diff --git a/README.md b/README.md
index 14fcc69..15b1c90 100644
--- a/README.md
+++ b/README.md
@@ -9,46 +9,152 @@ Material Design Dropdown Component using React Native Paper, now also with multi
## Dependencies
- react-native-paper
+```bash
+react-native-paper
+```
## Installation
```bash
-
yarn add react-native-paper-dropdown
-
```
or
-```
-
+```bash
npm i react-native-paper-dropdown
-
```
## Demo
-## Latest Documentation :
+## Basic Example
+
+### Single Select
+
+```javascript
+import React, { useState } from 'react';
+import { View } from 'react-native';
+import { Dropdown } from 'react-native-paper-dropdown';
+import { Provider as PaperProvider } from 'react-native-paper';
+
+const OPTIONS = [
+ { label: 'Male', value: 'male' },
+ { label: 'Female', value: 'female' },
+ { label: 'Other', value: 'other' },
+];
+
+export default function App() {
+ const [gender, setGender] = useState();
+
+ return (
+
+
+
+
+
+ );
+}
+```
+
+### Multi Select
+
+```javascript
+import React, { useState } from 'react';
+import { View } from 'react-native';
+import { MultiSelectDropdown } from 'react-native-paper-dropdown';
+import { Provider as PaperProvider } from 'react-native-paper';
+
+const MULTI_SELECT_OPTIONS = [
+ { label: 'White', value: 'white' },
+ { label: 'Red', value: 'red' },
+ { label: 'Blue', value: 'blue' },
+ { label: 'Green', value: 'green' },
+ { label: 'Orange', value: 'orange' },
+];
+
+export default function App() {
+ const [colors, setColors] = useState([]);
+
+ return (
+
+
+
+
+
+ );
+}
+```
+
+## Props
+
+### `DropdownProps`
+
+| Prop | Type | Description |
+| --------------------- | ----------------------------------------------------------------- | -------------------------------------------- |
+| `value` | `string` | The currently selected value. |
+| `onSelect` | `(value: string) => void` | Callback function to handle value selection. |
+| `options` | `Option[]` | Array of options for the dropdown. |
+| `menuUpIcon` | `JSX.Element` | Custom icon for menu up state. |
+| `menuDownIcon` | `JSX.Element` | Custom icon for menu down state. |
+| `maxMenuHeight` | `number` | Maximum height of the dropdown menu. |
+| `menuContentStyle` | `ViewStyle` | Style for the dropdown menu content. |
+| `CustomDropdownItem` | `(props: DropdownItemProps) => JSX.Element` | Custom component for dropdown item. |
+| `CustomDropdownInput` | `(props: DropdownInputProps) => JSX.Element` | Custom component for dropdown input. |
+| `Touchable` | `ForwardRefExoticComponent>` | Custom touchable component for the dropdown. |
+| `testID` | `string` | Test ID for the dropdown component. |
+| `menuTestID` | `string` | Test ID for the dropdown menu. |
+| `placeholder` | `string` | Placeholder text for the dropdown input. |
+| `label` | `TextInputLabelProp` | Label for the dropdown input. |
+| `mode` | `'flat' \| 'outlined'` | Mode for the dropdown input. |
+| `disabled` | `boolean` | Whether the dropdown is disabled. |
+| `error` | `boolean` | Whether the dropdown has an error. |
+
+### `MultiSelectDropdownProps`
+
+| Prop | Type | Description |
+| -------------------------------- | ----------------------------------------------------------------- | ------------------------------------------------- |
+| `value` | `string[]` | The currently selected values. |
+| `onSelect` | `(value: string[]) => void` | Callback function to handle value selection. |
+| `options` | `Option[]` | Array of options for the dropdown. |
+| `menuUpIcon` | `JSX.Element` | Custom icon for menu up state. |
+| `menuDownIcon` | `JSX.Element` | Custom icon for menu down state. |
+| `Touchable` | `ForwardRefExoticComponent>` | Custom touchable component for the dropdown. |
+| `maxMenuHeight` | `number` | Maximum height of the dropdown menu. |
+| `menuContentStyle` | `ViewStyle` | Style for the dropdown menu content. |
+| `CustomMultiSelectDropdownItem` | `(props: MultiSelectDropdownItemProps) => JSX.Element` | Custom component for multi-select dropdown item. |
+| `CustomMultiSelectDropdownInput` | `(props: DropdownInputProps) => JSX.Element` | Custom component for multi-select dropdown input. |
+| `testID` | `string` | Test ID for the dropdown component. |
+| `menuTestID` | `string` | Test ID for the dropdown menu. |
+| `placeholder` | `string` | Placeholder text for the dropdown input. |
+| `label` | `TextInputLabelProp` | Label for the dropdown input. |
+| `mode` | `'flat' \| 'outlined'` | Mode for the dropdown input. |
+| `disabled` | `boolean` | Whether the dropdown is disabled. |
+| `error` | `boolean` | Whether the dropdown has an error. |
+
+## Latest Documentation
- [https://fateh999.github.io/react-native-paper-dropdown](https://fateh999.github.io/react-native-paper-dropdown)
-## v1 Documentation :
+## v1 Documentation
- [https://fateh999.github.io/react-native-paper-dropdown/#/old-version](https://fateh999.github.io/react-native-paper-dropdown/#/old-version)
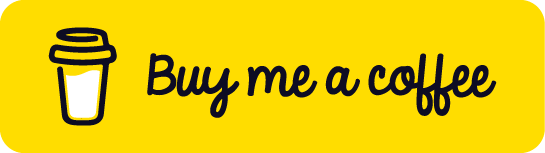
-## Contributing
-
-See the [contributing guide](CONTRIBUTING.md) to learn how to contribute to the repository and the development workflow.
-
## License
MIT
-
----
-
-Made with [create-react-native-library](https://github.com/callstack/react-native-builder-bob)
diff --git a/docs/README.md b/docs/README.md
index 2d430f7..05e1176 100644
--- a/docs/README.md
+++ b/docs/README.md
@@ -5,39 +5,109 @@
[](https://www.npmjs.com/package/react-native-paper-dropdown)
[](https://github.com/fateh999/react-native-paper-dropdown/blob/master/LICENSE)
-Material Design Dropdown Component using React Native Paper
-
-## Dependencies
-
- react-native-paper
+A Material Design Dropdown Component for React Native Paper
## Installation
-```bash
+To install the package, run:
+```bash
yarn add react-native-paper-dropdown
-
```
or
+```bash
+npm i react-native-paper-dropdown
```
-npm i react-native-paper-dropdown
+## Dependencies
+This package depends on `react-native-paper`. Ensure it's installed in your project:
+
+```bash
+yarn add react-native-paper
```
## Basic Example
+### Single Select
+
+```javascript
+import React, { useState } from 'react';
+import { View } from 'react-native';
+import { Dropdown } from 'react-native-paper-dropdown';
+import { Provider as PaperProvider } from 'react-native-paper';
+
+const OPTIONS = [
+ { label: 'Male', value: 'male' },
+ { label: 'Female', value: 'female' },
+ { label: 'Other', value: 'other' },
+];
+
+export default function App() {
+ const [gender, setGender] = useState();
+
+ return (
+
+
+
+
+
+ );
+}
+```
+
+### Multi Select
+
+```javascript
+import React, { useState } from 'react';
+import { View } from 'react-native';
+import { MultiSelectDropdown } from 'react-native-paper-dropdown';
+import { Provider as PaperProvider } from 'react-native-paper';
+
+const MULTI_SELECT_OPTIONS = [
+ { label: 'White', value: 'white' },
+ { label: 'Red', value: 'red' },
+ { label: 'Blue', value: 'blue' },
+ { label: 'Green', value: 'green' },
+ { label: 'Orange', value: 'orange' },
+];
+
+export default function App() {
+ const [colors, setColors] = useState([]);
+
+ return (
+
+
+
+
+
+ );
+}
+```
+
+## Advanced Example
+
```javascript
-import { useMemo, useState } from 'react';
+import React, { useMemo, useState } from 'react';
import { ScrollView, StyleSheet, View, ViewStyle } from 'react-native';
import {
Appbar,
Divider,
Headline,
- MD3DarkTheme,
- MD3LightTheme,
PaperProvider,
Paragraph,
TextInput,
@@ -58,26 +128,11 @@ const OPTIONS = [
];
const MULTI_SELECT_OPTIONS = [
- {
- label: 'White',
- value: 'white',
- },
- {
- label: 'Red',
- value: 'red',
- },
- {
- label: 'Blue',
- value: 'blue',
- },
- {
- label: 'Green',
- value: 'green',
- },
- {
- label: 'Orange',
- value: 'orange',
- },
+ { label: 'White', value: 'white' },
+ { label: 'Red', value: 'red' },
+ { label: 'Blue', value: 'blue' },
+ { label: 'Green', value: 'green' },
+ { label: 'Orange', value: 'orange' },
];
const CustomDropdownItem = ({
@@ -93,9 +148,7 @@ const CustomDropdownItem = ({
height: 50,
width,
backgroundColor:
- value === option.value
- ? MD3DarkTheme.colors.primary
- : MD3DarkTheme.colors.onPrimary,
+ value === option.value ? MD3DarkTheme.colors.primary : MD3DarkTheme.colors.onPrimary,
justifyContent: 'center',
paddingHorizontal: 16,
}),
@@ -113,10 +166,7 @@ const CustomDropdownItem = ({
>
{option.label}
@@ -131,24 +181,20 @@ const CustomDropdownInput = ({
placeholder,
selectedLabel,
rightIcon,
-}: DropdownInputProps) => {
- return (
-
- );
-};
+}: DropdownInputProps) => (
+
+);
export default function App() {
- const [nightMode, setNightmode] = useState(false);
+ const [nightMode, setNightMode] = useState(false);
const [gender, setGender] = useState();
const [colors, setColors] = useState([]);
const Theme = nightMode ? MD3DarkTheme : MD3LightTheme;
@@ -156,95 +202,65 @@ export default function App() {
return (
-
+
-
+
setNightmode(!nightMode)}
+ onPress={() => setNightMode(!nightMode)}
/>
-
+
Single Select
-
Default Dropdown
-
Default Dropdown (Outline Mode)
-
Custom Dropdown
- }
- menuDownIcon={
-
- }
+ menuContentStyle={{ backgroundColor: MD3DarkTheme.colors.onPrimary }}
+ menuUpIcon={}
+ menuDownIcon={}
CustomDropdownItem={CustomDropdownItem}
CustomDropdownInput={CustomDropdownInput}
/>
-
-
-
Multi Select
-
Default Dropdown
-
Default Dropdown (Outline Mode)
@@ -261,9 +277,6 @@ const styles = StyleSheet.create({
formWrapper: {
margin: 16,
},
- spacer: {
- height: 16,
- },
});
```
@@ -273,85 +286,52 @@ const styles = StyleSheet.create({
## Props
-```typescript
-import { ForwardRefExoticComponent } from 'react';
-import { PressableProps, View, ViewStyle } from 'react-native';
-import { TextInputLabelProp } from 'react-native-paper/lib/typescript/components/TextInput/types';
-import { TextInputProps } from 'react-native-paper';
-
-export type DropdownInputProps = {
- placeholder?: string;
- label?: TextInputLabelProp;
- rightIcon: JSX.Element;
- selectedLabel?: string;
- mode?: 'flat' | 'outlined';
- disabled?: boolean;
- error?: boolean;
-};
-
-export type Option = {
- label: string;
- value: string;
-};
-
-export type DropdownProps = {
- value?: string;
- onSelect?: (value: string) => void;
- options: Option[];
- menuUpIcon?: JSX.Element;
- menuDownIcon?: JSX.Element;
- maxMenuHeight?: number;
- menuContentStyle?: ViewStyle;
- CustomDropdownItem?: (props: DropdownItemProps) => JSX.Element;
- CustomDropdownInput?: (props: DropdownInputProps) => JSX.Element;
- Touchable?: ForwardRefExoticComponent<
- PressableProps & React.RefAttributes
- >;
- testID?: string;
- menuTestID?: string;
-} & Pick<
- TextInputProps,
- 'placeholder' | 'label' | 'mode' | 'disabled' | 'error'
->;
-
-export type MultiSelectDropdownProps = {
- value?: string[];
- onSelect?: (value: string[]) => void;
- options: Option[];
- menuUpIcon?: JSX.Element;
- menuDownIcon?: JSX.Element;
- Touchable?: ForwardRefExoticComponent<
- PressableProps & React.RefAttributes
- >;
- maxMenuHeight?: number;
- menuContentStyle?: ViewStyle;
- CustomMultiSelectDropdownItem?: (
- props: MultiSelectDropdownItemProps
- ) => JSX.Element;
- CustomMultiSelectDropdownInput?: (props: DropdownInputProps) => JSX.Element;
- testID?: string;
- menuTestID?: string;
-} & Pick<
- TextInputProps,
- 'placeholder' | 'label' | 'mode' | 'disabled' | 'error'
->;
-
-export type DropdownItemProps = {
- option: Option;
- value?: string;
- onSelect?: (value: string) => void;
- width: number;
- toggleMenu: () => void;
- isLast: boolean;
- menuItemTestID?: string;
-};
-
-export type MultiSelectDropdownItemProps = {
- option: Option;
- value?: string[];
- onSelect?: (value: string[]) => void;
- width: number;
- isLast: boolean;
- menuItemTestID?: string;
-};
-```
+### `DropdownProps`
+
+| Prop | Type | Description |
+| --------------------- | ----------------------------------------------------------------- | -------------------------------------------- |
+| `value` | `string` | The currently selected value. |
+| `onSelect` | `(value: string) => void` | Callback function to handle value selection. |
+| `options` | `Option[]` | Array of options for the dropdown. |
+| `menuUpIcon` | `JSX.Element` | Custom icon for menu up state. |
+| `menuDownIcon` | `JSX.Element` | Custom icon for menu down state. |
+| `maxMenuHeight` | `number` | Maximum height of the dropdown menu. |
+| `menuContentStyle` | `ViewStyle` | Style for the dropdown menu content. |
+| `CustomDropdownItem` | `(props: DropdownItemProps) => JSX.Element` | Custom component for dropdown item. |
+| `CustomDropdownInput` | `(props: DropdownInputProps) => JSX.Element` | Custom component for dropdown input. |
+| `Touchable` | `ForwardRefExoticComponent>` | Custom touchable component for the dropdown. |
+| `testID` | `string` | Test ID for the dropdown component. |
+| `menuTestID` | `string` | Test ID for the dropdown menu. |
+| `placeholder` | `string` | Placeholder text for the dropdown input. |
+| `label` | `TextInputLabelProp` | Label for the dropdown input. |
+| `mode` | `'flat' \| 'outlined'` | Mode for the dropdown input. |
+| `disabled` | `boolean` | Whether the dropdown is disabled. |
+| `error` | `boolean` | Whether the dropdown has an error. |
+
+### `MultiSelectDropdownProps`
+
+| Prop | Type | Description |
+| -------------------------------- | ----------------------------------------------------------------- | ------------------------------------------------- |
+| `value` | `string[]` | The currently selected values. |
+| `onSelect` | `(value: string[]) => void` | Callback function to handle value selection. |
+| `options` | `Option[]` | Array of options for the dropdown. |
+| `menuUpIcon` | `JSX.Element` | Custom icon for menu up state. |
+| `menuDownIcon` | `JSX.Element` | Custom icon for menu down state. |
+| `Touchable` | `ForwardRefExoticComponent>` | Custom touchable component for the dropdown. |
+| `maxMenuHeight` | `number` | Maximum height of the dropdown menu. |
+| `menuContentStyle` | `ViewStyle` | Style for the dropdown menu content. |
+| `CustomMultiSelectDropdownItem` | `(props: MultiSelectDropdownItemProps) => JSX.Element` | Custom component for multi-select dropdown item. |
+| `CustomMultiSelectDropdownInput` | `(props: DropdownInputProps) => JSX.Element` | Custom component for multi-select dropdown input. |
+| `testID` | `string` | Test ID for the dropdown component. |
+| `menuTestID` | `string` | Test ID for the dropdown menu. |
+| `placeholder` | `string` | Placeholder text for the dropdown input. |
+| `label` | `TextInputLabelProp` | Label for the dropdown input. |
+| `mode` | `'flat' \| 'outlined'` | Mode for the dropdown input. |
+| `disabled` | `boolean` | Whether the dropdown is disabled. |
+| `error` | `boolean` | Whether the dropdown has an error. |
+
+## Customization
+
+You can customize the appearance and behavior of the dropdowns by using the provided props like `menuUpIcon`, `menuDownIcon`, `CustomDropdownItem`, `CustomDropdownInput`, and `menuContentStyle`.
+
+Enjoy using `react-native-paper-dropdown`!