-
Notifications
You must be signed in to change notification settings - Fork 33
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
trying to match tf.nn.conv2d vs keras2c #14
Comments
The output was generated using conda with tensorflow cpu 2.3.0 vs the master branch of k2c on windows. |
I was able to match the k2c conv2d with keras. The trick is to specify the padding and the size of the padded image according to the rules in https://www.tensorflow.org/api_docs/python/tf/nn#notes_on_padding_2. The code below has the modification:
And the resulting images look as follows: The code in weights2c.py seems to have the computations for the padding. But they don't look the same as the notes in the link above. |
I've been having issues matching the output of conv2d from keras to the output of keras2c. I've been trying to unit test the k2c_conv2d vs the output of tf.nn.conv2d.
Below is the code that I used to generate my "truth" using tf.nn.conv2d. I have an 80x80 chip of ones convolved with a 7x7 kernel of ones. The script test_conv2d.py produces two outputs. The first output is the result of the convolution using a stride of 1 and the second output is the result of the convolution using a stride of 2. In the first case, the output of the first 3 rows is not 49. This makes sense since the mask goes outside the image and with padding set to 'same', the assumed value outside the image is assumed zero. In the second case, the first row and the last two rows is not 49 as expected.
I'm assuming that in order to match with keras2c, I need to pad the image to an 86x86 (by adding 3 rows and columns on each side set to zero), then convolve the padded image to generate an output image of 80x80 for stride 1 and 40x40 for stride 2.
Below is the C code that I used to perform the convolution in k2c.
I generated the resulting chips using these commands:
Then used the following script to compare the output from tensorflow vs k2c:
Attached is the image that I generated.
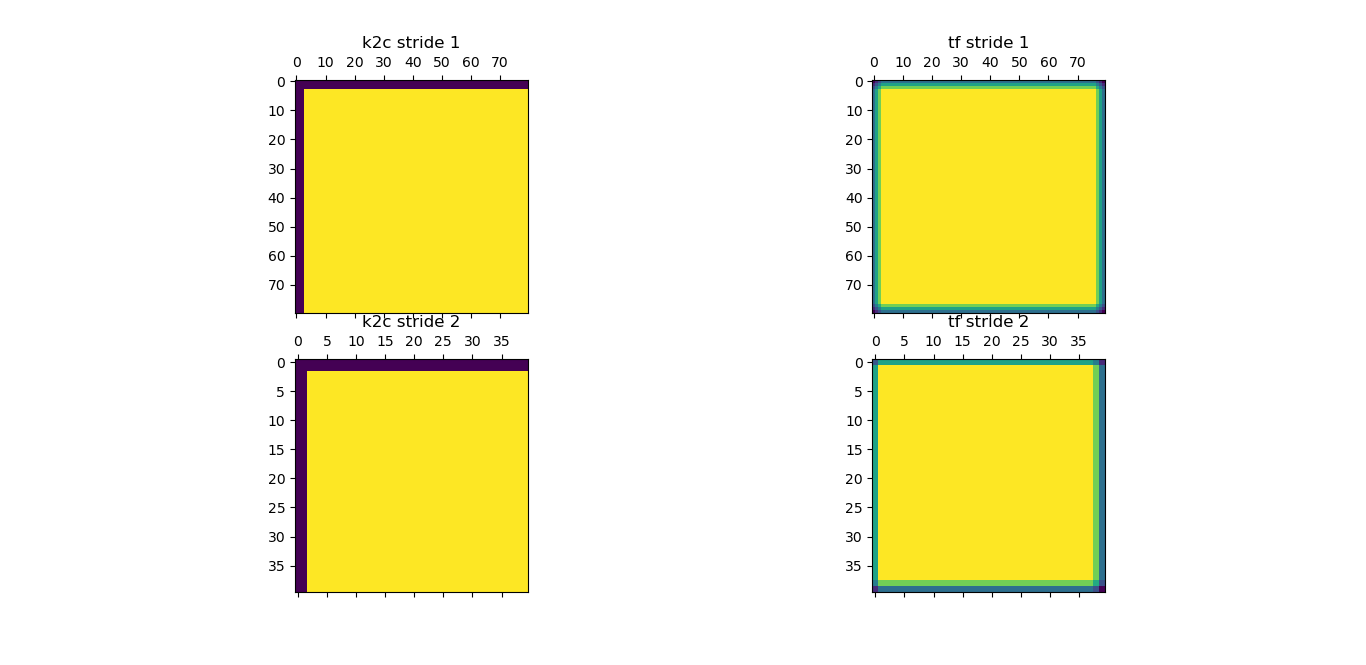
First, notice there is not gradient in the output of k2c. Second, pixel (2,2) is the first value on the k2c convolution with stride 2 with value 49 vs pixel(1,1) from the output of tf with stride 2.
I maybe simulating the tf.nn.conv2d incorrectly in C. Feedback in how to do it correctly is welcomed. But I think the shift of the stride is still different.
Your help will be greatly appreciated
The text was updated successfully, but these errors were encountered: