|
| 1 | +# Sample Go Base Code (gRPC) |
| 2 | + |
| 3 | +[](https://clsframework.github.io/docs/introduction/) |
| 4 | +[](https://opensource.org/licenses/MIT) |
| 5 | + |
| 6 | +This repository contains a sample decision-making server for the RoboCup 2D Soccer Simulation, which allows you to create a team by using Go. This server is compatible with the [Cross Language Soccer Framework](https://arxiv.org/pdf/2406.05621). This server is written in Golang and uses gRPC to communicate with the [proxy](https://github.com/CLSFramework/soccer-simulation-proxy). |
| 7 | + |
| 8 | +The Soccer Simulation Server sends the observations to the proxy, which processes the data, create state message and sends it to the decision-making server. The decision-making server then sends the actions to the proxy, and then the proxy convert actions to the server commands and sends them to the server. |
| 9 | + |
| 10 | +For more information, please refer to the [documentation](https://clsframework.github.io/). |
| 11 | + |
| 12 | +You can find more information about the services and messages in the [IDL section](../../3-idl/protobuf.md). |
| 13 | + |
| 14 | +## Quick start |
| 15 | + |
| 16 | +### Preparation |
| 17 | + |
| 18 | +Install the pre-requisites using the command below: |
| 19 | + |
| 20 | +``` Bash |
| 21 | +sudo apt-get install fuse #Used to run AppImages |
| 22 | +``` |
| 23 | + |
| 24 | +Clone this repository & install the required Golang libraries (such as gRPC). Don't forget to activate your virtual environment! |
| 25 | + |
| 26 | +``` Bash |
| 27 | +git clone #add repo link |
| 28 | +cd sample-playmaker-server-go-grpc |
| 29 | + |
| 30 | +go mod tidy # Install the required Golang libraries |
| 31 | + |
| 32 | + |
| 33 | +./generate.sh # Generate the gRPC files |
| 34 | +``` |
| 35 | + |
| 36 | +To download RoboCup Soccer 2D Server using the commands below: |
| 37 | + |
| 38 | +``` Bash |
| 39 | +pushd scripts |
| 40 | +sh download-rcssserver.sh # Download the soccer simulation server |
| 41 | +popd |
| 42 | +``` |
| 43 | + |
| 44 | +Next, download the soccer proxy, which uses C++ to read and pre-processes state data and passes them to the Golang server (this project) for decision-making. |
| 45 | + |
| 46 | +``` Bash |
| 47 | +pushd scripts |
| 48 | +sh download-proxy.sh #install C++ proxy |
| 49 | +popd |
| 50 | +``` |
| 51 | + |
| 52 | +Finally, to watch the game, download the monitor from [the original repository](https://github.com/rcsoccersim/rcssmonitor/releases) in order to view the games. |
| 53 | + |
| 54 | +### Running a game |
| 55 | + |
| 56 | +This section assumes you have installed the server & proxy using the scripts (as mentioned above) |
| 57 | +We must first run a RoboCup Server, in order to host the game: |
| 58 | + |
| 59 | +``` Bash |
| 60 | +cd scripts/rcssserver |
| 61 | +./rcssserver |
| 62 | +``` |
| 63 | + |
| 64 | +Then we must run the proxy & the decisionmaking server: |
| 65 | + |
| 66 | +``` Bash |
| 67 | +./start-team.sh |
| 68 | +``` |
| 69 | + |
| 70 | +### Options |
| 71 | + |
| 72 | +- `-t team_name`: Specify the team name. |
| 73 | +- `--rpc-port PORT`: Specify the RPC port (default: 50051). |
| 74 | +- `-d`: Enable debug mode. |
| 75 | + |
| 76 | + |
| 77 | +Launch the opponent team, start the monitor app image. press <kbd>Ctrl</kbd> + <kbd>C</kbd> to connect to the server, and <kbd>Ctrl</kbd> + <kbd>K</kbd> for kick-off! |
| 78 | + |
| 79 | +### Tutorial Video (English) |
| 80 | + |
| 81 | +[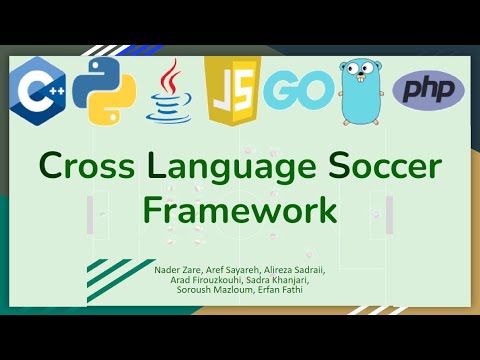](https://www.youtube.com/watch?v=hH-5rkhiQHg) |
| 82 | + |
| 83 | +### Tutorial Video (Persian) |
| 84 | + |
| 85 | +[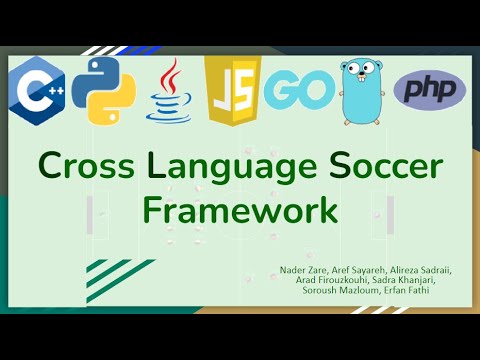](https://www.youtube.com/watch?v=97YDEumcVWU&t=0s) |
| 86 | + |
| 87 | +## How to change the code |
| 88 | + |
| 89 | +The `server.go` file contains the logic in 3 main functions: |
| 90 | +`GetPlayerActions` receives a game state, and returns a list of actions for a player for for that cycle. |
| 91 | +The actions we can output are equivalent to the Helios Base (Proxy), which are abstracted into multiple levels. |
| 92 | +You can use actions such as `DoDash`, `DoTurn`, `DoKick` which directly apply force, or use actions such as `GoToPoint`, `SmartKick`, `Shoot` or [more](https://clsframework.github.io/docs/idl/). |
| 93 | + |
| 94 | +Similarly, you can change `GetCoachActions` which is responsible for coach communication & substitutions. |
| 95 | + |
| 96 | +You can also use `GetTrainerActions` to move the players & the ball to make repeatable scenarios (when the server is in trainer mode). |
| 97 | + |
| 98 | +## Why & How it works |
| 99 | + |
| 100 | +Originally the RoboCup 2D Soccer Simulation teams used C++, as the main code base (Agent2D aka Helios Base) was written in this language due to its performance. |
| 101 | +Due to the popularity of Golang we decided to create a Golang platform which would be equivalent to Agent 2D. |
| 102 | +However, using Golang alone was too slow as preprocessing sensor information & tasks such as localization took too long. |
| 103 | + |
| 104 | +For this reason we have split up the code into two segments: |
| 105 | +The data processing section in proxy, which creates a World Model (state), and passes it to Golang for planning to occur. This repository uses gRPC to pass along the World Model, but there is a sister-repo which is compatible with thrift. |
| 106 | + |
| 107 | +```mermaid |
| 108 | +sequenceDiagram |
| 109 | + participant SS as SoccerSimulationServer |
| 110 | + participant SP as SoccerSimulationProxy |
| 111 | + participant PM as PlayMakerServer |
| 112 | + Note over SS,PM: Run |
| 113 | + SP->>SS: Connect |
| 114 | + SS->>SP: OK, Unum |
| 115 | + SP->>PM: Register |
| 116 | + PM->>SP: OK, ClientID |
| 117 | + SS->>SP: Observation |
| 118 | + Note over SP: Convert observation to State |
| 119 | + SP->>PM: State |
| 120 | + PM->>SP: Actions |
| 121 | + Note over SP: Convert Actions to Low-Level Commands |
| 122 | + SP->>SS: Commands |
| 123 | +``` |
| 124 | + |
| 125 | + |
| 126 | +As seen in the figure, the proxy handles connecting to the server, receiving sensor information and creating a world-model, and finds the action to take via a remote procedure call to a decision-making server, which is this repository. |
| 127 | + |
| 128 | +## Configuration |
| 129 | + |
| 130 | +### RoboCup Server configuration |
| 131 | + |
| 132 | +You can change the configuration of the RoboCup server and change parameters such as players' stamina, game length, field length, etc. by modifying `~/.rcssserver/server.conf`. Refer to the server's documents and repo for a more detailed guide. |
| 133 | + |
| 134 | +### Modifying Proxy & Running proxy and server seperately |
| 135 | + |
| 136 | +If you want to modify the algorithms of the base (such as ball interception, shooting, localization, etc.) you must modify the code of the [proxy repo](https://github.com/CLSFramework/soccer-simulation-proxy). After re-building from source, you can run the proxy by using `./start.sh --rpc-type grpc` in the bin folder of the proxy, and run the gRPC server with `go run server.go` in this repo's directory. It is highly recommended to launch the Golang server before the proxy. |
| 137 | + |
| 138 | +You can modify the rpc port by adding the argument `--rpc-port [VALUE]`, where the default is 50051. |
| 139 | + |
| 140 | +## Citation |
| 141 | + |
| 142 | +- [Cross Language Soccer Framework](https://arxiv.org/pdf/2406.05621) |
| 143 | +- Zare, N., Sayareh, A., Sadraii, A., Firouzkouhi, A. and Soares, A., 2024. Cross Language Soccer Framework |
0 commit comments