diff --git a/.github/workflows/PublishIncrementalNuget.yml b/.github/workflows/PublishIncrementalNuget.yml
new file mode 100644
index 00000000..0be36513
--- /dev/null
+++ b/.github/workflows/PublishIncrementalNuget.yml
@@ -0,0 +1,49 @@
+name: Publish Incremental Nuget Release
+
+on:
+ workflow_dispatch:
+
+jobs:
+ build:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout
+ uses: actions/checkout@v4
+
+ - name: Setup .NET
+ uses: actions/setup-dotnet@v3
+ with:
+ dotnet-version: 8.0.x
+
+ - name: Bump build version
+ id: bump
+ uses: vers-one/dotnet-project-version-updater@v1.5
+ with:
+ files: |
+ "**/Consolonia.csproj"
+ version: bump-build
+
+ - name: Restore dependencies
+ run: dotnet restore src/Consolonia.sln
+
+ - name: Build
+ run: dotnet build src/Consolonia.sln -c Release --no-restore
+
+ - name: dotnet pack
+ run: |
+ dotnet pack src/Consolonia.sln -c Release -o packages --include-symbols --property WarningLevel=0
+
+ - name: Publish NuGet and symbols
+ id: nuget-push
+ uses: edumserrano/nuget-push@v1
+ with:
+ api-key: '${{ secrets.NUGET_KEY }}'
+ working-directory: 'packages'
+ fail-if-exists: false
+
+ - name: Commit new version changes
+ run: |
+ git config --global user.name "Github Action"
+ git config --global user.email "jinek@users.noreply.github.com"
+ git commit -a -m "Bumped version for published nuget artifacts"
+ git push
diff --git a/.github/workflows/PublishNuget.yml b/.github/workflows/PublishNuget.yml
new file mode 100644
index 00000000..aca0ffa0
--- /dev/null
+++ b/.github/workflows/PublishNuget.yml
@@ -0,0 +1,35 @@
+name: Publish Nuget Release
+
+on:
+ workflow_dispatch:
+
+jobs:
+ build:
+ runs-on: ubuntu-latest
+ steps:
+ - name: Checkout
+ uses: actions/checkout@v4
+
+ - name: Setup .NET
+ uses: actions/setup-dotnet@v3
+ with:
+ dotnet-version: 8.0.x
+
+ - name: Restore dependencies
+ run: dotnet restore src/Consolonia.sln
+
+ - name: Build
+ run: dotnet build src/Consolonia.sln -c Release --no-restore
+
+ - name: dotnet pack
+ run: |
+ dotnet pack src/Consolonia.sln -c Release -o packages --include-symbols --property WarningLevel=0
+
+ - name: Publish NuGet and symbols
+ id: nuget-push
+ uses: edumserrano/nuget-push@v1
+ with:
+ api-key: '${{ secrets.NUGET_KEY }}'
+ working-directory: 'packages'
+ fail-if-exists: false
+
diff --git a/Directory.Build.props b/Directory.Build.props
index 5c03ebf1..5ac9b88e 100644
--- a/Directory.Build.props
+++ b/Directory.Build.props
@@ -5,13 +5,18 @@
disable
- 11.0.9
+ 11.2.1
https://github.com/jinek/Consolonia/graphs/contributors
Text User Interface implementation of Avalonia UI (GUI Framework)
Copyright © Evgeny Gorbovoy 2021 - 2022
+ Icon.png
AVA3001
11.2.1
+
+
+
+
\ No newline at end of file
diff --git a/src/Consolonia.Blazor/README.md b/src/Consolonia.Blazor/README.md
new file mode 100644
index 00000000..92292772
--- /dev/null
+++ b/src/Consolonia.Blazor/README.md
@@ -0,0 +1,14 @@
+# Consolonia UI
+
+TUI (Text User Interface) (GUI Framework) implementation for [Avalonia UI](https://github.com/AvaloniaUI)
+
+Supports XAML, data bindings, animation, styling and the rest from Avalonia.
+
+> Project is in proof of concept state and is looking for collaboration.
+
+## Showcase (click picture to see video)
+[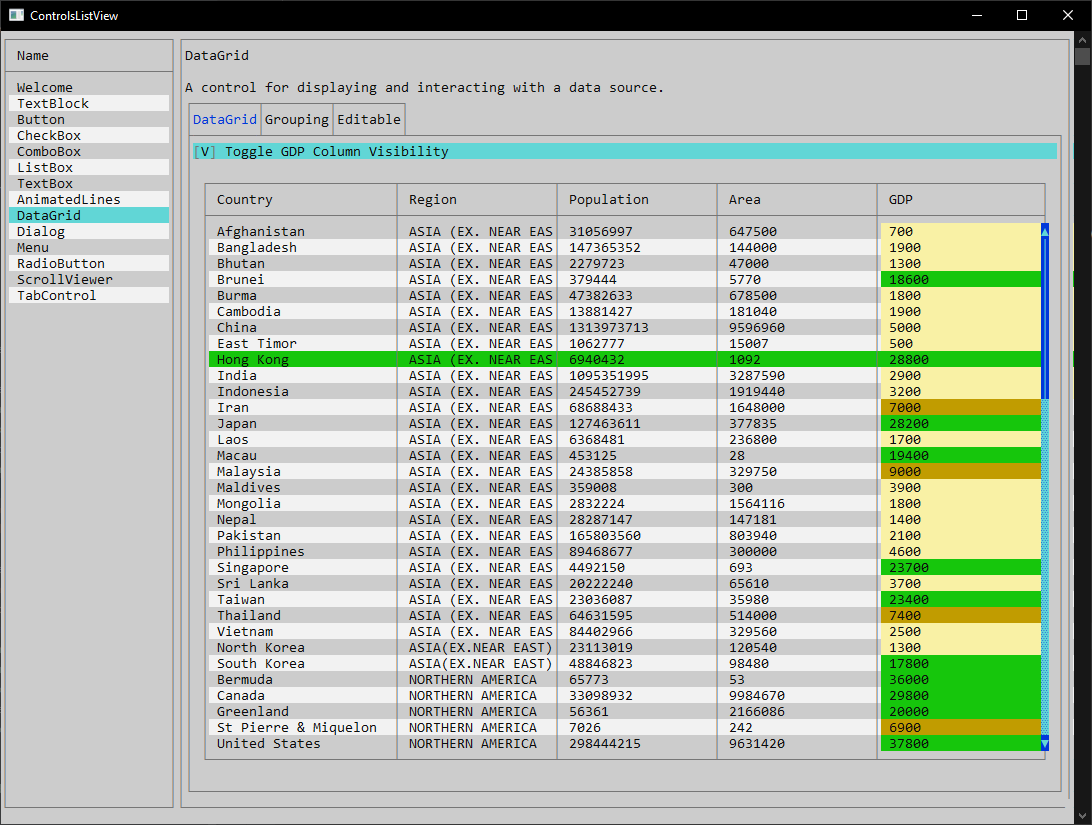](https://youtu.be/ttgZmbruk3Y)
+
+Example of usage: https://github.com/jinek/ToolUI
+
+Solution contains one more readme file with coding information.
diff --git a/src/Consolonia.Core/ApplicationStartup.cs b/src/Consolonia.Core/ApplicationStartup.cs
index 4570055f..498dcb68 100644
--- a/src/Consolonia.Core/ApplicationStartup.cs
+++ b/src/Consolonia.Core/ApplicationStartup.cs
@@ -6,10 +6,10 @@
using Consolonia.Core.Infrastructure;
// ReSharper disable UnusedMember.Global //todo: how to disable it for public methods?
-
+// ReSharper disable CheckNamespace
// ReSharper disable MemberCanBePrivate.Global
-namespace Consolonia.Core
+namespace Consolonia
{
public static class ApplicationStartup
{
diff --git a/src/Consolonia.Core/Infrastructure/ConsoloniaApplication.cs b/src/Consolonia.Core/Infrastructure/ConsoloniaApplication.cs
index cda946f7..9d0e778a 100644
--- a/src/Consolonia.Core/Infrastructure/ConsoloniaApplication.cs
+++ b/src/Consolonia.Core/Infrastructure/ConsoloniaApplication.cs
@@ -1,5 +1,7 @@
+using System;
using Avalonia;
using Avalonia.Controls;
+using Avalonia.Controls.ApplicationLifetimes;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Media;
@@ -59,4 +61,20 @@ public override void OnFrameworkInitializationCompleted()
}
}
}
+
+ public class ConsoloniaApplication : ConsoloniaApplication
+ where TMainWindow : Window, new()
+ {
+ public override void OnFrameworkInitializationCompleted()
+ {
+ if (ApplicationLifetime is IClassicDesktopStyleApplicationLifetime desktop)
+ {
+ var window = Activator.CreateInstance();
+ ArgumentNullException.ThrowIfNull(window, typeof(TMainWindow).Name);
+ desktop.MainWindow = window;
+ }
+
+ base.OnFrameworkInitializationCompleted();
+ }
+ }
}
\ No newline at end of file
diff --git a/src/Consolonia.Core/Infrastructure/ConsoloniaNotSupportedException.cs b/src/Consolonia.Core/Infrastructure/ConsoloniaNotSupportedException.cs
index 11394801..0e794a63 100644
--- a/src/Consolonia.Core/Infrastructure/ConsoloniaNotSupportedException.cs
+++ b/src/Consolonia.Core/Infrastructure/ConsoloniaNotSupportedException.cs
@@ -3,7 +3,6 @@
namespace Consolonia.Core.Infrastructure
{
- [Serializable]
public class ConsoloniaNotSupportedException : Exception
{
internal ConsoloniaNotSupportedException(NotSupportedRequest request)
diff --git a/src/Consolonia.Core/Infrastructure/ExceptionSink.cs b/src/Consolonia.Core/Infrastructure/ExceptionSink.cs
index 717fdf66..77c36bf8 100644
--- a/src/Consolonia.Core/Infrastructure/ExceptionSink.cs
+++ b/src/Consolonia.Core/Infrastructure/ExceptionSink.cs
@@ -1,10 +1,9 @@
using System;
using System.Globalization;
-using Avalonia;
using Avalonia.Logging;
namespace Consolonia.Core.Infrastructure
- // ReSharper disable once ArrangeNamespaceBody
+// ReSharper disable once ArrangeNamespaceBody
{
public class ExceptionSink : ILogSink
{
@@ -55,14 +54,5 @@ public void Log(LogEventLevel level, string area, object source, string messageT
throw consoloniaException;
}
}
-
- public static class ExceptionSinkExtensions
- {
- public static AppBuilder LogToException(this AppBuilder builder)
- {
- Logger.Sink = new ExceptionSink();
- return builder;
- }
- }
// ReSharper restore UnusedMember.Global
}
\ No newline at end of file
diff --git a/src/Consolonia.Core/Infrastructure/ExceptionSinkExtensions.cs b/src/Consolonia.Core/Infrastructure/ExceptionSinkExtensions.cs
new file mode 100644
index 00000000..ea4df246
--- /dev/null
+++ b/src/Consolonia.Core/Infrastructure/ExceptionSinkExtensions.cs
@@ -0,0 +1,18 @@
+using Avalonia;
+using Avalonia.Logging;
+using Consolonia.Core.Infrastructure;
+
+// ReSharper disable CheckNamespace
+namespace Consolonia
+// ReSharper disable once ArrangeNamespaceBody
+{
+ public static class ExceptionSinkExtensions
+ {
+ public static AppBuilder LogToException(this AppBuilder builder)
+ {
+ Logger.Sink = new ExceptionSink();
+ return builder;
+ }
+ }
+ // ReSharper restore UnusedMember.Global
+}
\ No newline at end of file
diff --git a/src/Consolonia.Core/README.md b/src/Consolonia.Core/README.md
new file mode 100644
index 00000000..c83aa315
--- /dev/null
+++ b/src/Consolonia.Core/README.md
@@ -0,0 +1,11 @@
+# Consolonia.PlatformSupport
+This package is the core Consolonia library.
+
+## Background
+Consolonia is a TUI (Text User Interface) (GUI Framework) implementation for [Avalonia UI](https://github.com/AvaloniaUI)
+
+Supports XAML, data bindings, animation, styling and the rest from Avalonia.
+
+## Showcase (click picture to see video)
+[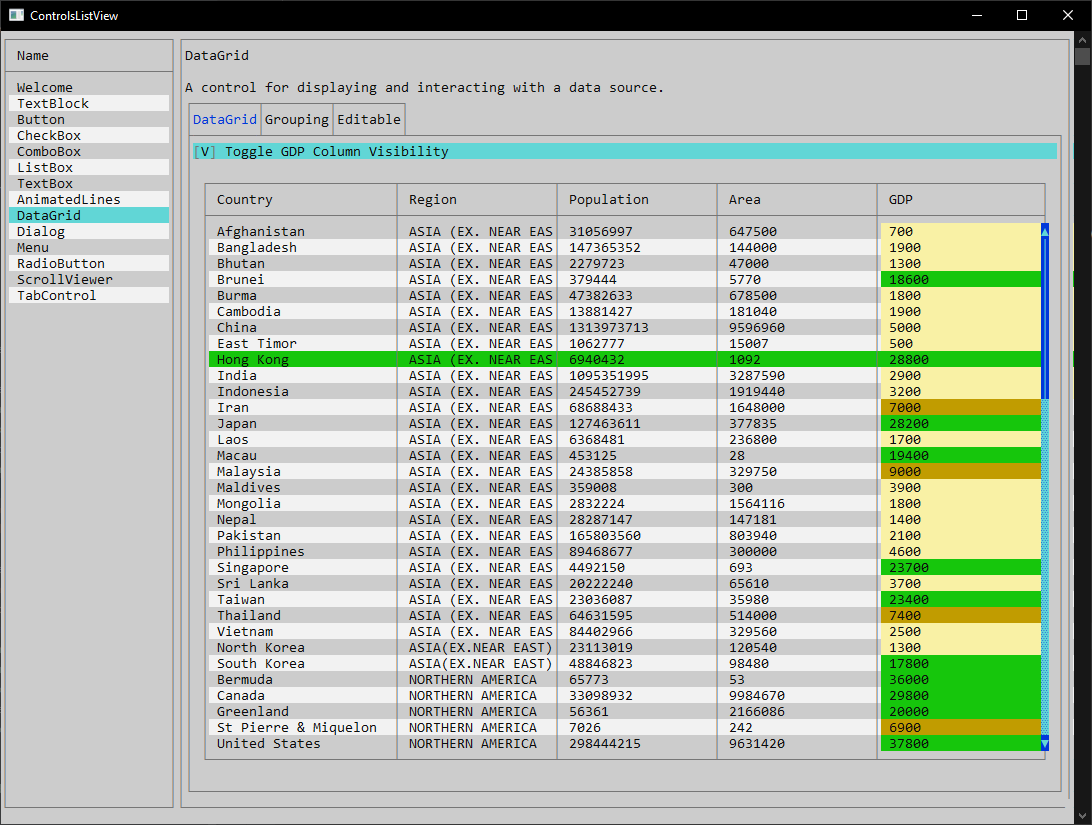](https://youtu.be/ttgZmbruk3Y)
+
diff --git a/src/Consolonia.Designer/Consolonia.Designer.csproj b/src/Consolonia.Designer/Consolonia.Designer.csproj
deleted file mode 100644
index d36a8f58..00000000
--- a/src/Consolonia.Designer/Consolonia.Designer.csproj
+++ /dev/null
@@ -1,18 +0,0 @@
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
diff --git a/src/Consolonia.Gallery/App.cs b/src/Consolonia.Gallery/App.cs
index a96ace76..6d9cfccd 100644
--- a/src/Consolonia.Gallery/App.cs
+++ b/src/Consolonia.Gallery/App.cs
@@ -1,13 +1,12 @@
using System.Globalization;
using System.Threading;
-using Avalonia.Controls.ApplicationLifetimes;
using Consolonia.Core.Infrastructure;
using Consolonia.Gallery.View;
-using Consolonia.Themes.TurboVision.Themes.Fluent;
+using Consolonia.Themes;
namespace Consolonia.Gallery
{
- internal class App : ConsoloniaApplication
+ internal class App : ConsoloniaApplication
{
static App()
{
@@ -23,14 +22,5 @@ public App()
Styles.Add(new FluentTheme());
/*Styles.Add(new MaterialTheme());*/
}
-
- public override void OnFrameworkInitializationCompleted()
- {
- var lifetime = ApplicationLifetime as IClassicDesktopStyleApplicationLifetime;
- if (lifetime != null)
- lifetime.MainWindow = new ControlsListView();
-
- base.OnFrameworkInitializationCompleted();
- }
}
}
\ No newline at end of file
diff --git a/src/Consolonia.Gallery/Consolonia.Gallery.csproj b/src/Consolonia.Gallery/Consolonia.Gallery.csproj
index e3f51ac4..3bb77c69 100644
--- a/src/Consolonia.Gallery/Consolonia.Gallery.csproj
+++ b/src/Consolonia.Gallery/Consolonia.Gallery.csproj
@@ -10,10 +10,7 @@
-
-
-
-
+
diff --git a/src/Consolonia.Gallery/Gallery/GalleryViews/GalleryButton.axaml b/src/Consolonia.Gallery/Gallery/GalleryViews/GalleryButton.axaml
index 4002c36e..b0b8f61f 100644
--- a/src/Consolonia.Gallery/Gallery/GalleryViews/GalleryButton.axaml
+++ b/src/Consolonia.Gallery/Gallery/GalleryViews/GalleryButton.axaml
@@ -14,8 +14,7 @@
-
-
+
+
+
+