diff --git a/README.md b/README.md
index 5ba9680..2a755cd 100644
--- a/README.md
+++ b/README.md
@@ -20,7 +20,7 @@
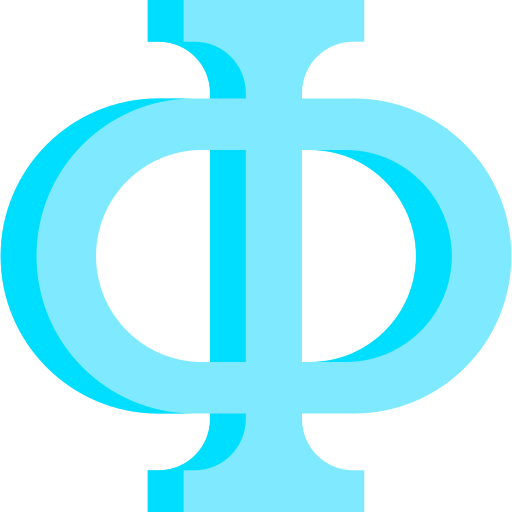
-LightPHE is a lightweight partially homomorphic encryption library for python. It is a hybrid homomoprhic encryption library wrapping many schemes such as [`RSA`](https://sefiks.com/2023/03/06/a-step-by-step-partially-homomorphic-encryption-example-with-rsa-in-python/), [`ElGamal`](https://sefiks.com/2023/03/27/a-step-by-step-partially-homomorphic-encryption-example-with-elgamal-in-python/), [`Exponential ElGamal`](https://sefiks.com/2023/03/27/a-step-by-step-partially-homomorphic-encryption-example-with-elgamal-in-python/), [`Elliptic Curve ElGamal`](https://sefiks.com/2018/08/21/elliptic-curve-elgamal-encryption/), [`Paillier`](https://sefiks.com/2023/04/03/a-step-by-step-partially-homomorphic-encryption-example-with-paillier-in-python/), [`Damgard-Jurik`](https://sefiks.com/2023/10/20/a-step-by-step-partially-homomorphic-encryption-example-with-damgard-jurik-in-python/), [`Okamoto–Uchiyama`](https://sefiks.com/2023/10/20/a-step-by-step-partially-homomorphic-encryption-example-with-okamoto-uchiyama-in-python/), [`Benaloh`](https://sefiks.com/2023/10/06/a-step-by-step-partially-homomorphic-encryption-example-with-benaloh-in-python-from-scratch/), [`Naccache–Stern`](https://sefiks.com/2023/10/26/a-step-by-step-partially-homomorphic-encryption-example-with-naccache-stern-in-python/), [`Goldwasser–Micali`](https://sefiks.com/2023/10/27/a-step-by-step-partially-homomorphic-encryption-example-with-goldwasser-micali-in-python/).
+LightPHE is a lightweight partially homomorphic encryption library for python. It is a hybrid homomoprhic encryption library wrapping many schemes such as [`RSA`](https://sefiks.com/2023/03/06/a-step-by-step-partially-homomorphic-encryption-example-with-rsa-in-python/), [`ElGamal`](https://sefiks.com/2023/03/27/a-step-by-step-partially-homomorphic-encryption-example-with-elgamal-in-python/), [`Exponential ElGamal`](https://sefiks.com/2023/03/27/a-step-by-step-partially-homomorphic-encryption-example-with-elgamal-in-python/), [`Elliptic Curve ElGamal`](https://sefiks.com/2018/08/21/elliptic-curve-elgamal-encryption/) ([`Weierstrass`](https://sefiks.com/2016/03/13/the-math-behind-elliptic-curve-cryptography/), [`Koblitz`](https://sefiks.com/2016/03/13/the-math-behind-elliptic-curves-over-binary-field/) and [`Edwards`](https://sefiks.com/2018/12/19/a-gentle-introduction-to-edwards-curves/) forms) [`Paillier`](https://sefiks.com/2023/04/03/a-step-by-step-partially-homomorphic-encryption-example-with-paillier-in-python/), [`Damgard-Jurik`](https://sefiks.com/2023/10/20/a-step-by-step-partially-homomorphic-encryption-example-with-damgard-jurik-in-python/), [`Okamoto–Uchiyama`](https://sefiks.com/2023/10/20/a-step-by-step-partially-homomorphic-encryption-example-with-okamoto-uchiyama-in-python/), [`Benaloh`](https://sefiks.com/2023/10/06/a-step-by-step-partially-homomorphic-encryption-example-with-benaloh-in-python-from-scratch/), [`Naccache–Stern`](https://sefiks.com/2023/10/26/a-step-by-step-partially-homomorphic-encryption-example-with-naccache-stern-in-python/), [`Goldwasser–Micali`](https://sefiks.com/2023/10/27/a-step-by-step-partially-homomorphic-encryption-example-with-goldwasser-micali-in-python/).
# Partially vs Fully Homomorphic Encryption
diff --git a/lightphe/__init__.py b/lightphe/__init__.py
index 7b628f9..2351947 100644
--- a/lightphe/__init__.py
+++ b/lightphe/__init__.py
@@ -43,7 +43,7 @@ def __init__(
key_size (int): key size in bits
precision (int): precision for homomorphic operations on tensors
form (str): specifies the form of the elliptic curve.
- Options: 'weierstrass' (default), 'edwards', 'twisted-edwards', 'koblitz'.
+ Options: 'weierstrass' (default), 'edwards', 'koblitz'.
This parameter is only used if `algorithm_name` is 'EllipticCurve-ElGamal'.
"""
self.algorithm_name = algorithm_name
@@ -83,7 +83,7 @@ def __build_cryptosystem(
key_file (str): if keys are exported, you can load them into cryptosystem
key_size (int): key size in bits
form (str): specifies the form of the elliptic curve.
- Options: 'weierstrass' (default), 'edwards', 'twisted-edwards', 'koblitz'.
+ Options: 'weierstrass' (default), 'edwards', 'koblitz'.
This parameter is only used if `algorithm_name` is 'EllipticCurve-ElGamal'.
Returns
cryptosystem
diff --git a/lightphe/cryptosystems/EllipticCurveElGamal.py b/lightphe/cryptosystems/EllipticCurveElGamal.py
index 41e4324..cdd6d12 100644
--- a/lightphe/cryptosystems/EllipticCurveElGamal.py
+++ b/lightphe/cryptosystems/EllipticCurveElGamal.py
@@ -25,11 +25,11 @@ def __init__(self, keys: Optional[dict] = None, key_size: Optional[int] = None,
key_size (int): key size in bits. default is 160.
this is equivalent to 1024 bit RSA.
form (str): specifies the elliptic curve form.
- Options are 'weierstrass' (default), 'koblitz', 'edwards', 'twisted-edwards'.
+ Options are 'weierstrass' (default), 'koblitz', 'edwards'.
"""
if form is None or form == "weierstrass":
self.curve = Weierstrass()
- elif form == "twisted-edwards":
+ elif form in "edwards":
self.curve = TwistedEdwards()
else:
raise ValueError(f"unimplemented curve form - {form}")
diff --git a/lightphe/elliptic_curve_forms/edwards.py b/lightphe/elliptic_curve_forms/edwards.py
index 4870f77..19af15c 100644
--- a/lightphe/elliptic_curve_forms/edwards.py
+++ b/lightphe/elliptic_curve_forms/edwards.py
@@ -4,8 +4,10 @@
class TwistedEdwards(EllipticCurve):
"""
- Builds twisted edwards curves
- Ref: https://sefiks.com/2018/12/26/twisted-edwards-curves/
+ Builds (twisted) edwards curves
+ Refs:
+ [1] https://sefiks.com/2018/12/19/a-gentle-introduction-to-edwards-curves/
+ [2] https://sefiks.com/2018/12/26/twisted-edwards-curves/
"""
def __init__(self, curve="ed25519"):
diff --git a/tests/test_ellipticcurveelgamal.py b/tests/test_ellipticcurveelgamal.py
index 4844928..798adc9 100644
--- a/tests/test_ellipticcurveelgamal.py
+++ b/tests/test_ellipticcurveelgamal.py
@@ -4,7 +4,7 @@
logger = Logger(module="tests/test_ellipticcurveelgamal.py")
-FORMS = ["weierstrass", "twisted-edwards"]
+FORMS = ["weierstrass", "edwards"]
def test_elliptic_curve_elgamal():