|
| 1 | +# Data Structures and Algorithms in JavaScript |
| 2 | + |
| 3 | +This repository covers the implementation of the classical algorithms and data structures in JavaScript. |
| 4 | + |
| 5 | +<!-- This goes along with [these posts series](https://adrianmejia.com/tags/tutorial-algorithms/) that explain each implementation in details and also this [book](https://gum.co/dsajs) that explain these topics and some with more examples and illustrations. --> |
| 6 | + |
| 7 | +## Book |
| 8 | + |
| 9 | +You can check out the book that goes deeper into each topic and provide addtional illustrations and explanations. |
| 10 | + |
| 11 | +- Algorithmic toolbox to avoid getting stuck while coding. |
| 12 | +- Explains data structures similarities and differences. |
| 13 | +- Algorithm analysis fundamentals (Big O notation, Time/Space complexity) and examples. |
| 14 | +- Time/space complexity cheatsheet. |
| 15 | + |
| 16 | +<a href="https://gum.co/dsajs"><img src="book/cover.png" height="400px" alt="dsajs algorithms javascript book"></a> |
| 17 | + |
| 18 | +## Data Structures |
| 19 | +We are covering the following data structures. |
| 20 | + |
| 21 | +[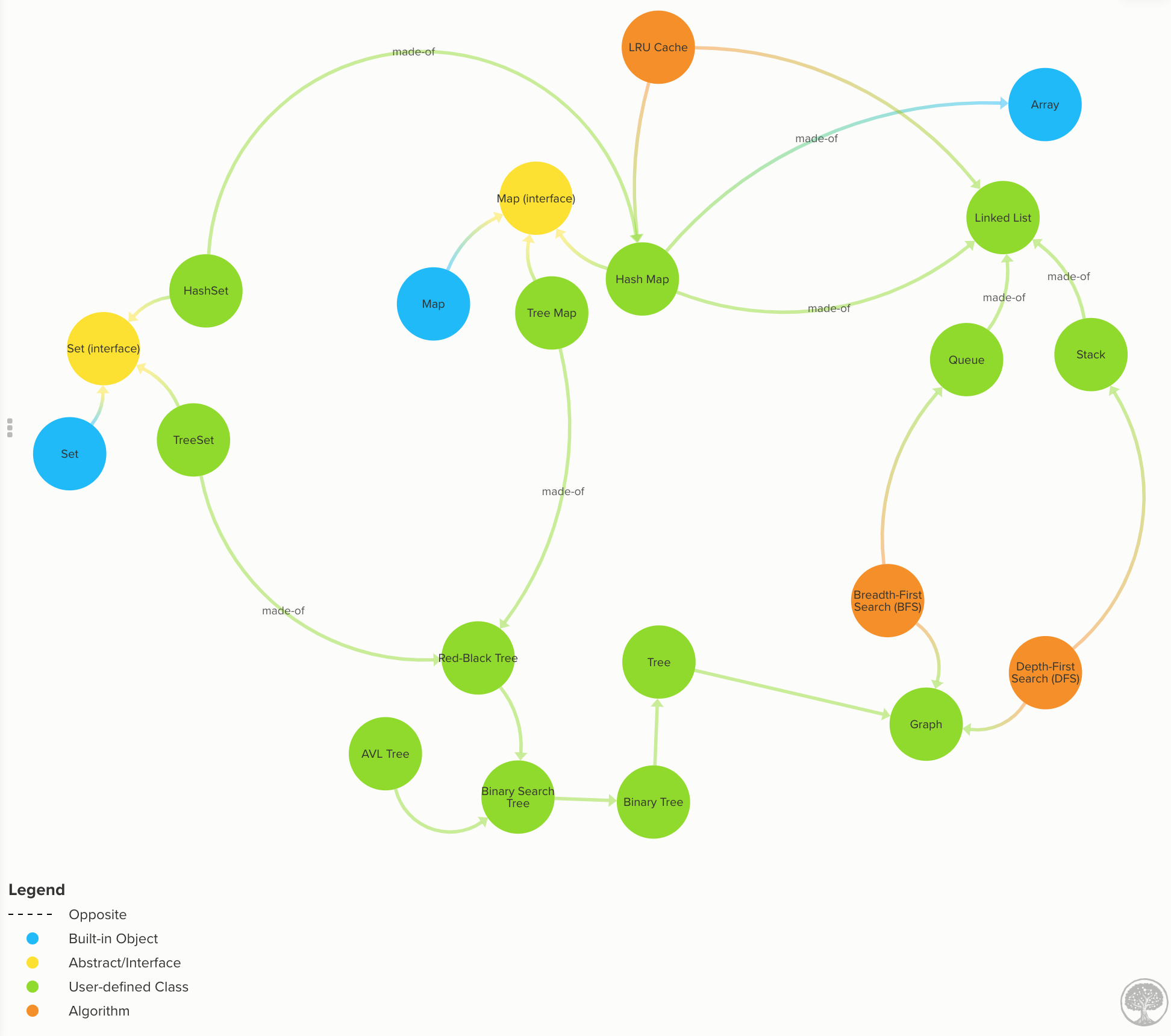](https://embed.kumu.io/85f1a4de5fb8430a10a1bf9c5118e015) |
| 22 | + |
| 23 | +### Linear Data Structures |
| 24 | +1. **Arrays**: Built-in in most languages so not implemented here. |
| 25 | + [Post](https://adrianmejia.com/blog/2018/04/28/data-structures-time-complexity-for-beginners-arrays-hashmaps-linked-lists-stacks-queues-tutorial/#Array). |
| 26 | + |
| 27 | +2. **Linked Lists**: each data node has a link to the next (and |
| 28 | + previous). |
| 29 | + [Code](https://github.com/amejiarosario/dsa.js/blob/master/src/data-structures/linked-lists/linked-list.js) |
| 30 | + | |
| 31 | + [Post](https://adrianmejia.com/blog/2018/04/28/data-structures-time-complexity-for-beginners-arrays-hashmaps-linked-lists-stacks-queues-tutorial/#Linked-Lists). |
| 32 | + |
| 33 | +3. **Queue**: data flows in a "first-in, first-out" (FIFO) manner. |
| 34 | + [Code](https://github.com/amejiarosario/dsa.js/blob/master/src/data-structures/queues/queue.js) |
| 35 | + | |
| 36 | + [Post](https://adrianmejia.com/blog/2018/04/28/data-structures-time-complexity-for-beginners-arrays-hashmaps-linked-lists-stacks-queues-tutorial/#Queues) |
| 37 | + |
| 38 | +4. **Stacks**: data flows in a "last-in, first-out" (LIFO) manner. |
| 39 | + [Code](https://github.com/amejiarosario/dsa.js/blob/master/src/data-structures/stacks/stack.js) |
| 40 | + | |
| 41 | + [Post](https://adrianmejia.com/blog/2018/04/28/data-structures-time-complexity-for-beginners-arrays-hashmaps-linked-lists-stacks-queues-tutorial/#Stacks). |
| 42 | + |
| 43 | +### Non-Linear Data Structures |
| 44 | +1. **Trees**: data nodes has zero or more adjacent nodes a.k.a. |
| 45 | + children. Each node can only have one parent node otherwise is a |
| 46 | + graph not a tree. |
| 47 | + [Code](https://github.com/amejiarosario/algorithms.js/tree/master/src/data-structures/trees) |
| 48 | + | |
| 49 | + [Post](https://adrianmejia.com/blog/2018/06/11/data-structures-for-beginners-trees-binary-search-tree-tutorial/) |
| 50 | + |
| 51 | + 1. **Binary Trees**: same as tree but only can have two children at |
| 52 | + most. |
| 53 | + [Code](https://github.com/amejiarosario/algorithms.js/tree/master/src/data-structures/trees) |
| 54 | + | |
| 55 | + [Post](https://adrianmejia.com/blog/2018/06/11/data-structures-for-beginners-trees-binary-search-tree-tutorial/#Binary-Trees) |
| 56 | + |
| 57 | + 2. **Binary Search Trees** (BST): same as binary tree, but the |
| 58 | + nodes value keep this order `left < parent < rigth`. |
| 59 | + [Code](https://github.com/amejiarosario/algorithms.js/blob/master/src/data-structures/trees/binary-search-tree.js) |
| 60 | + | |
| 61 | + [Post](https://adrianmejia.com/blog/2018/06/11/data-structures-for-beginners-trees-binary-search-tree-tutorial/#Binary-Search-Tree-BST) |
| 62 | + |
| 63 | + 3. **AVL Trees**: Self-balanced BST to maximize look up time. |
| 64 | + [Code](https://github.com/amejiarosario/algorithms.js/blob/master/src/data-structures/trees/avl-tree.js) |
| 65 | + | |
| 66 | + [Post](https://adrianmejia.com/blog/2018/07/16/self-balanced-binary-search-trees-with-avl-tree-data-structure-for-beginners/) |
| 67 | + |
| 68 | + 4. **Red-Black Trees**: Self-balanced BST more loose than AVL to |
| 69 | + maximize insertion speed. |
| 70 | + [Code](https://github.com/amejiarosario/algorithms.js/blob/master/src/data-structures/trees/red-black-tree.js) |
| 71 | + |
| 72 | +2. **Maps**: key-value store. |
| 73 | + |
| 74 | + 1. **Hash Maps**: implements map using a hash function. |
| 75 | + [Code](https://github.com/amejiarosario/algorithms.js/blob/master/src/data-structures/hash-maps/hashmap.js) |
| 76 | + | |
| 77 | + [Post](https://adrianmejia.com/blog/2018/04/28/data-structures-time-complexity-for-beginners-arrays-hashmaps-linked-lists-stacks-queues-tutorial/#HashMaps) |
| 78 | + |
| 79 | + 2. **Tree Maps**: implement map using a self-balanced BST. |
| 80 | + [Code](https://github.com/amejiarosario/dsa.js/blob/master/src/data-structures/maps/tree-maps/tree-map.js) |
| 81 | + |
| 82 | +3. **Graphs**: data **nodes** that can have a connection or **edge** to |
| 83 | + zero or more adjacent nodes. Unlike trees, nodes can have multiple |
| 84 | + parents, loops. |
| 85 | + [Code](https://github.com/amejiarosario/algorithms.js/blob/master/src/data-structures/graphs/graph.js) |
| 86 | + | |
| 87 | + [Post](https://adrianmejia.com/blog/2018/05/14/data-structures-for-beginners-graphs-time-complexity-tutorial/) |
| 88 | + |
| 89 | +## Algorithms |
| 90 | + |
| 91 | + - Sorting algorithms |
| 92 | + |
| 93 | + - Bubble Sort. |
| 94 | + [Code](https://github.com/amejiarosario/dsa.js/blob/master/src/algorithms/sorting/bubble-sort.js) |
| 95 | + |
| 96 | + - Insertion Sort. |
| 97 | + [Code](https://github.com/amejiarosario/dsa.js/blob/master/src/algorithms/sorting/insertion-sort.js) |
| 98 | + |
| 99 | + - Selection Sort. |
| 100 | + [Code](https://github.com/amejiarosario/dsa.js/blob/master/src/algorithms/sorting/selection-sort.js) |
| 101 | + |
| 102 | + - Merge Sort. |
| 103 | + [Code](https://github.com/amejiarosario/dsa.js/blob/master/src/algorithms/sorting/merge-sort.js) |
| 104 | + |
| 105 | + - Quicksort. |
| 106 | + [Code](https://github.com/amejiarosario/dsa.js/blob/master/src/algorithms/sorting/quick-sort.js) |
| 107 | + |
| 108 | + - Greedy Algorithms |
| 109 | + |
| 110 | + - Fractional Knapsack Problem. |
| 111 | + [Code](https://github.com/amejiarosario/dsa.js/blob/master/src/algorithms/knapsack-fractional.js) |
| 112 | + |
| 113 | + - Divide and Conquer |
| 114 | + |
| 115 | + - Fibonacci Numbers. |
| 116 | + [Code](https://github.com/amejiarosario/dsa.js/blob/master/src/algorithms/fibonacci-recursive.js) |
| 117 | + |
| 118 | + - Dynamic Programming |
| 119 | + |
| 120 | + - Fibonacci with memoization. |
| 121 | + [Code](https://github.com/amejiarosario/dsa.js/blob/master/src/algorithms/fibanacci-dynamic-programming.js) |
| 122 | + |
| 123 | + - Backtracking algorithms |
| 124 | + |
| 125 | + - Word permutations. |
| 126 | + [Code](https://github.com/amejiarosario/dsa.js/blob/master/src/algorithms/permutations-backtracking.js) |
0 commit comments