p.title, u.username FROM projects p JOIN users u ON p.user_id = u.id ORDER BY p.created_at DESC LIMIT 5");
+ $recent_projects = $stmt->fetchAll(PDO::FETCH_ASSOC);
+ ?>
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ İsim |
+ İçeriği |
+
+
+
+
+
+
+
+ 
+
+ Artado
+
+ |
+
+ Duyuru
+ |
+
+
+
+
+
+ 
+
+ Artado
+
+ |
+
+ Duyuru
+ |
+
+
+
+
+
+ 
+
+ Artado
+
+ |
+
+ Duyuru
+ |
+
+
+
+
+
+ 
+
+ Artado
+
+ |
+
+ Duyuru
+ |
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+

+
+
+
+
+
+
+
+
+
+
+
+
+
+
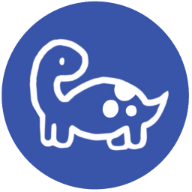
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/devs/user/login.php b/devs/user/login.php
new file mode 100644
index 0000000..19ab0f8
--- /dev/null
+++ b/devs/user/login.php
@@ -0,0 +1,552 @@
+
+
+
+
+
+
+
+
+
Giriş Yap
+
+
+
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/devs/user/projects.php b/devs/user/projects.php
new file mode 100644
index 0000000..920e03f
--- /dev/null
+++ b/devs/user/projects.php
@@ -0,0 +1,553 @@
+prepare("
+ SELECT p.*, u.username, u.profile_photo, pi.image_path
+ FROM projects p
+ LEFT JOIN users u ON p.user_id = u.id
+ LEFT JOIN project_images pi ON p.id = pi.project_id
+ WHERE p.user_id = :user_id
+ $category_filter
+ ORDER BY p.upload_date DESC
+");
+$stmt->bindParam(':user_id', $user_id);
+if (isset($_GET['category'])) {
+ $stmt->bindParam(':category', $category);
+}
+$stmt->execute();
+$projects = $stmt->fetchAll(PDO::FETCH_ASSOC);
+
+// Kategori adlarını Türkçe'ye çeviren fonksiyon
+function getCategoryName($category) {
+ $categories = [
+ 'pc_oyun' => 'PC Oyunu',
+ 'mobil_uygulama' => 'Mobil Uygulama',
+ 'pc_uygulama' => 'PC Uygulaması',
+ 'artado_eklenti' => 'Artado Eklentisi',
+ 'artado_tema' => 'Artado Teması',
+ 'artado_logo' => 'Artado Logo'
+ ];
+ return isset($categories[$category]) ? $categories[$category] : $category;
+}
+?>
+
+
+
+
+
+
+
Projelerim - Artado Developers
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
Projelerim
+
Projelerinizi buradan yönetebilirsiniz.
+
+
+
+
+
+
+
+
+
+
+ 0): ?>
+
+
+
+
+
+
+
+
+
+
+
![<?php echo htmlspecialchars($project['title']); ?>](<?php echo htmlspecialchars($project['image_path']); ?>)
+
+

+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ Özellikler:
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ Henüz hiç projeniz bulunmuyor. Yeni bir proje eklemek için "Proje Oluştur" menüsünü kullanabilirsiniz.
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/devs/user/update_project.php b/devs/user/update_project.php
new file mode 100644
index 0000000..bea3529
--- /dev/null
+++ b/devs/user/update_project.php
@@ -0,0 +1,132 @@
+prepare("SELECT user_id FROM projects WHERE id = :project_id");
+ $stmt->bindParam(':project_id', $project_id);
+ $stmt->execute();
+ $project = $stmt->fetch(PDO::FETCH_ASSOC);
+
+ if ($project && $project['user_id'] == $_SESSION['user_id']) {
+ // Proje bilgilerini güncelle
+ $stmt = $db->prepare("
+ UPDATE projects
+ SET title = :title,
+ category = :category,
+ description = :description,
+ features = :features,
+ updated_at = NOW()
+ WHERE id = :project_id
+ ");
+
+ $stmt->bindParam(':project_id', $project_id);
+ $stmt->bindParam(':title', $title);
+ $stmt->bindParam(':category', $category);
+ $stmt->bindParam(':description', $description);
+ $stmt->bindParam(':features', $features);
+ $stmt->execute();
+
+ // Eğer yeni bir resim yüklendiyse
+ if (isset($_FILES['image']) && $_FILES['image']['error'] === UPLOAD_ERR_OK) {
+ $image = $_FILES['image'];
+ $image_path = 'uploads/project_images/' . uniqid() . '_' . basename($image['name']);
+
+ // Uploads klasörü yoksa oluştur
+ if (!file_exists('uploads/project_images')) {
+ mkdir('uploads/project_images', 0777, true);
+ }
+
+ // Resmi yükle
+ if (move_uploaded_file($image['tmp_name'], $image_path)) {
+ // Eski resmi sil
+ $stmt = $db->prepare("SELECT image_path FROM project_images WHERE project_id = :project_id");
+ $stmt->bindParam(':project_id', $project_id);
+ $stmt->execute();
+ $old_image = $stmt->fetch(PDO::FETCH_ASSOC);
+
+ if ($old_image && file_exists($old_image['image_path'])) {
+ unlink($old_image['image_path']);
+ }
+
+ // Yeni resim yolunu veritabanına kaydet
+ $stmt = $db->prepare("
+ INSERT INTO project_images (project_id, image_path)
+ VALUES (:project_id, :image_path)
+ ON DUPLICATE KEY UPDATE image_path = :image_path
+ ");
+ $stmt->bindParam(':project_id', $project_id);
+ $stmt->bindParam(':image_path', $image_path);
+ $stmt->execute();
+ }
+ }
+
+ // Eğer yeni bir proje dosyası yüklendiyse
+ if (isset($_FILES['project_file']) && $_FILES['project_file']['error'] === UPLOAD_ERR_OK) {
+ $file = $_FILES['project_file'];
+
+ // Dosya boyutunu kontrol et (100MB)
+ if ($file['size'] > 100 * 1024 * 1024) {
+ throw new Exception("Dosya boyutu 100MB'dan büyük olamaz.");
+ }
+
+ // Dosya uzantısını kontrol et
+ $allowed_extensions = ['zip', 'rar', '7z'];
+ $file_extension = strtolower(pathinfo($file['name'], PATHINFO_EXTENSION));
+ if (!in_array($file_extension, $allowed_extensions)) {
+ throw new Exception("Sadece ZIP, RAR ve 7Z dosyaları yüklenebilir.");
+ }
+
+ $file_path = 'uploads/project_files/' . uniqid() . '_' . basename($file['name']);
+
+ // Uploads klasörü yoksa oluştur
+ if (!file_exists('uploads/project_files')) {
+ mkdir('uploads/project_files', 0777, true);
+ }
+
+ // Dosyayı yükle
+ if (move_uploaded_file($file['tmp_name'], $file_path)) {
+ // Eski dosyayı sil
+ $stmt = $db->prepare("SELECT project_file FROM projects WHERE id = :project_id");
+ $stmt->bindParam(':project_id', $project_id);
+ $stmt->execute();
+ $old_file = $stmt->fetch(PDO::FETCH_ASSOC);
+
+ if ($old_file && !empty($old_file['project_file']) && file_exists($old_file['project_file'])) {
+ unlink($old_file['project_file']);
+ }
+
+ // Yeni dosya yolunu veritabanına kaydet
+ $stmt = $db->prepare("UPDATE projects SET project_file = :file_path WHERE id = :project_id");
+ $stmt->bindParam(':project_id', $project_id);
+ $stmt->bindParam(':file_path', $file_path);
+ $stmt->execute();
+ }
+ }
+
+ $_SESSION['success_message'] = "Proje başarıyla güncellendi.";
+ } else {
+ $_SESSION['error_message'] = "Bu projeyi düzenleme yetkiniz yok.";
+ }
+ } catch (Exception $e) {
+ $_SESSION['error_message'] = "Proje güncellenirken bir hata oluştu: " . $e->getMessage();
+ }
+
+ header("Location: projects.php");
+ exit();
+}
+?>
\ No newline at end of file
diff --git a/devs/user/uploads/profile_photos/profile_67798e81a39e9.png b/devs/user/uploads/profile_photos/profile_67798e81a39e9.png
new file mode 100644
index 0000000..9a5dfdb
Binary files /dev/null and b/devs/user/uploads/profile_photos/profile_67798e81a39e9.png differ
diff --git a/devs/web.config b/devs/web.config
new file mode 100644
index 0000000..a39ef81
--- /dev/null
+++ b/devs/web.config
@@ -0,0 +1,31 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+