diff --git a/README.md b/README.md
new file mode 100644
index 0000000..37f2c1b
--- /dev/null
+++ b/README.md
@@ -0,0 +1 @@
+# windows-11_interface
\ No newline at end of file
diff --git a/components/application.css b/components/application.css
new file mode 100644
index 0000000..b2d4663
--- /dev/null
+++ b/components/application.css
@@ -0,0 +1,151 @@
+.application-box {
+ width: 100%;
+ height: calc(100vh - 60px);
+ display: flex;
+ flex-wrap: wrap;
+}
+
+.application {
+ width: 50%;
+ height: 50%;
+ box-shadow: 0 0 0 0.5px white;
+}
+
+.title-bar {
+ width: 100%;
+ height: 40px;
+ display: flex;
+ justify-content: space-between;
+ color: white;
+ background-color: black;
+}
+
+.app-name {
+ width: 100px;
+ height: 100%;
+ text-align: center;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.operation-box {
+ width: 120px;
+ height: 100%;
+ display: flex;
+ justify-content: flex-end;
+}
+
+.test {
+ width: calc(100% - 219px);
+ height: 40px;
+ min-width: 40px;
+ min-height: 40px;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.minimize-box {
+ width: 40px;
+ height: 40px;
+ min-width: 40px;
+ min-height: 40px;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.minimize-box:hover {
+ background-color: rgb(97, 94, 94);
+}
+
+.minimize-icon {
+ width: 22px;
+}
+
+.maximize-box {
+ width: 40px;
+ height: 40px;
+ min-width: 40px;
+ min-height: 40px;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.maximize-box:hover {
+ background-color: rgb(97, 94, 94);
+}
+
+.maximize-icon {
+ width: 15px;
+}
+
+.close-box {
+ width: 40px;
+ height: 40px;
+ min-width: 40px;
+ min-height: 40px;
+ background-color: rgb(167, 5, 5);
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.close-box:hover {
+ background-color: red;
+}
+
+.close-icon {
+ width: 15px;
+}
+
+.app-page {
+ width: 100%;
+ height: calc(100% - 40px);
+}
+
+.iframe {
+ width: 100%;
+ height: 100%;
+ background-color: white;
+}
+
+/* ********************** alert window ****************** */
+
+.alert-window-box {
+ width: 300px;
+ height: 100px;
+ padding-top: 20px;
+ background-color: rgb(230, 211, 188);
+ border: 2px solid black;
+ box-shadow: 0 0 0 1px white;
+ border-radius: 9px;
+ position: fixed;
+ top: 40%;
+ right: 45%;
+ display: flex;
+ flex-direction: column;
+ justify-content: center;
+ align-items: center;
+}
+
+.alert-message {
+ color: black;
+}
+
+.alert-button {
+ width: 40%;
+ height: 20px;
+ padding: 20px;
+ margin: 20px;
+ color: white;
+ background-color: rgb(236, 79, 79);
+ border: 2px solid black;
+ box-shadow: 0 0 0 1px white;
+ border-radius: 9px;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
\ No newline at end of file
diff --git a/components/application.js b/components/application.js
new file mode 100644
index 0000000..f69b809
--- /dev/null
+++ b/components/application.js
@@ -0,0 +1,180 @@
+let noOfApplicatons = 0;
+console.log(window.matchMedia)
+
+function applicationLayoutManager() {
+ let application = document.getElementsByClassName('application');
+
+ if (noOfApplicatons <= 2) {
+ for (let element of application) {
+ element.style.height = '100%';
+ }
+ }
+ else if (noOfApplicatons <= 4) {
+ for (let element of application) {
+ element.style.height = '50%';
+ }
+ }
+ else if (noOfApplicatons > 4) {
+ for (let element of application) {
+ element.style.width = '33%';
+ }
+ }
+}
+
+function nothingToDo() {
+ console.log('nothing for do');
+}
+
+function createApplication(text, url) {
+ if (noOfApplicatons >= 6) {
+ createAlertWindow();
+ }
+ else {
+ let randomId = (Math.random() + 1).toString(36).substring(7);
+
+ let applicatonBox = document.getElementById('applicationBox');
+ let applicaton = document.createElement('div');
+ applicaton.id = randomId;
+ let titleBar = document.createElement('div');
+ let appName = document.createElement('div');
+ appName.onclick = function () {
+ maximizeWindow(randomId);
+ }
+ let test = document.createElement('div');
+ test.onclick = function () {
+ maximizeWindow(randomId);
+ }
+ let operationBox = document.createElement('div');
+ let minimizeBox = document.createElement('div');
+ minimizeBox.onclick = function () {
+ minimizeWindow(randomId);
+ }
+ let minimizeIcon = document.createElement('img');
+ let maximizeBox = document.createElement('div')
+ maximizeBox.onclick = function () {
+ maximizeWindow(randomId);
+ }
+ let maximizeIcon = document.createElement('img')
+ let closeBox = document.createElement('div')
+ closeBox.onclick = function () {
+ deleteWindow(randomId);
+ }
+ let closeIcon = document.createElement('img')
+ let appPage = document.createElement('div')
+ let ifarme = document.createElement('iframe')
+
+ applicaton.classList.add('application');
+ titleBar.classList.add('title-bar');
+ appName.classList.add('app-name');
+ operationBox.classList.add('operation-box');
+ test.classList.add('test');
+ minimizeBox.classList.add('minimize-box');
+ minimizeIcon.classList.add('minimize-icon');
+ maximizeBox.classList.add('maximize-box');
+ maximizeIcon.classList.add('maximize-icon');
+ closeBox.classList.add('close-box');
+ closeIcon.classList.add('close-icon');
+ appPage.classList.add('app-page');
+ ifarme.classList.add('iframe');
+
+ minimizeIcon.src = 'images/minimize_icon.png';
+ maximizeIcon.src = 'images/maximize_icon.png';
+ closeIcon.src = 'https://static-00.iconduck.com/assets.00/cross-icon-2048x2048-vz9m0pj3.png';
+
+ noOfApplicatons = noOfApplicatons + 1;
+
+ minimizeBox.appendChild(minimizeIcon);
+ maximizeBox.appendChild(maximizeIcon);
+ closeBox.appendChild(closeIcon);
+ operationBox.appendChild(minimizeBox);
+ operationBox.appendChild(maximizeBox);
+ operationBox.appendChild(closeBox);
+ titleBar.appendChild(appName);
+ titleBar.appendChild(test);
+ titleBar.appendChild(operationBox);
+ appPage.appendChild(ifarme);
+ applicaton.appendChild(titleBar);
+ applicaton.appendChild(appPage);
+ applicatonBox.appendChild(applicaton);
+
+ appName.textContent = text;
+ ifarme.src = url;
+ ifarme.style.border = '0';
+
+ applicationLayoutManager();
+ }
+}
+
+function createAlertWindow() {
+ let alertWindowBox = document.createElement('div');
+ let alertmessage = document.createElement('div');
+ let alertbutton = document.createElement('div');
+ alertbutton.addEventListener('click', deleteAlertWindow);
+
+ alertWindowBox.id = "alertWindowBox";
+ alertWindowBox.classList.add('alert-window-box');
+ alertmessage.classList.add('alert-messag');
+ alertbutton.classList.add('alert-button');
+
+ alertmessage.textContent = "Can't open more then 6 application";
+ alertbutton.textContent = "OK";
+
+ alertWindowBox.appendChild(alertmessage);
+ alertWindowBox.appendChild(alertbutton);
+
+ let applicatonBox = document.getElementById('applicationBox');
+ applicatonBox.appendChild(alertWindowBox);
+}
+
+function deleteAlertWindow() {
+ var windowFrame = document.getElementById("alertWindowBox");
+ console.log(windowFrame);
+ windowFrame.remove();
+}
+
+let fullScreenCheck = false;
+function minimizeWindow(idName) {
+ if (fullScreenCheck == true) {
+ if (document.exitFullscreen) {
+ document.exitFullscreen();
+ } else if (document.webkitExitFullscreen) { /* Safari */
+ document.webkitExitFullscreen();
+ } else if (document.msExitFullscreen) { /* IE11 */
+ document.msExitFullscreen();
+ }
+ fullScreenCheck = false;
+ }
+
+ let fullScreen = document.getElementById('fullScreen');
+ if (fullScreen.requestFullscreen) {
+ fullScreen.requestFullscreen();
+ }
+ else if (fullScreen.webkitRequestFullscreen) { /* Safari */
+ fullScreen.webkitRequestFullscreen();
+ }
+ else if (fullScreen.msRequestFullscreen) { /* IE11 */
+ fullScreen.msRequestFullscreen();
+ }
+}
+
+function maximizeWindow(idName) {
+ let appFullScreen = document.getElementById(idName)
+
+ if (appFullScreen.requestFullscreen) {
+ appFullScreen.requestFullscreen();
+ }
+ else if (appFullScreen.webkitRequestFullscreen) { /* Safari */
+ appFullScreen.webkitRequestFullscreen();
+ }
+ else if (appFullScreen.msRequestFullscreen) { /* IE11 */
+ appFullScreen.msRequestFullscreen();
+ }
+ fullScreenCheck = true;
+}
+
+function deleteWindow(idName) {
+ var windowFrame = document.getElementById(idName);
+ windowFrame.remove();
+
+ noOfApplicatons = noOfApplicatons - 1;
+}
\ No newline at end of file
diff --git a/components/apps/chrome/chrome.css b/components/apps/chrome/chrome.css
new file mode 100644
index 0000000..d6d2dac
--- /dev/null
+++ b/components/apps/chrome/chrome.css
@@ -0,0 +1,73 @@
+* {
+ padding: 0px;
+ margin: 0px;
+ list-style: none;
+ text-decoration: none;
+ box-sizing: border-box;
+ user-select: none;
+ font-family:'Segoe UI';
+}
+
+.megacontainer {
+ width: 100%;
+ height: 100vh;
+ overflow: hidden;
+}
+
+.tool-bar-box {
+ width: calc(100% - 20px);
+ height: 20px;
+ padding: 13px;
+ color: white;
+ background-color: rgb(22, 231, 231);
+ box-sizing: content-box;
+ display: flex;
+ align-items: center;
+}
+
+.button-box {
+ width: 35px;
+ height: 35px;
+ padding: 5px;
+ margin-left: 5px;
+ border-radius: 100px;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.button-box:hover {
+ background-color: rgb(212, 212, 212);
+}
+
+.button-icon {
+ width: 20px;
+ height: 20px;
+}
+
+.search-bar-box {
+ width: 100%;
+ padding-left: 10px;
+ display: flex;
+ align-items: center;
+}
+
+.search-bar {
+ width: 80%;
+ height: 25px;
+ padding-left: 10px;
+ margin-left: 5px;
+ background-color: white;
+ box-shadow: 0 0 0 0.5px black;
+ border: 0px;
+ border-radius: 20px;
+}
+
+iframe {
+ width: 100%;
+ height: calc(100vh - 45px);
+ background-color: white;
+ border: 0px;
+
+}
+
diff --git a/components/apps/chrome/chrome.html b/components/apps/chrome/chrome.html
new file mode 100644
index 0000000..bed3504
--- /dev/null
+++ b/components/apps/chrome/chrome.html
@@ -0,0 +1,38 @@
+
+
+
+
+
+
+ Chrome
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/components/apps/chrome/chrome.js b/components/apps/chrome/chrome.js
new file mode 100644
index 0000000..9e32509
--- /dev/null
+++ b/components/apps/chrome/chrome.js
@@ -0,0 +1,53 @@
+let searchInput = document.querySelector('.search-bar');
+let iframe = document.querySelector('iframe');
+let searchHistoryUrl = [`https://www.google.com/search?q=&igu=1`];
+let searchHistoryQuery = [`https://www.google.com/search?q=&igu=1`];
+
+
+searchInput.addEventListener('keypress', function(event) {
+ if (event.key === 'Enter') {
+ searchCommand()
+ }
+});
+
+function searchCommand() {
+ // console.log(iframe);
+ searchQuery = searchInput.value;
+ let urlCheck = searchQuery.slice(0, 7);
+ console.log(searchHistoryUrl);
+
+ if (searchInput.value == searchHistoryUrl[searchHistoryUrl.length - 1] || searchInput.value == searchHistoryQuery[searchHistoryQuery.length - 1]) {
+ console.log('same ulr search');
+ }
+ else if (urlCheck == 'http://' || urlCheck == 'https:/') {
+ iframe.src = searchQuery;
+ searchHistoryUrl.push(searchQuery);
+ searchHistoryQuery.push(searchQuery);
+ }
+ else {
+ iframe.src = `https://www.google.com/search?q=${searchQuery}&igu=1`;
+ searchHistoryUrl.push(`https://www.google.com/search?q=${searchQuery}&igu=1`);
+ searchHistoryQuery.push(searchQuery);
+ }
+}
+
+function backButtonCommand() {
+ console.log('heloo backbut here!!!!!!!!!!');
+ console.log(searchHistoryUrl[searchHistoryUrl.length - 1]);
+ console.log(searchHistoryUrl[searchHistoryQuery.length - 1]);
+
+ if (searchHistoryUrl.length == 1) {
+ alert('you are at last!!!')
+ }
+ else {
+ iframe.src = searchHistoryUrl[searchHistoryUrl.length - 1];
+ searchInput.value = searchHistoryQuery[searchHistoryQuery.length - 1];
+ searchHistoryUrl.pop();
+ searchHistoryQuery.pop();
+ }
+
+}
+
+function refreshButtonCommand() {
+ iframe.src = iframe.src;
+}
\ No newline at end of file
diff --git a/components/apps/file_explorer/file_explorer.css b/components/apps/file_explorer/file_explorer.css
new file mode 100644
index 0000000..0922fcb
--- /dev/null
+++ b/components/apps/file_explorer/file_explorer.css
@@ -0,0 +1,271 @@
+* {
+ padding: 0px;
+ margin: 0px;
+ list-style: none;
+ text-decoration: none;
+ box-sizing: border-box;
+ user-select: none;
+ font-family: 'Segoe UI';
+ font-weight: 100;
+}
+
+.tool-bar-box {
+ width: 100%;
+ height: 55px;
+ color: white;
+ background-color: rgb(49, 49, 49);
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.button-box {
+ min-width: 30px;
+ min-height: 30px;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.button-icon {
+ width: 20px;
+ height: 20px;
+}
+
+#backButton {
+ transform: rotate(180deg);
+}
+
+.path-box {
+ width: calc(100% - 160px);
+ height: 30px;
+ background-color: rgb(89, 86, 86);
+ border-radius: 5px;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.path-show {
+ width: calc(100% - 10px);
+ height: 20px;
+ color: white;
+ background-color: inherit;
+ border: 0px;
+ font-size: 15px;
+ font-family: monospace;
+ overflow-x: hidden;
+ display: flex;
+ justify-content: flex-start;
+ align-items: center;
+}
+
+.search-bar {
+ width: 200px;
+ height: 30px;
+ padding-left: 5px;
+ margin: 0px 5px 0 10px;
+ color: white;
+ background-color: rgb(89, 86, 86);
+ border: 0px;
+ border-radius: 5px;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.search-bar::placeholder {
+ color: rgb(220, 220, 220);
+}
+
+.file-upload-box {
+ width: 100%;
+ height: 30px;
+ padding-left: 5px;
+ color: white;
+ background-color: rgb(30, 29, 29);
+ box-shadow: 0 0 0 1px gray;
+ display: flex;
+ justify-content: flex-start;
+ align-items: center;
+}
+
+.file-system {
+ width: 100%;
+ height: calc(100vh - 85px);
+ color: white;
+ background-color: rgb(30, 29, 29);
+ box-shadow: 0 0 0 1px gray;
+ display: flex;
+}
+
+.image-preview-box {
+ width: 100dvw;
+ height: calc(100% - 60px);
+ background-color: black;
+ box-shadow: 0 0 0 0.5px white;
+ position: fixed;
+ display: none;
+ flex-direction: column;
+ align-items: center;
+ overflow: auto;
+}
+
+.close {
+ width: 100%;
+ height: 17px;
+ background-color: black;
+ box-shadow: 0 0 0 0.5px white;
+ display: flex;
+ justify-content: flex-end;
+ align-items: center;
+}
+
+.close-icon {
+ width: 15px;
+}
+
+/* #imagePreview {
+
+} */
+
+.folder-navigation-box {
+ width: 10%;
+ min-width: 120px;
+ height: 100%;
+ box-shadow: 0 0 0 1px gray;
+}
+
+.folder-mini-box {
+ width: calc(100%- 30px);
+ height: 33px;
+ overflow: hidden;
+ white-space: nowrap;
+ box-sizing: content-box;
+ display: flex;
+ justify-content: space-between;
+ align-items: center;
+}
+
+.folder-mini-box:hover {
+ background-color: rgb(60, 59, 59);
+}
+
+.folder-icon-box {
+ width: 60px;
+ height: 20px;
+ box-sizing: content-box;
+ display: flex;
+ justify-content: flex-end;
+ align-items: center;
+}
+
+.folder-icon {
+ width: 20px;
+ height: 100%;
+ box-sizing: content-box;
+}
+
+.folder-name-box {
+ width: calc(100% - 35px);
+ font-size: 13px;
+ box-sizing: content-box;
+}
+
+.folder-content-box {
+ width: 90%;
+ height: 100%;
+ box-shadow: 0 0 0 1px gray;
+ overflow-x: hidden;
+}
+
+.label-bar-box {
+ width: 100%;
+ height: 30px;
+ display: flex;
+}
+
+.label-name-box {
+ /* width: 100px; */
+ height: 25px;
+ font-size: 12px;
+ padding-left: 10px;
+ border-right: 1px solid gray;
+ display: flex;
+ align-items: center;
+}
+
+.label-name-box:hover {
+ background-color: gray;
+}
+
+.folder-detail-box {
+ width: calc(100%- 440px);
+ height: 33px;
+ overflow: hidden;
+ white-space: nowrap;
+ box-sizing: content-box;
+ display: flex;
+ justify-content: flex-start;
+ align-items: center;
+}
+
+
+.folder-detail-box:hover {
+ background-color: rgb(60, 59, 59);
+}
+
+.folder-detail-icon-box {
+ width: 40px;
+ height: 20px;
+ box-sizing: content-box;
+ display: flex;
+ justify-content: flex-end;
+ align-items: center;
+}
+
+.folder-detail-name-box {
+ width: calc(200px - 30px);
+ padding-left: 5px;
+ font-size: 13px;
+ overflow-x: auto;
+}
+
+.folder-detail-name-box::-webkit-scrollbar {
+ display: none;
+}
+
+.date-modified-box {
+ width: 150px;
+ padding-left: 10px;
+ font-size: 12px;
+}
+
+.type-box {
+ width: 150px;
+ padding-left: 10px;
+ font-size: 12px;
+}
+
+.size-box {
+ width: 100px;
+ padding-left: 10px;
+ font-size: 12px;
+}
+
+iframe {
+ width: 100%;
+ height: 100vh;
+ background-color: white;
+ border: 0px;
+}
+
+@media only screen and (max-width: 500px) {
+ .folder-navigation-box {
+ display: none;
+ }
+
+ .folder-content-box {
+ width: 100%;
+ }
+}
\ No newline at end of file
diff --git a/components/apps/file_explorer/file_explorer.html b/components/apps/file_explorer/file_explorer.html
new file mode 100644
index 0000000..c2d1bb4
--- /dev/null
+++ b/components/apps/file_explorer/file_explorer.html
@@ -0,0 +1,193 @@
+
+
+
+
+
+
+ File Explorer
+
+
+
+
+
+
+
+
+
+
+
+
+
+
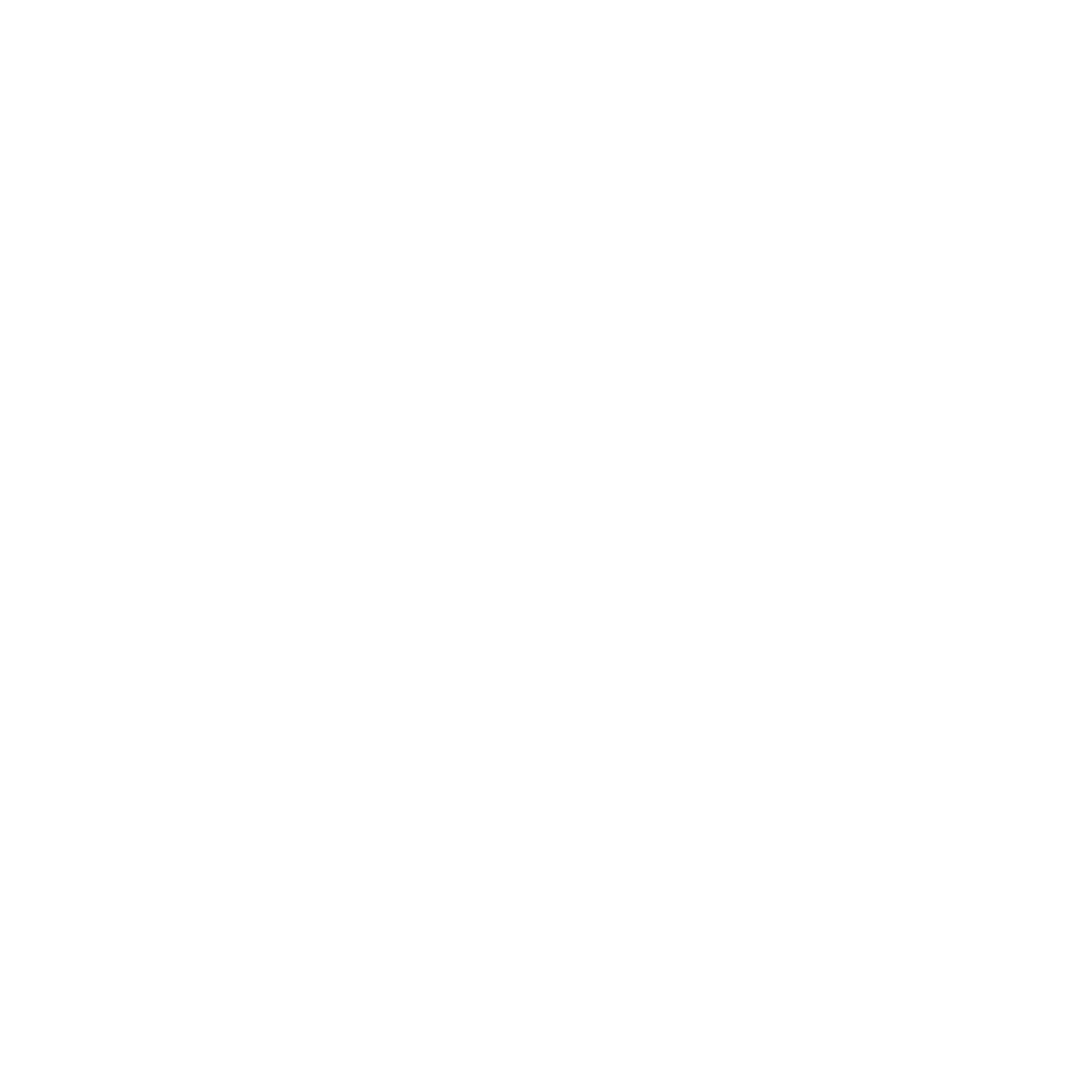
+
+

+
+
+
+
+
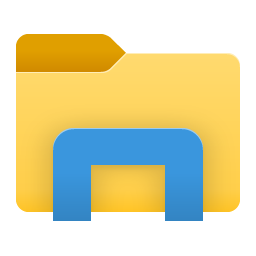
+
+
+ Home
+
+
+
+
+
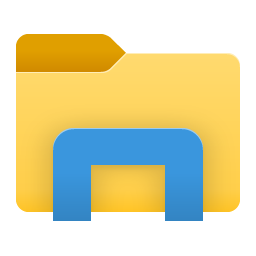
+
+
+ Photos
+
+
+
+
+
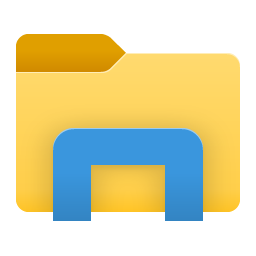
+
+
+ Videos
+
+
+
+
+
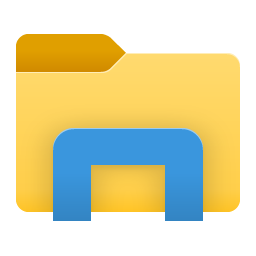
+
+
+ Document
+
+
+
+
+
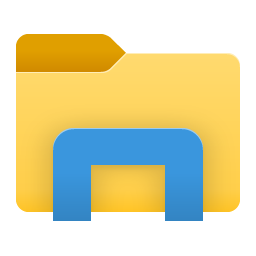
+
+
+ Download
+
+
+
+
+
+
+ Name
+
+
+ Date modified
+
+
+ Type
+
+
+ Size
+
+
+
+
+
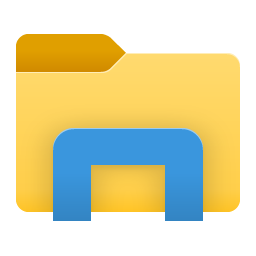
+
+
+
+ Python
+
+
+ 04-05-2024 06:08 PM
+
+
+ File Folder
+
+
+ 10GB
+
+
+
+
+
+
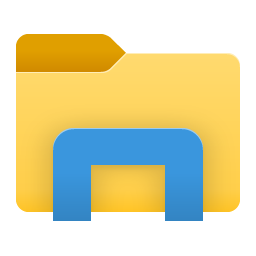
+
+
+
+ code-box
+
+
+ 04-05-2024 06:08 PM
+
+
+ File Folder
+
+
+ 10GB
+
+
+
+
+
+
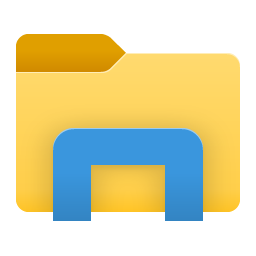
+
+
+
+ Movies
+
+
+ 04-05-2024 06:08 PM
+
+
+ File Folder
+
+
+ 10GB
+
+
+
+
+
+
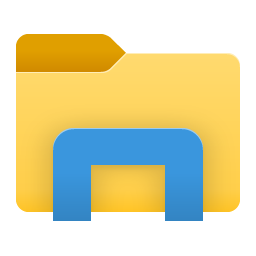
+
+
+
+ Music
+
+
+ 04-05-2024 06:08 PM
+
+
+ File Folder
+
+
+ 10GB
+
+
+
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/components/apps/file_explorer/file_explorer.js b/components/apps/file_explorer/file_explorer.js
new file mode 100644
index 0000000..1df1ce5
--- /dev/null
+++ b/components/apps/file_explorer/file_explorer.js
@@ -0,0 +1,108 @@
+const imageInput = document.getElementById('imageInput');
+const uploadButton = document.getElementById('uploadButton');
+const showButton = document.getElementById('showButton');
+const imagePreview = document.getElementById('imagePreview');
+
+imageInput.addEventListener('change',
+
+ function () {
+ const file = imageInput.files[0];
+ if (file) {
+ const reader = new FileReader();
+ reader.readAsDataURL(file);
+ reader.onload = function () {
+ const imageData = reader.result;
+ let imageName = file.name;
+ localStorage.setItem(imageName, imageData);
+ loadImageList();
+ };
+ }
+ }
+);
+
+function loadImageList() {
+ let allImage = document.querySelector('.loaded-file-box');
+ allImage.innerHTML = '';
+ const storage = window.localStorage;
+ const keys = Object.keys(storage);
+
+ for (const getImageName of keys) {
+ let imageCheck = ['.jpg', '.png', '.jpeg']
+
+ for (let i of imageCheck) {
+ let index = getImageName.indexOf(i);
+
+ if (index !== -1) {
+ // console.log('in if')
+
+ const folderDetailBox = document.createElement('div');
+ folderDetailBox.classList.add('folder-detail-box');
+ folderDetailBox.onclick = () => {
+ showImage(getImageName);
+ }
+
+ const folderDetailIconBox = document.createElement('div');
+ folderDetailIconBox.classList.add('folder-detail-icon-box');
+
+ const folderIcon = document.createElement('img');
+ folderIcon.src = 'images/image_icon.png';
+ folderIcon.alt = 'image';
+ folderIcon.classList.add('folder-icon');
+
+ const folderDetailContentBox = document.createElement('div');
+ folderDetailContentBox.classList.add('folder-detail-box');
+
+ const folderDetailNameBox = document.createElement('div');
+ folderDetailNameBox.classList.add('folder-detail-name-box');
+ folderDetailNameBox.textContent = getImageName;
+
+ const dateModifiedBox = document.createElement('div');
+ dateModifiedBox.classList.add('date-modified-box');
+ dateModifiedBox.textContent = '04-05-2024 06:08 PM';
+
+ const typeBox = document.createElement('div');
+ typeBox.classList.add('type-box');
+ typeBox.textContent = 'File Folder';
+
+ const sizeBox = document.createElement('div');
+ sizeBox.classList.add('size-box');
+ sizeBox.textContent = '100GB';
+
+ folderDetailIconBox.appendChild(folderIcon);
+ folderDetailBox.appendChild(folderDetailIconBox);
+ folderDetailContentBox.appendChild(folderDetailNameBox);
+ folderDetailContentBox.appendChild(dateModifiedBox);
+ folderDetailContentBox.appendChild(typeBox);
+ folderDetailContentBox.appendChild(sizeBox);
+ folderDetailBox.appendChild(folderDetailContentBox);
+ allImage.appendChild(folderDetailBox);
+ }
+ }
+ }
+}
+
+function showImage(imageName) {
+ let imagePreviewBox = document.querySelector('.image-preview-box');
+ let getImage = localStorage.getItem(imageName);
+ imagePreview.src = getImage;
+ imagePreviewBox.style.display = 'flex';
+ setTimeout(() => {
+ if (imagePreview.naturalWidth > imagePreview.naturalHeight) {
+ console.log('width is greater')
+ imagePreview.style.width = '90%';
+ }
+ else {
+ console.log('height is greater')
+ imagePreview.style.height = '90%';
+ }
+ }, 500);
+}
+
+function closeImage() {
+ let imagePreviewBox = document.querySelector('.image-preview-box');
+ imagePreviewBox.style.display = 'none';
+ imagePreview.style.width = 'auto';
+ imagePreview.style.height = 'auto';
+}
+
+loadImageList();
\ No newline at end of file
diff --git a/components/apps/file_explorer/images/arrow-icon.png b/components/apps/file_explorer/images/arrow-icon.png
new file mode 100644
index 0000000..b264647
Binary files /dev/null and b/components/apps/file_explorer/images/arrow-icon.png differ
diff --git a/components/apps/file_explorer/images/image_icon.png b/components/apps/file_explorer/images/image_icon.png
new file mode 100644
index 0000000..5cdf933
Binary files /dev/null and b/components/apps/file_explorer/images/image_icon.png differ
diff --git a/components/apps/youtube/components/video_player/video_player.css b/components/apps/youtube/components/video_player/video_player.css
new file mode 100644
index 0000000..e69de29
diff --git a/components/apps/youtube/components/video_player/video_player.html b/components/apps/youtube/components/video_player/video_player.html
new file mode 100644
index 0000000..0868b91
--- /dev/null
+++ b/components/apps/youtube/components/video_player/video_player.html
@@ -0,0 +1,188 @@
+
+
+
+
+
+
+ YouTube
+
+
+
+
+
+
+
+
+ No Title Avalible!!!
+
+
+
+

+
+
+
+ code with harry
+
+
+ 100M subscribers
+
+
+
+
+
+ The Discription is not avalible for this channel.!!!
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/components/apps/youtube/components/video_player/video_player.js b/components/apps/youtube/components/video_player/video_player.js
new file mode 100644
index 0000000..e69de29
diff --git a/components/apps/youtube/images/search_button_icon.png b/components/apps/youtube/images/search_button_icon.png
new file mode 100644
index 0000000..bd3dbd3
Binary files /dev/null and b/components/apps/youtube/images/search_button_icon.png differ
diff --git a/components/apps/youtube/images/youtube_icon.png b/components/apps/youtube/images/youtube_icon.png
new file mode 100644
index 0000000..88c0fe1
Binary files /dev/null and b/components/apps/youtube/images/youtube_icon.png differ
diff --git a/components/apps/youtube/test.html b/components/apps/youtube/test.html
new file mode 100644
index 0000000..df9e83f
--- /dev/null
+++ b/components/apps/youtube/test.html
@@ -0,0 +1,13 @@
+
+
+
+
+
+ Document
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/components/apps/youtube/test2.html b/components/apps/youtube/test2.html
new file mode 100644
index 0000000..60d4f33
--- /dev/null
+++ b/components/apps/youtube/test2.html
@@ -0,0 +1,90 @@
+
+
+
+
+
+YouTube Channel Page
+
+
+
+
+
+
+
![Channel Thumbnail]()
+
![Channel Logo]()
+
+
+
+
+
+
+
+
+
diff --git a/components/apps/youtube/youtube.css b/components/apps/youtube/youtube.css
new file mode 100644
index 0000000..bd355c0
--- /dev/null
+++ b/components/apps/youtube/youtube.css
@@ -0,0 +1,130 @@
+* {
+ padding: 0px;
+ margin: 0px;
+ list-style: none;
+ text-decoration: none;
+ box-sizing: border-box;
+ user-select: none;
+ font-family: 'Segoe UI';
+}
+
+.container {
+ width: 100%;
+ height: 100dvh;
+ background-color: rgb(22, 22, 22);
+}
+
+.tool-bar-box {
+ width: 100%;
+ height: 40px;
+ margin-bottom: 5px;
+ box-shadow: 0 0 0 1px rgb(255, 255, 255);
+ display: flex;
+ /* justify-content: space-between; */
+ align-items: center;
+}
+
+.logo-box {
+ width: 100px;
+ display: flex;
+ justify-content: center;
+}
+
+.logo-icon {
+ width: 35px;
+}
+
+.search-box {
+ width: 500px;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+#searchInput {
+ width: 80%;
+ height: 30px;
+ padding: 10px;
+ border-radius: 40px;
+}
+
+#searchInput:focus {
+ outline: none;
+ border-color: #3f88f8;
+}
+
+.search-button {
+ width: 30px;
+ height: 30px;
+ margin-left: 10px;
+ border-radius: 50px;
+ background-color: rgb(59, 59, 59);
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.search-button-icon {
+ width: 25px;
+ height: 25px;
+}
+
+h1 {
+ text-align: center;
+ margin-bottom: 20px;
+}
+
+.video-feed {
+ width: 100%;
+ height: calc(100dvh - 65px);
+ overflow: auto;
+}
+
+#searchResultsBox {
+ width: 100%;
+ padding: 5px;
+ display: grid;
+ grid-template-columns: repeat(auto-fit, minmax(300px, 1fr));
+ grid-gap: 10px;
+}
+
+.search-result {
+ background-color: #fff;
+ border: 1px solid #ccc;
+ border-radius: 15px;
+ overflow: hidden;
+ box-shadow: 0 0 5px rgba(0, 0, 0, 0.1);
+ transition: transform 0.2s;
+}
+
+.search-result:hover {
+ transform: translateY(-5px);
+}
+
+.video-detail-box {
+ display: block;
+ text-decoration: none;
+ color: #333;
+}
+
+.thumbnail-image {
+ width: 100%;
+ height: auto;
+ object-fit: cover;
+}
+
+.title {
+ margin: 10px;
+ font-size: 16px;
+ line-height: 1.2;
+}
+
+@media (max-width: 600px) {
+ .search-bar {
+ flex-direction: column;
+ }
+
+ #searchInput {
+ margin-bottom: 10px;
+ }
+}
\ No newline at end of file
diff --git a/components/apps/youtube/youtube.html b/components/apps/youtube/youtube.html
new file mode 100644
index 0000000..9b51b5c
--- /dev/null
+++ b/components/apps/youtube/youtube.html
@@ -0,0 +1,114 @@
+
+
+
+
+
+
+ YouTube
+
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/components/apps/youtube/youtube.js b/components/apps/youtube/youtube.js
new file mode 100644
index 0000000..e69de29
diff --git a/components/apps/youtube/youtube_data_fetch.txt b/components/apps/youtube/youtube_data_fetch.txt
new file mode 100644
index 0000000..2b0796d
--- /dev/null
+++ b/components/apps/youtube/youtube_data_fetch.txt
@@ -0,0 +1,1714 @@
+
+
+{
+ "kind": "youtube#searchListResponse",
+ "etag": "6fUEAJWZ3tdJVlqFcGJi0GF1e8o",
+ "nextPageToken": "CDIQAA",
+ "regionCode": "IN",
+ "pageInfo": {
+ "totalResults": 1000000,
+ "resultsPerPage": 50
+ },
+ "items": [
+ {
+ "kind": "youtube#searchResult",
+ "etag": "0bV1zaVAqCeoBt_INAfKZI1NgWc",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "hW7xDqSDmPE"
+ },
+ "snippet": {
+ "publishedAt": "2024-02-27T05:08:13Z",
+ "channelId": "UCaaDzp5WdD7pRLeu4ZImByA",
+ "title": "KING IS BACK महेश गायकवाड, राहुल भाई पाटील | Pratik Mhatre, Prashant Bhoir",
+ "description": "KING IS BACK महेश गायकवाड, राहुल भाई पाटील | Pratik Mhatre, Prashant Bhoir Singer - Pratik Mhatre ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/hW7xDqSDmPE/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/hW7xDqSDmPE/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/hW7xDqSDmPE/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Pratik Mhatre",
+ "liveBroadcastContent": "none",
+ "publishTime": "2024-02-27T05:08:13Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "dJscVEmalMZNrWcaQjh1dxYGTwM",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "PDNHh371EJ4"
+ },
+ "snippet": {
+ "publishedAt": "2023-11-03T09:57:19Z",
+ "channelId": "UCftNC1Wg5lQbSn3Wo3qXUvQ",
+ "title": "「 King Is Back 🗿」- Jujutsu Kaisen Episode 15 #anime #jujutsukaisen",
+ "description": "Please Don't Repost ⚠️ Edited By - @AX7-JK ——————————————————— Song - Montagem Coral ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/PDNHh371EJ4/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/PDNHh371EJ4/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/PDNHh371EJ4/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "AX7-JK",
+ "liveBroadcastContent": "none",
+ "publishTime": "2023-11-03T09:57:19Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "4vlmBaheY4FsWStYb_mkCb1Jpwo",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "O45HbxSpkDk"
+ },
+ "snippet": {
+ "publishedAt": "2011-11-19T11:14:03Z",
+ "channelId": "UCSejQtXZSp5FFP2K33Gy6DA",
+ "title": "Don 2 Theme song - The King Is Back.mp4",
+ "description": "The king is back (Don 2 theme) full.",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/O45HbxSpkDk/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/O45HbxSpkDk/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/O45HbxSpkDk/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Ratheesh Chandran",
+ "liveBroadcastContent": "none",
+ "publishTime": "2011-11-19T11:14:03Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "Mpv0NoGq1LdaswsOF9mi_kugfHM",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "9qAGMjBY49U"
+ },
+ "snippet": {
+ "publishedAt": "2021-05-15T18:06:30Z",
+ "channelId": "UCOifEyTZIh7fx8volAI1iiQ",
+ "title": "The King Is Back (TrapLion Remix)",
+ "description": "If you like what I do and want to see more content give me a subscribe! Thanks!❤️ Tell me in the comments what song you ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/9qAGMjBY49U/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/9qAGMjBY49U/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/9qAGMjBY49U/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "TrapLion",
+ "liveBroadcastContent": "none",
+ "publishTime": "2021-05-15T18:06:30Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "wwO5QQbAUhW-8EMVCamKQ2r1-6I",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "rDBxjsDO-Rc"
+ },
+ "snippet": {
+ "publishedAt": "2021-10-11T16:02:37Z",
+ "channelId": "UC4T65UWXBHe3KAdrqRVkH-w",
+ "title": "King Is Back || Latest Himachali Nonstop Album || Diwan Siwan || Rd Music Production",
+ "description": "Diwan Siwan & Rd Music Production Presents A Beautiful Himachali Audio Album King Is Back ... Audio Credits ♬ Album ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/rDBxjsDO-Rc/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/rDBxjsDO-Rc/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/rDBxjsDO-Rc/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "DIWAN SIWAN",
+ "liveBroadcastContent": "none",
+ "publishTime": "2021-10-11T16:02:37Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "EmDfePQJBecV-pXI_K5cFuZfNxo",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "naANphAwlXU"
+ },
+ "snippet": {
+ "publishedAt": "2024-02-05T12:30:01Z",
+ "channelId": "UCEvPPKIOUxJHUVK-z6MY65w",
+ "title": "The king is back (Official music video) | Sunday Salahe & Ebiangmitre Lyngdoh |",
+ "description": "WARNING!!!! RE-UPLOAD OF THESE MUSIC VIDEO IS STRICTLY PROHIBITED //WANSALAN DKHAR STUDIO// The king is ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/naANphAwlXU/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/naANphAwlXU/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/naANphAwlXU/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Wansalan Dkhar",
+ "liveBroadcastContent": "none",
+ "publishTime": "2024-02-05T12:30:01Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "6uAvUhiq5dUs3reZiG9scqItTzM",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "TIhxXnatSjY"
+ },
+ "snippet": {
+ "publishedAt": "2012-03-10T07:07:01Z",
+ "channelId": "UCcjRZNsSR8QLHk0ZfX1bAjw",
+ "title": "Don 2 - The King Is Back (Theme) Instrumental",
+ "description": "Subscribe for latest updates. http://www.youtube.com/user/BollywoodMp3Music Log In for more entertainment.",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/TIhxXnatSjY/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/TIhxXnatSjY/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/TIhxXnatSjY/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "BollywoodMp3Music",
+ "liveBroadcastContent": "none",
+ "publishTime": "2012-03-10T07:07:01Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "03Az_eicu94b2qmH54GjHHiI8Lw",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "aMwTRbomQV4"
+ },
+ "snippet": {
+ "publishedAt": "2024-03-25T06:00:01Z",
+ "channelId": "UCjXdbvuF0YyUcWQ-t7O5yFA",
+ "title": "Muhammad Amir Come Back🔥😱 King is Back 🔥 #cricket #amir #comeback #viral #youtubeshorts",
+ "description": "Muhammad Amir Come Back King is Back #cricket #amir #comeback #viral #youtubeshorts.",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/aMwTRbomQV4/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/aMwTRbomQV4/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/aMwTRbomQV4/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "faizi info",
+ "liveBroadcastContent": "none",
+ "publishTime": "2024-03-25T06:00:01Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "pc09nHYw20vm5NxEwpy20_NL9KM",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "6eHD-xyIjZY"
+ },
+ "snippet": {
+ "publishedAt": "2021-12-07T03:02:57Z",
+ "channelId": "UCCDWqfD48_Znk68MGwyfVlA",
+ "title": "Surprise Surprise The King Is Back English Dialogue || Attitude bgm ringtone",
+ "description": "Surprise Surprise The King Is Back English Dialogue|| Attitude bgm ringtone #darasalringtone #surpisesurpisemotherfuker ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/6eHD-xyIjZY/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/6eHD-xyIjZY/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/6eHD-xyIjZY/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "AS editor",
+ "liveBroadcastContent": "none",
+ "publishTime": "2021-12-07T03:02:57Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "tzPG_8_JkOpNzs4b0pULPR-pwbE",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "orDalxRXZfk"
+ },
+ "snippet": {
+ "publishedAt": "2024-02-28T22:20:35Z",
+ "channelId": "UCKyVTyhLyt6-zUgj-16kmxg",
+ "title": "KING IS BACK MAHESH BHAI RAHUL BHAI MAHESH GAIKWAD RAHUL BHAI PATIL 1001 (feat. PRASHANT BHOIR)",
+ "description": "Provided to YouTube by DistroKid KING IS BACK MAHESH BHAI RAHUL BHAI MAHESH GAIKWAD RAHUL BHAI PATIL 1001 ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/orDalxRXZfk/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/orDalxRXZfk/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/orDalxRXZfk/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Pratik Mhatre - Topic",
+ "liveBroadcastContent": "none",
+ "publishTime": "2024-02-28T22:20:35Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "y1ON6IOIzc4Fl2wSaqRfXvUby8Q",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "rDAF8MHpLsE"
+ },
+ "snippet": {
+ "publishedAt": "2020-10-22T15:45:54Z",
+ "channelId": "UCauN0UYA-xabmM5zmsVNQTg",
+ "title": "Eminem, 2Pac & Perły i Łotry - The King Is Back (2020)",
+ "description": "Show some love on my new channel if you like relax music and relax natures view My New Channel TNT Records Relax Nature ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/rDAF8MHpLsE/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/rDAF8MHpLsE/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/rDAF8MHpLsE/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "TNT Records Remix",
+ "liveBroadcastContent": "none",
+ "publishTime": "2020-10-22T15:45:54Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "XYkWS8I00etYpAMm_1asXr76uKw",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "5P3JLcF4q8c"
+ },
+ "snippet": {
+ "publishedAt": "2020-01-22T00:19:33Z",
+ "channelId": "UCVGi2atNt81_box8Rz4MjQg",
+ "title": "Conor McGregor - "The King Is Back"",
+ "description": "Conor \"The Notorious\" McGregor is back. UFC246 Thanks for watching! Like and Share if you enjoyed. Thumbnail by ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/5P3JLcF4q8c/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/5P3JLcF4q8c/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/5P3JLcF4q8c/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Mateo",
+ "liveBroadcastContent": "none",
+ "publishTime": "2020-01-22T00:19:33Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "eYbxJrA4Hba_q72HvnicTgcZ8m0",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "DYwxa7kE4qw"
+ },
+ "snippet": {
+ "publishedAt": "2024-05-05T11:30:07Z",
+ "channelId": "UCeFU6x_70qTaHAvrPD8UCVw",
+ "title": "എടാ മോനെ🥳Factory Top King Is Back🔥REDEEMCODE🔥Freefire Malayalam | Solo rush gameplay #megamer",
+ "description": "INSTAGRAM : megamer.og WHATS APP CHANNEL: https://whatsapp.com/channel/0029VaD3vAdDp2QH5RSYI61w LOVE U ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/DYwxa7kE4qw/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/DYwxa7kE4qw/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/DYwxa7kE4qw/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "ME Gamer",
+ "liveBroadcastContent": "none",
+ "publishTime": "2024-05-05T11:30:07Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "OmWKqukIC7JUvWjgAiLiDNk9Las",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "hpvTVX1LWGQ"
+ },
+ "snippet": {
+ "publishedAt": "2022-12-13T11:34:31Z",
+ "channelId": "UCx6F-rETGiz7xf_vkMmX2yQ",
+ "title": "PHOTOSHOP KING IS BACK 🔥",
+ "description": "Photoshop king vapas aa chuka hai dosto! sorry thara bhai joginder, technical guruji, flying beast, adnaan07 also I love Emiway!",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/hpvTVX1LWGQ/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/hpvTVX1LWGQ/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/hpvTVX1LWGQ/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Mythpat",
+ "liveBroadcastContent": "none",
+ "publishTime": "2022-12-13T11:34:31Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "tt9mCeZgt1asNUYwg6CfCRYPGVo",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "OnzFa8l2zo0"
+ },
+ "snippet": {
+ "publishedAt": "2023-01-20T20:00:11Z",
+ "channelId": "UCxnQDP0cJbhX96JL61h1h9Q",
+ "title": "Rytikal - The King is Back (Official Video)",
+ "description": "Official Music Video for \"The King is Back\" by Rytikal Prod: Dynasty Global / RMG / Primetime Music Download/Stream: ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/OnzFa8l2zo0/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/OnzFa8l2zo0/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/OnzFa8l2zo0/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "RytikalVEVO",
+ "liveBroadcastContent": "none",
+ "publishTime": "2023-01-20T20:00:11Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "V7JK4aeyv6ya2XJUFWqHW3dzTgU",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "cJaDOdz4-c0"
+ },
+ "snippet": {
+ "publishedAt": "2011-11-18T03:31:44Z",
+ "channelId": "UCY0922HCclcjB2orKGOjyxA",
+ "title": "The King Is Back (Theme) - DON 2 - 320 kbps. RIZ.",
+ "description": "The King Is Back (Theme) - DON 2 - 320 kbps. RIZ. Don 2, Shahrukh Khan, Priyanka Chopra.",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/cJaDOdz4-c0/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/cJaDOdz4-c0/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/cJaDOdz4-c0/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "bsrizwanreturns",
+ "liveBroadcastContent": "none",
+ "publishTime": "2011-11-18T03:31:44Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "SQCwwCF9aJS2sBQD3eS_PG-V4cI",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "_mRV0fL8pt0"
+ },
+ "snippet": {
+ "publishedAt": "2024-02-19T17:15:59Z",
+ "channelId": "UCkzCjdRMrW2vXLx8mvPVLdQ",
+ "title": "KEVIN DE BRUYNE | RETURN OF THE KING | Documentary",
+ "description": "In \"Return of the King\", we go behind the scenes to see how De Bruyne's recovery was managed as he worked closely with ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/_mRV0fL8pt0/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/_mRV0fL8pt0/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/_mRV0fL8pt0/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Man City",
+ "liveBroadcastContent": "none",
+ "publishTime": "2024-02-19T17:15:59Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "QD7HiUhFsP7G3dr4PaVLqPduCkQ",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "sW1fJOrlZDA"
+ },
+ "snippet": {
+ "publishedAt": "2024-03-26T04:59:29Z",
+ "channelId": "UCU1k-SomkSh-GELXnTm7h1g",
+ "title": "King is back😈🤯 #youtubeshorts #cricket #mohammadamir",
+ "description": "King is back #youtubeshorts #cricket #mohammadamir.",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/sW1fJOrlZDA/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/sW1fJOrlZDA/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/sW1fJOrlZDA/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Wesso All Rounder",
+ "liveBroadcastContent": "none",
+ "publishTime": "2024-03-26T04:59:29Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "tuIrN7Nl3vjIVgFu6OKxk_KaYaQ",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "Q7KuF4aImt8"
+ },
+ "snippet": {
+ "publishedAt": "2017-09-19T14:31:42Z",
+ "channelId": "UCwwHxdscieNH0dzWmOwnuqw",
+ "title": "King Back",
+ "description": "Provided to YouTube by AdShare for a Third party King Back · T.I. King ℗ Grand Hustle, LLC | Cinq Recordings Released on: ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/Q7KuF4aImt8/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/Q7KuF4aImt8/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/Q7KuF4aImt8/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "T.I. - Topic",
+ "liveBroadcastContent": "none",
+ "publishTime": "2017-09-19T14:31:42Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "Yk5DnDW6s_9NdNuEMblU2sYG_Ig",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "8ekbBEKyR0k"
+ },
+ "snippet": {
+ "publishedAt": "2024-04-12T07:00:06Z",
+ "channelId": "UC9DtoxhwIaP5RkBgy7jbqmA",
+ "title": "Re:drum - The King Is Back (Official Music Video)",
+ "description": "Official music video for \"Re:drum - The King Is Back\" Directed by @kirilltsvirkun Edited by @buyanccivisual Main camera - Eugene ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/8ekbBEKyR0k/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/8ekbBEKyR0k/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/8ekbBEKyR0k/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "RedrumVEVO",
+ "liveBroadcastContent": "none",
+ "publishTime": "2024-04-12T07:00:06Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "RjsPZUPTRY-upmgwepk0BSBw9BA",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "VqtJw67xM14"
+ },
+ "snippet": {
+ "publishedAt": "2016-08-21T05:33:12Z",
+ "channelId": "UCGghramr2D3HNgYx7JCPAfQ",
+ "title": ""Surprise, Surprise Muthafucka! The King is Back Conor McGregor",
+ "description": "",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/VqtJw67xM14/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/VqtJw67xM14/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/VqtJw67xM14/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "UNCLE BEN",
+ "liveBroadcastContent": "none",
+ "publishTime": "2016-08-21T05:33:12Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "wxK7--IXYoYfbCR3h44OZZaVxJM",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "xxhdFkOe8-8"
+ },
+ "snippet": {
+ "publishedAt": "2020-03-06T18:58:39Z",
+ "channelId": "UC65afEgL62PGFWXY7n6CUbA",
+ "title": "Fabian Mazur - King Is Back 👑",
+ "description": "Download/Stream: https://fabianmazur.ffm.to/kingisback Join our Notification Squad! Click the Trap City Discord!",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/xxhdFkOe8-8/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/xxhdFkOe8-8/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/xxhdFkOe8-8/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Trap City",
+ "liveBroadcastContent": "none",
+ "publishTime": "2020-03-06T18:58:39Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "OfilOqGsTX_sNcqSjO7CyrqAfRQ",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "ZLDDQRTFx90"
+ },
+ "snippet": {
+ "publishedAt": "2024-05-05T23:33:51Z",
+ "channelId": "UCg5iO7Pbg7f4gmufzOLD_zA",
+ "title": "Drake Disses Kendrick Lamar with 'The Heart Part 6'. WOW.. DID DRAKE FINESSE THE COMEBACK?",
+ "description": "Cop merch theakademy.shop rn Today's sponsor is Underdog Media, use promo code AKADEMIKS and get your first deposited ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/ZLDDQRTFx90/default_live.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/ZLDDQRTFx90/mqdefault_live.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/ZLDDQRTFx90/hqdefault_live.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "King Akademiks",
+ "liveBroadcastContent": "live",
+ "publishTime": "2024-05-05T23:33:51Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "PnSrrmJpvztF9JG0Br0aaoSK1pg",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "XAf60KH9984"
+ },
+ "snippet": {
+ "publishedAt": "2023-06-04T03:27:54Z",
+ "channelId": "UCjtcsbBSogpYEhS6AWDoVPg",
+ "title": "UFC 303: Conor McGregor “The King Is Back” | Official Trailer",
+ "description": "conormcgregor #michaelchandler #ufc #combat #fight #mma #promo #trailer #ufc303 Conor McGregor | The King Is Back 2023 ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/XAf60KH9984/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/XAf60KH9984/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/XAf60KH9984/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Cage Culture",
+ "liveBroadcastContent": "none",
+ "publishTime": "2023-06-04T03:27:54Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "nav1XOWzCQw7ZmfoZB4QB_ldwfU",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "QztFMc4uX-U"
+ },
+ "snippet": {
+ "publishedAt": "2024-04-05T15:00:06Z",
+ "channelId": "UCByMUlP_FZYGuM0G5iaYsYA",
+ "title": "Armin Nicoara - Instrumentala The King is Back | Videoclip Oficial",
+ "description": "Armin Nicoara - Instrumentala The King is Back | Instrumentală 2024 Dragilor, desi Armin se afla acum in competitia „Insula de 1 ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/QztFMc4uX-U/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/QztFMc4uX-U/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/QztFMc4uX-U/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Armin Nicoara",
+ "liveBroadcastContent": "none",
+ "publishTime": "2024-04-05T15:00:06Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "BJ4N9gOq_3AsThk7ZwkbF1erYps",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "BrG4npZYG40"
+ },
+ "snippet": {
+ "publishedAt": "2022-09-12T01:53:28Z",
+ "channelId": "UCEPpLkYZXBNsS07bMynAvEw",
+ "title": "The king is back👑",
+ "description": "The king is back and more ready than ever lol.",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/BrG4npZYG40/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/BrG4npZYG40/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/BrG4npZYG40/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Adam Fadumm",
+ "liveBroadcastContent": "none",
+ "publishTime": "2022-09-12T01:53:28Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "3Pyw9vQyZP5tiNS3BNCcNkaRYrA",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "9tZfHPAklSE"
+ },
+ "snippet": {
+ "publishedAt": "2017-07-20T13:00:00Z",
+ "channelId": "UCcFKiA_8CSzamBPw3dPNRbQ",
+ "title": "Aloe Blacc - King Is Born (Audio)",
+ "description": "Download or stream Aloe Blacc's \"King is Born\": http://smarturl.it/KingIsBorn Co-produced by Aloe Blacc For more: ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/9tZfHPAklSE/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/9tZfHPAklSE/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/9tZfHPAklSE/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "AloeBlaccVEVO",
+ "liveBroadcastContent": "none",
+ "publishTime": "2017-07-20T13:00:00Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "f8Mo4Pu2uNGd217OXFC3lRrkSwM",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "yt0iqTzdgiM"
+ },
+ "snippet": {
+ "publishedAt": "2022-07-25T12:00:41Z",
+ "channelId": "UCD651SLBWg7kX0EyivrLnQA",
+ "title": "【maimai外部出力(60fps)】KING is BACK!! MAS AP",
+ "description": "Title: KING is BACK!! Difficulty: Master Level: 13+ Speed: 7.0 *This is autogenerated, not my gameplay.",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/yt0iqTzdgiM/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/yt0iqTzdgiM/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/yt0iqTzdgiM/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "よぞら",
+ "liveBroadcastContent": "none",
+ "publishTime": "2022-07-25T12:00:41Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "fCEABiBzDZ8kDws2BJCZHajVm9o",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "0jf4gF6Xm9M"
+ },
+ "snippet": {
+ "publishedAt": "2023-05-04T15:20:41Z",
+ "channelId": "UCBL-zwQtYjRxhcKbYRBp4xw",
+ "title": "S.I.D | KING IS BACK | THE VIRAT KOHLI ANTHEM | MUSIC VIDEO | KANNADA RAP | 4K",
+ "description": "This anthem is my tribute to Virat's achievements and his ability to inspire millions of fans like me with his performances.",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/0jf4gF6Xm9M/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/0jf4gF6Xm9M/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/0jf4gF6Xm9M/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "S.I.D ",
+ "liveBroadcastContent": "none",
+ "publishTime": "2023-05-04T15:20:41Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "PKawnLkwAMjP2V_t_IdteLFGbI8",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "z8uT8Hostyg"
+ },
+ "snippet": {
+ "publishedAt": "2021-11-30T03:48:50Z",
+ "channelId": "UCYsL1HG25wiq3J_iVGQU_fA",
+ "title": "Eminem & 2pac the king is back 2020(Bass Boosted",
+ "description": "",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/z8uT8Hostyg/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/z8uT8Hostyg/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/z8uT8Hostyg/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "MIXs",
+ "liveBroadcastContent": "none",
+ "publishTime": "2021-11-30T03:48:50Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "c1BatNXyazPAlOz8AoGAyehnjnQ",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "OPGdyE-f7rc"
+ },
+ "snippet": {
+ "publishedAt": "2022-08-19T02:09:23Z",
+ "channelId": "UCSnaa0YdsUZBfpBzYVQy9oA",
+ "title": "Roman reigns wears crown | the king is back #shorts #youtubeshorts",
+ "description": "hi guys subscribe @chintureigns channel. -Roman reigns attitude status -Roman reigns status -Roman reigns -Roman reigns ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/OPGdyE-f7rc/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/OPGdyE-f7rc/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/OPGdyE-f7rc/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "CHINTU REIGNS",
+ "liveBroadcastContent": "none",
+ "publishTime": "2022-08-19T02:09:23Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "WDqix5SdCF_tymgXWd-gdh0ZHH0",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "85Nn_zJDfA4"
+ },
+ "snippet": {
+ "publishedAt": "2016-09-04T12:56:51Z",
+ "channelId": "UCmpdspDwUuff-6w3Ry81BXA",
+ "title": "Conor McGregor Surprise Surprise Motherfucker! The King Is Back!",
+ "description": "",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/85Nn_zJDfA4/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/85Nn_zJDfA4/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/85Nn_zJDfA4/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "SMITH Personal Trainer",
+ "liveBroadcastContent": "none",
+ "publishTime": "2016-09-04T12:56:51Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "f4nglHi2hfvsT3Fadhqpkeyj9Nw",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "LwOczsCU4jA"
+ },
+ "snippet": {
+ "publishedAt": "2022-10-22T02:30:09Z",
+ "channelId": "UCSBBlaJJ-tk9zg_1VTh0ndw",
+ "title": "ZEE - King Coming Back",
+ "description": "ZEE drops first visual of his new album \"Legendz Never Die\" \"King Coming Back\" is a song to prepare a generation for the second ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/LwOczsCU4jA/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/LwOczsCU4jA/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/LwOczsCU4jA/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Zee Ministries",
+ "liveBroadcastContent": "none",
+ "publishTime": "2022-10-22T02:30:09Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "NDjHyzyAy-ujZNxQepVDAHCazAk",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "vCQ3ZrE8DKw"
+ },
+ "snippet": {
+ "publishedAt": "2021-12-07T10:30:07Z",
+ "channelId": "UCObyBrdrtQ20BU9PxHQDY9w",
+ "title": "Singh Is Bliing (4K) | Akshay Kumar, Amy Jackson, Lara Dutta, Prabhu Deva | Full Hindi Movie",
+ "description": "Singh Is Bliing (4K) | Akshay Kumar, Amy Jackson, Lara Dutta, Prabhu Deva | Full Hindi Movie Raftaar Singh always runs away ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/vCQ3ZrE8DKw/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/vCQ3ZrE8DKw/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/vCQ3ZrE8DKw/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Pen Multiplex",
+ "liveBroadcastContent": "none",
+ "publishTime": "2021-12-07T10:30:07Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "fjv3hE27I5Wguw3c0Q9mdTvceHI",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "Eoz0vzHgGRY"
+ },
+ "snippet": {
+ "publishedAt": "2020-03-06T12:00:14Z",
+ "channelId": "UCK2CDEfRS-Wl3V0kAbIje-g",
+ "title": "Fabian Mazur - King Is Back",
+ "description": "Out now: https://fabianmazur.ffm.to/kingisback Listen to my music on Spotify: https://spoti.fi/2OI9QHA Check out my sample packs ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/Eoz0vzHgGRY/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/Eoz0vzHgGRY/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/Eoz0vzHgGRY/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Fabian Mazur",
+ "liveBroadcastContent": "none",
+ "publishTime": "2020-03-06T12:00:14Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "4LQj4YMrsk1x-wyAGuHp3bjXMeI",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "kr7sv-Gfc5w"
+ },
+ "snippet": {
+ "publishedAt": "2023-05-07T11:31:12Z",
+ "channelId": "UCFN7NMAjnwlpauE5bbnuf3w",
+ "title": "The King is Back | Conor McGregor Highlights",
+ "description": "Conor McGregor is an Irish mixed martial artist in the UFC featherweight division. He made his UFC debut in 2013 and quickly ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/kr7sv-Gfc5w/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/kr7sv-Gfc5w/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/kr7sv-Gfc5w/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Real Highlights",
+ "liveBroadcastContent": "none",
+ "publishTime": "2023-05-07T11:31:12Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "AwkFML6LjV5ITmtXkTI1T6AKGMs",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "7VMxtn4tU5k"
+ },
+ "snippet": {
+ "publishedAt": "2018-01-28T21:05:15Z",
+ "channelId": "UCG6QEHCBfWZOnv7UVxappyw",
+ "title": "The Brig - King Is Back",
+ "description": "The Brig - King Is Back STREAM NOW: https://dubstepgutter.komi.io/ The Brig ✗ https://www.facebook.com/brigsound ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/7VMxtn4tU5k/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/7VMxtn4tU5k/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/7VMxtn4tU5k/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "DubstepGutter",
+ "liveBroadcastContent": "none",
+ "publishTime": "2018-01-28T21:05:15Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "taQNmoA60eqOdhvzoGuCU53hlvI",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "Edhxj_XanKY"
+ },
+ "snippet": {
+ "publishedAt": "2019-11-06T14:00:02Z",
+ "channelId": "UCWDtuJ1TTocJsb9A3l2WLHw",
+ "title": "Burak Balkan - Feel #KingIsBack 👑",
+ "description": "Burak Balkan's new song, \"Feel\" is OUT NOW! Ücretsiz Abone Ol: https://bit.ly/BurakAbone Kanal ayrıcalıklarından yararlanmak ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/Edhxj_XanKY/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/Edhxj_XanKY/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/Edhxj_XanKY/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Burak Balkan",
+ "liveBroadcastContent": "none",
+ "publishTime": "2019-11-06T14:00:02Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "wiTxol_09nayZ_gullNgfCuQ_Es",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "IDCE2Em1xsQ"
+ },
+ "snippet": {
+ "publishedAt": "2017-10-20T22:07:18Z",
+ "channelId": "UCstRbi5Zg8m9BANs15YdZRQ",
+ "title": "SNAILS - King Is Back (Feat. Big Ali) (Snails & Sullivan King Metal Remix)",
+ "description": "SNAILS - King Is Back (Feat. Big Ali) (Snails & Sullivan King Metal Remix) Purchase/Stream: http://smarturl.it/theshell Follow ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/IDCE2Em1xsQ/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/IDCE2Em1xsQ/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/IDCE2Em1xsQ/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "SNAILS",
+ "liveBroadcastContent": "none",
+ "publishTime": "2017-10-20T22:07:18Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "NaGDnNMrQ0zcA5iDo9JxmT5MFsY",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "jX6sor_QNp0"
+ },
+ "snippet": {
+ "publishedAt": "2014-03-21T11:08:14Z",
+ "channelId": "UCdXZZIp5jAJJ63jy7h7eABQ",
+ "title": "Zeljko Obradović, "The King Is Back" - First comeback to OAKA of Athens",
+ "description": "Follow on official website www.invictussportsgroup.com, twitter @InvictusSports, facebook InvictusSportsGroup.",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/jX6sor_QNp0/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/jX6sor_QNp0/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/jX6sor_QNp0/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "INVICTUS ACADEMY",
+ "liveBroadcastContent": "none",
+ "publishTime": "2014-03-21T11:08:14Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "ILDK8G8-ilxmmTdfpkpzVz0I_fM",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "E0gQ2ArTF5k"
+ },
+ "snippet": {
+ "publishedAt": "2022-11-26T06:17:16Z",
+ "channelId": "UCTKmL5sJuLOkYEDCLkQy24A",
+ "title": "The king is back 2 : freefire animation",
+ "description": "Don't take it too seriously its just a fan Made Music : Music: Bonecrusher by Soundridemusic Copyright: GOLOVACH, ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/E0gQ2ArTF5k/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/E0gQ2ArTF5k/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/E0gQ2ArTF5k/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "GLENK10",
+ "liveBroadcastContent": "none",
+ "publishTime": "2022-11-26T06:17:16Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "HCJfWE9EnVFZc1iA3PBIswd5kp0",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "Cjx0wtpTEm4"
+ },
+ "snippet": {
+ "publishedAt": "2020-04-16T06:00:06Z",
+ "channelId": "UC8Gka-5Toy9TPvaU4o7Kr1w",
+ "title": "【maimai】KING is BACK!!/A-One",
+ "description": "今までのオリジナル楽曲をお家でも楽しめるように、ズババババーーーーンと大公開!!!!!! 楽曲タイトル:KING is BACK!",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/Cjx0wtpTEm4/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/Cjx0wtpTEm4/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/Cjx0wtpTEm4/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "ゲキ!チュウマイ公式ちゃんねる",
+ "liveBroadcastContent": "none",
+ "publishTime": "2020-04-16T06:00:06Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "BmQ3CYdxs9Fnz2GnBu05jqHVuoo",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "BLy-fB4CGhg"
+ },
+ "snippet": {
+ "publishedAt": "2022-01-11T17:58:46Z",
+ "channelId": "UC2_CgurqasNWY4JYjJBKfSg",
+ "title": "The King is Back👑",
+ "description": "I'm Finally back with my best Fortnite montage ever... Hope you guys enjoy this 150+ hour project. I'm back on the grind now and ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/BLy-fB4CGhg/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/BLy-fB4CGhg/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/BLy-fB4CGhg/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "FaZe Flea",
+ "liveBroadcastContent": "none",
+ "publishTime": "2022-01-11T17:58:46Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "w2J13ydk8JyxSzFxfU8YRrleOGI",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "yiprOesGghc"
+ },
+ "snippet": {
+ "publishedAt": "2009-01-18T00:40:17Z",
+ "channelId": "UC_AYBMNGha8rW1X8Mvq3SDw",
+ "title": "Daddy Yankee - Jefe",
+ "description": "Tu Sabes Que Yo Hago Falta Sintieran Un Vacio Sin Mi Ah Solamente Hay Un... (Puerto Rico)Jefe Jefe Jefe Jefe(New York City) ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/yiprOesGghc/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/yiprOesGghc/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/yiprOesGghc/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Ganar Dinero",
+ "liveBroadcastContent": "none",
+ "publishTime": "2009-01-18T00:40:17Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "TvP9gm9pm7brZ79408Vo1AEFGDg",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "qwfVXDS8PBo"
+ },
+ "snippet": {
+ "publishedAt": "2020-03-07T15:00:12Z",
+ "channelId": "UC8VP53f7SAb3RD0U5MqRyZA",
+ "title": "Fabian Mazur - King Is Back (Bass Boosted)",
+ "description": "Fabian Mazur - King Is Back Download Link ↪︎ https://fabianmazur.ffm.to/kingisback Submit your music here: ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/qwfVXDS8PBo/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/qwfVXDS8PBo/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/qwfVXDS8PBo/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Trap Woofer",
+ "liveBroadcastContent": "none",
+ "publishTime": "2020-03-07T15:00:12Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "MPowJtH5Ag6Yvo9GfZEvnNtVqwQ",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "_1YFUUFFGto"
+ },
+ "snippet": {
+ "publishedAt": "2022-05-18T12:01:39Z",
+ "channelId": "UCC_iD2pY80sjA0sZIi8Ym4g",
+ "title": "【maimaiでらっくす】KING is BACK!! スタンダード譜面 MASTER ALL PERFECT 【直撮り】",
+ "description": "The物量な譜面。個人的には好きだけど同じ繰り返しの譜面だから、体勢間違えたりするとすぐに体力持っていかれるイメージの ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/_1YFUUFFGto/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/_1YFUUFFGto/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/_1YFUUFFGto/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Amakage!",
+ "liveBroadcastContent": "none",
+ "publishTime": "2022-05-18T12:01:39Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "ZDHqrSvuUaPUgM7zRlZ_Ep1uQ6c",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "uebQuCgvpyY"
+ },
+ "snippet": {
+ "publishedAt": "2014-01-06T21:53:19Z",
+ "channelId": "UCJ8v1z1vgq9nLzyPyjD0KYg",
+ "title": "Wendy majeste the king is back",
+ "description": "Rap kreyol.",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/uebQuCgvpyY/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/uebQuCgvpyY/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/uebQuCgvpyY/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Jblack Gromo",
+ "liveBroadcastContent": "none",
+ "publishTime": "2014-01-06T21:53:19Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "rVgR-gTsb3CNuVfT-MjshD1tnAk",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "TYmgV06bIh8"
+ },
+ "snippet": {
+ "publishedAt": "2024-05-04T13:35:46Z",
+ "channelId": "UCXmjsTNeeBF5ZFjUmcFSDZQ",
+ "title": "INAZUMA ELEVEN VICTORY ROAD SHINLOCKE EP.6: THE KING IS BACK",
+ "description": "Sexto capítulo de #InazumaEleven Victory Road Shinlocke⚡ ➡️Puedes ver todos los episodios aquí ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/TYmgV06bIh8/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/TYmgV06bIh8/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/TYmgV06bIh8/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Shinbu",
+ "liveBroadcastContent": "none",
+ "publishTime": "2024-05-04T13:35:46Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "cpVfG4LJlufVVINTsj8stGAiqCI",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "torTDZIVKok"
+ },
+ "snippet": {
+ "publishedAt": "2022-07-23T12:30:13Z",
+ "channelId": "UCL23LAymRSo9Ka5kVCwmaCg",
+ "title": "किंग नाय मी किंगमेकर हाय King Nahi Kingmaker Hay Munna Bhalerao Bhaigiri Song Marathi Attitude Song",
+ "description": "किंग नाय मी किंगमेकर हाय | King Nay Mi Kingmaker Hay..Munna Bhalerao..Bhaigiri Marathi Attitude Song ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/torTDZIVKok/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/torTDZIVKok/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/torTDZIVKok/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Munna Bhalerao Official",
+ "liveBroadcastContent": "none",
+ "publishTime": "2022-07-23T12:30:13Z"
+ }
+ },
+ {
+ "kind": "youtube#searchResult",
+ "etag": "rOHnrJkHI0yPZc0ngY6JpzoR8Xg",
+ "id": {
+ "kind": "youtube#video",
+ "videoId": "fDC-5b5I1aA"
+ },
+ "snippet": {
+ "publishedAt": "2021-02-14T17:00:14Z",
+ "channelId": "UCa10nxShhzNrCE1o2ZOPztg",
+ "title": "Fabian Mazur - King Is Back",
+ "description": "Fabian Mazur - King Is Back STREAM NOW: https://trapnation.komi.io Fabian Mazur ✗ https://instagram.com/fabianmazur/ ...",
+ "thumbnails": {
+ "default": {
+ "url": "https://i.ytimg.com/vi/fDC-5b5I1aA/default.jpg",
+ "width": 120,
+ "height": 90
+ },
+ "medium": {
+ "url": "https://i.ytimg.com/vi/fDC-5b5I1aA/mqdefault.jpg",
+ "width": 320,
+ "height": 180
+ },
+ "high": {
+ "url": "https://i.ytimg.com/vi/fDC-5b5I1aA/hqdefault.jpg",
+ "width": 480,
+ "height": 360
+ }
+ },
+ "channelTitle": "Trap Nation",
+ "liveBroadcastContent": "none",
+ "publishTime": "2021-02-14T17:00:14Z"
+ }
+ }
+ ]
+}
\ No newline at end of file
diff --git a/images/battery_icon.png b/images/battery_icon.png
new file mode 100644
index 0000000..dc66dd6
Binary files /dev/null and b/images/battery_icon.png differ
diff --git a/images/maximize_icon.png b/images/maximize_icon.png
new file mode 100644
index 0000000..2de89d3
Binary files /dev/null and b/images/maximize_icon.png differ
diff --git a/images/minimize_icon.png b/images/minimize_icon.png
new file mode 100644
index 0000000..73b9fa9
Binary files /dev/null and b/images/minimize_icon.png differ
diff --git a/images/show_hidden_icons_arrow.png b/images/show_hidden_icons_arrow.png
new file mode 100644
index 0000000..a906537
--- /dev/null
+++ b/images/show_hidden_icons_arrow.png
@@ -0,0 +1 @@
+ssssssssssssfgfgfgfgfgfgfgfgfgfgfgfgfgfgfgddddddddddddfffffffffffffffffffffdfffffffffffffffdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdffffffffffffffffffffffdffffffffffffffffffddddddddddddddddddddddddfffffffffffffffffffffffffffffffdddddddddddddddddddddffffffffffffffffdfffffffffffffffffffffddddddddddddddddddddddddddddddfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffffdfffffffffffffffffffffffffffdffffffffffffffffffdfffffffffffffffdfffffffffffffffffdffffffffffffffdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdfdf
diff --git a/images/wifi_icon.png b/images/wifi_icon.png
new file mode 100644
index 0000000..18cd429
Binary files /dev/null and b/images/wifi_icon.png differ
diff --git a/index.html b/index.html
new file mode 100644
index 0000000..f0b2cb9
--- /dev/null
+++ b/index.html
@@ -0,0 +1,136 @@
+
+
+
+
+
+
+
+
+ Windows 11
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
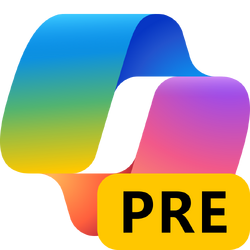
+
+
+
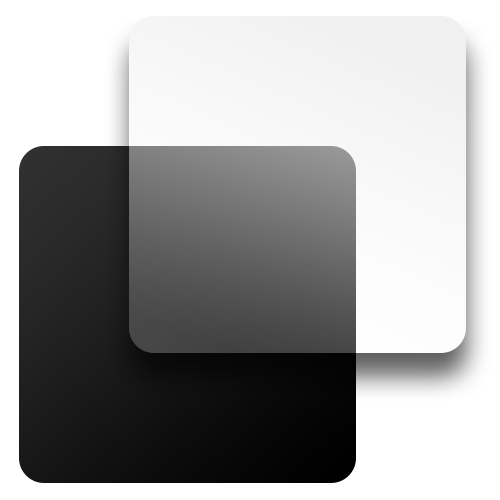
+
+
+
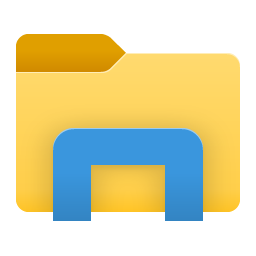
+
+
+

+
+
+
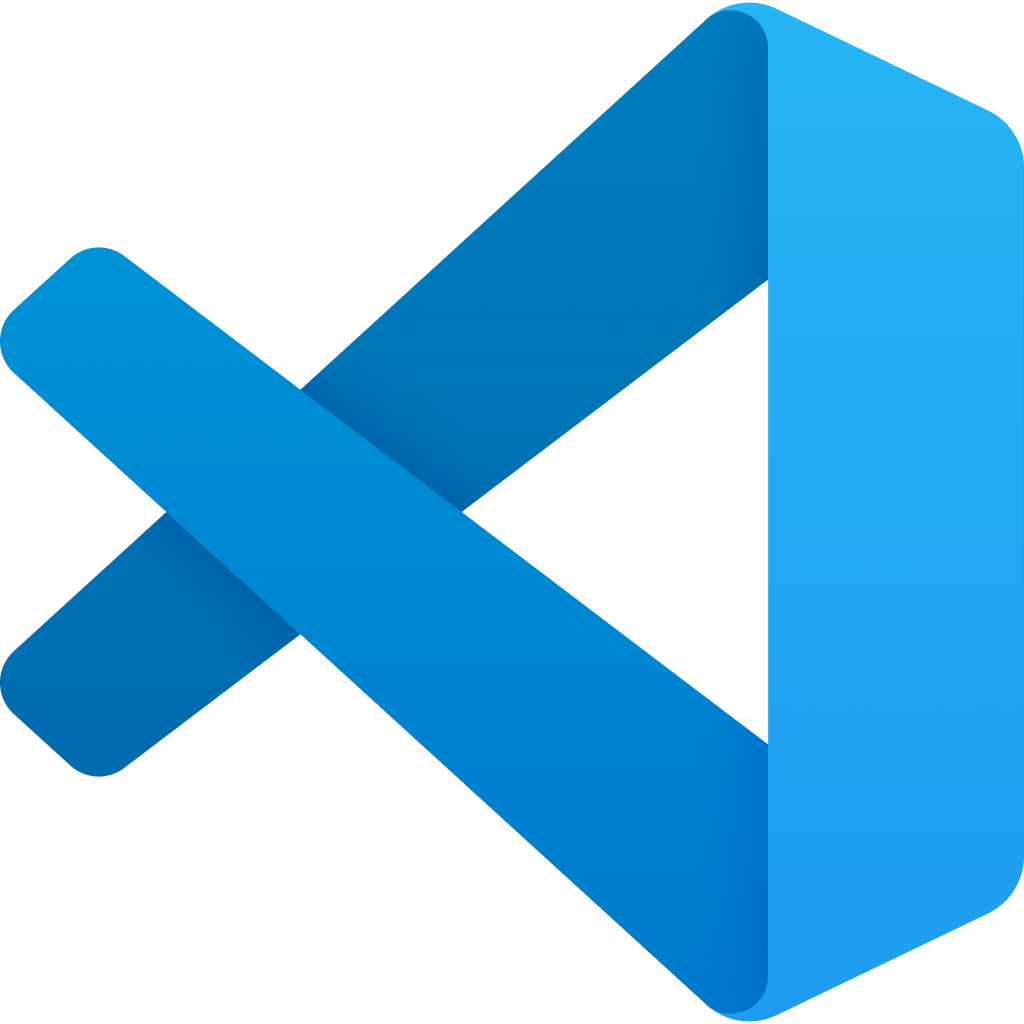
+
+
+

+
+
+
+
+
+
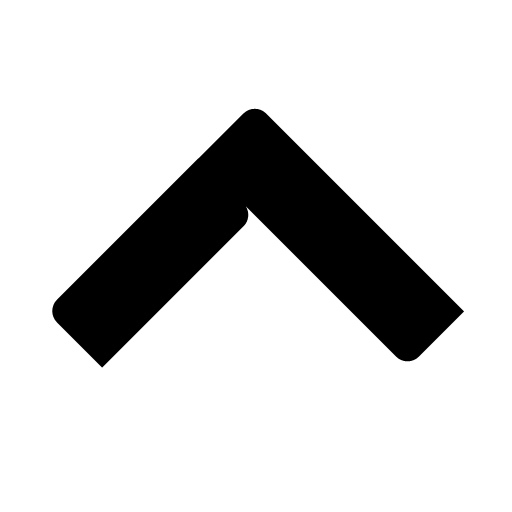
+
+
+ ENG
IN
+
+
+
+
+
+ 11:20 PM
+
+
+ 30-04-2024
+
+
+
+

+
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/script.js b/script.js
new file mode 100644
index 0000000..2096a4f
--- /dev/null
+++ b/script.js
@@ -0,0 +1,72 @@
+let time = document.getElementById('time');
+let date = document.getElementById('date');
+
+document.documentElement.requestFullscreen();
+// window.confirm("how are you!")
+
+function openFullscreen() {
+ let fullScreen = document.getElementById('fullScreen');
+ if (fullScreen.requestFullscreen) {
+ fullScreen.requestFullscreen();
+ }
+ else if (fullScreen.webkitRequestFullscreen) { /* Safari */
+ fullScreen.webkitRequestFullscreen();
+ }
+ else if (fullScreen.msRequestFullscreen) { /* IE11 */
+ fullScreen.msRequestFullscreen();
+ }
+}
+
+function changeTimeDate() {
+ let Dates = new Date();
+
+ currentDate = Dates.getDate();
+ currentMouth = Dates.getMonth();
+ currentMouth = currentMouth + 1
+ currentYear = Dates.getFullYear();
+ currentMinute = Dates.getMinutes();
+ currentHour = Dates.getHours();
+ let amPm = ' AM'
+
+ if (currentHour > 12) {
+ currentHour = currentHour - 12;
+ amPm = ' PM'
+ }
+
+ if (currentDate <= 9) {
+ currentDate = '0' + currentDate;
+ }
+ if (currentMouth <= 9) {
+ currentMouth = '0' + currentMouth;
+ }
+ if (currentHour <= 9) {
+ currentHour = '0' + currentHour;
+ }
+ if (currentMinute <= 9) {
+ currentMinute = '0' + currentMinute;
+ }
+
+ time.innerHTML = currentHour + ':' + currentMinute + amPm;
+ date.innerHTML = currentDate + '-' + currentMouth + '-' + currentYear;
+}
+
+let batteryBox = document.querySelector('.battery-box');
+let batteryLevelBox = document.createElement("div");
+batteryLevelBox.classList.add('battery-level-box');
+batteryBox.appendChild(batteryLevelBox);
+function batteryLevel() {
+ navigator.getBattery().then(function(battery) {
+ let batteryLevel = battery.level * 100;
+ // batteryLevelBox.textContent = parseInt(batteryLevel) + '%';
+ batteryLevelBox.textContent = batteryLevel + '%';
+ });
+}
+
+function refreshMainWindowComponents() {
+ changeTimeDate();
+ batteryLevel();
+}
+
+changeTimeDate();
+batteryLevel();
+setInterval(refreshMainWindowComponents, 60000)
\ No newline at end of file
diff --git a/style.css b/style.css
new file mode 100644
index 0000000..296cddb
--- /dev/null
+++ b/style.css
@@ -0,0 +1,250 @@
+* {
+ padding: 0px;
+ margin: 0px;
+ list-style: none;
+ text-decoration: none;
+ box-sizing: border-box;
+ user-select: none;
+ font-family:'Segoe UI';
+}
+
+.megacontainer {
+ overflow: hidden;
+}
+
+.screen {
+ width: 100%;
+ min-height: 100%;
+}
+
+.wallpaper {
+ width: 100%;
+ height: 100vh;
+ padding: 0.5px;
+ background-image: url('https://cdn.wallpaperhub.app/cloudcache/f/0/3/f/8/a/f03f8a91e5396f8bb7cebaf7410d9a619e14ed7f.jpg');
+ background-repeat: no-repeat;
+ background-size: cover;
+}
+
+.task-bar {
+ width: 100%;
+ height: 55px;
+ color: white;
+ background-color: rgba(30, 33, 36, 0.979);
+ border-top: 1px solid rgb(101, 101, 121);
+ backdrop-filter: blur(5px);
+ position: sticky;
+ top: 100%;
+ display: flex;
+ justify-content: space-between;
+}
+
+.task-bar-left-box {
+ width: 30%;
+ height: 100%;
+ display: flex;
+}
+
+.task-bar-center-box {
+ width: 30%;
+ min-width: 550px;
+ height: 100%;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.window-start-button {
+ width: 45px;
+ min-width: 45px;
+ height: 45px;
+ border-radius: 5px;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.window-start-button:checked {
+ padding: 20px;
+ background-color: red;
+}
+
+.window-start-button:hover {
+ background-color: rgb(51, 54, 58);
+}
+
+.window-start-button-icon {
+ width: 26px;
+ height: 26px;
+}
+
+.task-bar-search-box {
+ margin: 5px;
+}
+
+.search-bar {
+ width: 220px;
+ height: 30px;
+ padding: 17px;
+ color: white;
+ background-color: rgba(129, 128, 128, 0.596);
+ border-radius: 30px;
+ border: 0;
+}
+
+.search-bar:hover {
+ background-color: rgba(141, 137, 137, 0.596);
+}
+
+.task-bar-app-box {
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.task-bar-app {
+ width: 45px;
+ height: 45px;
+ border-radius: 5px;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.task-bar-app:hover {
+ background-color: rgb(51, 54, 58);
+}
+
+.task-bar-app-icon {
+ width: 27px;
+ height: 27px;
+ margin: 2px;
+}
+
+.task-bar-right-box {
+ width: 30%;
+ height: 100%;
+ display: flex;
+ justify-content: flex-end;
+}
+
+.hidden-icons {
+ width: 30px;
+ padding: 10px;
+ margin: 5px 0 5px 0;
+ border-radius: 5px;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.hidden-icons:hover {
+ background-color: rgb(51, 54, 58);
+}
+
+.hidden-icons-icon {
+ width: 20px;
+}
+
+.language-box {
+ width: 45px;
+ margin: 5px 2px 5px 2px;
+ border-radius: 5px;
+ font-size: 12px;
+ text-align: center;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.language-box:hover {
+ background-color: rgb(51, 54, 58);
+}
+
+.quick-setting-box {
+ width: 80px;
+ min-width: 80px;
+ padding: 0 2px 0 2px;
+ margin: 5px 2px 5px 2px;
+ border-radius: 5px;
+ display: flex;
+ justify-content: space-around;
+ align-items: center;
+}
+
+.quick-setting-box:hover {
+ background-color: rgb(51, 54, 58);
+}
+
+.wifi-box {
+ width: 18px;
+ height: 15px;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.speaker-box {
+ width: 16px;
+ height: 16px;
+ mix-blend-mode:color-dodge;
+ display: flex;
+ justify-content: center;
+ align-items: center;
+}
+
+.battery-box {
+ width: 20px;
+ height: 22px;
+ font-size: 10.4px;
+ letter-spacing: 1px;
+ display: flex;
+ flex-direction: column;
+ justify-content: flex-start;
+ align-items: center;
+}
+
+.quick-setting-icon {
+ width: 100%;
+ height: 100%;
+}
+
+.time-date-box {
+ width: 115px;
+ margin: 5px;
+ padding-left: 5px;
+ border-radius: 5px;
+ display: flex;
+ justify-content: flex-end;
+ align-items: center;
+}
+
+.time-date-box:hover {
+ background-color: rgb(51, 54, 58);
+}
+
+.time-date {
+ width: 75px;
+ margin-right: 5px;
+ font-size: 12px;
+ text-align: end;
+}
+
+.time-box {
+ width: 100%;
+ letter-spacing: 1.2px;
+}
+
+.date-box {
+ width: 100%;
+ letter-spacing: 1.2px;
+}
+
+.notification-box {
+ width: 30px;
+}
+
+.notification-icon {
+ width: 22px;
+ height: 20px;
+}
\ No newline at end of file