-
-
Notifications
You must be signed in to change notification settings - Fork 322
/
.yarn-metadata.json
45 lines (45 loc) · 10.7 KB
/
.yarn-metadata.json
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
{
"manifest": {
"name": "react-native-baidu-map",
"version": "1.0.2",
"description": "Baidu Map SDK modules and view for React Native(Android & IOS), support react native 0.57+. 百度地图 React Native 模块,支持 react native 0.57+,已更新到最新的百度地图SDK版本。",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"repository": {
"type": "git",
"url": "git+https://github.com/lovebing/react-native-baidu-map.git"
},
"keywords": [
"Baidu Map",
"React Native"
],
"author": {
"name": "lovebing"
},
"license": "MIT",
"bugs": {
"url": "https://github.com/lovebing/react-native-baidu-map/issues"
},
"homepage": "https://github.com/lovebing/react-native-baidu-map#readme",
"devDependencies": {
"react": "16.6.1",
"react-native": "^0.57.5"
},
"_registry": "npm",
"_loc": "/Users/lovebing/Library/Caches/Yarn/v1/npm-react-native-baidu-map-1.0.2-fa4f71f3-d352-42af-8c90-47f3abc5dacb-1549651247170/package.json",
"readmeFilename": "README.md",
"readme": "# react-native-baidu-map [](https://www.npmjs.com/package/react-native-baidu-map)\n\nBaidu Map SDK modules and view for React Native(Android & IOS), support react native 0.57+\n\n百度地图 React Native 模块,支持 react native 0.57+,已更新到最新的百度地图SDK版本。\n\nMarker icon 的实现参考了 https://github.com/react-native-community/react-native-maps 的相关代码。\n\n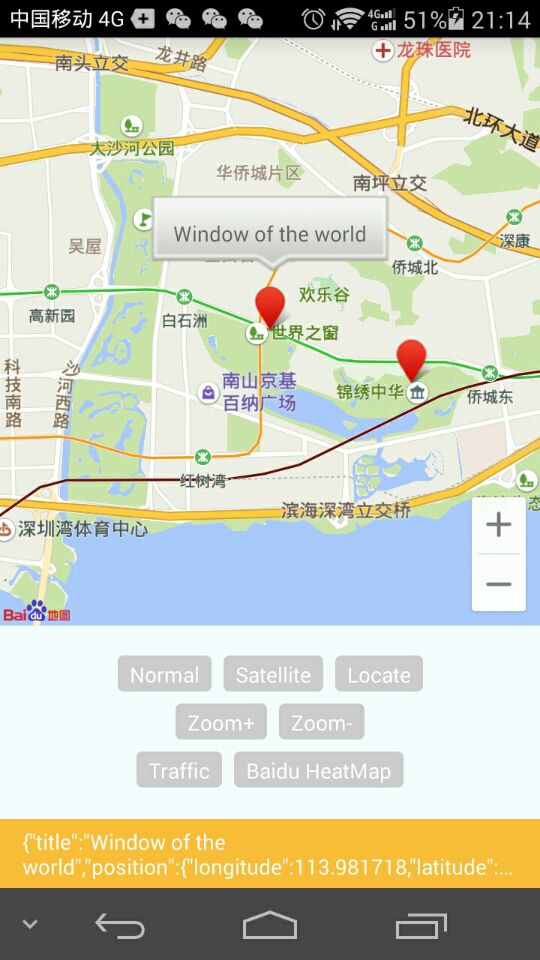\n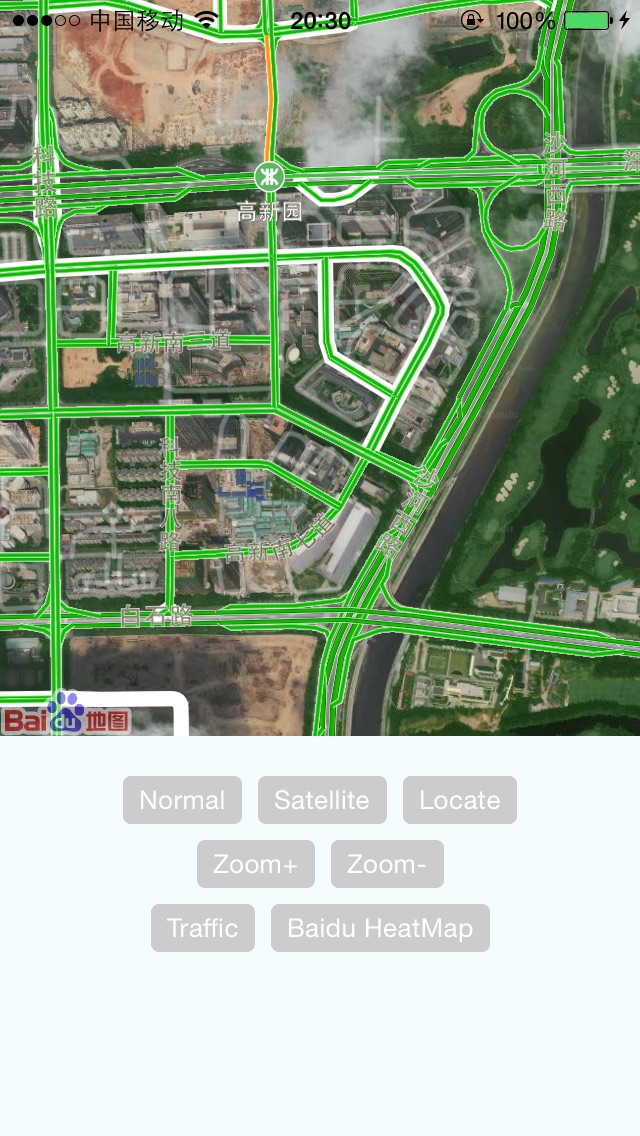\n### 环境要求\n1.JS\n- node: 8.0 及以上\n\n2.Android\n- Android SDK: api 28 及上以上\n- gradle: 4.6\n- Android Studio: 3.1.3 及以上\n\n3.IOS\n- XCode: 8.0 及以上\n\n### JS模块安装\n npm install react-native-baidu-map --save\n### 原生模块导入\n\n#### Android Studio\n\n1.导入\n\n方法一:\n`react-native link react-native-baidu-map`\n\n方法二:使用 mavenCentral,编辑 app/build.gradle,添加依赖\n```groovy\nrepositories {\n mavenCentral()\n}\ndependencies {\n implementation 'org.lovebing.reactnative:baidu-map:1.0.1'\n}\n```\n方法三:使用本地 aar (打包后生成)\n- 在 app/libs 目录下添加 baidu-map-release.aar\n- 编辑 app/build.gradle,添加依赖\n```groovy\nandroid {\n repositories {\n flatDir {\n dirs 'libs'\n }\n }\n}\ndependencies {\n implementation fileTree(dir: \"libs\", include: [\"*.jar\", \"*.aar\"])\n}\n```\n2.编辑 MainApplication.java,添加 BaiduMapPackage\n\n```java\npublic class MainApplication extends Application implements ReactApplication {\n @Override\n protected List<ReactPackage> getPackages() {\n return Arrays.<ReactPackage>asList(\n new MainReactPackage(), new BaiduMapPackage()\n );\n }\n}\n```\n\n#### IOS/Xcode (重构中)\n- Project navigator->Libraries->Add Files to 选择 react-native-baidu-map/ios/RCTBaiduMap.xcodeproj\n- Project navigator->Build Phases->Link Binary With Libraries 加入 libRCTBaiduMap.a\n- Project navigator->Build Settings->Search Paths, Framework search paths 添加 react-native-baidu-map/ios/lib,Header search paths 添加 react-native-baidu-map/ios/RCTBaiduMap\n- 添加依赖, react-native-baidu-map/ios/lib 下的全部 framwordk, CoreLocation.framework和QuartzCore.framework、OpenGLES.framework、SystemConfiguration.framework、CoreGraphics.framework、Security.framework、libsqlite3.0.tbd(xcode7以前为 libsqlite3.0.dylib)、CoreTelephony.framework 、libstdc++.6.0.9.tbd(xcode7以前为libstdc++.6.0.9.dylib)\n- 添加 BaiduMapAPI_Map.framework/Resources/mapapi.bundle\n\n- 其它一些注意事项可参考百度地图LBS文档\n\n##### AppDelegate.m init 初始化\n #import \"RCTBaiduMapViewManager.h\"\n - (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions\n {\n ...\n [RCTBaiduMapViewManager initSDK:@\"api key\"];\n ...\n }\n\n### Usage 使用方法\n\n import { MapView, MapTypes, Geolocation, Overelay } from 'react-native-baidu-map\n\n#### MapView Props 属性\n| Prop | Type | Default | Description\n| ----------------------- |:-----:| :-------:| -------\n| zoomControlsVisible | bool | true | Android only\n| trafficEnabled | bool | false |\n| baiduHeatMapEnabled | bool | false |\n| mapType | number| 1 |\n| zoom | number| 10 |\n| center | object| null | {latitude: 0, longitude: 0}\n| onMapStatusChangeStart | func | undefined| Android only\n| onMapStatusChange | func | undefined|\n| onMapStatusChangeFinish | func | undefined| Android only\n| onMapLoaded | func | undefined|\n| onMapClick | func | undefined|\n| onMapDoubleClick | func | undefined|\n| onMarkerClick | func | undefined|\n| onMapPoiClick | func | undefined|\n\n#### Overelay 覆盖物\n const { Marker, Arc, Circle, Polyline, Polygon, Text, InfoWindow } = Overelay;\n\n##### Marker Props 属性\n| Prop | Type | Default | Description\n| ----------------------- |:-----:| :-------:| -------\n| title | string| null |\n| location | object| {latitude: 0, longitude: 0} |\n| perspective | bool | null |\n| flat | bool | null |\n| rotate | float | 0 |\n| icon | any | null | icon图片,同 <Image> 的 source 属性\n| alpha | float | 1 |\n\n##### Arc Props 属性\n| Prop | Type | Default | Description\n| ----------------------- |:-----:| :-------:| -------\n| color | string| AA00FF00 |\n| width | int | 4 |\n| poins | array | [{latitude: 0, longitude: 0}, {latitude: 0, longitude: 0}, {latitude: 0, longitude: 0}] | 数值长度必须为 3\n\n##### Circle Props 属性\n| Prop | Type | Default | Description\n| ----------------------- |:-----:| :-------:| -------\n| radius | int | 1400 |\n| fillColor | string| 000000FF |\n| stroke | object| {width: 5, color: 'AA000000'} |\n| center | object| {latitude: 0, longitude: 0} |\n\n##### Polyline Props 属性\n| Prop | Type | Default | Description\n| ----------------------- |:-----:| :-------:| -------\n| points | array | [{latitude: 0, longitude: 0}] |\n| color | string| AAFF0000 |\n\n##### Polygon Props 属性\n| Prop | Type | Default | Description\n| ----------------------- |:-----:| :-------:| -------\n| points | array | [{latitude: 0, longitude: 0}] |\n| fillColor | string| AAFFFF00 |\n| stroke | object| {width: 5, color: 'AA00FF00'} |\n\n\n##### Text Props 属性\n| Prop | Type | Default | Description\n| ----------------------- |:-----:| :-------:| -------\n| text | string| |\n| fontSize | int | |\n| fontColor | string| |\n| bgColor | string| |\n| rotate | float | |\n| location | object|{latitude: 0, longitude: 0}\n\n##### InfoWindow Props 属性\n| Prop | Type | Default | Description\n| ----------------------- |:-----:| :-------:| -------\n| location | object|{latitude: 0, longitude: 0}\n| visible | bool | false | 点击 marker 后才能设为 true \n\n<MapView>\n <Marker/>\n <Arc />\n <Circle />\n <Polyline />\n <Polygon />\n <Text />\n <InfoWindow>\n <View></View>\n </InfoWindow>\n</MapView>\n\n#### Geolocation Methods\n\n| Method | Result\n| ------------------------- | -------\n| Promise reverseGeoCode(double lat, double lng) | `{\"address\": \"\", \"province\": \"\", \"cityCode\": \"\", \"city\": \"\", \"district\": \"\", \"streetName\": \"\", \"streetNumber\": \"\"}`\n| Promise reverseGeoCodeGPS(double lat, double lng) | `{\"address\": \"\", \"province\": \"\", \"cityCode\": \"\", \"city\": \"\", \"district\": \"\", \"streetName\": \"\", \"streetNumber\": \"\"}`\n| Promise geocode(String city, String addr) | {\"latitude\": 0.0, \"longitude\": 0.0}\n| Promise getCurrentPosition() | IOS: `{\"latitude\": 0.0, \"longitude\": 0.0, \"address\": \"\", \"province\": \"\", \"cityCode\": \"\", \"city\": \"\", \"district\": \"\", \"streetName\": \"\", \"streetNumber\": \"\"}` Android: `{\"latitude\": 0.0, \"longitude\": 0.0, \"direction\": -1, \"altitude\": 0.0, \"radius\": 0.0, \"address\": \"\", \"countryCode\": \"\", \"country\": \"\", \"province\": \"\", \"cityCode\": \"\", \"city\": \"\", \"district\": \"\", \"street\": \"\", \"streetNumber\": \"\", \"buildingId\": \"\", \"buildingName\": \"\"}`\n\n\n\n### 开发和测试说明\n根目录执行 `npm link`\n`cd example`\n`npm link react-native-baidu-map`\n",
"licenseText": "MIT License\n\nCopyright (c) 2016-present, lovebing.org.\n\nPermission is hereby granted, free of charge, to any person obtaining a copy\nof this software and associated documentation files (the \"Software\"), to deal\nin the Software without restriction, including without limitation the rights\nto use, copy, modify, merge, publish, distribute, sublicense, and/or sell\ncopies of the Software, and to permit persons to whom the Software is\nfurnished to do so, subject to the following conditions:\n\nThe above copyright notice and this permission notice shall be included in all\ncopies or substantial portions of the Software.\n\nTHE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR\nIMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,\nFITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE\nAUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER\nLIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,\nOUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE\nSOFTWARE."
},
"artifacts": [],
"remote": {
"type": "copy",
"registry": "npm",
"hash": "fa4f71f3-d352-42af-8c90-47f3abc5dacb-1549651247170",
"reference": "/usr/local/node/lib/node_modules/react-native-baidu-map"
},
"registry": "npm",
"hash": "fa4f71f3-d352-42af-8c90-47f3abc5dacb-1549651247170"
}