diff --git a/.env.example b/.env.example
index 2d70725b..1a5bb2f6 100644
--- a/.env.example
+++ b/.env.example
@@ -1,8 +1,9 @@
OPENAI_API_KEY="xx"
OTHER_API_KEY="yy"
-MINDWARE_URL="xx"
-MINDWARE_API_KEY="zz"
+PAYMENT_GATEWAY_URL=https://agent-payments-gateway.vercel.app/payment
CONFIG_PATH=examples/example_agent_setup.json
PRODUCT_CATALOG=examples/sample_product_catalog.txt
+PRODUCT_PRICE_MAPPING=examples/example_product_price_id_mapping.json
GPT_MODEL=gpt-3.5-turbo-0613
-USE_TOOLS_IN_API=True
\ No newline at end of file
+USE_TOOLS_IN_API=True
+
diff --git a/README.md b/README.md
index 828d7033..a92c912d 100644
--- a/README.md
+++ b/README.md
@@ -35,11 +35,13 @@ We are building SalesGPT to power your best AI Sales Agents. Hence, we would lov
# Demos and Use Cases
-Unload AI Sales Agent Demo - Powered by SalesGPT: *A New Way to Sell?* 🤔
+Unload AI Sales Agent Demos - Powered by SalesGPT: *Our new virtual workforce?* 🤔
-**Demo #1: Rachel - Mattress Sales Field Representative Outbound Sales**
+**Demo #1: Sarah - Patient Coordinator at South Orange Pediatrics**
-[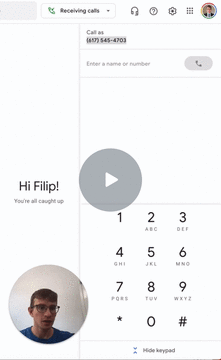](https://www.loom.com/share/f0fac42954904471b266980e4948b07d)
+- 100X Your Healthcare Admin with our Virtual Workforce
+
+[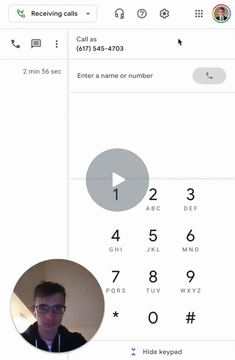](https://www.loom.com/share/314eb0562fda41ea94e25b267acda6f9)
**Demo #2: Ted - Autonomously create payment links and collect customer payments**
diff --git a/examples/example_product_price_id_mapping.json b/examples/example_product_price_id_mapping.json
new file mode 100644
index 00000000..e1f461d1
--- /dev/null
+++ b/examples/example_product_price_id_mapping.json
@@ -0,0 +1,7 @@
+{
+ "ai-consulting-services": "price_1Ow8ofB795AYY8p1goWGZi6m",
+ "Luxury Cloud-Comfort Memory Foam Mattress": "price_1Owv99B795AYY8p1mjtbKyxP",
+ "Classic Harmony Spring Mattress": "price_1Owv9qB795AYY8p1tPcxCM6T",
+ "EcoGreen Hybrid Latex Mattress": "price_1OwvLDB795AYY8p1YBAMBcbi",
+ "Plush Serenity Bamboo Mattress": "price_1OwvMQB795AYY8p1hJN2uS3S"
+}
\ No newline at end of file
diff --git a/examples/sales_agent_with_context.ipynb b/examples/sales_agent_with_context.ipynb
index c9024cbb..462eac64 100644
--- a/examples/sales_agent_with_context.ipynb
+++ b/examples/sales_agent_with_context.ipynb
@@ -67,38 +67,6 @@
"from typing import Union\n"
]
},
- {
- "cell_type": "markdown",
- "metadata": {},
- "source": [
- "### Optional - create a free [Mindware](https://www.mindware.co/) account\n",
- "\n",
- "This enables your AI sales agents to actually sell."
- ]
- },
- {
- "cell_type": "code",
- "execution_count": 2,
- "metadata": {},
- "outputs": [
- {
- "name": "stdout",
- "output_type": "stream",
- "text": [
- "I want to sell\n"
- ]
- }
- ],
- "source": [
- "I_WANT_TO_SELL=True\n",
- "if I_WANT_TO_SELL:\n",
- " if \"MINDWARE_API_KEY\" not in os.environ:\n",
- " raise ValueError(\"You cannot sell as you did not set up a Mindware account\")\n",
- " print(\"I want to sell\")\n",
- "\n",
- "MINDWARE_URL = os.getenv(\"MINDWARE_URL\", \"https://agent-payments-gateway.vercel.app/payment\")"
- ]
- },
{
"attachments": {},
"cell_type": "markdown",
@@ -471,6 +439,63 @@
"product_catalog='sample_product_catalog.txt'"
]
},
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "from litellm import completion\n",
+ "import json\n",
+ "\n",
+ "def get_product_id_from_query(query, product_price_id_mapping_path):\n",
+ " # Load product_price_id_mapping from a JSON file\n",
+ " with open(product_price_id_mapping_path, 'r') as f:\n",
+ " product_price_id_mapping = json.load(f)\n",
+ " \n",
+ " # Serialize the product_price_id_mapping to a JSON string for inclusion in the prompt\n",
+ " product_price_id_mapping_json_str = json.dumps(product_price_id_mapping)\n",
+ " \n",
+ " # Dynamically create the enum list from product_price_id_mapping keys\n",
+ " enum_list = list(product_price_id_mapping.values()) + [\"No relevant product id found\"]\n",
+ " enum_list_str = json.dumps(enum_list)\n",
+ " \n",
+ " prompt = f\"\"\"\n",
+ " You are an expert data scientist and you are working on a project to recommend products to customers based on their needs.\n",
+ " Given the following query:\n",
+ " {query}\n",
+ " and the following product price id mapping:\n",
+ " {product_price_id_mapping_json_str}\n",
+ " return the product id that is most relevant to the query.\n",
+ " ONLY return the product id, no other text. If no relevant product id is found, return 'No relevant product id found'.\n",
+ " FORMAT OUTPUT AS the following schema:\n",
+ " {{\n",
+ " \"$schema\": \"http://json-schema.org/draft-07/schema#\",\n",
+ " \"title\": \"Product ID Response\",\n",
+ " \"type\": \"object\",\n",
+ " \"properties\": {{\n",
+ " \"product_id\": {{\n",
+ " \"type\": \"string\",\n",
+ " \"enum\": {enum_list_str}\n",
+ " }}\n",
+ " }},\n",
+ " \"required\": [\"product_id\"]\n",
+ " }}\n",
+ " \"\"\"\n",
+ " \n",
+ " response = completion(\n",
+ " model=os.getenv(\"GPT_MODEL\", \"gpt-3.5-turbo-1106\"),\n",
+ " messages=[{\"content\": prompt, \"role\": \"user\"}],\n",
+ " max_tokens=1000,\n",
+ " temperature=0\n",
+ " )\n",
+ "\n",
+ " product_id = response.choices[0].message.content.strip()\n",
+ " return product_id\n",
+ " \n",
+ "\n"
+ ]
+ },
{
"cell_type": "code",
"execution_count": 10,
@@ -483,16 +508,21 @@
"def generate_stripe_payment_link(query: str) -> str:\n",
" \"\"\"Generate a stripe payment link for a customer based on a single query string.\"\"\"\n",
"\n",
- " url = os.getenv(\"MINDWARE_URL\", \"\")\n",
- " api_key = os.getenv(\"MINDWARE_API_KEY\", \"\")\n",
+ " # example testing payment gateway url\n",
+ " PAYMENT_GATEWAY_URL = os.getenv(\"PAYMENT_GATEWAY_URL\", \"https://agent-payments-gateway.vercel.app/payment\")\n",
+ " PRODUCT_PRICE_MAPPING = os.getenv(\"PRODUCT_PRICE_MAPPING\", \"example_product_price_id_mapping.json\")\n",
+ " \n",
+ " # use LLM to get the price_id from query\n",
+ " price_id = get_product_id_from_query(query, PRODUCT_PRICE_MAPPING)\n",
"\n",
- " payload = json.dumps({\"prompt\": query})\n",
+ " payload = json.dumps({\"prompt\": query,\n",
+ " \"price_id\": price_id\n",
+ " })\n",
" headers = {\n",
" 'Content-Type': 'application/json',\n",
- " 'Authorization': f'Bearer {api_key}'\n",
" }\n",
"\n",
- " response = requests.request(\"POST\", url, headers=headers, data=payload)\n",
+ " response = requests.request(\"POST\", PAYMENT_GATEWAY_URL, headers=headers, data=payload)\n",
" return response.text"
]
},