diff --git a/examples/for-tests/src/App.js b/examples/for-tests/src/App.js
index 7bdb1092c..2d668989c 100644
--- a/examples/for-tests/src/App.js
+++ b/examples/for-tests/src/App.js
@@ -443,6 +443,7 @@ if (testContext.enableMFA) {
SuperTokens.init({
usesDynamicLoginMethods: testContext.usesDynamicLoginMethods,
clientType: testContext.clientType,
+ enableDebugLogs: true,
appInfo: {
appName: "SuperTokens",
websiteDomain: getWebsiteDomain(),
diff --git a/examples/st-oauth2-authorization-server/README.md b/examples/st-oauth2-authorization-server/README.md
new file mode 100644
index 000000000..85f92edfd
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/README.md
@@ -0,0 +1,43 @@
+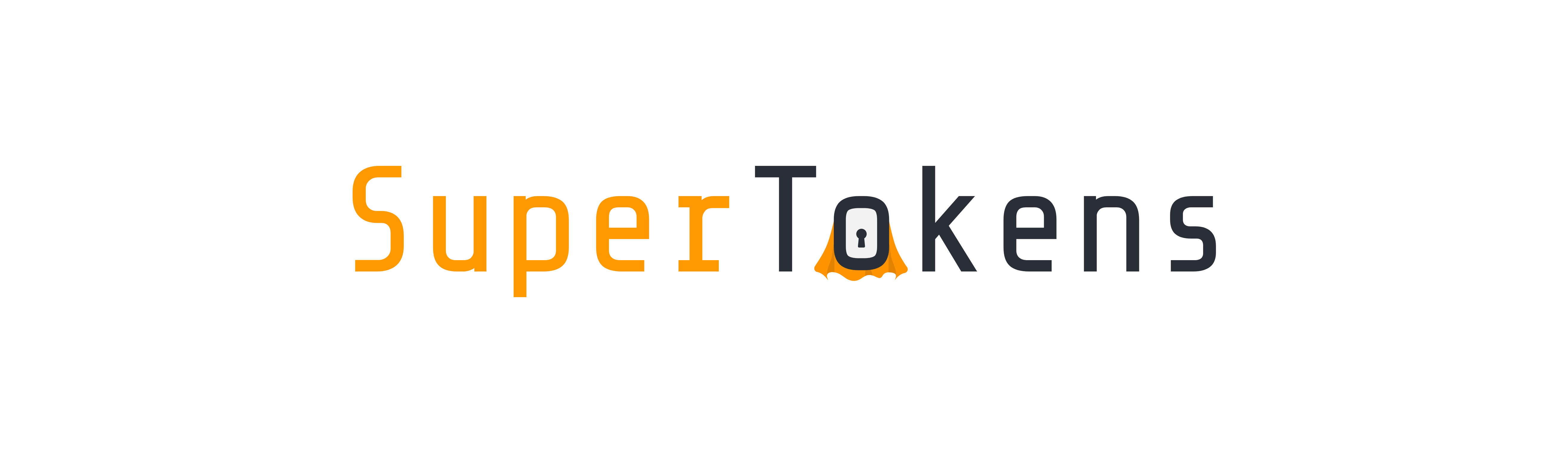
+
+# SuperTokens OAuth2 Authorization Server
+
+This example app uses SuperTokens `OAuth2Provider` recipe to expose OAuth2 APIs. This app acts as an OAuth2 authorization server for other OAuth2 examples in this repo.
+
+## Project setup
+
+Clone the repo, enter the directory, and use `npm` to install the project dependencies:
+
+```bash
+git clone https://github.com/supertokens/supertokens-auth-react
+cd supertokens-auth-react/examples/st-oauth2-authorization-server
+npm install
+```
+
+## Set Up Frontend and Backend URLs
+
+When running locally, we recommend using a different domain than `localhost` for the authorization server to prevent cookie sharing with other client apps running on `localhost`.
+
+By default, the frontend runs at `http://localhost.com:3005` and the backend at `http://localhost.com:3006`. You can customize these by setting the `REACT_APP_AUTH_SERVER_WEBSITE_URL` and `REACT_APP_AUTH_SERVER_API_URL` environment variables.
+
+After configuring the URLs, add the domain to `/etc/hosts`. For example, if your domain is `localhost.com`, add:
+
+```bash
+127.0.0.1 localhost.com
+```
+
+## Run the demo app
+
+This compiles and serves the React app and starts the backend API server.
+
+```bash
+npm run start
+```
+
+## Author
+
+Created with :heart: by the folks at supertokens.com.
+
+## License
+
+This project is licensed under the Apache 2.0 license.
diff --git a/examples/st-oauth2-authorization-server/backend/config.ts b/examples/st-oauth2-authorization-server/backend/config.ts
new file mode 100644
index 000000000..2e341ec42
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/backend/config.ts
@@ -0,0 +1,27 @@
+import EmailPassword from "supertokens-node/recipe/emailpassword";
+import OAuth2Provider from "supertokens-node/recipe/oauth2provider";
+import Session from "supertokens-node/recipe/session";
+import { TypeInput } from "supertokens-node/types";
+
+export function getWebsiteDomain() {
+ return process.env.REACT_APP_AUTH_SERVER_WEBSITE_URL || "http://localhost.com:3005";
+}
+
+export function getApiDomain() {
+ return process.env.REACT_APP_AUTH_SERVER_API_URL || "http://localhost.com:3006";
+}
+
+export const SuperTokensConfig: TypeInput = {
+ supertokens: {
+ // this is the location of the SuperTokens core.
+ connectionURI: "https://try.supertokens.com",
+ },
+ appInfo: {
+ appName: "SuperTokens Demo App",
+ apiDomain: getApiDomain(),
+ websiteDomain: getWebsiteDomain(),
+ },
+ // recipeList contains all the modules that you want to
+ // use from SuperTokens. See the full list here: https://supertokens.com/docs/guides
+ recipeList: [EmailPassword.init(), OAuth2Provider.init(), Session.init()],
+};
diff --git a/examples/st-oauth2-authorization-server/backend/index.ts b/examples/st-oauth2-authorization-server/backend/index.ts
new file mode 100644
index 000000000..a43b57bd4
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/backend/index.ts
@@ -0,0 +1,49 @@
+import express from "express";
+import cors from "cors";
+import supertokens from "supertokens-node";
+import { verifySession } from "supertokens-node/recipe/session/framework/express";
+import { middleware, errorHandler, SessionRequest } from "supertokens-node/framework/express";
+import { getApiDomain, getWebsiteDomain, SuperTokensConfig } from "./config";
+import Multitenancy from "supertokens-node/recipe/multitenancy";
+
+supertokens.init(SuperTokensConfig);
+
+const app = express();
+
+app.use(
+ cors({
+ origin: [getWebsiteDomain(), "http://localhost:3000"],
+ // origin: [getWebsiteDomain()],
+ allowedHeaders: ["content-type", ...supertokens.getAllCORSHeaders()],
+ methods: ["GET", "PUT", "POST", "DELETE"],
+ credentials: true,
+ })
+);
+
+// This exposes all the APIs from SuperTokens to the client.
+app.use(middleware());
+
+// An example API that requires session verification
+app.get("/sessioninfo", verifySession(), async (req: SessionRequest, res) => {
+ let session = req.session;
+ res.send({
+ sessionHandle: session!.getHandle(),
+ userId: session!.getUserId(),
+ accessTokenPayload: session!.getAccessTokenPayload(),
+ });
+});
+
+// This API is used by the frontend to create the tenants drop down when the app loads.
+// Depending on your UX, you can remove this API.
+app.get("/tenants", async (req, res) => {
+ let tenants = await Multitenancy.listAllTenants();
+ res.send(tenants);
+});
+
+// In case of session related errors, this error handler
+// returns 401 to the client.
+app.use(errorHandler());
+
+const PORT = process.env.PORT || 3006;
+
+app.listen(PORT, () => console.log(`API Server listening on ${getApiDomain()}`));
diff --git a/examples/st-oauth2-authorization-server/backend/package.json b/examples/st-oauth2-authorization-server/backend/package.json
new file mode 100644
index 000000000..67f613481
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/backend/package.json
@@ -0,0 +1,15 @@
+{
+ "name": "supertokens-node",
+ "version": "0.0.1",
+ "private": true,
+ "description": "",
+ "main": "index.js",
+ "scripts": {
+ "start": "PORT=3006 npx ts-node-dev --project ./tsconfig.json ./index.ts"
+ },
+ "dependencies": {},
+ "devDependencies": {},
+ "keywords": [],
+ "author": "",
+ "license": "ISC"
+}
diff --git a/examples/st-oauth2-authorization-server/backend/tsconfig.json b/examples/st-oauth2-authorization-server/backend/tsconfig.json
new file mode 100644
index 000000000..8a91acaae
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/backend/tsconfig.json
@@ -0,0 +1,62 @@
+{
+ "compilerOptions": {
+ /* Visit https://aka.ms/tsconfig.json to read more about this file */
+ /* Basic Options */
+ // "incremental": true, /* Enable incremental compilation */
+ "target": "es5" /* Specify ECMAScript target version: 'ES3' (default), 'ES5', 'ES2015', 'ES2016', 'ES2017', 'ES2018', 'ES2019', 'ES2020', or 'ESNEXT'. */,
+ "module": "commonjs" /* Specify module code generation: 'none', 'commonjs', 'amd', 'system', 'umd', 'es2015', 'es2020', or 'ESNext'. */,
+ // "lib": [], /* Specify library files to be included in the compilation. */
+ // "allowJs": true, /* Allow javascript files to be compiled. */
+ // "checkJs": true, /* Report errors in .js files. */
+ // "jsx": "preserve", /* Specify JSX code generation: 'preserve', 'react-native', or 'react'. */
+ // "declaration": true, /* Generates corresponding '.d.ts' file. */
+ // "declarationMap": true, /* Generates a sourcemap for each corresponding '.d.ts' file. */
+ // "sourceMap": true, /* Generates corresponding '.map' file. */
+ // "outFile": "./", /* Concatenate and emit output to single file. */
+ // "outDir": "./", /* Redirect output structure to the directory. */
+ // "rootDir": "./", /* Specify the root directory of input files. Use to control the output directory structure with --outDir. */
+ // "composite": true, /* Enable project compilation */
+ // "tsBuildInfoFile": "./", /* Specify file to store incremental compilation information */
+ // "removeComments": true, /* Do not emit comments to output. */
+ // "noEmit": true, /* Do not emit outputs. */
+ // "importHelpers": true, /* Import emit helpers from 'tslib'. */
+ // "downlevelIteration": true, /* Provide full support for iterables in 'for-of', spread, and destructuring when targeting 'ES5' or 'ES3'. */
+ // "isolatedModules": true, /* Transpile each file as a separate module (similar to 'ts.transpileModule'). */
+ /* Strict Type-Checking Options */
+ "strict": true /* Enable all strict type-checking options. */,
+ // "noImplicitAny": true, /* Raise error on expressions and declarations with an implied 'any' type. */
+ // "strictNullChecks": true, /* Enable strict null checks. */
+ // "strictFunctionTypes": true, /* Enable strict checking of function types. */
+ // "strictBindCallApply": true, /* Enable strict 'bind', 'call', and 'apply' methods on functions. */
+ // "strictPropertyInitialization": true, /* Enable strict checking of property initialization in classes. */
+ // "noImplicitThis": true, /* Raise error on 'this' expressions with an implied 'any' type. */
+ // "alwaysStrict": true, /* Parse in strict mode and emit "use strict" for each source file. */
+ /* Additional Checks */
+ // "noUnusedLocals": true, /* Report errors on unused locals. */
+ // "noUnusedParameters": true, /* Report errors on unused parameters. */
+ // "noImplicitReturns": true, /* Report error when not all code paths in function return a value. */
+ // "noFallthroughCasesInSwitch": true, /* Report errors for fallthrough cases in switch statement. */
+ /* Module Resolution Options */
+ // "moduleResolution": "node", /* Specify module resolution strategy: 'node' (Node.js) or 'classic' (TypeScript pre-1.6). */
+ // "baseUrl": "./", /* Base directory to resolve non-absolute module names. */
+ // "paths": {}, /* A series of entries which re-map imports to lookup locations relative to the 'baseUrl'. */
+ // "rootDirs": [], /* List of root folders whose combined content represents the structure of the project at runtime. */
+ // "typeRoots": [], /* List of folders to include type definitions from. */
+ // "types": [], /* Type declaration files to be included in compilation. */
+ // "allowSyntheticDefaultImports": true, /* Allow default imports from modules with no default export. This does not affect code emit, just typechecking. */
+ "esModuleInterop": true /* Enables emit interoperability between CommonJS and ES Modules via creation of namespace objects for all imports. Implies 'allowSyntheticDefaultImports'. */,
+ // "preserveSymlinks": true, /* Do not resolve the real path of symlinks. */
+ // "allowUmdGlobalAccess": true, /* Allow accessing UMD globals from modules. */
+ /* Source Map Options */
+ // "sourceRoot": "", /* Specify the location where debugger should locate TypeScript files instead of source locations. */
+ // "mapRoot": "", /* Specify the location where debugger should locate map files instead of generated locations. */
+ // "inlineSourceMap": true, /* Emit a single file with source maps instead of having a separate file. */
+ // "inlineSources": true, /* Emit the source alongside the sourcemaps within a single file; requires '--inlineSourceMap' or '--sourceMap' to be set. */
+ /* Experimental Options */
+ // "experimentalDecorators": true, /* Enables experimental support for ES7 decorators. */
+ // "emitDecoratorMetadata": true, /* Enables experimental support for emitting type metadata for decorators. */
+ /* Advanced Options */
+ "skipLibCheck": true /* Skip type checking of declaration files. */,
+ "forceConsistentCasingInFileNames": true /* Disallow inconsistently-cased references to the same file. */
+ }
+}
diff --git a/examples/st-oauth2-authorization-server/frontend/.env b/examples/st-oauth2-authorization-server/frontend/.env
new file mode 100644
index 000000000..7d910f148
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/.env
@@ -0,0 +1 @@
+SKIP_PREFLIGHT_CHECK=true
\ No newline at end of file
diff --git a/examples/st-oauth2-authorization-server/frontend/.gitignore b/examples/st-oauth2-authorization-server/frontend/.gitignore
new file mode 100644
index 000000000..4d29575de
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/.gitignore
@@ -0,0 +1,23 @@
+# See https://help.github.com/articles/ignoring-files/ for more about ignoring files.
+
+# dependencies
+/node_modules
+/.pnp
+.pnp.js
+
+# testing
+/coverage
+
+# production
+/build
+
+# misc
+.DS_Store
+.env.local
+.env.development.local
+.env.test.local
+.env.production.local
+
+npm-debug.log*
+yarn-debug.log*
+yarn-error.log*
diff --git a/examples/st-oauth2-authorization-server/frontend/LICENSE.md b/examples/st-oauth2-authorization-server/frontend/LICENSE.md
new file mode 100644
index 000000000..588f27e68
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/LICENSE.md
@@ -0,0 +1,192 @@
+Copyright (c) 2020, VRAI Labs and/or its affiliates. All rights reserved.
+
+This software is licensed under the Apache License, Version 2.0 (the
+"License") as published by the Apache Software Foundation.
+
+You may not use this software except in compliance with the License. A copy
+of the License is available below the line.
+
+Unless required by applicable law or agreed to in writing, software
+distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+License for the specific language governing permissions and limitations
+under the License.
+
+---
+
+ Apache License
+ Version 2.0, January 2004
+ http://www.apache.org/licenses/
+
+TERMS AND CONDITIONS FOR USE, REPRODUCTION, AND DISTRIBUTION
+
+1. Definitions.
+
+ "License" shall mean the terms and conditions for use, reproduction,
+ and distribution as defined by Sections 1 through 9 of this document.
+
+ "Licensor" shall mean the copyright owner or entity authorized by
+ the copyright owner that is granting the License.
+
+ "Legal Entity" shall mean the union of the acting entity and all
+ other entities that control, are controlled by, or are under common
+ control with that entity. For the purposes of this definition,
+ "control" means (i) the power, direct or indirect, to cause the
+ direction or management of such entity, whether by contract or
+ otherwise, or (ii) ownership of fifty percent (50%) or more of the
+ outstanding shares, or (iii) beneficial ownership of such entity.
+
+ "You" (or "Your") shall mean an individual or Legal Entity
+ exercising permissions granted by this License.
+
+ "Source" form shall mean the preferred form for making modifications,
+ including but not limited to software source code, documentation
+ source, and configuration files.
+
+ "Object" form shall mean any form resulting from mechanical
+ transformation or translation of a Source form, including but
+ not limited to compiled object code, generated documentation,
+ and conversions to other media types.
+
+ "Work" shall mean the work of authorship, whether in Source or
+ Object form, made available under the License, as indicated by a
+ copyright notice that is included in or attached to the work
+ (an example is provided in the Appendix below).
+
+ "Derivative Works" shall mean any work, whether in Source or Object
+ form, that is based on (or derived from) the Work and for which the
+ editorial revisions, annotations, elaborations, or other modifications
+ represent, as a whole, an original work of authorship. For the purposes
+ of this License, Derivative Works shall not include works that remain
+ separable from, or merely link (or bind by name) to the interfaces of,
+ the Work and Derivative Works thereof.
+
+ "Contribution" shall mean any work of authorship, including
+ the original version of the Work and any modifications or additions
+ to that Work or Derivative Works thereof, that is intentionally
+ submitted to Licensor for inclusion in the Work by the copyright owner
+ or by an individual or Legal Entity authorized to submit on behalf of
+ the copyright owner. For the purposes of this definition, "submitted"
+ means any form of electronic, verbal, or written communication sent
+ to the Licensor or its representatives, including but not limited to
+ communication on electronic mailing lists, source code control systems,
+ and issue tracking systems that are managed by, or on behalf of, the
+ Licensor for the purpose of discussing and improving the Work, but
+ excluding communication that is conspicuously marked or otherwise
+ designated in writing by the copyright owner as "Not a Contribution."
+
+ "Contributor" shall mean Licensor and any individual or Legal Entity
+ on behalf of whom a Contribution has been received by Licensor and
+ subsequently incorporated within the Work.
+
+2. Grant of Copyright License. Subject to the terms and conditions of
+ this License, each Contributor hereby grants to You a perpetual,
+ worldwide, non-exclusive, no-charge, royalty-free, irrevocable
+ copyright license to reproduce, prepare Derivative Works of,
+ publicly display, publicly perform, sublicense, and distribute the
+ Work and such Derivative Works in Source or Object form.
+
+3. Grant of Patent License. Subject to the terms and conditions of
+ this License, each Contributor hereby grants to You a perpetual,
+ worldwide, non-exclusive, no-charge, royalty-free, irrevocable
+ (except as stated in this section) patent license to make, have made,
+ use, offer to sell, sell, import, and otherwise transfer the Work,
+ where such license applies only to those patent claims licensable
+ by such Contributor that are necessarily infringed by their
+ Contribution(s) alone or by combination of their Contribution(s)
+ with the Work to which such Contribution(s) was submitted. If You
+ institute patent litigation against any entity (including a
+ cross-claim or counterclaim in a lawsuit) alleging that the Work
+ or a Contribution incorporated within the Work constitutes direct
+ or contributory patent infringement, then any patent licenses
+ granted to You under this License for that Work shall terminate
+ as of the date such litigation is filed.
+
+4. Redistribution. You may reproduce and distribute copies of the
+ Work or Derivative Works thereof in any medium, with or without
+ modifications, and in Source or Object form, provided that You
+ meet the following conditions:
+
+ (a) You must give any other recipients of the Work or
+ Derivative Works a copy of this License; and
+
+ (b) You must cause any modified files to carry prominent notices
+ stating that You changed the files; and
+
+ (c) You must retain, in the Source form of any Derivative Works
+ that You distribute, all copyright, patent, trademark, and
+ attribution notices from the Source form of the Work,
+ excluding those notices that do not pertain to any part of
+ the Derivative Works; and
+
+ (d) If the Work includes a "NOTICE" text file as part of its
+ distribution, then any Derivative Works that You distribute must
+ include a readable copy of the attribution notices contained
+ within such NOTICE file, excluding those notices that do not
+ pertain to any part of the Derivative Works, in at least one
+ of the following places: within a NOTICE text file distributed
+ as part of the Derivative Works; within the Source form or
+ documentation, if provided along with the Derivative Works; or,
+ within a display generated by the Derivative Works, if and
+ wherever such third-party notices normally appear. The contents
+ of the NOTICE file are for informational purposes only and
+ do not modify the License. You may add Your own attribution
+ notices within Derivative Works that You distribute, alongside
+ or as an addendum to the NOTICE text from the Work, provided
+ that such additional attribution notices cannot be construed
+ as modifying the License.
+
+ You may add Your own copyright statement to Your modifications and
+ may provide additional or different license terms and conditions
+ for use, reproduction, or distribution of Your modifications, or
+ for any such Derivative Works as a whole, provided Your use,
+ reproduction, and distribution of the Work otherwise complies with
+ the conditions stated in this License.
+
+5. Submission of Contributions. Unless You explicitly state otherwise,
+ any Contribution intentionally submitted for inclusion in the Work
+ by You to the Licensor shall be under the terms and conditions of
+ this License, without any additional terms or conditions.
+ Notwithstanding the above, nothing herein shall supersede or modify
+ the terms of any separate license agreement you may have executed
+ with Licensor regarding such Contributions.
+
+6. Trademarks. This License does not grant permission to use the trade
+ names, trademarks, service marks, or product names of the Licensor,
+ except as required for reasonable and customary use in describing the
+ origin of the Work and reproducing the content of the NOTICE file.
+
+7. Disclaimer of Warranty. Unless required by applicable law or
+ agreed to in writing, Licensor provides the Work (and each
+ Contributor provides its Contributions) on an "AS IS" BASIS,
+ WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
+ implied, including, without limitation, any warranties or conditions
+ of TITLE, NON-INFRINGEMENT, MERCHANTABILITY, or FITNESS FOR A
+ PARTICULAR PURPOSE. You are solely responsible for determining the
+ appropriateness of using or redistributing the Work and assume any
+ risks associated with Your exercise of permissions under this License.
+
+8. Limitation of Liability. In no event and under no legal theory,
+ whether in tort (including negligence), contract, or otherwise,
+ unless required by applicable law (such as deliberate and grossly
+ negligent acts) or agreed to in writing, shall any Contributor be
+ liable to You for damages, including any direct, indirect, special,
+ incidental, or consequential damages of any character arising as a
+ result of this License or out of the use or inability to use the
+ Work (including but not limited to damages for loss of goodwill,
+ work stoppage, computer failure or malfunction, or any and all
+ other commercial damages or losses), even if such Contributor
+ has been advised of the possibility of such damages.
+
+9. Accepting Warranty or Additional Liability. While redistributing
+ the Work or Derivative Works thereof, You may choose to offer,
+ and charge a fee for, acceptance of support, warranty, indemnity,
+ or other liability obligations and/or rights consistent with this
+ License. However, in accepting such obligations, You may act only
+ on Your own behalf and on Your sole responsibility, not on behalf
+ of any other Contributor, and only if You agree to indemnify,
+ defend, and hold each Contributor harmless for any liability
+ incurred by, or claims asserted against, such Contributor by reason
+ of your accepting any such warranty or additional liability.
+
+END OF TERMS AND CONDITIONS
diff --git a/examples/st-oauth2-authorization-server/frontend/package.json b/examples/st-oauth2-authorization-server/frontend/package.json
new file mode 100644
index 000000000..a86573ad4
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/package.json
@@ -0,0 +1,30 @@
+{
+ "name": "supertokens-react",
+ "version": "0.1.0",
+ "private": true,
+ "dependencies": {},
+ "scripts": {
+ "start": "PORT=3005 react-scripts start",
+ "build": "react-scripts build",
+ "test": "react-scripts test",
+ "eject": "react-scripts eject"
+ },
+ "eslintConfig": {
+ "extends": [
+ "react-app",
+ "react-app/jest"
+ ]
+ },
+ "browserslist": {
+ "production": [
+ ">0.2%",
+ "not dead",
+ "not op_mini all"
+ ],
+ "development": [
+ "last 1 chrome version",
+ "last 1 firefox version",
+ "last 1 safari version"
+ ]
+ }
+}
diff --git a/examples/st-oauth2-authorization-server/frontend/public/favicon.ico b/examples/st-oauth2-authorization-server/frontend/public/favicon.ico
new file mode 100644
index 000000000..a11777cc4
Binary files /dev/null and b/examples/st-oauth2-authorization-server/frontend/public/favicon.ico differ
diff --git a/examples/st-oauth2-authorization-server/frontend/public/index.html b/examples/st-oauth2-authorization-server/frontend/public/index.html
new file mode 100644
index 000000000..6f1f7cb51
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/public/index.html
@@ -0,0 +1,40 @@
+
+
+
+
+ {/* This shows the login UI on "/auth" route */}
+ {getSuperTokensRoutesForReactRouterDom(require("react-router-dom"), PreBuiltUIList)}
+ only if the user is logged in.
+ Else it redirects the user to "/auth" */
+
+
+
+ }
+ />
+
+
+
+
+
+
+ );
+}
+
+export default App;
diff --git a/examples/st-oauth2-authorization-server/frontend/src/Home/CallAPIView.tsx b/examples/st-oauth2-authorization-server/frontend/src/Home/CallAPIView.tsx
new file mode 100644
index 000000000..6a9d510d4
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/src/Home/CallAPIView.tsx
@@ -0,0 +1,15 @@
+import axios from "axios";
+import { getApiDomain } from "../config";
+
+export default function CallAPIView() {
+ async function callAPIClicked() {
+ let response = await axios.get(getApiDomain() + "/sessioninfo");
+ window.alert("Session Information:\n" + JSON.stringify(response.data, null, 2));
+ }
+
+ return (
+
+ );
+}
diff --git a/examples/st-oauth2-authorization-server/frontend/src/assets/fonts/MenloRegular.ttf b/examples/st-oauth2-authorization-server/frontend/src/assets/fonts/MenloRegular.ttf
new file mode 100644
index 000000000..033dc6d21
Binary files /dev/null and b/examples/st-oauth2-authorization-server/frontend/src/assets/fonts/MenloRegular.ttf differ
diff --git a/examples/st-oauth2-authorization-server/frontend/src/assets/images/arrow-right-icon.svg b/examples/st-oauth2-authorization-server/frontend/src/assets/images/arrow-right-icon.svg
new file mode 100644
index 000000000..95aa1fec6
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/src/assets/images/arrow-right-icon.svg
@@ -0,0 +1,3 @@
+
diff --git a/examples/st-oauth2-authorization-server/frontend/src/assets/images/background.png b/examples/st-oauth2-authorization-server/frontend/src/assets/images/background.png
new file mode 100644
index 000000000..2147c15c2
Binary files /dev/null and b/examples/st-oauth2-authorization-server/frontend/src/assets/images/background.png differ
diff --git a/examples/st-oauth2-authorization-server/frontend/src/assets/images/blogs-icon.svg b/examples/st-oauth2-authorization-server/frontend/src/assets/images/blogs-icon.svg
new file mode 100644
index 000000000..a2fc9dd62
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/src/assets/images/blogs-icon.svg
@@ -0,0 +1,3 @@
+
diff --git a/examples/st-oauth2-authorization-server/frontend/src/assets/images/celebrate-icon.svg b/examples/st-oauth2-authorization-server/frontend/src/assets/images/celebrate-icon.svg
new file mode 100644
index 000000000..3b40b1efa
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/src/assets/images/celebrate-icon.svg
@@ -0,0 +1,9 @@
+
diff --git a/examples/st-oauth2-authorization-server/frontend/src/assets/images/guide-icon.svg b/examples/st-oauth2-authorization-server/frontend/src/assets/images/guide-icon.svg
new file mode 100644
index 000000000..bd85af72b
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/src/assets/images/guide-icon.svg
@@ -0,0 +1,3 @@
+
diff --git a/examples/st-oauth2-authorization-server/frontend/src/assets/images/index.ts b/examples/st-oauth2-authorization-server/frontend/src/assets/images/index.ts
new file mode 100644
index 000000000..7adf036c4
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/src/assets/images/index.ts
@@ -0,0 +1,8 @@
+import SeparatorLine from "./separator-line.svg";
+import ArrowRight from "./arrow-right-icon.svg";
+import SignOutIcon from "./sign-out-icon.svg";
+import GuideIcon from "./guide-icon.svg";
+import BlogsIcon from "./blogs-icon.svg";
+import CelebrateIcon from "./celebrate-icon.svg";
+
+export { SeparatorLine, ArrowRight, SignOutIcon, GuideIcon, BlogsIcon, CelebrateIcon };
diff --git a/examples/st-oauth2-authorization-server/frontend/src/assets/images/separator-line.svg b/examples/st-oauth2-authorization-server/frontend/src/assets/images/separator-line.svg
new file mode 100644
index 000000000..7127a00dc
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/src/assets/images/separator-line.svg
@@ -0,0 +1,10 @@
+
diff --git a/examples/st-oauth2-authorization-server/frontend/src/assets/images/sign-out-icon.svg b/examples/st-oauth2-authorization-server/frontend/src/assets/images/sign-out-icon.svg
new file mode 100644
index 000000000..6cc4f85fd
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/src/assets/images/sign-out-icon.svg
@@ -0,0 +1,3 @@
+
diff --git a/examples/st-oauth2-authorization-server/frontend/src/config.tsx b/examples/st-oauth2-authorization-server/frontend/src/config.tsx
new file mode 100644
index 000000000..fcb9378eb
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/src/config.tsx
@@ -0,0 +1,33 @@
+import EmailPassword from "supertokens-auth-react/recipe/emailpassword";
+import OAuth2Provider from "supertokens-auth-react/recipe/oauth2provider";
+import { EmailPasswordPreBuiltUI } from "supertokens-auth-react/recipe/emailpassword/prebuiltui";
+import Session from "supertokens-auth-react/recipe/session";
+
+export function getWebsiteDomain() {
+ return process.env.REACT_APP_AUTH_SERVER_WEBSITE_URL || "http://localhost.com:3005";
+}
+
+export function getApiDomain() {
+ return process.env.REACT_APP_AUTH_SERVER_API_URL || "http://localhost.com:3006";
+}
+
+export const SuperTokensConfig = {
+ appInfo: {
+ appName: "SuperTokens Demo App",
+ apiDomain: getApiDomain(),
+ websiteDomain: getWebsiteDomain(),
+ },
+ // recipeList contains all the modules that you want to
+ // use from SuperTokens. See the full list here: https://supertokens.com/docs/guides
+ recipeList: [EmailPassword.init(), OAuth2Provider.init(), Session.init()],
+};
+
+export const recipeDetails = {
+ docsLink: "https://supertokens.com/docs/thirdparty/introduction",
+};
+
+export const PreBuiltUIList = [EmailPasswordPreBuiltUI];
+
+export const ComponentWrapper = (props: { children: JSX.Element }): JSX.Element => {
+ return props.children;
+};
diff --git a/examples/st-oauth2-authorization-server/frontend/src/index.css b/examples/st-oauth2-authorization-server/frontend/src/index.css
new file mode 100644
index 000000000..04146b5e7
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/src/index.css
@@ -0,0 +1,11 @@
+body {
+ margin: 0;
+ font-family: -apple-system, BlinkMacSystemFont, "Segoe UI", "Roboto", "Oxygen", "Ubuntu", "Cantarell", "Fira Sans",
+ "Droid Sans", "Helvetica Neue", sans-serif;
+ -webkit-font-smoothing: antialiased;
+ -moz-osx-font-smoothing: grayscale;
+}
+
+code {
+ font-family: source-code-pro, Menlo, Monaco, Consolas, "Courier New", monospace;
+}
diff --git a/examples/st-oauth2-authorization-server/frontend/src/index.tsx b/examples/st-oauth2-authorization-server/frontend/src/index.tsx
new file mode 100644
index 000000000..399c737cd
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/src/index.tsx
@@ -0,0 +1,11 @@
+import React from "react";
+import ReactDOM from "react-dom/client";
+import "./index.css";
+import App from "./App";
+
+const root = ReactDOM.createRoot(document.getElementById("root") as HTMLElement);
+root.render(
+
+
+
+);
diff --git a/examples/st-oauth2-authorization-server/frontend/src/react-app-env.d.ts b/examples/st-oauth2-authorization-server/frontend/src/react-app-env.d.ts
new file mode 100644
index 000000000..6431bc5fc
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/src/react-app-env.d.ts
@@ -0,0 +1 @@
+///
diff --git a/examples/st-oauth2-authorization-server/frontend/tsconfig.json b/examples/st-oauth2-authorization-server/frontend/tsconfig.json
new file mode 100644
index 000000000..c0555cbc6
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/frontend/tsconfig.json
@@ -0,0 +1,20 @@
+{
+ "compilerOptions": {
+ "target": "es5",
+ "lib": ["dom", "dom.iterable", "esnext"],
+ "allowJs": true,
+ "skipLibCheck": true,
+ "esModuleInterop": true,
+ "allowSyntheticDefaultImports": true,
+ "strict": true,
+ "forceConsistentCasingInFileNames": true,
+ "noFallthroughCasesInSwitch": true,
+ "module": "esnext",
+ "moduleResolution": "node",
+ "resolveJsonModule": true,
+ "isolatedModules": true,
+ "noEmit": true,
+ "jsx": "react-jsx"
+ },
+ "include": ["src"]
+}
diff --git a/examples/st-oauth2-authorization-server/package.json b/examples/st-oauth2-authorization-server/package.json
new file mode 100644
index 000000000..7f4d72673
--- /dev/null
+++ b/examples/st-oauth2-authorization-server/package.json
@@ -0,0 +1,49 @@
+{
+ "name": "st-oauth2-authorization-server",
+ "version": "0.0.1",
+ "description": "",
+ "main": "index.js",
+ "scripts": {
+ "start:frontend": "cd frontend && npm run start",
+ "start:frontend-live-demo-app": "cd frontend && npx serve -s build",
+ "start:backend": "cd backend && npm run start",
+ "start:backend-live-demo-app": "cd backend && ./startLiveDemoApp.sh",
+ "start": "npm-run-all --parallel start:frontend start:backend",
+ "start-live-demo-app": "npx npm-run-all --parallel start:frontend-live-demo-app start:backend-live-demo-app"
+ },
+ "keywords": [],
+ "author": "",
+ "license": "ISC",
+ "dependencies": {
+ "@testing-library/jest-dom": "^5.16.5",
+ "@testing-library/react": "^13.4.0",
+ "@testing-library/user-event": "^13.5.0",
+ "@types/jest": "^27.5.2",
+ "@types/node": "^16.11.56",
+ "@types/react": "^18.0.18",
+ "@types/react-dom": "^18.0.6",
+ "axios": "^0.21.0",
+ "cors": "^2.8.5",
+ "express": "^4.18.1",
+ "helmet": "^5.1.0",
+ "morgan": "^1.10.0",
+ "react": "^18.2.0",
+ "react-dom": "^18.2.0",
+ "react-router-dom": "^6.2.1",
+ "react-scripts": "5.0.1",
+ "supertokens-auth-react": "github:supertokens/supertokens-auth-react#feat/oauth2/base",
+ "supertokens-node": "github:supertokens/supertokens-node#feat/oauth2/base",
+ "supertokens-web-js": "github:supertokens/supertokens-web-js#feat/oauth2/base",
+ "ts-node-dev": "^2.0.0",
+ "typescript": "^4.8.2",
+ "web-vitals": "^2.1.4"
+ },
+ "devDependencies": {
+ "@types/cors": "^2.8.12",
+ "@types/express": "^4.17.17",
+ "@types/morgan": "^1.9.3",
+ "@types/node": "^16.11.38",
+ "nodemon": "^2.0.16",
+ "npm-run-all": "^4.1.5"
+ }
+}
diff --git a/examples/with-oauth2-with-supertokens/README.md b/examples/with-oauth2-with-supertokens/README.md
new file mode 100644
index 000000000..8734320ea
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/README.md
@@ -0,0 +1,57 @@
+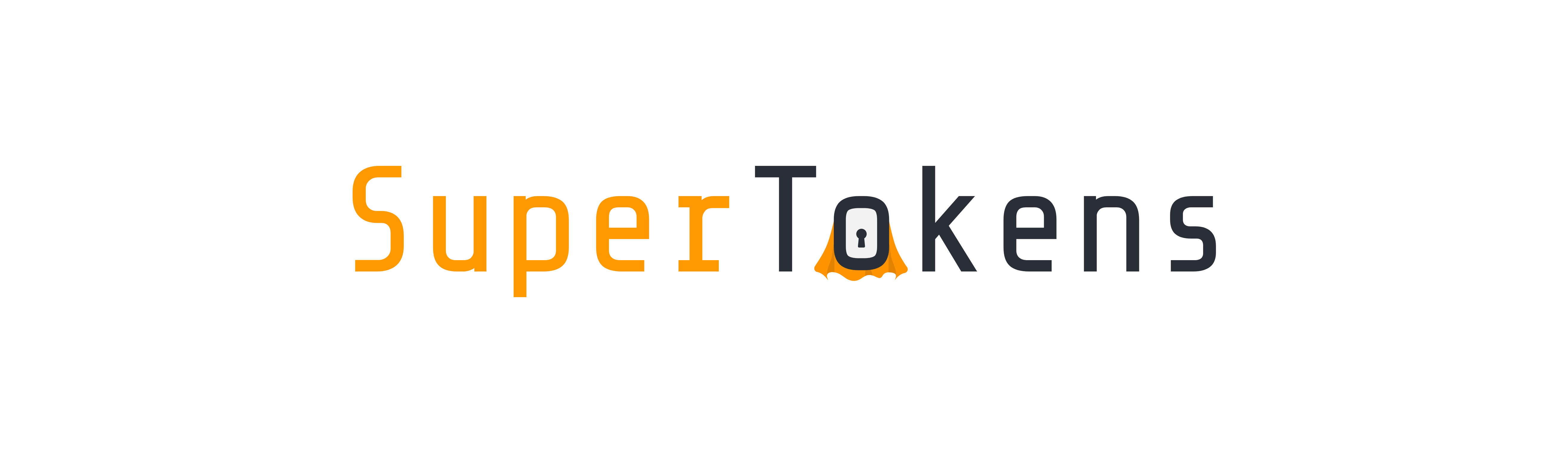
+
+# Example App with SuperTokens Auth and SuperTokens as OAuth2 Provider
+
+This example app shows how to use SuperTokens as an OAuth2 provider in a React app that already implements SuperTokens Auth, utilizing the `OAuth2Client` recipe on the backend.
+
+## Project setup
+
+Clone the repo, enter the directory, and use `npm` to install the project dependencies:
+
+```bash
+git clone https://github.com/supertokens/supertokens-auth-react
+cd supertokens-auth-react/examples/with-oauth2-with-supertokens
+npm install
+```
+
+## 1. Create an OAuth2 Client
+
+```bash
+curl -X POST http://localhost:3567/recipe/oauth/clients \
+ -H "Content-Type: application/json" \
+ -d '{
+ "scope": "offline_access openid email",
+ "redirectUris": ["http://localhost:3000/auth/callback"],
+ "tokenEndpointAuthMethod": "none",
+ "grantTypes": ["authorization_code", "refresh_token"],
+ "responseTypes": ["code", "id_token"]
+ }'
+```
+
+Note down the `client_id` from the response.
+
+## 2. Run the st-oauth2-authorization-server
+
+1. Open a new terminal window and navigate to `supertokens-auth-react/examples/
+st-oauth2-authorization-server`
+2. Read the README.md to setup `st-oauth2-authorization-server ` and run it using `npm start`
+
+## 3. Update config
+
+Update `clientId` and `AUTH_SERVER_URL` values as per step 1 and step 2 in both `frontend/src/App.tsx` and `backend/src/config.ts`.
+
+## 4. Run the demo app
+
+This compiles and serves the React app.
+
+```bash
+npm run start
+```
+
+## Author
+
+Created with :heart: by the folks at supertokens.com.
+
+## License
+
+This project is licensed under the Apache 2.0 license.
diff --git a/examples/with-oauth2-with-supertokens/backend/config.ts b/examples/with-oauth2-with-supertokens/backend/config.ts
new file mode 100644
index 000000000..02b0370ec
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/backend/config.ts
@@ -0,0 +1,40 @@
+import OAuth2Client from "supertokens-node/recipe/oauth2client";
+import Session from "supertokens-node/recipe/session";
+import { TypeInput } from "supertokens-node/types";
+
+const AUTH_SERVER_URL = process.env.REACT_APP_AUTH_SERVER_API_URL || "http://localhost.com:3006";
+
+export function getApiDomain() {
+ const apiPort = process.env.REACT_APP_API_PORT || 3001;
+ const apiUrl = process.env.REACT_APP_API_URL || `http://localhost:${apiPort}`;
+ return apiUrl;
+}
+
+export function getWebsiteDomain() {
+ const websitePort = process.env.REACT_APP_WEBSITE_PORT || 3000;
+ const websiteUrl = process.env.REACT_APP_WEBSITE_URL || `http://localhost:${websitePort}`;
+ return websiteUrl;
+}
+
+export const SuperTokensConfig: TypeInput = {
+ supertokens: {
+ // this is the location of the SuperTokens core.
+ connectionURI: "https://try.supertokens.com",
+ },
+ appInfo: {
+ appName: "SuperTokens Demo App",
+ apiDomain: getApiDomain(),
+ websiteDomain: getWebsiteDomain(),
+ },
+ // recipeList contains all the modules that you want to
+ // use from SuperTokens. See the full list here: https://supertokens.com/docs/guides
+ recipeList: [
+ OAuth2Client.init({
+ providerConfig: {
+ clientId: "REPLACE_WITH_YOUR_CLIENT_ID",
+ oidcDiscoveryEndpoint: `${AUTH_SERVER_URL}/auth/.well-known/openid-configuration`,
+ },
+ }),
+ Session.init(),
+ ],
+};
diff --git a/examples/with-oauth2-with-supertokens/backend/index.ts b/examples/with-oauth2-with-supertokens/backend/index.ts
new file mode 100644
index 000000000..67c228198
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/backend/index.ts
@@ -0,0 +1,38 @@
+import express from "express";
+import cors from "cors";
+import supertokens from "supertokens-node";
+import { verifySession } from "supertokens-node/recipe/session/framework/express";
+import { middleware, errorHandler, SessionRequest } from "supertokens-node/framework/express";
+import { getWebsiteDomain, SuperTokensConfig } from "./config";
+
+supertokens.init(SuperTokensConfig);
+
+const app = express();
+
+app.use(
+ cors({
+ origin: getWebsiteDomain(),
+ allowedHeaders: ["content-type", ...supertokens.getAllCORSHeaders()],
+ methods: ["GET", "PUT", "POST", "DELETE"],
+ credentials: true,
+ })
+);
+
+// This exposes all the APIs from SuperTokens to the client.
+app.use(middleware());
+
+// An example API that requires session verification
+app.get("/sessioninfo", verifySession(), async (req: SessionRequest, res) => {
+ let session = req.session;
+ res.send({
+ sessionHandle: session!.getHandle(),
+ userId: session!.getUserId(),
+ accessTokenPayload: session!.getAccessTokenPayload(),
+ });
+});
+
+// In case of session related errors, this error handler
+// returns 401 to the client.
+app.use(errorHandler());
+
+app.listen(3001, () => console.log(`API Server listening on port 3001`));
diff --git a/examples/with-oauth2-with-supertokens/backend/package.json b/examples/with-oauth2-with-supertokens/backend/package.json
new file mode 100644
index 000000000..5d69ff8af
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/backend/package.json
@@ -0,0 +1,15 @@
+{
+ "name": "supertokens-node",
+ "version": "0.0.1",
+ "private": true,
+ "description": "",
+ "main": "index.js",
+ "scripts": {
+ "start": "npx ts-node-dev --project ./tsconfig.json ./index.ts"
+ },
+ "dependencies": {},
+ "devDependencies": {},
+ "keywords": [],
+ "author": "",
+ "license": "ISC"
+}
diff --git a/examples/with-oauth2-with-supertokens/backend/tsconfig.json b/examples/with-oauth2-with-supertokens/backend/tsconfig.json
new file mode 100644
index 000000000..8a91acaae
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/backend/tsconfig.json
@@ -0,0 +1,62 @@
+{
+ "compilerOptions": {
+ /* Visit https://aka.ms/tsconfig.json to read more about this file */
+ /* Basic Options */
+ // "incremental": true, /* Enable incremental compilation */
+ "target": "es5" /* Specify ECMAScript target version: 'ES3' (default), 'ES5', 'ES2015', 'ES2016', 'ES2017', 'ES2018', 'ES2019', 'ES2020', or 'ESNEXT'. */,
+ "module": "commonjs" /* Specify module code generation: 'none', 'commonjs', 'amd', 'system', 'umd', 'es2015', 'es2020', or 'ESNext'. */,
+ // "lib": [], /* Specify library files to be included in the compilation. */
+ // "allowJs": true, /* Allow javascript files to be compiled. */
+ // "checkJs": true, /* Report errors in .js files. */
+ // "jsx": "preserve", /* Specify JSX code generation: 'preserve', 'react-native', or 'react'. */
+ // "declaration": true, /* Generates corresponding '.d.ts' file. */
+ // "declarationMap": true, /* Generates a sourcemap for each corresponding '.d.ts' file. */
+ // "sourceMap": true, /* Generates corresponding '.map' file. */
+ // "outFile": "./", /* Concatenate and emit output to single file. */
+ // "outDir": "./", /* Redirect output structure to the directory. */
+ // "rootDir": "./", /* Specify the root directory of input files. Use to control the output directory structure with --outDir. */
+ // "composite": true, /* Enable project compilation */
+ // "tsBuildInfoFile": "./", /* Specify file to store incremental compilation information */
+ // "removeComments": true, /* Do not emit comments to output. */
+ // "noEmit": true, /* Do not emit outputs. */
+ // "importHelpers": true, /* Import emit helpers from 'tslib'. */
+ // "downlevelIteration": true, /* Provide full support for iterables in 'for-of', spread, and destructuring when targeting 'ES5' or 'ES3'. */
+ // "isolatedModules": true, /* Transpile each file as a separate module (similar to 'ts.transpileModule'). */
+ /* Strict Type-Checking Options */
+ "strict": true /* Enable all strict type-checking options. */,
+ // "noImplicitAny": true, /* Raise error on expressions and declarations with an implied 'any' type. */
+ // "strictNullChecks": true, /* Enable strict null checks. */
+ // "strictFunctionTypes": true, /* Enable strict checking of function types. */
+ // "strictBindCallApply": true, /* Enable strict 'bind', 'call', and 'apply' methods on functions. */
+ // "strictPropertyInitialization": true, /* Enable strict checking of property initialization in classes. */
+ // "noImplicitThis": true, /* Raise error on 'this' expressions with an implied 'any' type. */
+ // "alwaysStrict": true, /* Parse in strict mode and emit "use strict" for each source file. */
+ /* Additional Checks */
+ // "noUnusedLocals": true, /* Report errors on unused locals. */
+ // "noUnusedParameters": true, /* Report errors on unused parameters. */
+ // "noImplicitReturns": true, /* Report error when not all code paths in function return a value. */
+ // "noFallthroughCasesInSwitch": true, /* Report errors for fallthrough cases in switch statement. */
+ /* Module Resolution Options */
+ // "moduleResolution": "node", /* Specify module resolution strategy: 'node' (Node.js) or 'classic' (TypeScript pre-1.6). */
+ // "baseUrl": "./", /* Base directory to resolve non-absolute module names. */
+ // "paths": {}, /* A series of entries which re-map imports to lookup locations relative to the 'baseUrl'. */
+ // "rootDirs": [], /* List of root folders whose combined content represents the structure of the project at runtime. */
+ // "typeRoots": [], /* List of folders to include type definitions from. */
+ // "types": [], /* Type declaration files to be included in compilation. */
+ // "allowSyntheticDefaultImports": true, /* Allow default imports from modules with no default export. This does not affect code emit, just typechecking. */
+ "esModuleInterop": true /* Enables emit interoperability between CommonJS and ES Modules via creation of namespace objects for all imports. Implies 'allowSyntheticDefaultImports'. */,
+ // "preserveSymlinks": true, /* Do not resolve the real path of symlinks. */
+ // "allowUmdGlobalAccess": true, /* Allow accessing UMD globals from modules. */
+ /* Source Map Options */
+ // "sourceRoot": "", /* Specify the location where debugger should locate TypeScript files instead of source locations. */
+ // "mapRoot": "", /* Specify the location where debugger should locate map files instead of generated locations. */
+ // "inlineSourceMap": true, /* Emit a single file with source maps instead of having a separate file. */
+ // "inlineSources": true, /* Emit the source alongside the sourcemaps within a single file; requires '--inlineSourceMap' or '--sourceMap' to be set. */
+ /* Experimental Options */
+ // "experimentalDecorators": true, /* Enables experimental support for ES7 decorators. */
+ // "emitDecoratorMetadata": true, /* Enables experimental support for emitting type metadata for decorators. */
+ /* Advanced Options */
+ "skipLibCheck": true /* Skip type checking of declaration files. */,
+ "forceConsistentCasingInFileNames": true /* Disallow inconsistently-cased references to the same file. */
+ }
+}
diff --git a/examples/with-oauth2-with-supertokens/frontend/.env b/examples/with-oauth2-with-supertokens/frontend/.env
new file mode 100644
index 000000000..7d910f148
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/.env
@@ -0,0 +1 @@
+SKIP_PREFLIGHT_CHECK=true
\ No newline at end of file
diff --git a/examples/with-oauth2-with-supertokens/frontend/.gitignore b/examples/with-oauth2-with-supertokens/frontend/.gitignore
new file mode 100644
index 000000000..4d29575de
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/.gitignore
@@ -0,0 +1,23 @@
+# See https://help.github.com/articles/ignoring-files/ for more about ignoring files.
+
+# dependencies
+/node_modules
+/.pnp
+.pnp.js
+
+# testing
+/coverage
+
+# production
+/build
+
+# misc
+.DS_Store
+.env.local
+.env.development.local
+.env.test.local
+.env.production.local
+
+npm-debug.log*
+yarn-debug.log*
+yarn-error.log*
diff --git a/examples/with-oauth2-with-supertokens/frontend/LICENSE.md b/examples/with-oauth2-with-supertokens/frontend/LICENSE.md
new file mode 100644
index 000000000..588f27e68
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/LICENSE.md
@@ -0,0 +1,192 @@
+Copyright (c) 2020, VRAI Labs and/or its affiliates. All rights reserved.
+
+This software is licensed under the Apache License, Version 2.0 (the
+"License") as published by the Apache Software Foundation.
+
+You may not use this software except in compliance with the License. A copy
+of the License is available below the line.
+
+Unless required by applicable law or agreed to in writing, software
+distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+License for the specific language governing permissions and limitations
+under the License.
+
+---
+
+ Apache License
+ Version 2.0, January 2004
+ http://www.apache.org/licenses/
+
+TERMS AND CONDITIONS FOR USE, REPRODUCTION, AND DISTRIBUTION
+
+1. Definitions.
+
+ "License" shall mean the terms and conditions for use, reproduction,
+ and distribution as defined by Sections 1 through 9 of this document.
+
+ "Licensor" shall mean the copyright owner or entity authorized by
+ the copyright owner that is granting the License.
+
+ "Legal Entity" shall mean the union of the acting entity and all
+ other entities that control, are controlled by, or are under common
+ control with that entity. For the purposes of this definition,
+ "control" means (i) the power, direct or indirect, to cause the
+ direction or management of such entity, whether by contract or
+ otherwise, or (ii) ownership of fifty percent (50%) or more of the
+ outstanding shares, or (iii) beneficial ownership of such entity.
+
+ "You" (or "Your") shall mean an individual or Legal Entity
+ exercising permissions granted by this License.
+
+ "Source" form shall mean the preferred form for making modifications,
+ including but not limited to software source code, documentation
+ source, and configuration files.
+
+ "Object" form shall mean any form resulting from mechanical
+ transformation or translation of a Source form, including but
+ not limited to compiled object code, generated documentation,
+ and conversions to other media types.
+
+ "Work" shall mean the work of authorship, whether in Source or
+ Object form, made available under the License, as indicated by a
+ copyright notice that is included in or attached to the work
+ (an example is provided in the Appendix below).
+
+ "Derivative Works" shall mean any work, whether in Source or Object
+ form, that is based on (or derived from) the Work and for which the
+ editorial revisions, annotations, elaborations, or other modifications
+ represent, as a whole, an original work of authorship. For the purposes
+ of this License, Derivative Works shall not include works that remain
+ separable from, or merely link (or bind by name) to the interfaces of,
+ the Work and Derivative Works thereof.
+
+ "Contribution" shall mean any work of authorship, including
+ the original version of the Work and any modifications or additions
+ to that Work or Derivative Works thereof, that is intentionally
+ submitted to Licensor for inclusion in the Work by the copyright owner
+ or by an individual or Legal Entity authorized to submit on behalf of
+ the copyright owner. For the purposes of this definition, "submitted"
+ means any form of electronic, verbal, or written communication sent
+ to the Licensor or its representatives, including but not limited to
+ communication on electronic mailing lists, source code control systems,
+ and issue tracking systems that are managed by, or on behalf of, the
+ Licensor for the purpose of discussing and improving the Work, but
+ excluding communication that is conspicuously marked or otherwise
+ designated in writing by the copyright owner as "Not a Contribution."
+
+ "Contributor" shall mean Licensor and any individual or Legal Entity
+ on behalf of whom a Contribution has been received by Licensor and
+ subsequently incorporated within the Work.
+
+2. Grant of Copyright License. Subject to the terms and conditions of
+ this License, each Contributor hereby grants to You a perpetual,
+ worldwide, non-exclusive, no-charge, royalty-free, irrevocable
+ copyright license to reproduce, prepare Derivative Works of,
+ publicly display, publicly perform, sublicense, and distribute the
+ Work and such Derivative Works in Source or Object form.
+
+3. Grant of Patent License. Subject to the terms and conditions of
+ this License, each Contributor hereby grants to You a perpetual,
+ worldwide, non-exclusive, no-charge, royalty-free, irrevocable
+ (except as stated in this section) patent license to make, have made,
+ use, offer to sell, sell, import, and otherwise transfer the Work,
+ where such license applies only to those patent claims licensable
+ by such Contributor that are necessarily infringed by their
+ Contribution(s) alone or by combination of their Contribution(s)
+ with the Work to which such Contribution(s) was submitted. If You
+ institute patent litigation against any entity (including a
+ cross-claim or counterclaim in a lawsuit) alleging that the Work
+ or a Contribution incorporated within the Work constitutes direct
+ or contributory patent infringement, then any patent licenses
+ granted to You under this License for that Work shall terminate
+ as of the date such litigation is filed.
+
+4. Redistribution. You may reproduce and distribute copies of the
+ Work or Derivative Works thereof in any medium, with or without
+ modifications, and in Source or Object form, provided that You
+ meet the following conditions:
+
+ (a) You must give any other recipients of the Work or
+ Derivative Works a copy of this License; and
+
+ (b) You must cause any modified files to carry prominent notices
+ stating that You changed the files; and
+
+ (c) You must retain, in the Source form of any Derivative Works
+ that You distribute, all copyright, patent, trademark, and
+ attribution notices from the Source form of the Work,
+ excluding those notices that do not pertain to any part of
+ the Derivative Works; and
+
+ (d) If the Work includes a "NOTICE" text file as part of its
+ distribution, then any Derivative Works that You distribute must
+ include a readable copy of the attribution notices contained
+ within such NOTICE file, excluding those notices that do not
+ pertain to any part of the Derivative Works, in at least one
+ of the following places: within a NOTICE text file distributed
+ as part of the Derivative Works; within the Source form or
+ documentation, if provided along with the Derivative Works; or,
+ within a display generated by the Derivative Works, if and
+ wherever such third-party notices normally appear. The contents
+ of the NOTICE file are for informational purposes only and
+ do not modify the License. You may add Your own attribution
+ notices within Derivative Works that You distribute, alongside
+ or as an addendum to the NOTICE text from the Work, provided
+ that such additional attribution notices cannot be construed
+ as modifying the License.
+
+ You may add Your own copyright statement to Your modifications and
+ may provide additional or different license terms and conditions
+ for use, reproduction, or distribution of Your modifications, or
+ for any such Derivative Works as a whole, provided Your use,
+ reproduction, and distribution of the Work otherwise complies with
+ the conditions stated in this License.
+
+5. Submission of Contributions. Unless You explicitly state otherwise,
+ any Contribution intentionally submitted for inclusion in the Work
+ by You to the Licensor shall be under the terms and conditions of
+ this License, without any additional terms or conditions.
+ Notwithstanding the above, nothing herein shall supersede or modify
+ the terms of any separate license agreement you may have executed
+ with Licensor regarding such Contributions.
+
+6. Trademarks. This License does not grant permission to use the trade
+ names, trademarks, service marks, or product names of the Licensor,
+ except as required for reasonable and customary use in describing the
+ origin of the Work and reproducing the content of the NOTICE file.
+
+7. Disclaimer of Warranty. Unless required by applicable law or
+ agreed to in writing, Licensor provides the Work (and each
+ Contributor provides its Contributions) on an "AS IS" BASIS,
+ WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
+ implied, including, without limitation, any warranties or conditions
+ of TITLE, NON-INFRINGEMENT, MERCHANTABILITY, or FITNESS FOR A
+ PARTICULAR PURPOSE. You are solely responsible for determining the
+ appropriateness of using or redistributing the Work and assume any
+ risks associated with Your exercise of permissions under this License.
+
+8. Limitation of Liability. In no event and under no legal theory,
+ whether in tort (including negligence), contract, or otherwise,
+ unless required by applicable law (such as deliberate and grossly
+ negligent acts) or agreed to in writing, shall any Contributor be
+ liable to You for damages, including any direct, indirect, special,
+ incidental, or consequential damages of any character arising as a
+ result of this License or out of the use or inability to use the
+ Work (including but not limited to damages for loss of goodwill,
+ work stoppage, computer failure or malfunction, or any and all
+ other commercial damages or losses), even if such Contributor
+ has been advised of the possibility of such damages.
+
+9. Accepting Warranty or Additional Liability. While redistributing
+ the Work or Derivative Works thereof, You may choose to offer,
+ and charge a fee for, acceptance of support, warranty, indemnity,
+ or other liability obligations and/or rights consistent with this
+ License. However, in accepting such obligations, You may act only
+ on Your own behalf and on Your sole responsibility, not on behalf
+ of any other Contributor, and only if You agree to indemnify,
+ defend, and hold each Contributor harmless for any liability
+ incurred by, or claims asserted against, such Contributor by reason
+ of your accepting any such warranty or additional liability.
+
+END OF TERMS AND CONDITIONS
diff --git a/examples/with-oauth2-with-supertokens/frontend/package.json b/examples/with-oauth2-with-supertokens/frontend/package.json
new file mode 100644
index 000000000..0c9b5703f
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/package.json
@@ -0,0 +1,29 @@
+{
+ "name": "supertokens-react",
+ "version": "0.1.0",
+ "private": true,
+ "scripts": {
+ "start": "react-scripts start",
+ "build": "react-scripts build",
+ "test": "react-scripts test",
+ "eject": "react-scripts eject"
+ },
+ "eslintConfig": {
+ "extends": [
+ "react-app",
+ "react-app/jest"
+ ]
+ },
+ "browserslist": {
+ "production": [
+ ">0.2%",
+ "not dead",
+ "not op_mini all"
+ ],
+ "development": [
+ "last 1 chrome version",
+ "last 1 firefox version",
+ "last 1 safari version"
+ ]
+ }
+}
diff --git a/examples/with-oauth2-with-supertokens/frontend/public/favicon.ico b/examples/with-oauth2-with-supertokens/frontend/public/favicon.ico
new file mode 100644
index 000000000..a11777cc4
Binary files /dev/null and b/examples/with-oauth2-with-supertokens/frontend/public/favicon.ico differ
diff --git a/examples/with-oauth2-with-supertokens/frontend/public/index.html b/examples/with-oauth2-with-supertokens/frontend/public/index.html
new file mode 100644
index 000000000..6f1f7cb51
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/public/index.html
@@ -0,0 +1,40 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+ React App
+
+
+
+
+
+
diff --git a/examples/with-oauth2-with-supertokens/frontend/public/manifest.json b/examples/with-oauth2-with-supertokens/frontend/public/manifest.json
new file mode 100644
index 000000000..f01493ff0
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/public/manifest.json
@@ -0,0 +1,25 @@
+{
+ "short_name": "React App",
+ "name": "Create React App Sample",
+ "icons": [
+ {
+ "src": "favicon.ico",
+ "sizes": "64x64 32x32 24x24 16x16",
+ "type": "image/x-icon"
+ },
+ {
+ "src": "logo192.png",
+ "type": "image/png",
+ "sizes": "192x192"
+ },
+ {
+ "src": "logo512.png",
+ "type": "image/png",
+ "sizes": "512x512"
+ }
+ ],
+ "start_url": ".",
+ "display": "standalone",
+ "theme_color": "#000000",
+ "background_color": "#ffffff"
+}
diff --git a/examples/with-oauth2-with-supertokens/frontend/public/robots.txt b/examples/with-oauth2-with-supertokens/frontend/public/robots.txt
new file mode 100644
index 000000000..e9e57dc4d
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/public/robots.txt
@@ -0,0 +1,3 @@
+# https://www.robotstxt.org/robotstxt.html
+User-agent: *
+Disallow:
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/App.css b/examples/with-oauth2-with-supertokens/frontend/src/App.css
new file mode 100644
index 000000000..b9ee98c15
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/src/App.css
@@ -0,0 +1,34 @@
+.App {
+ display: flex;
+ flex-direction: column;
+ width: 100vw;
+ height: 100vh;
+ font-family: Rubik;
+}
+
+button,
+.sessionButton {
+ padding-left: 13px;
+ padding-right: 13px;
+ padding-top: 8px;
+ padding-bottom: 8px;
+ background-color: black;
+ border-radius: 10px;
+ cursor: pointer;
+ color: white;
+ font-weight: bold;
+ font-size: 17px;
+}
+
+.center {
+ display: flex;
+ flex-direction: column;
+ align-items: center;
+}
+
+.fill {
+ display: flex;
+ flex-direction: column;
+ justify-content: center;
+ flex: 1;
+}
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/App.tsx b/examples/with-oauth2-with-supertokens/frontend/src/App.tsx
new file mode 100644
index 000000000..26367cd49
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/src/App.tsx
@@ -0,0 +1,127 @@
+import "./App.css";
+import SuperTokens, { SuperTokensWrapper } from "supertokens-auth-react";
+import { SessionAuth, doesSessionExist } from "supertokens-auth-react/recipe/session";
+import { Routes, BrowserRouter as Router, Route, useNavigate } from "react-router-dom";
+import Home from "./Home";
+import { SuperTokensConfig, ComponentWrapper, getApiDomain, getWebsiteDomain } from "./config";
+import { useEffect, useRef } from "react";
+import { AuthProvider, AuthProviderProps, useAuth } from "react-oidc-context";
+import "./App.css";
+
+SuperTokens.init(SuperTokensConfig);
+
+const AUTH_SERVER_URL = process.env.REACT_APP_AUTH_SERVER_API_URL || "http://localhost.com:3006";
+const clientId = "REPLACE_WITH_YOUR_CLIENT_ID";
+
+const oidcConfig: AuthProviderProps = {
+ client_id: clientId,
+ authority: `${AUTH_SERVER_URL}/auth`,
+ response_type: "code",
+ redirect_uri: `${getWebsiteDomain()}/auth/callback`,
+ scope: "openid offline_access email",
+};
+
+function AuthPage() {
+ const navigate = useNavigate();
+ const authCallbackHandled = useRef(false);
+ const { signinRedirect, signoutSilent, isLoading, user } = useAuth();
+
+ useEffect(() => {
+ // Redirect to home if supertokens session exists
+ doesSessionExist().then((sessionExists) => {
+ if (sessionExists) {
+ navigate("/");
+ }
+ });
+ }, []);
+
+ useEffect(() => {
+ if (authCallbackHandled.current) return;
+ if (!user?.access_token) return;
+
+ authCallbackHandled.current = true;
+
+ async function handleSign() {
+ const res = await fetch(`${getApiDomain()}/auth/oauth/client/signin`, {
+ method: "POST",
+ headers: {
+ "Content-Type": "application/json",
+ },
+ body: JSON.stringify({
+ oAuthTokens: {
+ access_token: user?.access_token,
+ },
+ }),
+ });
+ if (res.ok) {
+ const data = await res.json();
+ if (data.status === "OK") {
+ signoutSilent();
+ navigate("/");
+ } else {
+ // setIsLoading(false);
+ window.alert("Login Failed ");
+ }
+ }
+ }
+
+ handleSign();
+ }, [user, navigate, signoutSilent]);
+
+ if (isLoading || user) {
+ return (
+
+
Loading...
+
+ );
+ }
+
+ return (
+
+
OAuth2 Example With Supertokens
+
+
+
+
+
+
+ );
+}
+
+function App() {
+ return (
+
+
+
+
+
+
+
+
+
+ }
+ />
+ only if the user is logged in.
+ Else it redirects the user to "/auth" */
+
+
+
+ }
+ />
+
+
+
+
+
+
+ );
+}
+
+export default App;
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/Home/CallAPIView.tsx b/examples/with-oauth2-with-supertokens/frontend/src/Home/CallAPIView.tsx
new file mode 100644
index 000000000..6a9d510d4
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/src/Home/CallAPIView.tsx
@@ -0,0 +1,15 @@
+import axios from "axios";
+import { getApiDomain } from "../config";
+
+export default function CallAPIView() {
+ async function callAPIClicked() {
+ let response = await axios.get(getApiDomain() + "/sessioninfo");
+ window.alert("Session Information:\n" + JSON.stringify(response.data, null, 2));
+ }
+
+ return (
+
+ );
+}
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/assets/fonts/MenloRegular.ttf b/examples/with-oauth2-with-supertokens/frontend/src/assets/fonts/MenloRegular.ttf
new file mode 100644
index 000000000..033dc6d21
Binary files /dev/null and b/examples/with-oauth2-with-supertokens/frontend/src/assets/fonts/MenloRegular.ttf differ
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/assets/images/arrow-right-icon.svg b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/arrow-right-icon.svg
new file mode 100644
index 000000000..95aa1fec6
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/arrow-right-icon.svg
@@ -0,0 +1,3 @@
+
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/assets/images/background.png b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/background.png
new file mode 100644
index 000000000..2147c15c2
Binary files /dev/null and b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/background.png differ
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/assets/images/blogs-icon.svg b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/blogs-icon.svg
new file mode 100644
index 000000000..a2fc9dd62
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/blogs-icon.svg
@@ -0,0 +1,3 @@
+
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/assets/images/celebrate-icon.svg b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/celebrate-icon.svg
new file mode 100644
index 000000000..3b40b1efa
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/celebrate-icon.svg
@@ -0,0 +1,9 @@
+
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/assets/images/guide-icon.svg b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/guide-icon.svg
new file mode 100644
index 000000000..bd85af72b
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/guide-icon.svg
@@ -0,0 +1,3 @@
+
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/assets/images/index.ts b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/index.ts
new file mode 100644
index 000000000..7adf036c4
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/index.ts
@@ -0,0 +1,8 @@
+import SeparatorLine from "./separator-line.svg";
+import ArrowRight from "./arrow-right-icon.svg";
+import SignOutIcon from "./sign-out-icon.svg";
+import GuideIcon from "./guide-icon.svg";
+import BlogsIcon from "./blogs-icon.svg";
+import CelebrateIcon from "./celebrate-icon.svg";
+
+export { SeparatorLine, ArrowRight, SignOutIcon, GuideIcon, BlogsIcon, CelebrateIcon };
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/assets/images/separator-line.svg b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/separator-line.svg
new file mode 100644
index 000000000..7127a00dc
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/separator-line.svg
@@ -0,0 +1,10 @@
+
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/assets/images/sign-out-icon.svg b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/sign-out-icon.svg
new file mode 100644
index 000000000..6cc4f85fd
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/src/assets/images/sign-out-icon.svg
@@ -0,0 +1,3 @@
+
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/config.tsx b/examples/with-oauth2-with-supertokens/frontend/src/config.tsx
new file mode 100644
index 000000000..a12a11d12
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/src/config.tsx
@@ -0,0 +1,34 @@
+import Session from "supertokens-auth-react/recipe/session";
+
+export function getApiDomain() {
+ const apiPort = process.env.REACT_APP_API_PORT || 3001;
+ const apiUrl = process.env.REACT_APP_API_URL || `http://localhost:${apiPort}`;
+ return apiUrl;
+}
+
+export function getWebsiteDomain() {
+ const websitePort = process.env.REACT_APP_WEBSITE_PORT || 3000;
+ const websiteUrl = process.env.REACT_APP_WEBSITE_URL || `http://localhost:${websitePort}`;
+ return websiteUrl;
+}
+
+export const SuperTokensConfig = {
+ appInfo: {
+ appName: "SuperTokens Demo App",
+ apiDomain: getApiDomain(),
+ websiteDomain: getWebsiteDomain(),
+ },
+ // recipeList contains all the modules that you want to
+ // use from SuperTokens. See the full list here: https://supertokens.com/docs/guides
+ recipeList: [Session.init()],
+};
+
+export const recipeDetails = {
+ docsLink: "https://supertokens.com/docs/thirdparty/introduction",
+};
+
+export const PreBuiltUIList = [];
+
+export const ComponentWrapper = (props: { children: JSX.Element }): JSX.Element => {
+ return props.children;
+};
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/index.css b/examples/with-oauth2-with-supertokens/frontend/src/index.css
new file mode 100644
index 000000000..04146b5e7
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/src/index.css
@@ -0,0 +1,11 @@
+body {
+ margin: 0;
+ font-family: -apple-system, BlinkMacSystemFont, "Segoe UI", "Roboto", "Oxygen", "Ubuntu", "Cantarell", "Fira Sans",
+ "Droid Sans", "Helvetica Neue", sans-serif;
+ -webkit-font-smoothing: antialiased;
+ -moz-osx-font-smoothing: grayscale;
+}
+
+code {
+ font-family: source-code-pro, Menlo, Monaco, Consolas, "Courier New", monospace;
+}
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/index.tsx b/examples/with-oauth2-with-supertokens/frontend/src/index.tsx
new file mode 100644
index 000000000..126116f9b
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/src/index.tsx
@@ -0,0 +1,12 @@
+import React from "react";
+import ReactDOM from "react-dom/client";
+import "./index.css";
+import App from "./App";
+
+const root = ReactDOM.createRoot(document.getElementById("root") as HTMLElement);
+
+root.render(
+
+
+
+);
diff --git a/examples/with-oauth2-with-supertokens/frontend/src/react-app-env.d.ts b/examples/with-oauth2-with-supertokens/frontend/src/react-app-env.d.ts
new file mode 100644
index 000000000..6431bc5fc
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/src/react-app-env.d.ts
@@ -0,0 +1 @@
+///
diff --git a/examples/with-oauth2-with-supertokens/frontend/tsconfig.json b/examples/with-oauth2-with-supertokens/frontend/tsconfig.json
new file mode 100644
index 000000000..c0555cbc6
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/frontend/tsconfig.json
@@ -0,0 +1,20 @@
+{
+ "compilerOptions": {
+ "target": "es5",
+ "lib": ["dom", "dom.iterable", "esnext"],
+ "allowJs": true,
+ "skipLibCheck": true,
+ "esModuleInterop": true,
+ "allowSyntheticDefaultImports": true,
+ "strict": true,
+ "forceConsistentCasingInFileNames": true,
+ "noFallthroughCasesInSwitch": true,
+ "module": "esnext",
+ "moduleResolution": "node",
+ "resolveJsonModule": true,
+ "isolatedModules": true,
+ "noEmit": true,
+ "jsx": "react-jsx"
+ },
+ "include": ["src"]
+}
diff --git a/examples/with-oauth2-with-supertokens/package.json b/examples/with-oauth2-with-supertokens/package.json
new file mode 100644
index 000000000..ed281d3c3
--- /dev/null
+++ b/examples/with-oauth2-with-supertokens/package.json
@@ -0,0 +1,51 @@
+{
+ "name": "with-oauth2-with-supertokens",
+ "version": "0.0.1",
+ "description": "",
+ "main": "index.js",
+ "scripts": {
+ "start:frontend": "cd frontend && npm run start",
+ "start:frontend-live-demo-app": "cd frontend && npx serve -s build",
+ "start:backend": "cd backend && npm run start",
+ "start:backend-live-demo-app": "cd backend && ./startLiveDemoApp.sh",
+ "start": "npm-run-all --parallel start:frontend start:backend",
+ "start-live-demo-app": "npx npm-run-all --parallel start:frontend-live-demo-app start:backend-live-demo-app"
+ },
+ "keywords": [],
+ "author": "",
+ "license": "ISC",
+ "dependencies": {
+ "@testing-library/jest-dom": "^5.16.5",
+ "@testing-library/react": "^13.4.0",
+ "@testing-library/user-event": "^13.5.0",
+ "@types/jest": "^27.5.2",
+ "@types/node": "^16.11.56",
+ "@types/react": "^18.0.18",
+ "@types/react-dom": "^18.0.6",
+ "axios": "^0.21.0",
+ "cors": "^2.8.5",
+ "express": "^4.18.1",
+ "helmet": "^5.1.0",
+ "morgan": "^1.10.0",
+ "oidc-client-ts": "^3.0.1",
+ "react": "^18.2.0",
+ "react-dom": "^18.2.0",
+ "react-oidc-context": "^3.1.0",
+ "react-router-dom": "^6.2.1",
+ "react-scripts": "5.0.1",
+ "supertokens-auth-react": "github:supertokens/supertokens-auth-react#feat/oauth2/base",
+ "supertokens-node": "github:supertokens/supertokens-node#feat/oauth2/base",
+ "supertokens-web-js": "github:supertokens/supertokens-web-js#feat/oauth2/base",
+ "ts-node-dev": "^2.0.0",
+ "typescript": "^4.8.2",
+ "web-vitals": "^2.1.4"
+ },
+ "devDependencies": {
+ "@types/cors": "^2.8.12",
+ "@types/express": "^4.17.17",
+ "@types/morgan": "^1.9.3",
+ "@types/node": "^16.11.38",
+ "nodemon": "^2.0.16",
+ "npm-run-all": "^4.1.5"
+ }
+}
diff --git a/examples/with-oauth2-without-supertokens/LICENSE.md b/examples/with-oauth2-without-supertokens/LICENSE.md
new file mode 100644
index 000000000..588f27e68
--- /dev/null
+++ b/examples/with-oauth2-without-supertokens/LICENSE.md
@@ -0,0 +1,192 @@
+Copyright (c) 2020, VRAI Labs and/or its affiliates. All rights reserved.
+
+This software is licensed under the Apache License, Version 2.0 (the
+"License") as published by the Apache Software Foundation.
+
+You may not use this software except in compliance with the License. A copy
+of the License is available below the line.
+
+Unless required by applicable law or agreed to in writing, software
+distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+License for the specific language governing permissions and limitations
+under the License.
+
+---
+
+ Apache License
+ Version 2.0, January 2004
+ http://www.apache.org/licenses/
+
+TERMS AND CONDITIONS FOR USE, REPRODUCTION, AND DISTRIBUTION
+
+1. Definitions.
+
+ "License" shall mean the terms and conditions for use, reproduction,
+ and distribution as defined by Sections 1 through 9 of this document.
+
+ "Licensor" shall mean the copyright owner or entity authorized by
+ the copyright owner that is granting the License.
+
+ "Legal Entity" shall mean the union of the acting entity and all
+ other entities that control, are controlled by, or are under common
+ control with that entity. For the purposes of this definition,
+ "control" means (i) the power, direct or indirect, to cause the
+ direction or management of such entity, whether by contract or
+ otherwise, or (ii) ownership of fifty percent (50%) or more of the
+ outstanding shares, or (iii) beneficial ownership of such entity.
+
+ "You" (or "Your") shall mean an individual or Legal Entity
+ exercising permissions granted by this License.
+
+ "Source" form shall mean the preferred form for making modifications,
+ including but not limited to software source code, documentation
+ source, and configuration files.
+
+ "Object" form shall mean any form resulting from mechanical
+ transformation or translation of a Source form, including but
+ not limited to compiled object code, generated documentation,
+ and conversions to other media types.
+
+ "Work" shall mean the work of authorship, whether in Source or
+ Object form, made available under the License, as indicated by a
+ copyright notice that is included in or attached to the work
+ (an example is provided in the Appendix below).
+
+ "Derivative Works" shall mean any work, whether in Source or Object
+ form, that is based on (or derived from) the Work and for which the
+ editorial revisions, annotations, elaborations, or other modifications
+ represent, as a whole, an original work of authorship. For the purposes
+ of this License, Derivative Works shall not include works that remain
+ separable from, or merely link (or bind by name) to the interfaces of,
+ the Work and Derivative Works thereof.
+
+ "Contribution" shall mean any work of authorship, including
+ the original version of the Work and any modifications or additions
+ to that Work or Derivative Works thereof, that is intentionally
+ submitted to Licensor for inclusion in the Work by the copyright owner
+ or by an individual or Legal Entity authorized to submit on behalf of
+ the copyright owner. For the purposes of this definition, "submitted"
+ means any form of electronic, verbal, or written communication sent
+ to the Licensor or its representatives, including but not limited to
+ communication on electronic mailing lists, source code control systems,
+ and issue tracking systems that are managed by, or on behalf of, the
+ Licensor for the purpose of discussing and improving the Work, but
+ excluding communication that is conspicuously marked or otherwise
+ designated in writing by the copyright owner as "Not a Contribution."
+
+ "Contributor" shall mean Licensor and any individual or Legal Entity
+ on behalf of whom a Contribution has been received by Licensor and
+ subsequently incorporated within the Work.
+
+2. Grant of Copyright License. Subject to the terms and conditions of
+ this License, each Contributor hereby grants to You a perpetual,
+ worldwide, non-exclusive, no-charge, royalty-free, irrevocable
+ copyright license to reproduce, prepare Derivative Works of,
+ publicly display, publicly perform, sublicense, and distribute the
+ Work and such Derivative Works in Source or Object form.
+
+3. Grant of Patent License. Subject to the terms and conditions of
+ this License, each Contributor hereby grants to You a perpetual,
+ worldwide, non-exclusive, no-charge, royalty-free, irrevocable
+ (except as stated in this section) patent license to make, have made,
+ use, offer to sell, sell, import, and otherwise transfer the Work,
+ where such license applies only to those patent claims licensable
+ by such Contributor that are necessarily infringed by their
+ Contribution(s) alone or by combination of their Contribution(s)
+ with the Work to which such Contribution(s) was submitted. If You
+ institute patent litigation against any entity (including a
+ cross-claim or counterclaim in a lawsuit) alleging that the Work
+ or a Contribution incorporated within the Work constitutes direct
+ or contributory patent infringement, then any patent licenses
+ granted to You under this License for that Work shall terminate
+ as of the date such litigation is filed.
+
+4. Redistribution. You may reproduce and distribute copies of the
+ Work or Derivative Works thereof in any medium, with or without
+ modifications, and in Source or Object form, provided that You
+ meet the following conditions:
+
+ (a) You must give any other recipients of the Work or
+ Derivative Works a copy of this License; and
+
+ (b) You must cause any modified files to carry prominent notices
+ stating that You changed the files; and
+
+ (c) You must retain, in the Source form of any Derivative Works
+ that You distribute, all copyright, patent, trademark, and
+ attribution notices from the Source form of the Work,
+ excluding those notices that do not pertain to any part of
+ the Derivative Works; and
+
+ (d) If the Work includes a "NOTICE" text file as part of its
+ distribution, then any Derivative Works that You distribute must
+ include a readable copy of the attribution notices contained
+ within such NOTICE file, excluding those notices that do not
+ pertain to any part of the Derivative Works, in at least one
+ of the following places: within a NOTICE text file distributed
+ as part of the Derivative Works; within the Source form or
+ documentation, if provided along with the Derivative Works; or,
+ within a display generated by the Derivative Works, if and
+ wherever such third-party notices normally appear. The contents
+ of the NOTICE file are for informational purposes only and
+ do not modify the License. You may add Your own attribution
+ notices within Derivative Works that You distribute, alongside
+ or as an addendum to the NOTICE text from the Work, provided
+ that such additional attribution notices cannot be construed
+ as modifying the License.
+
+ You may add Your own copyright statement to Your modifications and
+ may provide additional or different license terms and conditions
+ for use, reproduction, or distribution of Your modifications, or
+ for any such Derivative Works as a whole, provided Your use,
+ reproduction, and distribution of the Work otherwise complies with
+ the conditions stated in this License.
+
+5. Submission of Contributions. Unless You explicitly state otherwise,
+ any Contribution intentionally submitted for inclusion in the Work
+ by You to the Licensor shall be under the terms and conditions of
+ this License, without any additional terms or conditions.
+ Notwithstanding the above, nothing herein shall supersede or modify
+ the terms of any separate license agreement you may have executed
+ with Licensor regarding such Contributions.
+
+6. Trademarks. This License does not grant permission to use the trade
+ names, trademarks, service marks, or product names of the Licensor,
+ except as required for reasonable and customary use in describing the
+ origin of the Work and reproducing the content of the NOTICE file.
+
+7. Disclaimer of Warranty. Unless required by applicable law or
+ agreed to in writing, Licensor provides the Work (and each
+ Contributor provides its Contributions) on an "AS IS" BASIS,
+ WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
+ implied, including, without limitation, any warranties or conditions
+ of TITLE, NON-INFRINGEMENT, MERCHANTABILITY, or FITNESS FOR A
+ PARTICULAR PURPOSE. You are solely responsible for determining the
+ appropriateness of using or redistributing the Work and assume any
+ risks associated with Your exercise of permissions under this License.
+
+8. Limitation of Liability. In no event and under no legal theory,
+ whether in tort (including negligence), contract, or otherwise,
+ unless required by applicable law (such as deliberate and grossly
+ negligent acts) or agreed to in writing, shall any Contributor be
+ liable to You for damages, including any direct, indirect, special,
+ incidental, or consequential damages of any character arising as a
+ result of this License or out of the use or inability to use the
+ Work (including but not limited to damages for loss of goodwill,
+ work stoppage, computer failure or malfunction, or any and all
+ other commercial damages or losses), even if such Contributor
+ has been advised of the possibility of such damages.
+
+9. Accepting Warranty or Additional Liability. While redistributing
+ the Work or Derivative Works thereof, You may choose to offer,
+ and charge a fee for, acceptance of support, warranty, indemnity,
+ or other liability obligations and/or rights consistent with this
+ License. However, in accepting such obligations, You may act only
+ on Your own behalf and on Your sole responsibility, not on behalf
+ of any other Contributor, and only if You agree to indemnify,
+ defend, and hold each Contributor harmless for any liability
+ incurred by, or claims asserted against, such Contributor by reason
+ of your accepting any such warranty or additional liability.
+
+END OF TERMS AND CONDITIONS
diff --git a/examples/with-oauth2-without-supertokens/README.md b/examples/with-oauth2-without-supertokens/README.md
new file mode 100644
index 000000000..1507029d7
--- /dev/null
+++ b/examples/with-oauth2-without-supertokens/README.md
@@ -0,0 +1,57 @@
+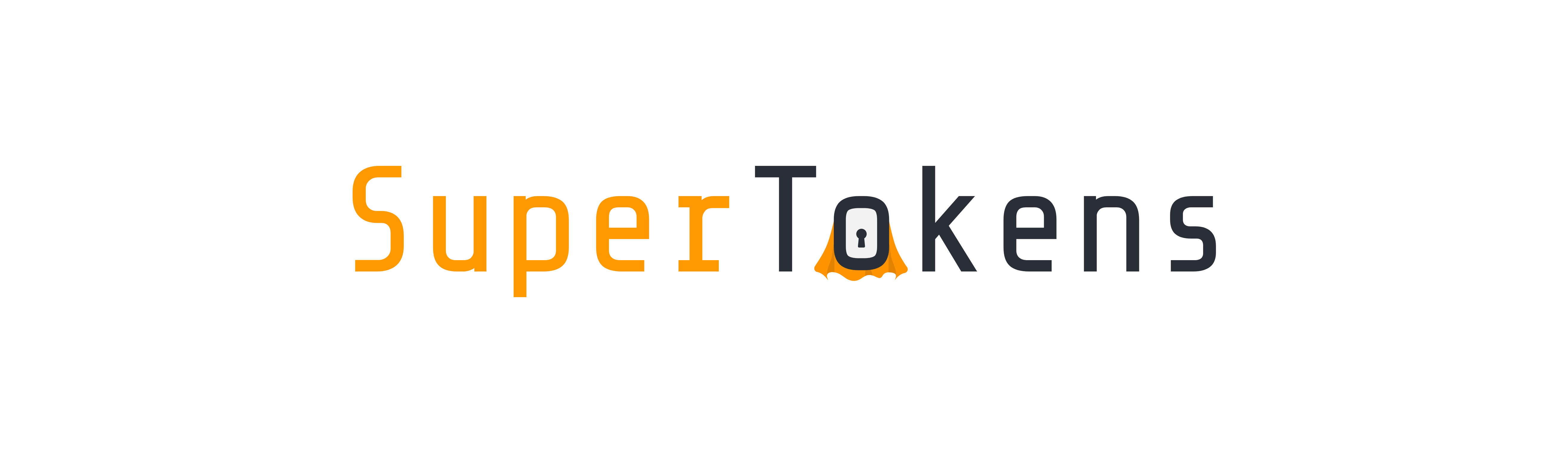
+
+# Example React App using a Generic OAuth2 Library and SuperTokens as OAuth2 Provider
+
+This examples app demonstrates how to use SuperTokens as OAuth2 Provider in a React App using a generic OAuth2 library such as [react-oidc-context](https://github.com/authts/react-oidc-context).
+
+## Project setup
+
+Clone the repo, enter the directory, and use `npm` to install the project dependencies:
+
+```bash
+git clone https://github.com/supertokens/supertokens-auth-react
+cd supertokens-auth-react/examples/with-oauth2-without-supertokens
+npm install
+```
+
+## 1. Create an OAuth2 Client
+
+```bash
+curl -X POST http://localhost:3567/recipe/oauth/clients \
+ -H "Content-Type: application/json" \
+ -d '{
+ "scope": "offline_access openid email",
+ "redirectUris": ["http://localhost:3000"],
+ "grantTypes": ["authorization_code", "refresh_token"],
+ "responseTypes": ["code", "id_token"],
+ "tokenEndpointAuthMethod": "none"
+ }'
+```
+
+Note down the `client_id` from the response.
+
+## 2. Run the st-oauth2-authorization-server
+
+1. Open a new terminal window and navigate to `supertokens-auth-react/examples/
+st-oauth2-authorization-server`
+2. Read the README.md to setup `st-oauth2-authorization-server ` and run it using `npm start`
+
+## 3. Update config
+
+Open the `App.tsx` file and update `clientId` and `authServerUrl` values as per step 1 and step 2.
+
+## 4. Run the demo app
+
+This compiles and serves the React app.
+
+```bash
+npm run start
+```
+
+## Author
+
+Created with :heart: by the folks at supertokens.com.
+
+## License
+
+This project is licensed under the Apache 2.0 license.
diff --git a/examples/with-oauth2-without-supertokens/package.json b/examples/with-oauth2-without-supertokens/package.json
new file mode 100644
index 000000000..adb7336c5
--- /dev/null
+++ b/examples/with-oauth2-without-supertokens/package.json
@@ -0,0 +1,50 @@
+{
+ "name": "with-oauth2-without-supertokens",
+ "version": "0.1.0",
+ "private": true,
+ "dependencies": {
+ "@testing-library/jest-dom": "^5.16.5",
+ "@testing-library/react": "^13.4.0",
+ "@testing-library/user-event": "^13.5.0",
+ "@types/jest": "^27.5.2",
+ "@types/node": "^16.11.56",
+ "@types/react": "^18.0.18",
+ "@types/react-dom": "^18.0.6",
+ "axios": "^0.21.0",
+ "helmet": "^5.1.0",
+ "oidc-client-ts": "^3.0.1",
+ "react": "^18.2.0",
+ "react-dom": "^18.2.0",
+ "react-oauth2-code-pkce": "^1.20.1",
+ "react-oidc-context": "^3.1.0",
+ "react-router-dom": "^6.2.1",
+ "react-scripts": "5.0.1",
+ "ts-node-dev": "^2.0.0",
+ "typescript": "^4.8.2",
+ "web-vitals": "^2.1.4"
+ },
+ "scripts": {
+ "start": "BROWSER=none react-scripts start",
+ "build": "react-scripts build",
+ "test": "react-scripts test",
+ "eject": "react-scripts eject"
+ },
+ "eslintConfig": {
+ "extends": [
+ "react-app",
+ "react-app/jest"
+ ]
+ },
+ "browserslist": {
+ "production": [
+ ">0.2%",
+ "not dead",
+ "not op_mini all"
+ ],
+ "development": [
+ "last 1 chrome version",
+ "last 1 firefox version",
+ "last 1 safari version"
+ ]
+ }
+}
diff --git a/examples/with-oauth2-without-supertokens/public/favicon.ico b/examples/with-oauth2-without-supertokens/public/favicon.ico
new file mode 100644
index 000000000..a11777cc4
Binary files /dev/null and b/examples/with-oauth2-without-supertokens/public/favicon.ico differ
diff --git a/examples/with-oauth2-without-supertokens/public/index.html b/examples/with-oauth2-without-supertokens/public/index.html
new file mode 100644
index 000000000..6f1f7cb51
--- /dev/null
+++ b/examples/with-oauth2-without-supertokens/public/index.html
@@ -0,0 +1,40 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+ React App
+
+
+
+
+
+
diff --git a/examples/with-oauth2-without-supertokens/public/manifest.json b/examples/with-oauth2-without-supertokens/public/manifest.json
new file mode 100644
index 000000000..f01493ff0
--- /dev/null
+++ b/examples/with-oauth2-without-supertokens/public/manifest.json
@@ -0,0 +1,25 @@
+{
+ "short_name": "React App",
+ "name": "Create React App Sample",
+ "icons": [
+ {
+ "src": "favicon.ico",
+ "sizes": "64x64 32x32 24x24 16x16",
+ "type": "image/x-icon"
+ },
+ {
+ "src": "logo192.png",
+ "type": "image/png",
+ "sizes": "192x192"
+ },
+ {
+ "src": "logo512.png",
+ "type": "image/png",
+ "sizes": "512x512"
+ }
+ ],
+ "start_url": ".",
+ "display": "standalone",
+ "theme_color": "#000000",
+ "background_color": "#ffffff"
+}
diff --git a/examples/with-oauth2-without-supertokens/public/robots.txt b/examples/with-oauth2-without-supertokens/public/robots.txt
new file mode 100644
index 000000000..e9e57dc4d
--- /dev/null
+++ b/examples/with-oauth2-without-supertokens/public/robots.txt
@@ -0,0 +1,3 @@
+# https://www.robotstxt.org/robotstxt.html
+User-agent: *
+Disallow:
diff --git a/examples/with-oauth2-without-supertokens/src/App.css b/examples/with-oauth2-without-supertokens/src/App.css
new file mode 100644
index 000000000..9886f003d
--- /dev/null
+++ b/examples/with-oauth2-without-supertokens/src/App.css
@@ -0,0 +1,26 @@
+.App {
+ display: flex;
+ flex-direction: column;
+ width: 100vw;
+ height: 100vh;
+ font-family: Rubik;
+}
+
+button {
+ padding-left: 13px;
+ padding-right: 13px;
+ padding-top: 8px;
+ padding-bottom: 8px;
+ background-color: black;
+ border-radius: 10px;
+ cursor: pointer;
+ color: white;
+ font-weight: bold;
+ font-size: 17px;
+}
+
+.center {
+ display: flex;
+ flex-direction: column;
+ align-items: center;
+}
diff --git a/examples/with-oauth2-without-supertokens/src/App.tsx b/examples/with-oauth2-without-supertokens/src/App.tsx
new file mode 100644
index 000000000..d390207b0
--- /dev/null
+++ b/examples/with-oauth2-without-supertokens/src/App.tsx
@@ -0,0 +1,48 @@
+import { AuthProvider, AuthProviderProps, useAuth } from "react-oidc-context";
+import "./App.css";
+
+const authServerUrl = process.env.REACT_APP_AUTH_SERVER_API_URL || "http://localhost.com:3006";
+const clientId = "REPLACE_WITH_YOUR_CLIENT_ID";
+
+const oidcConfig: AuthProviderProps = {
+ client_id: clientId,
+ authority: `${authServerUrl}/auth`,
+ response_type: "code",
+ redirect_uri: "http://localhost:3000",
+ scope: "openid offline_access email",
+ revokeTokensOnSignout: true,
+ onSigninCallback: async () => {
+ // Clears the response code and other params from the callback url
+ window.history.replaceState({}, document.title, window.location.pathname);
+ },
+};
+
+function AuthPage() {
+ const { signinRedirect, signoutSilent, user } = useAuth();
+
+ return (
+