diff --git a/components/Navbar.tsx b/components/Navbar.tsx
index f00eb14..7c8d706 100644
--- a/components/Navbar.tsx
+++ b/components/Navbar.tsx
@@ -1,31 +1,86 @@
import Image from 'next/image';
import Link from 'next/link';
-import React from 'react';
-import WordmarkLogo from '../public/acm-logo-wordmark-extended.png';
+import React, { useState } from 'react';
+
+import WordmarkLogo from '../public/acm_wordmark_chapter.svg';
function Navbar(): JSX.Element {
+ // set states
+ const [menuActive, setMenuActive] = useState(false);
+
+ // switches mobile menu state
+ const menuActivate = () => {
+ setMenuActive(menuActive ? false : true);
+ };
+
return (
-
);
}
diff --git a/next-env.d.ts b/next-env.d.ts
index 9bc3dd4..4f11a03 100644
--- a/next-env.d.ts
+++ b/next-env.d.ts
@@ -1,5 +1,4 @@
///
-///
///
// NOTE: This file should not be edited
diff --git a/public/acm-logo-wordmark-extended.png b/public/acm-logo-wordmark-extended.png
deleted file mode 100644
index f5c32e3..0000000
Binary files a/public/acm-logo-wordmark-extended.png and /dev/null differ
diff --git a/public/acm_wordmark_chapter.svg b/public/acm_wordmark_chapter.svg
new file mode 100644
index 0000000..606a24c
--- /dev/null
+++ b/public/acm_wordmark_chapter.svg
@@ -0,0 +1,367 @@
+
+
+
+
diff --git a/styles/globals.css b/styles/globals.css
index 8d987b0..ba62ea6 100644
--- a/styles/globals.css
+++ b/styles/globals.css
@@ -4,6 +4,7 @@ html,
body {
padding: 0;
margin: 0;
+ margin-top: 70px;
}
* {
@@ -35,7 +36,7 @@ body {
.assignee-image {
border-radius: 50%;
- overflow: hidden;
+ overflow: hidden;
width: 30px;
height: 30px;
}
@@ -52,12 +53,182 @@ body {
}
/* overriding the WestwoodCSS default */
-.navbar-link a {
- padding-left: 1em !important;
+#navbar {
+ background: white;
+ box-shadow: 0 0 4px #e2e2e2;
+ color: #333;
+ font-family: 'Poppins', 'Helvetica Neue', 'Helvetica', sans-serif;
+ font-weight: 600;
+ height: 72px;
+ left: 0;
+ margin: 0;
+ padding: 0;
+ position: fixed;
+ text-transform: lowercase;
+ top: 0;
+ width: 100%;
+ z-index: 10;
+}
+
+#navbar #nav-container {
+ align-items: center;
+ display: flex;
+ justify-content: space-between;
+ padding: 0 20px;
}
-.navbar-link > button {
- margin-left: 1em;
+#navbar #nav-container #nav-title {
+ z-index: 12;
+ margin-top: 5px;
+}
+
+#navbar #nav-container #nav-title #acm-logo {
+ height: 60px;
+ margin-top: 05px;
+ width: 180px;
+}
+
+#navbar #nav-container #hamburger {
+ align-items: center;
+ background-color: transparent;
+ border: none;
+ border-radius: 50%;
+ cursor: pointer;
+ display: none;
+ flex-direction: column;
+ height: 50px;
+ justify-content: center;
+ margin: 0 20px;
+ overflow: hidden;
+ position: relative;
+ width: 50px;
+ z-index: 12;
+ box-shadow: none;
+}
+
+#navbar #nav-container #hamburger .bar {
+ background-color: #595959;
+ border-radius: 04px;
+ height: 03px;
+ margin: 2px 0;
+ position: relative;
+ transition: all 0.5s ease-in-out;
+ width: 20px;
+}
+
+#navbar #nav-container #hamburger.active #bar-one {
+ transform: rotate(45deg) translate(5px, 4px);
+}
+
+#navbar #nav-container #hamburger.active #bar-two {
+ transform: translateX(-50px);
+}
+
+#navbar #nav-container #hamburger.active #bar-three {
+ transform: rotate(-45deg) translate(5px, -5px);
+}
+
+#navbar #nav-container #hamburger:hover .bar {
+ background-color: #1e6cff;
+}
+
+#navbar #nav-container .nav-items {
+ align-items: center;
+ display: flex;
+ list-style: none;
+ margin: 0;
+ padding: 0;
+}
+
+#navbar #nav-container .nav-items li {
+ line-height: 40px;
+ padding: 0px;
+}
+
+#navbar #nav-container .nav-items li button {
+ background-color: transparent;
+ outline: none;
+ color: inherit;
+ font-family: inherit;
+ font-size: 0.95em;
+ font-weight: 600;
+ text-transform: lowercase;
+ transition: 0.2s;
+ margin: 0;
+ box-shadow: none;
+ border-radius: 15px;
+}
+
+#navbar #nav-container .nav-items li button:hover {
+ color: #1e6cff;
+ cursor: pointer;
+}
+
+#navbar #nav-container .nav-items li .github {
+ border: 0.7mm solid;
+ color: #1e6cff;
+ text-transform: none;
+ margin: 0 15px;
+}
+
+#navbar #nav-container .nav-items li .github:hover {
+ background-color: #1e6cff;
+ color: white;
+ transition: none;
+}
+
+@media (max-width: 1160px) {
+ #navbar #nav-container #hamburger {
+ display: flex;
+ }
+
+ #navbar #menu-modal {
+ background: rgba(255, 255, 255, 0.95);
+ height: 100vh;
+ left: 50vw;
+ overflow-y: scroll;
+ position: absolute;
+ top: 50vh;
+ transform: translate(-50%, -150%);
+ transition: transform 0.35s cubic-bezier(0.05, 1.04, 0.72, 0.98);
+ width: 100vw;
+ z-index: 11;
+ }
+
+ #navbar #menu-modal .nav-items {
+ display: none;
+ font-size: 1.8em;
+ font-weight: 600;
+ left: 50vw;
+ margin: 10px 0;
+ position: absolute;
+ top: 50vh;
+ transform: translate(-50%, -50%);
+ }
+
+ #navbar #menu-modal .nav-items .button {
+ padding: 0 20px;
+ white-space: nowrap;
+ border: #2b7489;
+ }
+ #navbar #menu-modal .nav-items .button :focus {
+ outline: none;
+ }
+
+ #navbar #menu-modal .nav-items li {
+ margin: 5px 0;
+ }
+
+ #navbar #menu-modal .nav-items.active {
+ align-items: center;
+ display: flex;
+ flex-direction: column;
+ }
+
+ #navbar #menu-modal.active {
+ transform: translate(-50%, -50%);
+ transition: transform 0.35s cubic-bezier(0.05, 1.04, 0.72, 0.98);
+ }
}
.dev-language-badge {
@@ -72,27 +243,27 @@ body {
}
.dev-language-badge.lang-js {
- background-color: #EFDF6A;
+ background-color: #efdf6a;
}
.dev-language-badge.lang-ts {
- background-color: #2B7489;
+ background-color: #2b7489;
}
.dev-language-badge.lang-scss {
- background-color: #C6538C;
+ background-color: #c6538c;
}
.dev-language-badge.lang-go {
- background-color: #4AABD5;
+ background-color: #4aabd5;
}
.dev-language-badge.lang-html {
- background-color: #D35A31;
+ background-color: #d35a31;
}
.dev-language-badge.lang-rust {
- background-color: #DEA584;
+ background-color: #dea584;
}
.vertically-aligned-row {
@@ -118,7 +289,7 @@ body {
}
.gfi-title > h3 {
- margin: 0
+ margin: 0;
}
.gfi-image {
@@ -127,12 +298,12 @@ body {
}
.gfi-button {
- background-color: #1E6CFF;
+ background-color: #1e6cff;
color: white;
}
.gfi-button-displayed {
- color: #1E6CFF;
+ color: #1e6cff;
background-color: rgb(244, 244, 244);
}
@@ -149,4 +320,4 @@ dl * {
font-weight: bold;
color: black;
font-size: 36px;
-}
\ No newline at end of file
+}
diff --git a/test/fixtures/gfiProjects.json b/test/fixtures/gfiProjects.json
index b907406..61e2f85 100644
--- a/test/fixtures/gfiProjects.json
+++ b/test/fixtures/gfiProjects.json
@@ -1 +1 @@
-[{"issues":[{"url":"https://api.github.com/repos/uclaacm/opensource/issues/118","repository_url":"https://api.github.com/repos/uclaacm/opensource","labels_url":"https://api.github.com/repos/uclaacm/opensource/issues/118/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/opensource/issues/118/comments","events_url":"https://api.github.com/repos/uclaacm/opensource/issues/118/events","html_url":"https://github.com/uclaacm/opensource/issues/118","id":1250243723,"node_id":"I_kwDOFbnmps5KhTSL","number":118,"title":"Working on ucla-opensource page!","user":{"login":"matthewcn56","id":65370631,"node_id":"MDQ6VXNlcjY1MzcwNjMx","avatar_url":"https://avatars.githubusercontent.com/u/65370631?v=4","gravatar_id":"","url":"https://api.github.com/users/matthewcn56","html_url":"https://github.com/matthewcn56","followers_url":"https://api.github.com/users/matthewcn56/followers","following_url":"https://api.github.com/users/matthewcn56/following{/other_user}","gists_url":"https://api.github.com/users/matthewcn56/gists{/gist_id}","starred_url":"https://api.github.com/users/matthewcn56/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/matthewcn56/subscriptions","organizations_url":"https://api.github.com/users/matthewcn56/orgs","repos_url":"https://api.github.com/users/matthewcn56/repos","events_url":"https://api.github.com/users/matthewcn56/events{/privacy}","received_events_url":"https://api.github.com/users/matthewcn56/received_events","type":"User","site_admin":false},"labels":[{"id":2973665525,"node_id":"MDU6TGFiZWwyOTczNjY1NTI1","url":"https://api.github.com/repos/uclaacm/opensource/labels/enhancement","name":"enhancement","color":"a2eeef","default":true,"description":"New feature or request"},{"id":2973665526,"node_id":"MDU6TGFiZWwyOTczNjY1NTI2","url":"https://api.github.com/repos/uclaacm/opensource/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":{"login":"JCamyre","id":68767176,"node_id":"MDQ6VXNlcjY4NzY3MTc2","avatar_url":"https://avatars.githubusercontent.com/u/68767176?v=4","gravatar_id":"","url":"https://api.github.com/users/JCamyre","html_url":"https://github.com/JCamyre","followers_url":"https://api.github.com/users/JCamyre/followers","following_url":"https://api.github.com/users/JCamyre/following{/other_user}","gists_url":"https://api.github.com/users/JCamyre/gists{/gist_id}","starred_url":"https://api.github.com/users/JCamyre/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/JCamyre/subscriptions","organizations_url":"https://api.github.com/users/JCamyre/orgs","repos_url":"https://api.github.com/users/JCamyre/repos","events_url":"https://api.github.com/users/JCamyre/events{/privacy}","received_events_url":"https://api.github.com/users/JCamyre/received_events","type":"User","site_admin":false},"assignees":[{"login":"JCamyre","id":68767176,"node_id":"MDQ6VXNlcjY4NzY3MTc2","avatar_url":"https://avatars.githubusercontent.com/u/68767176?v=4","gravatar_id":"","url":"https://api.github.com/users/JCamyre","html_url":"https://github.com/JCamyre","followers_url":"https://api.github.com/users/JCamyre/followers","following_url":"https://api.github.com/users/JCamyre/following{/other_user}","gists_url":"https://api.github.com/users/JCamyre/gists{/gist_id}","starred_url":"https://api.github.com/users/JCamyre/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/JCamyre/subscriptions","organizations_url":"https://api.github.com/users/JCamyre/orgs","repos_url":"https://api.github.com/users/JCamyre/repos","events_url":"https://api.github.com/users/JCamyre/events{/privacy}","received_events_url":"https://api.github.com/users/JCamyre/received_events","type":"User","site_admin":false}],"milestone":null,"comments":0,"created_at":"2022-05-27T01:34:55Z","updated_at":"2022-05-27T01:34:55Z","closed_at":null,"author_association":"MEMBER","active_lock_reason":null,"body":"Short and sweet: Add a `ucla-opensource` page, with the tag `ucla` up on the top of the navbar, and make a small blurb about what the page will be about (it should show all projects with the `ucla-opensource` topic tag), and we can make it similar to the actual projects page! be sure to say that this page will be a work in progress","reactions":{"url":"https://api.github.com/repos/uclaacm/opensource/issues/118/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/opensource/issues/118/timeline","performed_via_github_app":null,"state_reason":null,"score":1}],"project":{"name":"opensource","description":"opensource @ acm, work-in-progress","link":"https://opensource.uclaacm.com","repo":"https://github.com/uclaacm/opensource","lang":"TypeScript","topics":["ucla-opensource"],"image":"/logo.png","alt":"ACM @ UCLA's Logo"},"repoURL":"https://api.github.com/repos/uclaacm/opensource"}]
\ No newline at end of file
+[{"issues":[{"url":"https://api.github.com/repos/uclaacm/dev-pathways-next/issues/2","repository_url":"https://api.github.com/repos/uclaacm/dev-pathways-next","labels_url":"https://api.github.com/repos/uclaacm/dev-pathways-next/issues/2/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/dev-pathways-next/issues/2/comments","events_url":"https://api.github.com/repos/uclaacm/dev-pathways-next/issues/2/events","html_url":"https://github.com/uclaacm/dev-pathways-next/issues/2","id":2149725393,"node_id":"I_kwDOKrpL7s6AIjTR","number":2,"title":"Landing page","user":{"login":"utk7arsh","id":66383982,"node_id":"MDQ6VXNlcjY2MzgzOTgy","avatar_url":"https://avatars.githubusercontent.com/u/66383982?v=4","gravatar_id":"","url":"https://api.github.com/users/utk7arsh","html_url":"https://github.com/utk7arsh","followers_url":"https://api.github.com/users/utk7arsh/followers","following_url":"https://api.github.com/users/utk7arsh/following{/other_user}","gists_url":"https://api.github.com/users/utk7arsh/gists{/gist_id}","starred_url":"https://api.github.com/users/utk7arsh/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/utk7arsh/subscriptions","organizations_url":"https://api.github.com/users/utk7arsh/orgs","repos_url":"https://api.github.com/users/utk7arsh/repos","events_url":"https://api.github.com/users/utk7arsh/events{/privacy}","received_events_url":"https://api.github.com/users/utk7arsh/received_events","type":"User","site_admin":false},"labels":[{"id":6191690412,"node_id":"LA_kwDOKrpL7s8AAAABcQ2yrA","url":"https://api.github.com/repos/uclaacm/dev-pathways-next/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":null,"assignees":[],"milestone":null,"comments":0,"created_at":"2024-02-22T18:46:16Z","updated_at":"2024-04-22T23:55:20Z","closed_at":null,"author_association":"NONE","active_lock_reason":null,"body":"## Aim\r\n\r\nWelcome to Dev Pathways. These are some UI bugs on the landing page which could be fixed by css changes, state handling, etc. This issue details the tasks we need to complete in order to reach this milestone.\r\n\r\n## Tasks\r\n\r\n- [x] Horizontal overflow on every page - On the landing page, the crucial resources button overlows to the right which can totally be avoided by a minor css. I feel like this issue is in every page on the web right now\r\n\r\n- [x] If you click the button \"Generate\" at the bottom of the page. and come back to the home page, you will find it disappeared. Attached image for reference\r\n\r\n\r\n\r\n\r\n- [x] The images aren't loading when we switched from React to nextjs. This needs to be looked into and at certain times, the issue can be the image compatiblity with next. For example the social media images aren't generated\r\n\r\n","reactions":{"url":"https://api.github.com/repos/uclaacm/dev-pathways-next/issues/2/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/dev-pathways-next/issues/2/timeline","performed_via_github_app":null,"state_reason":null,"score":1}],"project":{"name":"dev-pathways-next","description":"","repo":"https://github.com/uclaacm/dev-pathways-next","lang":"JavaScript","topics":[],"image":"/logo.png","alt":"ACM @ UCLA's Logo"},"repoURL":"https://api.github.com/repos/uclaacm/dev-pathways-next"},{"issues":[{"url":"https://api.github.com/repos/uclaacm/website/issues/671","repository_url":"https://api.github.com/repos/uclaacm/website","labels_url":"https://api.github.com/repos/uclaacm/website/issues/671/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/website/issues/671/comments","events_url":"https://api.github.com/repos/uclaacm/website/issues/671/events","html_url":"https://github.com/uclaacm/website/issues/671","id":2034521839,"node_id":"I_kwDOBjCw5s55RFbv","number":671,"title":"Modularize GM Page","user":{"login":"ArshMalik02","id":51771221,"node_id":"MDQ6VXNlcjUxNzcxMjIx","avatar_url":"https://avatars.githubusercontent.com/u/51771221?v=4","gravatar_id":"","url":"https://api.github.com/users/ArshMalik02","html_url":"https://github.com/ArshMalik02","followers_url":"https://api.github.com/users/ArshMalik02/followers","following_url":"https://api.github.com/users/ArshMalik02/following{/other_user}","gists_url":"https://api.github.com/users/ArshMalik02/gists{/gist_id}","starred_url":"https://api.github.com/users/ArshMalik02/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/ArshMalik02/subscriptions","organizations_url":"https://api.github.com/users/ArshMalik02/orgs","repos_url":"https://api.github.com/users/ArshMalik02/repos","events_url":"https://api.github.com/users/ArshMalik02/events{/privacy}","received_events_url":"https://api.github.com/users/ArshMalik02/received_events","type":"User","site_admin":false},"labels":[{"id":695018202,"node_id":"MDU6TGFiZWw2OTUwMTgyMDI=","url":"https://api.github.com/repos/uclaacm/website/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"byte-sized change that should be easy to make; typically has a fleshed out ticket!"}],"state":"open","locked":false,"assignee":{"login":"g1oom","id":51779517,"node_id":"MDQ6VXNlcjUxNzc5NTE3","avatar_url":"https://avatars.githubusercontent.com/u/51779517?v=4","gravatar_id":"","url":"https://api.github.com/users/g1oom","html_url":"https://github.com/g1oom","followers_url":"https://api.github.com/users/g1oom/followers","following_url":"https://api.github.com/users/g1oom/following{/other_user}","gists_url":"https://api.github.com/users/g1oom/gists{/gist_id}","starred_url":"https://api.github.com/users/g1oom/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/g1oom/subscriptions","organizations_url":"https://api.github.com/users/g1oom/orgs","repos_url":"https://api.github.com/users/g1oom/repos","events_url":"https://api.github.com/users/g1oom/events{/privacy}","received_events_url":"https://api.github.com/users/g1oom/received_events","type":"User","site_admin":false},"assignees":[{"login":"g1oom","id":51779517,"node_id":"MDQ6VXNlcjUxNzc5NTE3","avatar_url":"https://avatars.githubusercontent.com/u/51779517?v=4","gravatar_id":"","url":"https://api.github.com/users/g1oom","html_url":"https://github.com/g1oom","followers_url":"https://api.github.com/users/g1oom/followers","following_url":"https://api.github.com/users/g1oom/following{/other_user}","gists_url":"https://api.github.com/users/g1oom/gists{/gist_id}","starred_url":"https://api.github.com/users/g1oom/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/g1oom/subscriptions","organizations_url":"https://api.github.com/users/g1oom/orgs","repos_url":"https://api.github.com/users/g1oom/repos","events_url":"https://api.github.com/users/g1oom/events{/privacy}","received_events_url":"https://api.github.com/users/g1oom/received_events","type":"User","site_admin":false}],"milestone":null,"comments":0,"created_at":"2023-12-10T20:28:31Z","updated_at":"2024-02-24T04:41:26Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"## Current State\r\n\r\nWe need to create a new page every quarter for our general meeting. This leads to code bloat on our frontend, as most of the code is the same. The changes that need to be made are the date, time, venue, and the slide deck. \r\n\r\n## What do we aim for?\r\n\r\nModularize the page to eliminate the need to create new pages every quarter (suggested by @jainsujay02, #670). \r\n\r\nOne approach could be to parameterize the changes we make every quarter and fetch the required details from a common source such as a sheet that the organizers of the GM could update. This approach would allow for a streamlined method of creating the page and eliminate the need to request relevant information from the required sources.\r\n\r\nThere could be other ways to implement this idea, and I would encourage open discussion to arrive on the best approach.","reactions":{"url":"https://api.github.com/repos/uclaacm/website/issues/671/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/website/issues/671/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/website/issues/492","repository_url":"https://api.github.com/repos/uclaacm/website","labels_url":"https://api.github.com/repos/uclaacm/website/issues/492/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/website/issues/492/comments","events_url":"https://api.github.com/repos/uclaacm/website/issues/492/events","html_url":"https://github.com/uclaacm/website/issues/492","id":1216216403,"node_id":"I_kwDOBjCw5s5Iff1T","number":492,"title":"Updates to About Page","user":{"login":"jainsujay02","id":78530348,"node_id":"MDQ6VXNlcjc4NTMwMzQ4","avatar_url":"https://avatars.githubusercontent.com/u/78530348?v=4","gravatar_id":"","url":"https://api.github.com/users/jainsujay02","html_url":"https://github.com/jainsujay02","followers_url":"https://api.github.com/users/jainsujay02/followers","following_url":"https://api.github.com/users/jainsujay02/following{/other_user}","gists_url":"https://api.github.com/users/jainsujay02/gists{/gist_id}","starred_url":"https://api.github.com/users/jainsujay02/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/jainsujay02/subscriptions","organizations_url":"https://api.github.com/users/jainsujay02/orgs","repos_url":"https://api.github.com/users/jainsujay02/repos","events_url":"https://api.github.com/users/jainsujay02/events{/privacy}","received_events_url":"https://api.github.com/users/jainsujay02/received_events","type":"User","site_admin":false},"labels":[{"id":695018202,"node_id":"MDU6TGFiZWw2OTUwMTgyMDI=","url":"https://api.github.com/repos/uclaacm/website/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"byte-sized change that should be easy to make; typically has a fleshed out ticket!"}],"state":"open","locked":false,"assignee":{"login":"jainsujay02","id":78530348,"node_id":"MDQ6VXNlcjc4NTMwMzQ4","avatar_url":"https://avatars.githubusercontent.com/u/78530348?v=4","gravatar_id":"","url":"https://api.github.com/users/jainsujay02","html_url":"https://github.com/jainsujay02","followers_url":"https://api.github.com/users/jainsujay02/followers","following_url":"https://api.github.com/users/jainsujay02/following{/other_user}","gists_url":"https://api.github.com/users/jainsujay02/gists{/gist_id}","starred_url":"https://api.github.com/users/jainsujay02/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/jainsujay02/subscriptions","organizations_url":"https://api.github.com/users/jainsujay02/orgs","repos_url":"https://api.github.com/users/jainsujay02/repos","events_url":"https://api.github.com/users/jainsujay02/events{/privacy}","received_events_url":"https://api.github.com/users/jainsujay02/received_events","type":"User","site_admin":false},"assignees":[{"login":"jainsujay02","id":78530348,"node_id":"MDQ6VXNlcjc4NTMwMzQ4","avatar_url":"https://avatars.githubusercontent.com/u/78530348?v=4","gravatar_id":"","url":"https://api.github.com/users/jainsujay02","html_url":"https://github.com/jainsujay02","followers_url":"https://api.github.com/users/jainsujay02/followers","following_url":"https://api.github.com/users/jainsujay02/following{/other_user}","gists_url":"https://api.github.com/users/jainsujay02/gists{/gist_id}","starred_url":"https://api.github.com/users/jainsujay02/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/jainsujay02/subscriptions","organizations_url":"https://api.github.com/users/jainsujay02/orgs","repos_url":"https://api.github.com/users/jainsujay02/repos","events_url":"https://api.github.com/users/jainsujay02/events{/privacy}","received_events_url":"https://api.github.com/users/jainsujay02/received_events","type":"User","site_admin":false}],"milestone":null,"comments":0,"created_at":"2022-04-26T16:41:22Z","updated_at":"2023-09-03T05:32:41Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"Changes requested to `https://acm.cs.ucla.edu/about`\r\n\r\nAs an Ops Intern, I often interacted with general members who had no clue what the board did. In the interest of transparency and recognizing the amazing work of the people on board, I'd like to make the following changes. \r\n\r\n- [x] Increase font size for the body text \r\n- [x] Add a `What's ACM board?` This section should provide an overview of internal and external in 2-3 brief sentences and some pictures of board meetings to go with it. \r\n- [x] Add a `What are ACM Initiatives?` after what's board. A sentence explaining that these are independent teams within the board who work on super cool ideas/projects followed by links to all of our initiatives - Dev Team, JEDI, Impact, and Rustaceans! We should also have a collage of their logos next to links/descriptions! \r\n- [x] Add a quick explanation for `Leadership.`\r\n- [x] Add a line on our event calends and link to it for `How do I get involved? `\r\n- [ ] #551\r\n- [ ] Once the history page is ready, we should add a `How did ACM came to be?` or something along these lines and link to the history page with a sentence or two for context. \r\n- [ ] Once the officer's page is ready, we should add a` Who's who` section after the leadership and link it to the page! ","reactions":{"url":"https://api.github.com/repos/uclaacm/website/issues/492/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/website/issues/492/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/website/issues/225","repository_url":"https://api.github.com/repos/uclaacm/website","labels_url":"https://api.github.com/repos/uclaacm/website/issues/225/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/website/issues/225/comments","events_url":"https://api.github.com/repos/uclaacm/website/issues/225/events","html_url":"https://github.com/uclaacm/website/issues/225","id":958339143,"node_id":"MDU6SXNzdWU5NTgzMzkxNDM=","number":225,"title":"Clean up accessibility tree","user":{"login":"mattxwang","id":14893287,"node_id":"MDQ6VXNlcjE0ODkzMjg3","avatar_url":"https://avatars.githubusercontent.com/u/14893287?v=4","gravatar_id":"","url":"https://api.github.com/users/mattxwang","html_url":"https://github.com/mattxwang","followers_url":"https://api.github.com/users/mattxwang/followers","following_url":"https://api.github.com/users/mattxwang/following{/other_user}","gists_url":"https://api.github.com/users/mattxwang/gists{/gist_id}","starred_url":"https://api.github.com/users/mattxwang/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/mattxwang/subscriptions","organizations_url":"https://api.github.com/users/mattxwang/orgs","repos_url":"https://api.github.com/users/mattxwang/repos","events_url":"https://api.github.com/users/mattxwang/events{/privacy}","received_events_url":"https://api.github.com/users/mattxwang/received_events","type":"User","site_admin":false},"labels":[{"id":695018201,"node_id":"MDU6TGFiZWw2OTUwMTgyMDE=","url":"https://api.github.com/repos/uclaacm/website/labels/help%20wanted","name":"help wanted","color":"33aa3f","default":true,"description":null},{"id":695018202,"node_id":"MDU6TGFiZWw2OTUwMTgyMDI=","url":"https://api.github.com/repos/uclaacm/website/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"byte-sized change that should be easy to make; typically has a fleshed out ticket!"},{"id":3199883558,"node_id":"MDU6TGFiZWwzMTk5ODgzNTU4","url":"https://api.github.com/repos/uclaacm/website/labels/long%20term","name":"long term","color":"AB5F85","default":false,"description":"a problem that we'd have to work on over a long chunk of time"},{"id":3322443259,"node_id":"MDU6TGFiZWwzMzIyNDQzMjU5","url":"https://api.github.com/repos/uclaacm/website/labels/low-priority","name":"low-priority","color":"C2E0C6","default":false,"description":"not a huge deal if we don't get done, but great extra work to pick up"}],"state":"open","locked":false,"assignee":null,"assignees":[],"milestone":null,"comments":3,"created_at":"2021-08-02T17:07:32Z","updated_at":"2022-11-21T23:28:34Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"Like many of my ideas for this website, this one comes from poking around Hack's (see uclaacm/hack.uclaacm.com#229) - our accessibility tree is rather disgusting.\r\n\r\n
\r\n\r\nIn particular - the nav is poorly marked, many alt tags are not helpful, and the page is lacking a parseable structure.\r\n\r\nLet's make this an \"Epic\" of sorts - we want to run manual audits and resolve them on:\r\n\r\n- [ ] home page\r\n- [ ] about page\r\n- [ ] committees page (this one will be *hard*)\r\n- [ ] events page\r\n- [ ] sponsors page \r\n\r\nFurthermore, for our new pages, let's build them in gracefully:\r\n\r\n- [ ] internship page, #222 \r\n- [ ] events page / events archive page #179 \r\n- [ ] impact page \r\n- [ ] jedi page\r\n- [ ] dev team page\r\n- [ ] \"friends\" page \r\n\r\nDebating if this is a walkthrough I'll do with the dev team!","reactions":{"url":"https://api.github.com/repos/uclaacm/website/issues/225/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/website/issues/225/timeline","performed_via_github_app":null,"state_reason":null,"score":1}],"project":{"name":"website","description":"The official (award-winning) website for ACM at UCLA, the largest tech community on campus!","link":"https://acm.cs.ucla.edu","repo":"https://github.com/uclaacm/website","lang":"JavaScript","topics":["react","ucla-opensource"],"image":"/logo.png","alt":"ACM @ UCLA's Logo"},"repoURL":"https://api.github.com/repos/uclaacm/website"},{"issues":[{"url":"https://api.github.com/repos/uclaacm/committee-website-template-cms/issues/40","repository_url":"https://api.github.com/repos/uclaacm/committee-website-template-cms","labels_url":"https://api.github.com/repos/uclaacm/committee-website-template-cms/issues/40/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/committee-website-template-cms/issues/40/comments","events_url":"https://api.github.com/repos/uclaacm/committee-website-template-cms/issues/40/events","html_url":"https://github.com/uclaacm/committee-website-template-cms/issues/40","id":1909762012,"node_id":"I_kwDOHzqfRM5x1Kfc","number":40,"title":"navbar committee wordmark styling","user":{"login":"ArshMalik02","id":51771221,"node_id":"MDQ6VXNlcjUxNzcxMjIx","avatar_url":"https://avatars.githubusercontent.com/u/51771221?v=4","gravatar_id":"","url":"https://api.github.com/users/ArshMalik02","html_url":"https://github.com/ArshMalik02","followers_url":"https://api.github.com/users/ArshMalik02/followers","following_url":"https://api.github.com/users/ArshMalik02/following{/other_user}","gists_url":"https://api.github.com/users/ArshMalik02/gists{/gist_id}","starred_url":"https://api.github.com/users/ArshMalik02/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/ArshMalik02/subscriptions","organizations_url":"https://api.github.com/users/ArshMalik02/orgs","repos_url":"https://api.github.com/users/ArshMalik02/repos","events_url":"https://api.github.com/users/ArshMalik02/events{/privacy}","received_events_url":"https://api.github.com/users/ArshMalik02/received_events","type":"User","site_admin":false},"labels":[{"id":4418395172,"node_id":"LA_kwDOHzqfRM8AAAABB1tcJA","url":"https://api.github.com/repos/uclaacm/committee-website-template-cms/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"},{"id":6084862285,"node_id":"LA_kwDOHzqfRM8AAAABaq-hTQ","url":"https://api.github.com/repos/uclaacm/committee-website-template-cms/labels/low-priority","name":"low-priority","color":"B3654C","default":false,"description":""}],"state":"open","locked":false,"assignee":{"login":"ChiweiChen","id":43721631,"node_id":"MDQ6VXNlcjQzNzIxNjMx","avatar_url":"https://avatars.githubusercontent.com/u/43721631?v=4","gravatar_id":"","url":"https://api.github.com/users/ChiweiChen","html_url":"https://github.com/ChiweiChen","followers_url":"https://api.github.com/users/ChiweiChen/followers","following_url":"https://api.github.com/users/ChiweiChen/following{/other_user}","gists_url":"https://api.github.com/users/ChiweiChen/gists{/gist_id}","starred_url":"https://api.github.com/users/ChiweiChen/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/ChiweiChen/subscriptions","organizations_url":"https://api.github.com/users/ChiweiChen/orgs","repos_url":"https://api.github.com/users/ChiweiChen/repos","events_url":"https://api.github.com/users/ChiweiChen/events{/privacy}","received_events_url":"https://api.github.com/users/ChiweiChen/received_events","type":"User","site_admin":false},"assignees":[{"login":"ChiweiChen","id":43721631,"node_id":"MDQ6VXNlcjQzNzIxNjMx","avatar_url":"https://avatars.githubusercontent.com/u/43721631?v=4","gravatar_id":"","url":"https://api.github.com/users/ChiweiChen","html_url":"https://github.com/ChiweiChen","followers_url":"https://api.github.com/users/ChiweiChen/followers","following_url":"https://api.github.com/users/ChiweiChen/following{/other_user}","gists_url":"https://api.github.com/users/ChiweiChen/gists{/gist_id}","starred_url":"https://api.github.com/users/ChiweiChen/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/ChiweiChen/subscriptions","organizations_url":"https://api.github.com/users/ChiweiChen/orgs","repos_url":"https://api.github.com/users/ChiweiChen/repos","events_url":"https://api.github.com/users/ChiweiChen/events{/privacy}","received_events_url":"https://api.github.com/users/ChiweiChen/received_events","type":"User","site_admin":false}],"milestone":null,"comments":1,"created_at":"2023-09-23T08:05:20Z","updated_at":"2024-01-05T06:43:28Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"The Committee wordmark logo appears to be a bit squished. The styling needs to be modified. Here's a screenshot for reference:\r\n\r\n\r\n(Courtesy: Jordan Lin)\r\n","reactions":{"url":"https://api.github.com/repos/uclaacm/committee-website-template-cms/issues/40/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/committee-website-template-cms/issues/40/timeline","performed_via_github_app":null,"state_reason":null,"score":1}],"project":{"name":"committee-website-template-cms","description":"This template enables any ACM Committee to put up their website in minutes!","repo":"https://github.com/uclaacm/committee-website-template-cms","lang":"JavaScript","topics":[],"image":"/logo.png","alt":"ACM @ UCLA's Logo"},"repoURL":"https://api.github.com/repos/uclaacm/committee-website-template-cms"},{"issues":[{"url":"https://api.github.com/repos/uclaacm/opensource/issues/152","repository_url":"https://api.github.com/repos/uclaacm/opensource","labels_url":"https://api.github.com/repos/uclaacm/opensource/issues/152/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/opensource/issues/152/comments","events_url":"https://api.github.com/repos/uclaacm/opensource/issues/152/events","html_url":"https://github.com/uclaacm/opensource/issues/152","id":1897748894,"node_id":"I_kwDOFbnmps5xHVme","number":152,"title":"Update Navigation ","user":{"login":"jainsujay02","id":78530348,"node_id":"MDQ6VXNlcjc4NTMwMzQ4","avatar_url":"https://avatars.githubusercontent.com/u/78530348?v=4","gravatar_id":"","url":"https://api.github.com/users/jainsujay02","html_url":"https://github.com/jainsujay02","followers_url":"https://api.github.com/users/jainsujay02/followers","following_url":"https://api.github.com/users/jainsujay02/following{/other_user}","gists_url":"https://api.github.com/users/jainsujay02/gists{/gist_id}","starred_url":"https://api.github.com/users/jainsujay02/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/jainsujay02/subscriptions","organizations_url":"https://api.github.com/users/jainsujay02/orgs","repos_url":"https://api.github.com/users/jainsujay02/repos","events_url":"https://api.github.com/users/jainsujay02/events{/privacy}","received_events_url":"https://api.github.com/users/jainsujay02/received_events","type":"User","site_admin":false},"labels":[{"id":2973665526,"node_id":"MDU6TGFiZWwyOTczNjY1NTI2","url":"https://api.github.com/repos/uclaacm/opensource/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":{"login":"ansh-saini","id":32511936,"node_id":"MDQ6VXNlcjMyNTExOTM2","avatar_url":"https://avatars.githubusercontent.com/u/32511936?v=4","gravatar_id":"","url":"https://api.github.com/users/ansh-saini","html_url":"https://github.com/ansh-saini","followers_url":"https://api.github.com/users/ansh-saini/followers","following_url":"https://api.github.com/users/ansh-saini/following{/other_user}","gists_url":"https://api.github.com/users/ansh-saini/gists{/gist_id}","starred_url":"https://api.github.com/users/ansh-saini/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/ansh-saini/subscriptions","organizations_url":"https://api.github.com/users/ansh-saini/orgs","repos_url":"https://api.github.com/users/ansh-saini/repos","events_url":"https://api.github.com/users/ansh-saini/events{/privacy}","received_events_url":"https://api.github.com/users/ansh-saini/received_events","type":"User","site_admin":false},"assignees":[{"login":"ansh-saini","id":32511936,"node_id":"MDQ6VXNlcjMyNTExOTM2","avatar_url":"https://avatars.githubusercontent.com/u/32511936?v=4","gravatar_id":"","url":"https://api.github.com/users/ansh-saini","html_url":"https://github.com/ansh-saini","followers_url":"https://api.github.com/users/ansh-saini/followers","following_url":"https://api.github.com/users/ansh-saini/following{/other_user}","gists_url":"https://api.github.com/users/ansh-saini/gists{/gist_id}","starred_url":"https://api.github.com/users/ansh-saini/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/ansh-saini/subscriptions","organizations_url":"https://api.github.com/users/ansh-saini/orgs","repos_url":"https://api.github.com/users/ansh-saini/repos","events_url":"https://api.github.com/users/ansh-saini/events{/privacy}","received_events_url":"https://api.github.com/users/ansh-saini/received_events","type":"User","site_admin":false}],"milestone":null,"comments":5,"created_at":"2023-09-15T05:48:57Z","updated_at":"2023-10-10T00:05:11Z","closed_at":null,"author_association":"NONE","active_lock_reason":null,"body":"The open-source site was originally intended to be a stand-alone website. However, we would now like to keep it linked to ACM's main website. This naturally causes a weird navigation experience with no way to navigate back to the main ACM website from the open-source website. \r\n\r\nTo address think, I can think of two approaches: \r\n\r\n1. We add a back to the main site button on the home page (which is a little cringe) but acceptable for our purposes. \r\n2. We modify the navigation bar so that it includes links to our main website. \r\n\r\nIdeally, you'd experiment with both of these and see which feels more intuitive from a user's perspective. ","reactions":{"url":"https://api.github.com/repos/uclaacm/opensource/issues/152/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/opensource/issues/152/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/opensource/issues/118","repository_url":"https://api.github.com/repos/uclaacm/opensource","labels_url":"https://api.github.com/repos/uclaacm/opensource/issues/118/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/opensource/issues/118/comments","events_url":"https://api.github.com/repos/uclaacm/opensource/issues/118/events","html_url":"https://github.com/uclaacm/opensource/issues/118","id":1250243723,"node_id":"I_kwDOFbnmps5KhTSL","number":118,"title":"Working on ucla-opensource page!","user":{"login":"matthewcn56","id":65370631,"node_id":"MDQ6VXNlcjY1MzcwNjMx","avatar_url":"https://avatars.githubusercontent.com/u/65370631?v=4","gravatar_id":"","url":"https://api.github.com/users/matthewcn56","html_url":"https://github.com/matthewcn56","followers_url":"https://api.github.com/users/matthewcn56/followers","following_url":"https://api.github.com/users/matthewcn56/following{/other_user}","gists_url":"https://api.github.com/users/matthewcn56/gists{/gist_id}","starred_url":"https://api.github.com/users/matthewcn56/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/matthewcn56/subscriptions","organizations_url":"https://api.github.com/users/matthewcn56/orgs","repos_url":"https://api.github.com/users/matthewcn56/repos","events_url":"https://api.github.com/users/matthewcn56/events{/privacy}","received_events_url":"https://api.github.com/users/matthewcn56/received_events","type":"User","site_admin":false},"labels":[{"id":2973665525,"node_id":"MDU6TGFiZWwyOTczNjY1NTI1","url":"https://api.github.com/repos/uclaacm/opensource/labels/enhancement","name":"enhancement","color":"a2eeef","default":true,"description":"New feature or request"},{"id":2973665526,"node_id":"MDU6TGFiZWwyOTczNjY1NTI2","url":"https://api.github.com/repos/uclaacm/opensource/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":{"login":"JCamyre","id":68767176,"node_id":"MDQ6VXNlcjY4NzY3MTc2","avatar_url":"https://avatars.githubusercontent.com/u/68767176?v=4","gravatar_id":"","url":"https://api.github.com/users/JCamyre","html_url":"https://github.com/JCamyre","followers_url":"https://api.github.com/users/JCamyre/followers","following_url":"https://api.github.com/users/JCamyre/following{/other_user}","gists_url":"https://api.github.com/users/JCamyre/gists{/gist_id}","starred_url":"https://api.github.com/users/JCamyre/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/JCamyre/subscriptions","organizations_url":"https://api.github.com/users/JCamyre/orgs","repos_url":"https://api.github.com/users/JCamyre/repos","events_url":"https://api.github.com/users/JCamyre/events{/privacy}","received_events_url":"https://api.github.com/users/JCamyre/received_events","type":"User","site_admin":false},"assignees":[{"login":"JCamyre","id":68767176,"node_id":"MDQ6VXNlcjY4NzY3MTc2","avatar_url":"https://avatars.githubusercontent.com/u/68767176?v=4","gravatar_id":"","url":"https://api.github.com/users/JCamyre","html_url":"https://github.com/JCamyre","followers_url":"https://api.github.com/users/JCamyre/followers","following_url":"https://api.github.com/users/JCamyre/following{/other_user}","gists_url":"https://api.github.com/users/JCamyre/gists{/gist_id}","starred_url":"https://api.github.com/users/JCamyre/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/JCamyre/subscriptions","organizations_url":"https://api.github.com/users/JCamyre/orgs","repos_url":"https://api.github.com/users/JCamyre/repos","events_url":"https://api.github.com/users/JCamyre/events{/privacy}","received_events_url":"https://api.github.com/users/JCamyre/received_events","type":"User","site_admin":false}],"milestone":null,"comments":0,"created_at":"2022-05-27T01:34:55Z","updated_at":"2022-05-27T01:34:55Z","closed_at":null,"author_association":"MEMBER","active_lock_reason":null,"body":"Short and sweet: Add a `ucla-opensource` page, with the tag `ucla` up on the top of the navbar, and make a small blurb about what the page will be about (it should show all projects with the `ucla-opensource` topic tag), and we can make it similar to the actual projects page! be sure to say that this page will be a work in progress","reactions":{"url":"https://api.github.com/repos/uclaacm/opensource/issues/118/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/opensource/issues/118/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/opensource/issues/112","repository_url":"https://api.github.com/repos/uclaacm/opensource","labels_url":"https://api.github.com/repos/uclaacm/opensource/issues/112/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/opensource/issues/112/comments","events_url":"https://api.github.com/repos/uclaacm/opensource/issues/112/events","html_url":"https://github.com/uclaacm/opensource/issues/112","id":1222267599,"node_id":"I_kwDOFbnmps5I2lLP","number":112,"title":"Remove dependabot from \"what we've been doing recently...\"","user":{"login":"advaithg","id":67539191,"node_id":"MDQ6VXNlcjY3NTM5MTkx","avatar_url":"https://avatars.githubusercontent.com/u/67539191?v=4","gravatar_id":"","url":"https://api.github.com/users/advaithg","html_url":"https://github.com/advaithg","followers_url":"https://api.github.com/users/advaithg/followers","following_url":"https://api.github.com/users/advaithg/following{/other_user}","gists_url":"https://api.github.com/users/advaithg/gists{/gist_id}","starred_url":"https://api.github.com/users/advaithg/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/advaithg/subscriptions","organizations_url":"https://api.github.com/users/advaithg/orgs","repos_url":"https://api.github.com/users/advaithg/repos","events_url":"https://api.github.com/users/advaithg/events{/privacy}","received_events_url":"https://api.github.com/users/advaithg/received_events","type":"User","site_admin":false},"labels":[{"id":2973665526,"node_id":"MDU6TGFiZWwyOTczNjY1NTI2","url":"https://api.github.com/repos/uclaacm/opensource/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":null,"assignees":[],"milestone":null,"comments":1,"created_at":"2022-05-01T19:56:25Z","updated_at":"2023-09-15T05:41:28Z","closed_at":null,"author_association":"NONE","active_lock_reason":null,"body":"Something I've noticed is that dependabot tends to take over our recent activity a lot of the time, and I think we'd rather highlight people's work than the PRs made by the bot!\r\n\r\nOne way I can think of doing this is after getting `recentEvents` from the octokit API in `index.tsx`, iterate through and remove events with dependabot as the author (you'd have to look into what the API returns to do this). Feel free to implement it however you'd like though - especially if you can think of a more efficient way to do it!","reactions":{"url":"https://api.github.com/repos/uclaacm/opensource/issues/112/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/opensource/issues/112/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/opensource/issues/75","repository_url":"https://api.github.com/repos/uclaacm/opensource","labels_url":"https://api.github.com/repos/uclaacm/opensource/issues/75/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/opensource/issues/75/comments","events_url":"https://api.github.com/repos/uclaacm/opensource/issues/75/events","html_url":"https://github.com/uclaacm/opensource/issues/75","id":1096522219,"node_id":"I_kwDOFbnmps5BW5nr","number":75,"title":"add (composable) filters for projects","user":{"login":"mattxwang","id":14893287,"node_id":"MDQ6VXNlcjE0ODkzMjg3","avatar_url":"https://avatars.githubusercontent.com/u/14893287?v=4","gravatar_id":"","url":"https://api.github.com/users/mattxwang","html_url":"https://github.com/mattxwang","followers_url":"https://api.github.com/users/mattxwang/followers","following_url":"https://api.github.com/users/mattxwang/following{/other_user}","gists_url":"https://api.github.com/users/mattxwang/gists{/gist_id}","starred_url":"https://api.github.com/users/mattxwang/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/mattxwang/subscriptions","organizations_url":"https://api.github.com/users/mattxwang/orgs","repos_url":"https://api.github.com/users/mattxwang/repos","events_url":"https://api.github.com/users/mattxwang/events{/privacy}","received_events_url":"https://api.github.com/users/mattxwang/received_events","type":"User","site_admin":false},"labels":[{"id":2973665526,"node_id":"MDU6TGFiZWwyOTczNjY1NTI2","url":"https://api.github.com/repos/uclaacm/opensource/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":null,"assignees":[],"milestone":null,"comments":0,"created_at":"2022-01-07T17:32:45Z","updated_at":"2023-09-15T05:41:49Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"With #57 on the horizon, we are going to have *a lot* of projects. It might get hard to sift through as the end user, which is where a filtering system would help!\r\n\r\nThe simplest filter to implement is probably off of language. I would suggest starting there; to implement it, I would ask that you:\r\n\r\n1. Create a UI element (it's okay if the styling isn't perfect)\r\n2. Devise some mechanism to perform the filtering - noting that it could get more complicated once we add more filters\r\n3. Add some sort of state to manage this\r\n4. *Optional*: save the result of the filter to the URL, so you can \"send\" someone a filter with a link\r\n\r\nI would suggest you either branch off of #57 or wait for it to resolve, since it changes the language structure.","reactions":{"url":"https://api.github.com/repos/uclaacm/opensource/issues/75/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/opensource/issues/75/timeline","performed_via_github_app":null,"state_reason":null,"score":1}],"project":{"name":"opensource","description":"opensource @ acm, work-in-progress","link":"https://opensource.uclaacm.com","repo":"https://github.com/uclaacm/opensource","lang":"TypeScript","topics":["ucla-opensource"],"image":"/logo.png","alt":"ACM @ UCLA's Logo"},"repoURL":"https://api.github.com/repos/uclaacm/opensource"},{"issues":[{"url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/1043","repository_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend","labels_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/1043/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/1043/comments","events_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/1043/events","html_url":"https://github.com/uclaacm/TeachLAFrontend/issues/1043","id":1714924397,"node_id":"I_kwDOCePRrs5mN6tt","number":1043,"title":"Refactor: clean up sorting by key","user":{"login":"TomBinford","id":28466971,"node_id":"MDQ6VXNlcjI4NDY2OTcx","avatar_url":"https://avatars.githubusercontent.com/u/28466971?v=4","gravatar_id":"","url":"https://api.github.com/users/TomBinford","html_url":"https://github.com/TomBinford","followers_url":"https://api.github.com/users/TomBinford/followers","following_url":"https://api.github.com/users/TomBinford/following{/other_user}","gists_url":"https://api.github.com/users/TomBinford/gists{/gist_id}","starred_url":"https://api.github.com/users/TomBinford/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/TomBinford/subscriptions","organizations_url":"https://api.github.com/users/TomBinford/orgs","repos_url":"https://api.github.com/users/TomBinford/repos","events_url":"https://api.github.com/users/TomBinford/events{/privacy}","received_events_url":"https://api.github.com/users/TomBinford/received_events","type":"User","site_admin":false},"labels":[{"id":1194843223,"node_id":"MDU6TGFiZWwxMTk0ODQzMjIz","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/refactor","name":"refactor","color":"cfd3d7","default":false,"description":"Refactoring & cleanup"},{"id":1194843226,"node_id":"MDU6TGFiZWwxMTk0ODQzMjI2","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/good%20first%20issue","name":"good first issue","color":"EF82C8","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":null,"assignees":[],"milestone":null,"comments":1,"created_at":"2023-05-18T03:28:17Z","updated_at":"2023-11-02T17:30:03Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"https://github.com/uclaacm/TeachLAFrontend/blob/17be7ace2957237bf4121c62344b47ef061fad62/src/components/Sketches/index.js#L84-L85\r\nhttps://github.com/uclaacm/TeachLAFrontend/blob/17be7ace2957237bf4121c62344b47ef061fad62/src/components/Sketches/index.js#L101-L107\r\nWe can rewrite this more functionally (without even a `newList` variable) using [spread syntax](https://stackoverflow.com/a/48057162) and [localeCompare](https://stackoverflow.com/a/2167619)","reactions":{"url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/1043/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/1043/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/962","repository_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend","labels_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/962/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/962/comments","events_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/962/events","html_url":"https://github.com/uclaacm/TeachLAFrontend/issues/962","id":1450583851,"node_id":"I_kwDOCePRrs5Wdicr","number":962,"title":"Change JoinClass request","user":{"login":"timothycpoon","id":8311368,"node_id":"MDQ6VXNlcjgzMTEzNjg=","avatar_url":"https://avatars.githubusercontent.com/u/8311368?v=4","gravatar_id":"","url":"https://api.github.com/users/timothycpoon","html_url":"https://github.com/timothycpoon","followers_url":"https://api.github.com/users/timothycpoon/followers","following_url":"https://api.github.com/users/timothycpoon/following{/other_user}","gists_url":"https://api.github.com/users/timothycpoon/gists{/gist_id}","starred_url":"https://api.github.com/users/timothycpoon/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/timothycpoon/subscriptions","organizations_url":"https://api.github.com/users/timothycpoon/orgs","repos_url":"https://api.github.com/users/timothycpoon/repos","events_url":"https://api.github.com/users/timothycpoon/events{/privacy}","received_events_url":"https://api.github.com/users/timothycpoon/received_events","type":"User","site_admin":false},"labels":[{"id":1194843222,"node_id":"MDU6TGFiZWwxMTk0ODQzMjIy","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/bug","name":"bug","color":"d73a4a","default":true,"description":"Something isn't working"},{"id":1194843226,"node_id":"MDU6TGFiZWwxMTk0ODQzMjI2","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/good%20first%20issue","name":"good first issue","color":"EF82C8","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":{"login":"zhangallison","id":57781635,"node_id":"MDQ6VXNlcjU3NzgxNjM1","avatar_url":"https://avatars.githubusercontent.com/u/57781635?v=4","gravatar_id":"","url":"https://api.github.com/users/zhangallison","html_url":"https://github.com/zhangallison","followers_url":"https://api.github.com/users/zhangallison/followers","following_url":"https://api.github.com/users/zhangallison/following{/other_user}","gists_url":"https://api.github.com/users/zhangallison/gists{/gist_id}","starred_url":"https://api.github.com/users/zhangallison/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/zhangallison/subscriptions","organizations_url":"https://api.github.com/users/zhangallison/orgs","repos_url":"https://api.github.com/users/zhangallison/repos","events_url":"https://api.github.com/users/zhangallison/events{/privacy}","received_events_url":"https://api.github.com/users/zhangallison/received_events","type":"User","site_admin":false},"assignees":[{"login":"zhangallison","id":57781635,"node_id":"MDQ6VXNlcjU3NzgxNjM1","avatar_url":"https://avatars.githubusercontent.com/u/57781635?v=4","gravatar_id":"","url":"https://api.github.com/users/zhangallison","html_url":"https://github.com/zhangallison","followers_url":"https://api.github.com/users/zhangallison/followers","following_url":"https://api.github.com/users/zhangallison/following{/other_user}","gists_url":"https://api.github.com/users/zhangallison/gists{/gist_id}","starred_url":"https://api.github.com/users/zhangallison/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/zhangallison/subscriptions","organizations_url":"https://api.github.com/users/zhangallison/orgs","repos_url":"https://api.github.com/users/zhangallison/repos","events_url":"https://api.github.com/users/zhangallison/events{/privacy}","received_events_url":"https://api.github.com/users/zhangallison/received_events","type":"User","site_admin":false}],"milestone":null,"comments":0,"created_at":"2022-11-16T00:12:24Z","updated_at":"2023-01-31T02:17:34Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"Fix JoinClassModal providing the WID, but labelling it the CID. This should be a one line change!\r\n\r\nhttps://github.com/uclaacm/TeachLAFrontend/blob/master/src/components/Classes/components/JoinClassModal.js","reactions":{"url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/962/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/962/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/726","repository_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend","labels_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/726/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/726/comments","events_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/726/events","html_url":"https://github.com/uclaacm/TeachLAFrontend/issues/726","id":1052539482,"node_id":"I_kwDOCePRrs4-vHpa","number":726,"title":"Refactor ViewOnly.js into a functional component w/ hooks","user":{"login":"timothycpoon","id":8311368,"node_id":"MDQ6VXNlcjgzMTEzNjg=","avatar_url":"https://avatars.githubusercontent.com/u/8311368?v=4","gravatar_id":"","url":"https://api.github.com/users/timothycpoon","html_url":"https://github.com/timothycpoon","followers_url":"https://api.github.com/users/timothycpoon/followers","following_url":"https://api.github.com/users/timothycpoon/following{/other_user}","gists_url":"https://api.github.com/users/timothycpoon/gists{/gist_id}","starred_url":"https://api.github.com/users/timothycpoon/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/timothycpoon/subscriptions","organizations_url":"https://api.github.com/users/timothycpoon/orgs","repos_url":"https://api.github.com/users/timothycpoon/repos","events_url":"https://api.github.com/users/timothycpoon/events{/privacy}","received_events_url":"https://api.github.com/users/timothycpoon/received_events","type":"User","site_admin":false},"labels":[{"id":1194843223,"node_id":"MDU6TGFiZWwxMTk0ODQzMjIz","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/refactor","name":"refactor","color":"cfd3d7","default":false,"description":"Refactoring & cleanup"},{"id":1194843226,"node_id":"MDU6TGFiZWwxMTk0ODQzMjI2","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/good%20first%20issue","name":"good first issue","color":"EF82C8","default":true,"description":"Good for newcomers"},{"id":3523090144,"node_id":"LA_kwDOCePRrs7R_hbg","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/taken","name":"taken","color":"9619EF","default":false,"description":"this ticket is taken"}],"state":"open","locked":false,"assignee":{"login":"BilalG1","id":1523448,"node_id":"MDQ6VXNlcjE1MjM0NDg=","avatar_url":"https://avatars.githubusercontent.com/u/1523448?v=4","gravatar_id":"","url":"https://api.github.com/users/BilalG1","html_url":"https://github.com/BilalG1","followers_url":"https://api.github.com/users/BilalG1/followers","following_url":"https://api.github.com/users/BilalG1/following{/other_user}","gists_url":"https://api.github.com/users/BilalG1/gists{/gist_id}","starred_url":"https://api.github.com/users/BilalG1/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/BilalG1/subscriptions","organizations_url":"https://api.github.com/users/BilalG1/orgs","repos_url":"https://api.github.com/users/BilalG1/repos","events_url":"https://api.github.com/users/BilalG1/events{/privacy}","received_events_url":"https://api.github.com/users/BilalG1/received_events","type":"User","site_admin":false},"assignees":[{"login":"BilalG1","id":1523448,"node_id":"MDQ6VXNlcjE1MjM0NDg=","avatar_url":"https://avatars.githubusercontent.com/u/1523448?v=4","gravatar_id":"","url":"https://api.github.com/users/BilalG1","html_url":"https://github.com/BilalG1","followers_url":"https://api.github.com/users/BilalG1/followers","following_url":"https://api.github.com/users/BilalG1/following{/other_user}","gists_url":"https://api.github.com/users/BilalG1/gists{/gist_id}","starred_url":"https://api.github.com/users/BilalG1/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/BilalG1/subscriptions","organizations_url":"https://api.github.com/users/BilalG1/orgs","repos_url":"https://api.github.com/users/BilalG1/repos","events_url":"https://api.github.com/users/BilalG1/events{/privacy}","received_events_url":"https://api.github.com/users/BilalG1/received_events","type":"User","site_admin":false}],"milestone":null,"comments":0,"created_at":"2021-11-13T04:21:37Z","updated_at":"2021-11-28T04:53:23Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"https://github.com/uclaacm/TeachLAFrontend/blob/master/src/components/ViewOnly.js","reactions":{"url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/726/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/726/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/724","repository_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend","labels_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/724/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/724/comments","events_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/724/events","html_url":"https://github.com/uclaacm/TeachLAFrontend/issues/724","id":1052538879,"node_id":"I_kwDOCePRrs4-vHf_","number":724,"title":"Refactor LoginForm.js to a functional component w/ hooks","user":{"login":"timothycpoon","id":8311368,"node_id":"MDQ6VXNlcjgzMTEzNjg=","avatar_url":"https://avatars.githubusercontent.com/u/8311368?v=4","gravatar_id":"","url":"https://api.github.com/users/timothycpoon","html_url":"https://github.com/timothycpoon","followers_url":"https://api.github.com/users/timothycpoon/followers","following_url":"https://api.github.com/users/timothycpoon/following{/other_user}","gists_url":"https://api.github.com/users/timothycpoon/gists{/gist_id}","starred_url":"https://api.github.com/users/timothycpoon/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/timothycpoon/subscriptions","organizations_url":"https://api.github.com/users/timothycpoon/orgs","repos_url":"https://api.github.com/users/timothycpoon/repos","events_url":"https://api.github.com/users/timothycpoon/events{/privacy}","received_events_url":"https://api.github.com/users/timothycpoon/received_events","type":"User","site_admin":false},"labels":[{"id":1194843223,"node_id":"MDU6TGFiZWwxMTk0ODQzMjIz","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/refactor","name":"refactor","color":"cfd3d7","default":false,"description":"Refactoring & cleanup"},{"id":1194843226,"node_id":"MDU6TGFiZWwxMTk0ODQzMjI2","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/good%20first%20issue","name":"good first issue","color":"EF82C8","default":true,"description":"Good for newcomers"},{"id":3548395051,"node_id":"LA_kwDOCePRrs7TgDYr","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/reserved","name":"reserved","color":"47BC30","default":false,"description":""}],"state":"open","locked":false,"assignee":{"login":"ArushRam","id":60648382,"node_id":"MDQ6VXNlcjYwNjQ4Mzgy","avatar_url":"https://avatars.githubusercontent.com/u/60648382?v=4","gravatar_id":"","url":"https://api.github.com/users/ArushRam","html_url":"https://github.com/ArushRam","followers_url":"https://api.github.com/users/ArushRam/followers","following_url":"https://api.github.com/users/ArushRam/following{/other_user}","gists_url":"https://api.github.com/users/ArushRam/gists{/gist_id}","starred_url":"https://api.github.com/users/ArushRam/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/ArushRam/subscriptions","organizations_url":"https://api.github.com/users/ArushRam/orgs","repos_url":"https://api.github.com/users/ArushRam/repos","events_url":"https://api.github.com/users/ArushRam/events{/privacy}","received_events_url":"https://api.github.com/users/ArushRam/received_events","type":"User","site_admin":false},"assignees":[{"login":"ArushRam","id":60648382,"node_id":"MDQ6VXNlcjYwNjQ4Mzgy","avatar_url":"https://avatars.githubusercontent.com/u/60648382?v=4","gravatar_id":"","url":"https://api.github.com/users/ArushRam","html_url":"https://github.com/ArushRam","followers_url":"https://api.github.com/users/ArushRam/followers","following_url":"https://api.github.com/users/ArushRam/following{/other_user}","gists_url":"https://api.github.com/users/ArushRam/gists{/gist_id}","starred_url":"https://api.github.com/users/ArushRam/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/ArushRam/subscriptions","organizations_url":"https://api.github.com/users/ArushRam/orgs","repos_url":"https://api.github.com/users/ArushRam/repos","events_url":"https://api.github.com/users/ArushRam/events{/privacy}","received_events_url":"https://api.github.com/users/ArushRam/received_events","type":"User","site_admin":false}],"milestone":null,"comments":5,"created_at":"2021-11-13T04:17:25Z","updated_at":"2021-11-14T15:40:21Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"https://github.com/uclaacm/TeachLAFrontend/blob/master/src/components/Login/LoginForm.js","reactions":{"url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/724/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/724/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/670","repository_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend","labels_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/670/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/670/comments","events_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/670/events","html_url":"https://github.com/uclaacm/TeachLAFrontend/issues/670","id":1036774679,"node_id":"I_kwDOCePRrs49y-0X","number":670,"title":"Refactor Output.js into a functional component w/ hooks","user":{"login":"timothycpoon","id":8311368,"node_id":"MDQ6VXNlcjgzMTEzNjg=","avatar_url":"https://avatars.githubusercontent.com/u/8311368?v=4","gravatar_id":"","url":"https://api.github.com/users/timothycpoon","html_url":"https://github.com/timothycpoon","followers_url":"https://api.github.com/users/timothycpoon/followers","following_url":"https://api.github.com/users/timothycpoon/following{/other_user}","gists_url":"https://api.github.com/users/timothycpoon/gists{/gist_id}","starred_url":"https://api.github.com/users/timothycpoon/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/timothycpoon/subscriptions","organizations_url":"https://api.github.com/users/timothycpoon/orgs","repos_url":"https://api.github.com/users/timothycpoon/repos","events_url":"https://api.github.com/users/timothycpoon/events{/privacy}","received_events_url":"https://api.github.com/users/timothycpoon/received_events","type":"User","site_admin":false},"labels":[{"id":1194843223,"node_id":"MDU6TGFiZWwxMTk0ODQzMjIz","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/refactor","name":"refactor","color":"cfd3d7","default":false,"description":"Refactoring & cleanup"},{"id":1194843226,"node_id":"MDU6TGFiZWwxMTk0ODQzMjI2","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/good%20first%20issue","name":"good first issue","color":"EF82C8","default":true,"description":"Good for newcomers"},{"id":3523090144,"node_id":"LA_kwDOCePRrs7R_hbg","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/taken","name":"taken","color":"9619EF","default":false,"description":"this ticket is taken"}],"state":"open","locked":false,"assignee":null,"assignees":[],"milestone":null,"comments":0,"created_at":"2021-10-26T21:55:15Z","updated_at":"2021-11-06T06:01:06Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"https://github.com/uclaacm/TeachLAFrontend/blob/master/src/components/Output/Output.js","reactions":{"url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/670/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/670/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/489","repository_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend","labels_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/489/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/489/comments","events_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/489/events","html_url":"https://github.com/uclaacm/TeachLAFrontend/issues/489","id":867199776,"node_id":"MDU6SXNzdWU4NjcxOTk3NzY=","number":489,"title":"Refactor common/Radio.js to a functional component w/ hooks","user":{"login":"timothycpoon","id":8311368,"node_id":"MDQ6VXNlcjgzMTEzNjg=","avatar_url":"https://avatars.githubusercontent.com/u/8311368?v=4","gravatar_id":"","url":"https://api.github.com/users/timothycpoon","html_url":"https://github.com/timothycpoon","followers_url":"https://api.github.com/users/timothycpoon/followers","following_url":"https://api.github.com/users/timothycpoon/following{/other_user}","gists_url":"https://api.github.com/users/timothycpoon/gists{/gist_id}","starred_url":"https://api.github.com/users/timothycpoon/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/timothycpoon/subscriptions","organizations_url":"https://api.github.com/users/timothycpoon/orgs","repos_url":"https://api.github.com/users/timothycpoon/repos","events_url":"https://api.github.com/users/timothycpoon/events{/privacy}","received_events_url":"https://api.github.com/users/timothycpoon/received_events","type":"User","site_admin":false},"labels":[{"id":1194843223,"node_id":"MDU6TGFiZWwxMTk0ODQzMjIz","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/refactor","name":"refactor","color":"cfd3d7","default":false,"description":"Refactoring & cleanup"},{"id":1194843226,"node_id":"MDU6TGFiZWwxMTk0ODQzMjI2","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/good%20first%20issue","name":"good first issue","color":"EF82C8","default":true,"description":"Good for newcomers"},{"id":3523090144,"node_id":"LA_kwDOCePRrs7R_hbg","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/taken","name":"taken","color":"9619EF","default":false,"description":"this ticket is taken"}],"state":"open","locked":false,"assignee":{"login":"edmondywen","id":71907436,"node_id":"MDQ6VXNlcjcxOTA3NDM2","avatar_url":"https://avatars.githubusercontent.com/u/71907436?v=4","gravatar_id":"","url":"https://api.github.com/users/edmondywen","html_url":"https://github.com/edmondywen","followers_url":"https://api.github.com/users/edmondywen/followers","following_url":"https://api.github.com/users/edmondywen/following{/other_user}","gists_url":"https://api.github.com/users/edmondywen/gists{/gist_id}","starred_url":"https://api.github.com/users/edmondywen/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/edmondywen/subscriptions","organizations_url":"https://api.github.com/users/edmondywen/orgs","repos_url":"https://api.github.com/users/edmondywen/repos","events_url":"https://api.github.com/users/edmondywen/events{/privacy}","received_events_url":"https://api.github.com/users/edmondywen/received_events","type":"User","site_admin":false},"assignees":[{"login":"edmondywen","id":71907436,"node_id":"MDQ6VXNlcjcxOTA3NDM2","avatar_url":"https://avatars.githubusercontent.com/u/71907436?v=4","gravatar_id":"","url":"https://api.github.com/users/edmondywen","html_url":"https://github.com/edmondywen","followers_url":"https://api.github.com/users/edmondywen/followers","following_url":"https://api.github.com/users/edmondywen/following{/other_user}","gists_url":"https://api.github.com/users/edmondywen/gists{/gist_id}","starred_url":"https://api.github.com/users/edmondywen/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/edmondywen/subscriptions","organizations_url":"https://api.github.com/users/edmondywen/orgs","repos_url":"https://api.github.com/users/edmondywen/repos","events_url":"https://api.github.com/users/edmondywen/events{/privacy}","received_events_url":"https://api.github.com/users/edmondywen/received_events","type":"User","site_admin":false}],"milestone":null,"comments":0,"created_at":"2021-04-26T02:32:13Z","updated_at":"2021-11-09T18:55:07Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"https://github.com/uclaacm/TeachLAFrontend/blob/master/src/components/common/Radio.js\r\nThis would require test changes as well.","reactions":{"url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/489/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/489/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/429","repository_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend","labels_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/429/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/429/comments","events_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/429/events","html_url":"https://github.com/uclaacm/TeachLAFrontend/issues/429","id":796574968,"node_id":"MDU6SXNzdWU3OTY1NzQ5Njg=","number":429,"title":"Refactor modal-like components to single component-variants.","user":{"login":"krashanoff","id":7704542,"node_id":"MDQ6VXNlcjc3MDQ1NDI=","avatar_url":"https://avatars.githubusercontent.com/u/7704542?v=4","gravatar_id":"","url":"https://api.github.com/users/krashanoff","html_url":"https://github.com/krashanoff","followers_url":"https://api.github.com/users/krashanoff/followers","following_url":"https://api.github.com/users/krashanoff/following{/other_user}","gists_url":"https://api.github.com/users/krashanoff/gists{/gist_id}","starred_url":"https://api.github.com/users/krashanoff/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/krashanoff/subscriptions","organizations_url":"https://api.github.com/users/krashanoff/orgs","repos_url":"https://api.github.com/users/krashanoff/repos","events_url":"https://api.github.com/users/krashanoff/events{/privacy}","received_events_url":"https://api.github.com/users/krashanoff/received_events","type":"User","site_admin":false},"labels":[{"id":1194843223,"node_id":"MDU6TGFiZWwxMTk0ODQzMjIz","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/refactor","name":"refactor","color":"cfd3d7","default":false,"description":"Refactoring & cleanup"},{"id":1194843226,"node_id":"MDU6TGFiZWwxMTk0ODQzMjI2","url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/labels/good%20first%20issue","name":"good first issue","color":"EF82C8","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":null,"assignees":[],"milestone":null,"comments":0,"created_at":"2021-01-29T04:47:39Z","updated_at":"2021-10-11T00:22:35Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"Right now, we have a small army of components to fulfill our `Modal` purposes. We can consolidate their functionality into a single component that changes its render routine based on the props passed to it. Refactors like this will make our code more readable and more maintainable.","reactions":{"url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/429/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/TeachLAFrontend/issues/429/timeline","performed_via_github_app":null,"state_reason":null,"score":1}],"project":{"name":"TeachLAFrontend","description":"🌱 The frontend for Teach LA's online IDE, designed to teach kids how to code!","link":"https://editor.uclaacm.com","repo":"https://github.com/uclaacm/TeachLAFrontend","lang":"JavaScript","topics":["education","firebase","ide","react","redux","teach-la"],"image":"/committee-logos/teachla-logo.png","alt":"ACM Teach LA Logo"},"repoURL":"https://api.github.com/repos/uclaacm/TeachLAFrontend"},{"issues":[{"url":"https://api.github.com/repos/uclaacm/status-check/issues/9","repository_url":"https://api.github.com/repos/uclaacm/status-check","labels_url":"https://api.github.com/repos/uclaacm/status-check/issues/9/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/status-check/issues/9/comments","events_url":"https://api.github.com/repos/uclaacm/status-check/issues/9/events","html_url":"https://github.com/uclaacm/status-check/issues/9","id":1115641879,"node_id":"I_kwDOGQh08s5Cf1gX","number":9,"title":"Set up CI","user":{"login":"mattxwang","id":14893287,"node_id":"MDQ6VXNlcjE0ODkzMjg3","avatar_url":"https://avatars.githubusercontent.com/u/14893287?v=4","gravatar_id":"","url":"https://api.github.com/users/mattxwang","html_url":"https://github.com/mattxwang","followers_url":"https://api.github.com/users/mattxwang/followers","following_url":"https://api.github.com/users/mattxwang/following{/other_user}","gists_url":"https://api.github.com/users/mattxwang/gists{/gist_id}","starred_url":"https://api.github.com/users/mattxwang/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/mattxwang/subscriptions","organizations_url":"https://api.github.com/users/mattxwang/orgs","repos_url":"https://api.github.com/users/mattxwang/repos","events_url":"https://api.github.com/users/mattxwang/events{/privacy}","received_events_url":"https://api.github.com/users/mattxwang/received_events","type":"User","site_admin":false},"labels":[{"id":3476186026,"node_id":"LA_kwDOGQh08s7PMmOq","url":"https://api.github.com/repos/uclaacm/status-check/labels/enhancement","name":"enhancement","color":"a2eeef","default":true,"description":"New feature or request"},{"id":3476186030,"node_id":"LA_kwDOGQh08s7PMmOu","url":"https://api.github.com/repos/uclaacm/status-check/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":null,"assignees":[],"milestone":null,"comments":2,"created_at":"2022-01-27T00:39:31Z","updated_at":"2022-03-02T21:22:53Z","closed_at":null,"author_association":"NONE","active_lock_reason":null,"body":"It would be great to have some helpful CI for reviewing PRs! This could include:\r\n\r\n* checking `yarn build`\r\n* if relevant, running `yarn test`\r\n* linting (FE & BE) - ESLint, Stylelint, etc.\r\n\r\nIf you're able to split up the FE & BE cleanly, you can also blend in the Netlify CD deploy for the frontend.\r\n\r\nWe should use GitHub Actions - you can browse around other ACM repos that use JavaScript to see how they're implemented. The trickiest thing might be resolving the monorepo nature of this project.","reactions":{"url":"https://api.github.com/repos/uclaacm/status-check/issues/9/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/status-check/issues/9/timeline","performed_via_github_app":null,"state_reason":null,"score":1}],"project":{"name":"status-check","description":"Check the health/status of ACM's various websites/deployments!","link":"https://acm-status-check.netlify.app","repo":"https://github.com/uclaacm/status-check","lang":"JavaScript","topics":[],"image":"/logo.png","alt":"ACM @ UCLA's Logo"},"repoURL":"https://api.github.com/repos/uclaacm/status-check"},{"issues":[{"url":"https://api.github.com/repos/uclaacm/dev-pathways/issues/98","repository_url":"https://api.github.com/repos/uclaacm/dev-pathways","labels_url":"https://api.github.com/repos/uclaacm/dev-pathways/issues/98/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/dev-pathways/issues/98/comments","events_url":"https://api.github.com/repos/uclaacm/dev-pathways/issues/98/events","html_url":"https://github.com/uclaacm/dev-pathways/issues/98","id":1105191838,"node_id":"I_kwDOE8dP8s5B3-Oe","number":98,"title":"Prevent moving from the quiz page to pathways page if not all prompts have been filled","user":{"login":"doubleiis02","id":50722281,"node_id":"MDQ6VXNlcjUwNzIyMjgx","avatar_url":"https://avatars.githubusercontent.com/u/50722281?v=4","gravatar_id":"","url":"https://api.github.com/users/doubleiis02","html_url":"https://github.com/doubleiis02","followers_url":"https://api.github.com/users/doubleiis02/followers","following_url":"https://api.github.com/users/doubleiis02/following{/other_user}","gists_url":"https://api.github.com/users/doubleiis02/gists{/gist_id}","starred_url":"https://api.github.com/users/doubleiis02/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/doubleiis02/subscriptions","organizations_url":"https://api.github.com/users/doubleiis02/orgs","repos_url":"https://api.github.com/users/doubleiis02/repos","events_url":"https://api.github.com/users/doubleiis02/events{/privacy}","received_events_url":"https://api.github.com/users/doubleiis02/received_events","type":"User","site_admin":false},"labels":[{"id":2677692716,"node_id":"MDU6TGFiZWwyNjc3NjkyNzE2","url":"https://api.github.com/repos/uclaacm/dev-pathways/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"},{"id":3735630534,"node_id":"LA_kwDOE8dP8s7eqTLG","url":"https://api.github.com/repos/uclaacm/dev-pathways/labels/easy","name":"easy","color":"c7c7c7","default":false,"description":""}],"state":"open","locked":false,"assignee":{"login":"SruthiR03","id":67874140,"node_id":"MDQ6VXNlcjY3ODc0MTQw","avatar_url":"https://avatars.githubusercontent.com/u/67874140?v=4","gravatar_id":"","url":"https://api.github.com/users/SruthiR03","html_url":"https://github.com/SruthiR03","followers_url":"https://api.github.com/users/SruthiR03/followers","following_url":"https://api.github.com/users/SruthiR03/following{/other_user}","gists_url":"https://api.github.com/users/SruthiR03/gists{/gist_id}","starred_url":"https://api.github.com/users/SruthiR03/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/SruthiR03/subscriptions","organizations_url":"https://api.github.com/users/SruthiR03/orgs","repos_url":"https://api.github.com/users/SruthiR03/repos","events_url":"https://api.github.com/users/SruthiR03/events{/privacy}","received_events_url":"https://api.github.com/users/SruthiR03/received_events","type":"User","site_admin":false},"assignees":[{"login":"SruthiR03","id":67874140,"node_id":"MDQ6VXNlcjY3ODc0MTQw","avatar_url":"https://avatars.githubusercontent.com/u/67874140?v=4","gravatar_id":"","url":"https://api.github.com/users/SruthiR03","html_url":"https://github.com/SruthiR03","followers_url":"https://api.github.com/users/SruthiR03/followers","following_url":"https://api.github.com/users/SruthiR03/following{/other_user}","gists_url":"https://api.github.com/users/SruthiR03/gists{/gist_id}","starred_url":"https://api.github.com/users/SruthiR03/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/SruthiR03/subscriptions","organizations_url":"https://api.github.com/users/SruthiR03/orgs","repos_url":"https://api.github.com/users/SruthiR03/repos","events_url":"https://api.github.com/users/SruthiR03/events{/privacy}","received_events_url":"https://api.github.com/users/SruthiR03/received_events","type":"User","site_admin":false}],"milestone":null,"comments":0,"created_at":"2022-01-16T21:25:53Z","updated_at":"2022-01-26T06:04:56Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"All selections in the `Quiz.js` page should be filled out in order for the `Generate` button to work and allow the user to move to the generated `Pathway.js` page. If that is not the case, then alert the user that all questions must be answered.","reactions":{"url":"https://api.github.com/repos/uclaacm/dev-pathways/issues/98/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/dev-pathways/issues/98/timeline","performed_via_github_app":null,"state_reason":null,"score":1}],"project":{"name":"dev-pathways","description":"Providing learning pathways to new devs at ACM since 2021. 📕📗📘","link":"https://dev-pathways.netlify.app/","repo":"https://github.com/uclaacm/dev-pathways","lang":"JavaScript","topics":["teach-la"],"image":"/committee-logos/teachla-logo.png","alt":"ACM Teach LA Logo"},"repoURL":"https://api.github.com/repos/uclaacm/dev-pathways"},{"issues":[{"url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/68","repository_url":"https://api.github.com/repos/uclaacm/teach-la-go-backend","labels_url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/68/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/68/comments","events_url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/68/events","html_url":"https://github.com/uclaacm/teach-la-go-backend/issues/68","id":1025535836,"node_id":"I_kwDODQTu2849IG9c","number":68,"title":"Refactor db#Db.UpdateProgram into a handler function","user":{"login":"timothycpoon","id":8311368,"node_id":"MDQ6VXNlcjgzMTEzNjg=","avatar_url":"https://avatars.githubusercontent.com/u/8311368?v=4","gravatar_id":"","url":"https://api.github.com/users/timothycpoon","html_url":"https://github.com/timothycpoon","followers_url":"https://api.github.com/users/timothycpoon/followers","following_url":"https://api.github.com/users/timothycpoon/following{/other_user}","gists_url":"https://api.github.com/users/timothycpoon/gists{/gist_id}","starred_url":"https://api.github.com/users/timothycpoon/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/timothycpoon/subscriptions","organizations_url":"https://api.github.com/users/timothycpoon/orgs","repos_url":"https://api.github.com/users/timothycpoon/repos","events_url":"https://api.github.com/users/timothycpoon/events{/privacy}","received_events_url":"https://api.github.com/users/timothycpoon/received_events","type":"User","site_admin":false},"labels":[{"id":1646363861,"node_id":"MDU6TGFiZWwxNjQ2MzYzODYx","url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"},{"id":3053709627,"node_id":"MDU6TGFiZWwzMDUzNzA5NjI3","url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/labels/refactor","name":"refactor","color":"e99695","default":false,"description":"Reorganizes existing code without modifying functionality"}],"state":"open","locked":false,"assignee":null,"assignees":[],"milestone":null,"comments":0,"created_at":"2021-10-13T17:51:59Z","updated_at":"2022-10-13T01:10:30Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"See https://github.com/uclaacm/teach-la-go-backend/wiki/How-to-refactor-a-Backend-API, or https://github.com/uclaacm/teach-la-go-backend/pull/57 for an example.","reactions":{"url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/68/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/68/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/63","repository_url":"https://api.github.com/repos/uclaacm/teach-la-go-backend","labels_url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/63/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/63/comments","events_url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/63/events","html_url":"https://github.com/uclaacm/teach-la-go-backend/issues/63","id":1018940273,"node_id":"I_kwDODQTu2848u8tx","number":63,"title":"Refactor db#DB.UpdateUser into a handler function","user":{"login":"timothycpoon","id":8311368,"node_id":"MDQ6VXNlcjgzMTEzNjg=","avatar_url":"https://avatars.githubusercontent.com/u/8311368?v=4","gravatar_id":"","url":"https://api.github.com/users/timothycpoon","html_url":"https://github.com/timothycpoon","followers_url":"https://api.github.com/users/timothycpoon/followers","following_url":"https://api.github.com/users/timothycpoon/following{/other_user}","gists_url":"https://api.github.com/users/timothycpoon/gists{/gist_id}","starred_url":"https://api.github.com/users/timothycpoon/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/timothycpoon/subscriptions","organizations_url":"https://api.github.com/users/timothycpoon/orgs","repos_url":"https://api.github.com/users/timothycpoon/repos","events_url":"https://api.github.com/users/timothycpoon/events{/privacy}","received_events_url":"https://api.github.com/users/timothycpoon/received_events","type":"User","site_admin":false},"labels":[{"id":1646363861,"node_id":"MDU6TGFiZWwxNjQ2MzYzODYx","url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"},{"id":3053709627,"node_id":"MDU6TGFiZWwzMDUzNzA5NjI3","url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/labels/refactor","name":"refactor","color":"e99695","default":false,"description":"Reorganizes existing code without modifying functionality"}],"state":"open","locked":false,"assignee":{"login":"tr-vs","id":70074725,"node_id":"MDQ6VXNlcjcwMDc0NzI1","avatar_url":"https://avatars.githubusercontent.com/u/70074725?v=4","gravatar_id":"","url":"https://api.github.com/users/tr-vs","html_url":"https://github.com/tr-vs","followers_url":"https://api.github.com/users/tr-vs/followers","following_url":"https://api.github.com/users/tr-vs/following{/other_user}","gists_url":"https://api.github.com/users/tr-vs/gists{/gist_id}","starred_url":"https://api.github.com/users/tr-vs/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/tr-vs/subscriptions","organizations_url":"https://api.github.com/users/tr-vs/orgs","repos_url":"https://api.github.com/users/tr-vs/repos","events_url":"https://api.github.com/users/tr-vs/events{/privacy}","received_events_url":"https://api.github.com/users/tr-vs/received_events","type":"User","site_admin":false},"assignees":[{"login":"tr-vs","id":70074725,"node_id":"MDQ6VXNlcjcwMDc0NzI1","avatar_url":"https://avatars.githubusercontent.com/u/70074725?v=4","gravatar_id":"","url":"https://api.github.com/users/tr-vs","html_url":"https://github.com/tr-vs","followers_url":"https://api.github.com/users/tr-vs/followers","following_url":"https://api.github.com/users/tr-vs/following{/other_user}","gists_url":"https://api.github.com/users/tr-vs/gists{/gist_id}","starred_url":"https://api.github.com/users/tr-vs/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/tr-vs/subscriptions","organizations_url":"https://api.github.com/users/tr-vs/orgs","repos_url":"https://api.github.com/users/tr-vs/repos","events_url":"https://api.github.com/users/tr-vs/events{/privacy}","received_events_url":"https://api.github.com/users/tr-vs/received_events","type":"User","site_admin":false}],"milestone":null,"comments":0,"created_at":"2021-10-06T18:31:38Z","updated_at":"2024-02-06T04:49:44Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"See https://github.com/uclaacm/teach-la-go-backend/wiki/How-to-refactor-a-Backend-API, or https://github.com/uclaacm/teach-la-go-backend/pull/57 for an example.","reactions":{"url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/63/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/63/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/18","repository_url":"https://api.github.com/repos/uclaacm/teach-la-go-backend","labels_url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/18/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/18/comments","events_url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/18/events","html_url":"https://github.com/uclaacm/teach-la-go-backend/issues/18","id":722796370,"node_id":"MDU6SXNzdWU3MjI3OTYzNzA=","number":18,"title":"Rigorous testing for DeleteUser","user":{"login":"krashanoff","id":7704542,"node_id":"MDQ6VXNlcjc3MDQ1NDI=","avatar_url":"https://avatars.githubusercontent.com/u/7704542?v=4","gravatar_id":"","url":"https://api.github.com/users/krashanoff","html_url":"https://github.com/krashanoff","followers_url":"https://api.github.com/users/krashanoff/followers","following_url":"https://api.github.com/users/krashanoff/following{/other_user}","gists_url":"https://api.github.com/users/krashanoff/gists{/gist_id}","starred_url":"https://api.github.com/users/krashanoff/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/krashanoff/subscriptions","organizations_url":"https://api.github.com/users/krashanoff/orgs","repos_url":"https://api.github.com/users/krashanoff/repos","events_url":"https://api.github.com/users/krashanoff/events{/privacy}","received_events_url":"https://api.github.com/users/krashanoff/received_events","type":"User","site_admin":false},"labels":[{"id":1646363861,"node_id":"MDU6TGFiZWwxNjQ2MzYzODYx","url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":{"login":"matthewcyy","id":28741405,"node_id":"MDQ6VXNlcjI4NzQxNDA1","avatar_url":"https://avatars.githubusercontent.com/u/28741405?v=4","gravatar_id":"","url":"https://api.github.com/users/matthewcyy","html_url":"https://github.com/matthewcyy","followers_url":"https://api.github.com/users/matthewcyy/followers","following_url":"https://api.github.com/users/matthewcyy/following{/other_user}","gists_url":"https://api.github.com/users/matthewcyy/gists{/gist_id}","starred_url":"https://api.github.com/users/matthewcyy/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/matthewcyy/subscriptions","organizations_url":"https://api.github.com/users/matthewcyy/orgs","repos_url":"https://api.github.com/users/matthewcyy/repos","events_url":"https://api.github.com/users/matthewcyy/events{/privacy}","received_events_url":"https://api.github.com/users/matthewcyy/received_events","type":"User","site_admin":false},"assignees":[{"login":"matthewcyy","id":28741405,"node_id":"MDQ6VXNlcjI4NzQxNDA1","avatar_url":"https://avatars.githubusercontent.com/u/28741405?v=4","gravatar_id":"","url":"https://api.github.com/users/matthewcyy","html_url":"https://github.com/matthewcyy","followers_url":"https://api.github.com/users/matthewcyy/followers","following_url":"https://api.github.com/users/matthewcyy/following{/other_user}","gists_url":"https://api.github.com/users/matthewcyy/gists{/gist_id}","starred_url":"https://api.github.com/users/matthewcyy/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/matthewcyy/subscriptions","organizations_url":"https://api.github.com/users/matthewcyy/orgs","repos_url":"https://api.github.com/users/matthewcyy/repos","events_url":"https://api.github.com/users/matthewcyy/events{/privacy}","received_events_url":"https://api.github.com/users/matthewcyy/received_events","type":"User","site_admin":false}],"milestone":null,"comments":1,"created_at":"2020-10-16T01:42:17Z","updated_at":"2021-04-24T22:13:09Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"","reactions":{"url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/18/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/teach-la-go-backend/issues/18/timeline","performed_via_github_app":null,"state_reason":null,"score":1}],"project":{"name":"teach-la-go-backend","description":"🌱 Go Backend for Teach LA's Editor","link":"https://documenter.getpostman.com/view/10224331/TW6xmnn2","repo":"https://github.com/uclaacm/teach-la-go-backend","lang":"Go","topics":["backend","education","go","golang","teach-la"],"image":"/committee-logos/teachla-logo.png","alt":"ACM Teach LA Logo"},"repoURL":"https://api.github.com/repos/uclaacm/teach-la-go-backend"},{"issues":[{"url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/347","repository_url":"https://api.github.com/repos/uclaacm/teach-la-website","labels_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/347/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/347/comments","events_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/347/events","html_url":"https://github.com/uclaacm/teach-la-website/issues/347","id":895786712,"node_id":"MDU6SXNzdWU4OTU3ODY3MTI=","number":347,"title":"Link Classes in the About Page to Class Page","user":{"login":"vivianha534","id":56574435,"node_id":"MDQ6VXNlcjU2NTc0NDM1","avatar_url":"https://avatars.githubusercontent.com/u/56574435?v=4","gravatar_id":"","url":"https://api.github.com/users/vivianha534","html_url":"https://github.com/vivianha534","followers_url":"https://api.github.com/users/vivianha534/followers","following_url":"https://api.github.com/users/vivianha534/following{/other_user}","gists_url":"https://api.github.com/users/vivianha534/gists{/gist_id}","starred_url":"https://api.github.com/users/vivianha534/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/vivianha534/subscriptions","organizations_url":"https://api.github.com/users/vivianha534/orgs","repos_url":"https://api.github.com/users/vivianha534/repos","events_url":"https://api.github.com/users/vivianha534/events{/privacy}","received_events_url":"https://api.github.com/users/vivianha534/received_events","type":"User","site_admin":false},"labels":[{"id":1408021965,"node_id":"MDU6TGFiZWwxNDA4MDIxOTY1","url":"https://api.github.com/repos/uclaacm/teach-la-website/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":{"login":"cjmaduno","id":40917956,"node_id":"MDQ6VXNlcjQwOTE3OTU2","avatar_url":"https://avatars.githubusercontent.com/u/40917956?v=4","gravatar_id":"","url":"https://api.github.com/users/cjmaduno","html_url":"https://github.com/cjmaduno","followers_url":"https://api.github.com/users/cjmaduno/followers","following_url":"https://api.github.com/users/cjmaduno/following{/other_user}","gists_url":"https://api.github.com/users/cjmaduno/gists{/gist_id}","starred_url":"https://api.github.com/users/cjmaduno/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/cjmaduno/subscriptions","organizations_url":"https://api.github.com/users/cjmaduno/orgs","repos_url":"https://api.github.com/users/cjmaduno/repos","events_url":"https://api.github.com/users/cjmaduno/events{/privacy}","received_events_url":"https://api.github.com/users/cjmaduno/received_events","type":"User","site_admin":false},"assignees":[{"login":"cjmaduno","id":40917956,"node_id":"MDQ6VXNlcjQwOTE3OTU2","avatar_url":"https://avatars.githubusercontent.com/u/40917956?v=4","gravatar_id":"","url":"https://api.github.com/users/cjmaduno","html_url":"https://github.com/cjmaduno","followers_url":"https://api.github.com/users/cjmaduno/followers","following_url":"https://api.github.com/users/cjmaduno/following{/other_user}","gists_url":"https://api.github.com/users/cjmaduno/gists{/gist_id}","starred_url":"https://api.github.com/users/cjmaduno/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/cjmaduno/subscriptions","organizations_url":"https://api.github.com/users/cjmaduno/orgs","repos_url":"https://api.github.com/users/cjmaduno/repos","events_url":"https://api.github.com/users/cjmaduno/events{/privacy}","received_events_url":"https://api.github.com/users/cjmaduno/received_events","type":"User","site_admin":false}],"milestone":null,"comments":0,"created_at":"2021-05-19T19:07:37Z","updated_at":"2021-11-10T02:28:34Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"Currently North Hollywood Web & Mobile App Dev's class is linked to its corresponding class pages. However, the rest of the classes are not linked to their class pages.\r\n\r\n- Link the rest of the classes to their class pages\r\n - If you need a refrence, look at how North Hollywood has the classes linked\r\n - You'll most likely need to modify the about.html file\r\n- Class pages can be found: https://teachla.uclaacm.com/classes\r\n\r\nThis ticket should also update the class schedules on the about page. Follow the existing format on the about page.\r\n- Emerson - Wednesdays 8-8:50am\r\n- Western Ave T.E.C.H. Magnet - Tuesdays 10:19-11:49am\r\n- North Hollywood High (AI) - Mondays 8-8:57am (likely to change to Thursdays 8-8:57am)\r\n- North Hollywood High (React Native) - Not finalized \r\n- Scratch- Fridays 12:40-1:30pm (may change)","reactions":{"url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/347/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/347/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/340","repository_url":"https://api.github.com/repos/uclaacm/teach-la-website","labels_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/340/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/340/comments","events_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/340/events","html_url":"https://github.com/uclaacm/teach-la-website/issues/340","id":875101389,"node_id":"MDU6SXNzdWU4NzUxMDEzODk=","number":340,"title":"Update Careers in CS and AI ethics","user":{"login":"vivianha534","id":56574435,"node_id":"MDQ6VXNlcjU2NTc0NDM1","avatar_url":"https://avatars.githubusercontent.com/u/56574435?v=4","gravatar_id":"","url":"https://api.github.com/users/vivianha534","html_url":"https://github.com/vivianha534","followers_url":"https://api.github.com/users/vivianha534/followers","following_url":"https://api.github.com/users/vivianha534/following{/other_user}","gists_url":"https://api.github.com/users/vivianha534/gists{/gist_id}","starred_url":"https://api.github.com/users/vivianha534/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/vivianha534/subscriptions","organizations_url":"https://api.github.com/users/vivianha534/orgs","repos_url":"https://api.github.com/users/vivianha534/repos","events_url":"https://api.github.com/users/vivianha534/events{/privacy}","received_events_url":"https://api.github.com/users/vivianha534/received_events","type":"User","site_admin":false},"labels":[{"id":1408021965,"node_id":"MDU6TGFiZWwxNDA4MDIxOTY1","url":"https://api.github.com/repos/uclaacm/teach-la-website/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":{"login":"celebilaw","id":72050500,"node_id":"MDQ6VXNlcjcyMDUwNTAw","avatar_url":"https://avatars.githubusercontent.com/u/72050500?v=4","gravatar_id":"","url":"https://api.github.com/users/celebilaw","html_url":"https://github.com/celebilaw","followers_url":"https://api.github.com/users/celebilaw/followers","following_url":"https://api.github.com/users/celebilaw/following{/other_user}","gists_url":"https://api.github.com/users/celebilaw/gists{/gist_id}","starred_url":"https://api.github.com/users/celebilaw/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/celebilaw/subscriptions","organizations_url":"https://api.github.com/users/celebilaw/orgs","repos_url":"https://api.github.com/users/celebilaw/repos","events_url":"https://api.github.com/users/celebilaw/events{/privacy}","received_events_url":"https://api.github.com/users/celebilaw/received_events","type":"User","site_admin":false},"assignees":[{"login":"celebilaw","id":72050500,"node_id":"MDQ6VXNlcjcyMDUwNTAw","avatar_url":"https://avatars.githubusercontent.com/u/72050500?v=4","gravatar_id":"","url":"https://api.github.com/users/celebilaw","html_url":"https://github.com/celebilaw","followers_url":"https://api.github.com/users/celebilaw/followers","following_url":"https://api.github.com/users/celebilaw/following{/other_user}","gists_url":"https://api.github.com/users/celebilaw/gists{/gist_id}","starred_url":"https://api.github.com/users/celebilaw/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/celebilaw/subscriptions","organizations_url":"https://api.github.com/users/celebilaw/orgs","repos_url":"https://api.github.com/users/celebilaw/repos","events_url":"https://api.github.com/users/celebilaw/events{/privacy}","received_events_url":"https://api.github.com/users/celebilaw/received_events","type":"User","site_admin":false}],"milestone":null,"comments":0,"created_at":"2021-05-04T04:52:45Z","updated_at":"2021-10-27T21:36:48Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"Create an archive for AI Ethics similar to Careers in CS which contains\r\n- Facial Recognition @ Bernstein High School (WildHacks Hackathon) - 2021\r\n- Facial Recognition @ University High School - 2021\r\n\r\nAdd the following to the existing Careers in CS archive\r\n- Careers in CS @ Bernstein High School (WildHacks Hackathon) - 2021\r\n\r\nFor reference the archive for the AI Ethics page will look really similar to CS Careers in CS and the code for that can be found in career-pathways.html\r\n","reactions":{"url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/340/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/340/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/312","repository_url":"https://api.github.com/repos/uclaacm/teach-la-website","labels_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/312/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/312/comments","events_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/312/events","html_url":"https://github.com/uclaacm/teach-la-website/issues/312","id":854034055,"node_id":"MDU6SXNzdWU4NTQwMzQwNTU=","number":312,"title":"Add React Native Lessons","user":{"login":"vivianha534","id":56574435,"node_id":"MDQ6VXNlcjU2NTc0NDM1","avatar_url":"https://avatars.githubusercontent.com/u/56574435?v=4","gravatar_id":"","url":"https://api.github.com/users/vivianha534","html_url":"https://github.com/vivianha534","followers_url":"https://api.github.com/users/vivianha534/followers","following_url":"https://api.github.com/users/vivianha534/following{/other_user}","gists_url":"https://api.github.com/users/vivianha534/gists{/gist_id}","starred_url":"https://api.github.com/users/vivianha534/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/vivianha534/subscriptions","organizations_url":"https://api.github.com/users/vivianha534/orgs","repos_url":"https://api.github.com/users/vivianha534/repos","events_url":"https://api.github.com/users/vivianha534/events{/privacy}","received_events_url":"https://api.github.com/users/vivianha534/received_events","type":"User","site_admin":false},"labels":[{"id":1408021965,"node_id":"MDU6TGFiZWwxNDA4MDIxOTY1","url":"https://api.github.com/repos/uclaacm/teach-la-website/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":{"login":"josephramirezgit","id":87892893,"node_id":"MDQ6VXNlcjg3ODkyODkz","avatar_url":"https://avatars.githubusercontent.com/u/87892893?v=4","gravatar_id":"","url":"https://api.github.com/users/josephramirezgit","html_url":"https://github.com/josephramirezgit","followers_url":"https://api.github.com/users/josephramirezgit/followers","following_url":"https://api.github.com/users/josephramirezgit/following{/other_user}","gists_url":"https://api.github.com/users/josephramirezgit/gists{/gist_id}","starred_url":"https://api.github.com/users/josephramirezgit/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/josephramirezgit/subscriptions","organizations_url":"https://api.github.com/users/josephramirezgit/orgs","repos_url":"https://api.github.com/users/josephramirezgit/repos","events_url":"https://api.github.com/users/josephramirezgit/events{/privacy}","received_events_url":"https://api.github.com/users/josephramirezgit/received_events","type":"User","site_admin":false},"assignees":[{"login":"josephramirezgit","id":87892893,"node_id":"MDQ6VXNlcjg3ODkyODkz","avatar_url":"https://avatars.githubusercontent.com/u/87892893?v=4","gravatar_id":"","url":"https://api.github.com/users/josephramirezgit","html_url":"https://github.com/josephramirezgit","followers_url":"https://api.github.com/users/josephramirezgit/followers","following_url":"https://api.github.com/users/josephramirezgit/following{/other_user}","gists_url":"https://api.github.com/users/josephramirezgit/gists{/gist_id}","starred_url":"https://api.github.com/users/josephramirezgit/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/josephramirezgit/subscriptions","organizations_url":"https://api.github.com/users/josephramirezgit/orgs","repos_url":"https://api.github.com/users/josephramirezgit/repos","events_url":"https://api.github.com/users/josephramirezgit/events{/privacy}","received_events_url":"https://api.github.com/users/josephramirezgit/received_events","type":"User","site_admin":false}],"milestone":null,"comments":0,"created_at":"2021-04-09T00:15:10Z","updated_at":"2021-10-28T03:25:47Z","closed_at":null,"author_association":"CONTRIBUTOR","active_lock_reason":null,"body":"Currently our React Native Class Page is not up to date. https://teachla.uclaacm.com/classes/rnative\r\n- In order to make it up to date, you will need to create new files in the _rnative directory for each of the missing lessons\r\n- Here's a link to the github repository with all of the lesson plans: https://github.com/uclaacm/react-native-course-20-21\r\n- You can use 02-intro-html-css.md as an example of how you should add new lessons","reactions":{"url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/312/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/312/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/245","repository_url":"https://api.github.com/repos/uclaacm/teach-la-website","labels_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/245/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/245/comments","events_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/245/events","html_url":"https://github.com/uclaacm/teach-la-website/issues/245","id":801645108,"node_id":"MDU6SXNzdWU4MDE2NDUxMDg=","number":245,"title":"Change \"More info\" lead to check for blog posts","user":{"login":"mattxwang","id":14893287,"node_id":"MDQ6VXNlcjE0ODkzMjg3","avatar_url":"https://avatars.githubusercontent.com/u/14893287?v=4","gravatar_id":"","url":"https://api.github.com/users/mattxwang","html_url":"https://github.com/mattxwang","followers_url":"https://api.github.com/users/mattxwang/followers","following_url":"https://api.github.com/users/mattxwang/following{/other_user}","gists_url":"https://api.github.com/users/mattxwang/gists{/gist_id}","starred_url":"https://api.github.com/users/mattxwang/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/mattxwang/subscriptions","organizations_url":"https://api.github.com/users/mattxwang/orgs","repos_url":"https://api.github.com/users/mattxwang/repos","events_url":"https://api.github.com/users/mattxwang/events{/privacy}","received_events_url":"https://api.github.com/users/mattxwang/received_events","type":"User","site_admin":false},"labels":[{"id":1408021965,"node_id":"MDU6TGFiZWwxNDA4MDIxOTY1","url":"https://api.github.com/repos/uclaacm/teach-la-website/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":{"login":"kmpablo","id":77227941,"node_id":"MDQ6VXNlcjc3MjI3OTQx","avatar_url":"https://avatars.githubusercontent.com/u/77227941?v=4","gravatar_id":"","url":"https://api.github.com/users/kmpablo","html_url":"https://github.com/kmpablo","followers_url":"https://api.github.com/users/kmpablo/followers","following_url":"https://api.github.com/users/kmpablo/following{/other_user}","gists_url":"https://api.github.com/users/kmpablo/gists{/gist_id}","starred_url":"https://api.github.com/users/kmpablo/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/kmpablo/subscriptions","organizations_url":"https://api.github.com/users/kmpablo/orgs","repos_url":"https://api.github.com/users/kmpablo/repos","events_url":"https://api.github.com/users/kmpablo/events{/privacy}","received_events_url":"https://api.github.com/users/kmpablo/received_events","type":"User","site_admin":false},"assignees":[{"login":"kmpablo","id":77227941,"node_id":"MDQ6VXNlcjc3MjI3OTQx","avatar_url":"https://avatars.githubusercontent.com/u/77227941?v=4","gravatar_id":"","url":"https://api.github.com/users/kmpablo","html_url":"https://github.com/kmpablo","followers_url":"https://api.github.com/users/kmpablo/followers","following_url":"https://api.github.com/users/kmpablo/following{/other_user}","gists_url":"https://api.github.com/users/kmpablo/gists{/gist_id}","starred_url":"https://api.github.com/users/kmpablo/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/kmpablo/subscriptions","organizations_url":"https://api.github.com/users/kmpablo/orgs","repos_url":"https://api.github.com/users/kmpablo/repos","events_url":"https://api.github.com/users/kmpablo/events{/privacy}","received_events_url":"https://api.github.com/users/kmpablo/received_events","type":"User","site_admin":false}],"milestone":null,"comments":1,"created_at":"2021-02-04T21:22:38Z","updated_at":"2021-02-26T02:26:04Z","closed_at":null,"author_association":"COLLABORATOR","active_lock_reason":null,"body":"Thanks to @MDZHX in #213, we now have a list on each team member's page of the blog posts that they've written!\r\n\r\nHowever, we now need to update our check for the \"More Info\" lead in in `team.html` to also include whether or not the user has written a blog post. I think this is blocked by #242!","reactions":{"url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/245/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/245/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/231","repository_url":"https://api.github.com/repos/uclaacm/teach-la-website","labels_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/231/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/231/comments","events_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/231/events","html_url":"https://github.com/uclaacm/teach-la-website/issues/231","id":792643549,"node_id":"MDU6SXNzdWU3OTI2NDM1NDk=","number":231,"title":"Configure HTML Proofer to run basic checks","user":{"login":"mattxwang","id":14893287,"node_id":"MDQ6VXNlcjE0ODkzMjg3","avatar_url":"https://avatars.githubusercontent.com/u/14893287?v=4","gravatar_id":"","url":"https://api.github.com/users/mattxwang","html_url":"https://github.com/mattxwang","followers_url":"https://api.github.com/users/mattxwang/followers","following_url":"https://api.github.com/users/mattxwang/following{/other_user}","gists_url":"https://api.github.com/users/mattxwang/gists{/gist_id}","starred_url":"https://api.github.com/users/mattxwang/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/mattxwang/subscriptions","organizations_url":"https://api.github.com/users/mattxwang/orgs","repos_url":"https://api.github.com/users/mattxwang/repos","events_url":"https://api.github.com/users/mattxwang/events{/privacy}","received_events_url":"https://api.github.com/users/mattxwang/received_events","type":"User","site_admin":false},"labels":[{"id":1408021965,"node_id":"MDU6TGFiZWwxNDA4MDIxOTY1","url":"https://api.github.com/repos/uclaacm/teach-la-website/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":{"login":"maggieelli","id":69731426,"node_id":"MDQ6VXNlcjY5NzMxNDI2","avatar_url":"https://avatars.githubusercontent.com/u/69731426?v=4","gravatar_id":"","url":"https://api.github.com/users/maggieelli","html_url":"https://github.com/maggieelli","followers_url":"https://api.github.com/users/maggieelli/followers","following_url":"https://api.github.com/users/maggieelli/following{/other_user}","gists_url":"https://api.github.com/users/maggieelli/gists{/gist_id}","starred_url":"https://api.github.com/users/maggieelli/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/maggieelli/subscriptions","organizations_url":"https://api.github.com/users/maggieelli/orgs","repos_url":"https://api.github.com/users/maggieelli/repos","events_url":"https://api.github.com/users/maggieelli/events{/privacy}","received_events_url":"https://api.github.com/users/maggieelli/received_events","type":"User","site_admin":false},"assignees":[{"login":"maggieelli","id":69731426,"node_id":"MDQ6VXNlcjY5NzMxNDI2","avatar_url":"https://avatars.githubusercontent.com/u/69731426?v=4","gravatar_id":"","url":"https://api.github.com/users/maggieelli","html_url":"https://github.com/maggieelli","followers_url":"https://api.github.com/users/maggieelli/followers","following_url":"https://api.github.com/users/maggieelli/following{/other_user}","gists_url":"https://api.github.com/users/maggieelli/gists{/gist_id}","starred_url":"https://api.github.com/users/maggieelli/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/maggieelli/subscriptions","organizations_url":"https://api.github.com/users/maggieelli/orgs","repos_url":"https://api.github.com/users/maggieelli/repos","events_url":"https://api.github.com/users/maggieelli/events{/privacy}","received_events_url":"https://api.github.com/users/maggieelli/received_events","type":"User","site_admin":false}],"milestone":null,"comments":0,"created_at":"2021-01-23T20:29:32Z","updated_at":"2021-02-08T18:15:21Z","closed_at":null,"author_association":"COLLABORATOR","active_lock_reason":null,"body":"As our codebase becomes bigger and more and more contributors join our team, we should run some basic checks to make sure our (generated) HTML is valid; things like:\r\n\r\n* are all of our (non-self-closing) tags paired properly?\r\n* do all of our images have `alt` tags?\r\n* do we have typos or invalid props?\r\n* bonus: get opengraph checks to work 😊 \r\n\r\nWe've actually already built this in to our `rake` system with [HTMLProofer](https://github.com/gjtorikian/html-proofer) as `rake test`, with two caveats:\r\n\r\n* we don't run it in our CI action (though we should) - see `.github/workflows`\r\n* we haven't configured it at all - in particular, I'd like to disable the \"no invalid link\" check (`LinkCheck`), since it often is a false positive on Jekyll-generated sites. bonus points if we can get this to work properly too though!\r\n\r\nSo, let's do those two things by making a new `htmlproof` action! This is a great second/third ticket for devs to learn more about how our tooling works 😊 \r\n\r\n(p.s. there is an example on how to configure the `Rakefile` in the [HTMLProofer docs](https://github.com/gjtorikian/html-proofer/wiki/Using-HTMLProofer-From-Ruby-and-Travis); [here are the config options](https://github.com/gjtorikian/html-proofer#configuration))","reactions":{"url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/231/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/231/timeline","performed_via_github_app":null,"state_reason":null,"score":1},{"url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/127","repository_url":"https://api.github.com/repos/uclaacm/teach-la-website","labels_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/127/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/127/comments","events_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/127/events","html_url":"https://github.com/uclaacm/teach-la-website/issues/127","id":727781992,"node_id":"MDU6SXNzdWU3Mjc3ODE5OTI=","number":127,"title":"Alt text passover","user":{"login":"mattxwang","id":14893287,"node_id":"MDQ6VXNlcjE0ODkzMjg3","avatar_url":"https://avatars.githubusercontent.com/u/14893287?v=4","gravatar_id":"","url":"https://api.github.com/users/mattxwang","html_url":"https://github.com/mattxwang","followers_url":"https://api.github.com/users/mattxwang/followers","following_url":"https://api.github.com/users/mattxwang/following{/other_user}","gists_url":"https://api.github.com/users/mattxwang/gists{/gist_id}","starred_url":"https://api.github.com/users/mattxwang/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/mattxwang/subscriptions","organizations_url":"https://api.github.com/users/mattxwang/orgs","repos_url":"https://api.github.com/users/mattxwang/repos","events_url":"https://api.github.com/users/mattxwang/events{/privacy}","received_events_url":"https://api.github.com/users/mattxwang/received_events","type":"User","site_admin":false},"labels":[{"id":1408021965,"node_id":"MDU6TGFiZWwxNDA4MDIxOTY1","url":"https://api.github.com/repos/uclaacm/teach-la-website/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"}],"state":"open","locked":false,"assignee":null,"assignees":[],"milestone":null,"comments":0,"created_at":"2020-10-22T23:23:28Z","updated_at":"2020-10-22T23:23:28Z","closed_at":null,"author_association":"COLLABORATOR","active_lock_reason":null,"body":"@Gautier404 mentioned that not all of our images (especially our vector art) have alt text - we should take a closer look at our existing images and how descriptive our alt texts are!","reactions":{"url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/127/reactions","total_count":1,"+1":1,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/teach-la-website/issues/127/timeline","performed_via_github_app":null,"state_reason":null,"score":1}],"project":{"name":"teach-la-website","description":"🌱a website showcasing how awesome Teach LA is! ","link":"https://teachla.uclaacm.com","repo":"https://github.com/uclaacm/teach-la-website","lang":"HTML","topics":["education","jekyll","teach-la"],"image":"/committee-logos/teachla-logo.png","alt":"ACM Teach LA Logo"},"repoURL":"https://api.github.com/repos/uclaacm/teach-la-website"},{"issues":[{"url":"https://api.github.com/repos/uclaacm/bruin-quest-website/issues/135","repository_url":"https://api.github.com/repos/uclaacm/bruin-quest-website","labels_url":"https://api.github.com/repos/uclaacm/bruin-quest-website/issues/135/labels{/name}","comments_url":"https://api.github.com/repos/uclaacm/bruin-quest-website/issues/135/comments","events_url":"https://api.github.com/repos/uclaacm/bruin-quest-website/issues/135/events","html_url":"https://github.com/uclaacm/bruin-quest-website/issues/135","id":741315635,"node_id":"MDU6SXNzdWU3NDEzMTU2MzU=","number":135,"title":"Error messages in Team Registration","user":{"login":"nikilrselvam","id":45131126,"node_id":"MDQ6VXNlcjQ1MTMxMTI2","avatar_url":"https://avatars.githubusercontent.com/u/45131126?v=4","gravatar_id":"","url":"https://api.github.com/users/nikilrselvam","html_url":"https://github.com/nikilrselvam","followers_url":"https://api.github.com/users/nikilrselvam/followers","following_url":"https://api.github.com/users/nikilrselvam/following{/other_user}","gists_url":"https://api.github.com/users/nikilrselvam/gists{/gist_id}","starred_url":"https://api.github.com/users/nikilrselvam/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/nikilrselvam/subscriptions","organizations_url":"https://api.github.com/users/nikilrselvam/orgs","repos_url":"https://api.github.com/users/nikilrselvam/repos","events_url":"https://api.github.com/users/nikilrselvam/events{/privacy}","received_events_url":"https://api.github.com/users/nikilrselvam/received_events","type":"User","site_admin":false},"labels":[{"id":2259332854,"node_id":"MDU6TGFiZWwyMjU5MzMyODU0","url":"https://api.github.com/repos/uclaacm/bruin-quest-website/labels/good%20first%20issue","name":"good first issue","color":"7057ff","default":true,"description":"Good for newcomers"}],"state":"open","locked":true,"assignee":null,"assignees":[],"milestone":null,"comments":0,"created_at":"2020-11-12T06:21:23Z","updated_at":"2020-11-12T06:21:23Z","closed_at":null,"author_association":"NONE","active_lock_reason":null,"body":"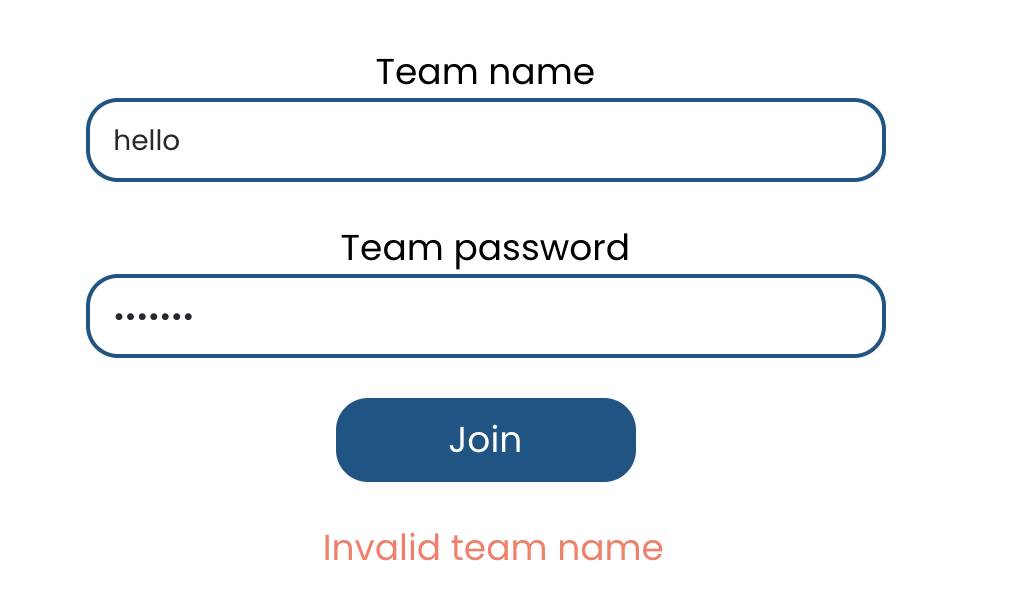\r\nWhen entering a team name that has already been taken, it might be better to explicitly say something along the lines of `this name as been taken` as opposed to `invalid team name`, as the latter might be potentially confusing/misleading for users. ","reactions":{"url":"https://api.github.com/repos/uclaacm/bruin-quest-website/issues/135/reactions","total_count":0,"+1":0,"-1":0,"laugh":0,"hooray":0,"confused":0,"heart":0,"rocket":0,"eyes":0},"timeline_url":"https://api.github.com/repos/uclaacm/bruin-quest-website/issues/135/timeline","performed_via_github_app":null,"state_reason":null,"score":1}],"project":{"name":"bruin-quest-website","description":"Website for ACM Cyber x Hack x ICPC's Bruin Quest","repo":"https://github.com/uclaacm/bruin-quest-website","lang":"JavaScript","topics":[],"image":"/logo.png","alt":"ACM @ UCLA's Logo"},"repoURL":"https://api.github.com/repos/uclaacm/bruin-quest-website"}]
\ No newline at end of file
diff --git a/tsconfig.json b/tsconfig.json
index bdafa88..432a336 100644
--- a/tsconfig.json
+++ b/tsconfig.json
@@ -1,7 +1,11 @@
{
"compilerOptions": {
"target": "es5",
- "lib": ["dom", "dom.iterable", "esnext"],
+ "lib": [
+ "dom",
+ "dom.iterable",
+ "esnext"
+ ],
"allowJs": true,
"skipLibCheck": true,
"strict": true,
@@ -12,8 +16,18 @@
"moduleResolution": "node",
"resolveJsonModule": true,
"isolatedModules": true,
- "jsx": "preserve"
+ "jsx": "preserve",
+ "incremental": true
},
- "exclude": ["node_modules", ".next", "out"],
- "include": ["next-env.d.ts", "**/*.ts", "**/*.tsx", "**/*.js"]
+ "exclude": [
+ "node_modules",
+ ".next",
+ "out"
+ ],
+ "include": [
+ "next-env.d.ts",
+ "**/*.ts",
+ "**/*.tsx",
+ "**/*.js"
+ ]
}
diff --git a/yarn.lock b/yarn.lock
index 1c479f0..be46fa5 100644
--- a/yarn.lock
+++ b/yarn.lock
@@ -181,7 +181,7 @@
"@babel/traverse" "^7.16.7"
"@babel/types" "^7.16.7"
-"@babel/helper-plugin-utils@^7.0.0", "@babel/helper-plugin-utils@^7.10.4", "@babel/helper-plugin-utils@^7.12.13", "@babel/helper-plugin-utils@^7.14.5", "@babel/helper-plugin-utils@^7.16.7", "@babel/helper-plugin-utils@^7.8.0":
+"@babel/helper-plugin-utils@^7.0.0", "@babel/helper-plugin-utils@^7.10.4", "@babel/helper-plugin-utils@^7.12.13", "@babel/helper-plugin-utils@^7.16.7", "@babel/helper-plugin-utils@^7.8.0":
version "7.16.7"
resolved "https://registry.npmjs.org/@babel/helper-plugin-utils/-/helper-plugin-utils-7.16.7.tgz"
integrity sha512-Qg3Nk7ZxpgMrsox6HreY1ZNKdBq7K72tDSliA6dCl5f007jR4ne8iD5UzuNnCJH2xBf2BEEVGr+/OL6Gdp7RxA==
@@ -200,7 +200,7 @@
dependencies:
"@babel/types" "^7.16.7"
-"@babel/helper-validator-identifier@^7.14.9", "@babel/helper-validator-identifier@^7.16.7":
+"@babel/helper-validator-identifier@^7.16.7":
version "7.16.7"
resolved "https://registry.npmjs.org/@babel/helper-validator-identifier/-/helper-validator-identifier-7.16.7.tgz"
integrity sha512-hsEnFemeiW4D08A5gUAZxLBTXpZ39P+a+DGDsHw1yxqyQ/jzFEnxf5uTEGp+3bzAbNOxU1paTgYS4ECU/IgfDw==
@@ -287,13 +287,6 @@
dependencies:
"@babel/helper-plugin-utils" "^7.8.0"
-"@babel/plugin-syntax-jsx@7.14.5":
- version "7.14.5"
- resolved "https://registry.npmjs.org/@babel/plugin-syntax-jsx/-/plugin-syntax-jsx-7.14.5.tgz"
- integrity sha512-ohuFIsOMXJnbOMRfX7/w7LocdR6R7whhuRD4ax8IipLcLPlZGJKkBxgHp++U4N/vKyU16/YDQr2f5seajD3jIw==
- dependencies:
- "@babel/helper-plugin-utils" "^7.14.5"
-
"@babel/plugin-syntax-logical-assignment-operators@^7.8.3":
version "7.10.4"
resolved "https://registry.npmjs.org/@babel/plugin-syntax-logical-assignment-operators/-/plugin-syntax-logical-assignment-operators-7.10.4.tgz"
@@ -350,7 +343,7 @@
dependencies:
"@babel/helper-plugin-utils" "^7.16.7"
-"@babel/runtime@7.15.3", "@babel/runtime@^7.12.5":
+"@babel/runtime@^7.12.5":
version "7.15.3"
resolved "https://registry.npmjs.org/@babel/runtime/-/runtime-7.15.3.tgz"
integrity sha512-OvwMLqNXkCXSz1kSm58sEsNuhqOx/fKpnUnKnFB5v8uDda5bLNEHNgKPvhDN6IU0LDcnHQ90LlJ0Q6jnyBSIBA==
@@ -414,14 +407,6 @@
debug "^4.1.0"
globals "^11.1.0"
-"@babel/types@7.15.0":
- version "7.15.0"
- resolved "https://registry.npmjs.org/@babel/types/-/types-7.15.0.tgz"
- integrity sha512-OBvfqnllOIdX4ojTHpwZbpvz4j3EWyjkZEdmjH0/cgsd6QOdSgU8rLSk6ard/pcW7rlmjdVSX/AWOaORR1uNOQ==
- dependencies:
- "@babel/helper-validator-identifier" "^7.14.9"
- to-fast-properties "^2.0.0"
-
"@babel/types@^7.0.0", "@babel/types@^7.16.7", "@babel/types@^7.3.0", "@babel/types@^7.3.3":
version "7.16.7"
resolved "https://registry.npmjs.org/@babel/types/-/types-7.16.7.tgz"
@@ -466,26 +451,6 @@
minimatch "^3.0.4"
strip-json-comments "^3.1.1"
-"@hapi/accept@5.0.2":
- version "5.0.2"
- resolved "https://registry.npmjs.org/@hapi/accept/-/accept-5.0.2.tgz"
- integrity sha512-CmzBx/bXUR8451fnZRuZAJRlzgm0Jgu5dltTX/bszmR2lheb9BpyN47Q1RbaGTsvFzn0PXAEs+lXDKfshccYZw==
- dependencies:
- "@hapi/boom" "9.x.x"
- "@hapi/hoek" "9.x.x"
-
-"@hapi/boom@9.x.x":
- version "9.1.2"
- resolved "https://registry.npmjs.org/@hapi/boom/-/boom-9.1.2.tgz"
- integrity sha512-uJEJtiNHzKw80JpngDGBCGAmWjBtzxDCz17A9NO2zCi8LLBlb5Frpq4pXwyN+2JQMod4pKz5BALwyneCgDg89Q==
- dependencies:
- "@hapi/hoek" "9.x.x"
-
-"@hapi/hoek@9.x.x":
- version "9.2.0"
- resolved "https://registry.npmjs.org/@hapi/hoek/-/hoek-9.2.0.tgz"
- integrity sha512-sqKVVVOe5ivCaXDWivIJYVSaEgdQK9ul7a4Kity5Iw7u9+wBAPbX1RMSnLLmp7O4Vzj0WOWwMAJsTL00xwaNug==
-
"@humanwhocodes/config-array@^0.5.0":
version "0.5.0"
resolved "https://registry.npmjs.org/@humanwhocodes/config-array/-/config-array-0.5.0.tgz"
@@ -739,15 +704,10 @@
"@jridgewell/resolve-uri" "^3.0.3"
sourcemap-codec "1.4.8"
-"@napi-rs/triples@^1.0.3":
- version "1.1.0"
- resolved "https://registry.npmjs.org/@napi-rs/triples/-/triples-1.1.0.tgz"
- integrity sha512-XQr74QaLeMiqhStEhLn1im9EOMnkypp7MZOwQhGzqp2Weu5eQJbpPxWxixxlYRKWPOmJjsk6qYfYH9kq43yc2w==
-
-"@next/env@11.1.3":
- version "11.1.3"
- resolved "https://registry.npmjs.org/@next/env/-/env-11.1.3.tgz"
- integrity sha512-5+vaeooJuWmICSlmVaAC8KG3O8hwKasACVfkHj58xQuCB5SW0TKW3hWxgxkBuefMBn1nM0yEVPKokXCsYjBtng==
+"@next/env@12.3.4":
+ version "12.3.4"
+ resolved "https://registry.yarnpkg.com/@next/env/-/env-12.3.4.tgz#c787837d36fcad75d72ff8df6b57482027d64a47"
+ integrity sha512-H/69Lc5Q02dq3o+dxxy5O/oNxFsZpdL6WREtOOtOM1B/weonIwDXkekr1KV5DPVPr12IHFPrMrcJQ6bgPMfn7A==
"@next/eslint-plugin-next@^12.0.10":
version "12.0.10"
@@ -756,59 +716,70 @@
dependencies:
glob "7.1.7"
-"@next/polyfill-module@11.1.3":
- version "11.1.3"
- resolved "https://registry.npmjs.org/@next/polyfill-module/-/polyfill-module-11.1.3.tgz"
- integrity sha512-7yr9cr4a0SrBoVE8psxXWK1wTFc8UzsY8Wc2cWGL7qA0hgtqACHaXC47M1ByJB410hFZenGrpE+KFaT1unQMyw==
+"@next/swc-android-arm-eabi@12.3.4":
+ version "12.3.4"
+ resolved "https://registry.yarnpkg.com/@next/swc-android-arm-eabi/-/swc-android-arm-eabi-12.3.4.tgz#fd1c2dafe92066c6120761c6a39d19e666dc5dd0"
+ integrity sha512-cM42Cw6V4Bz/2+j/xIzO8nK/Q3Ly+VSlZJTa1vHzsocJRYz8KT6MrreXaci2++SIZCF1rVRCDgAg5PpqRibdIA==
-"@next/react-dev-overlay@11.1.3":
- version "11.1.3"
- resolved "https://registry.npmjs.org/@next/react-dev-overlay/-/react-dev-overlay-11.1.3.tgz"
- integrity sha512-zIwtMliSUR+IKl917ToFNB+0fD7bI5kYMdjHU/UEKpfIXAZPnXRHHISCvPDsczlr+bRsbjlUFW1CsNiuFedeuQ==
- dependencies:
- "@babel/code-frame" "7.12.11"
- anser "1.4.9"
- chalk "4.0.0"
- classnames "2.2.6"
- css.escape "1.5.1"
- data-uri-to-buffer "3.0.1"
- platform "1.3.6"
- shell-quote "1.7.2"
- source-map "0.8.0-beta.0"
- stacktrace-parser "0.1.10"
- strip-ansi "6.0.0"
-
-"@next/react-refresh-utils@11.1.3":
- version "11.1.3"
- resolved "https://registry.npmjs.org/@next/react-refresh-utils/-/react-refresh-utils-11.1.3.tgz"
- integrity sha512-144kD8q2nChw67V3AJJlPQ6NUJVFczyn10bhTynn9o2rY5DEnkzuBipcyMuQl2DqfxMkV7sn+yOCOYbrLCk9zg==
-
-"@next/swc-darwin-arm64@11.1.3":
- version "11.1.3"
- resolved "https://registry.yarnpkg.com/@next/swc-darwin-arm64/-/swc-darwin-arm64-11.1.3.tgz#062eb7871048fdb313304e42ace5f91402dbc39f"
- integrity sha512-TwP4krjhs+uU9pesDYCShEXZrLSbJr78p12e7XnLBBaNf20SgWLlVmQUT9gX9KbWan5V0sUbJfmcS8MRNHgYuA==
-
-"@next/swc-darwin-x64@11.1.3":
- version "11.1.3"
- resolved "https://registry.npmjs.org/@next/swc-darwin-x64/-/swc-darwin-x64-11.1.3.tgz"
- integrity sha512-ZSWmkg/PxccHFNUSeBdrfaH8KwSkoeUtewXKvuYYt7Ph0yRsbqSyNIvhUezDua96lApiXXq6EL2d1THfeWomvw==
-
-"@next/swc-linux-x64-gnu@11.1.3":
- version "11.1.3"
- resolved "https://registry.yarnpkg.com/@next/swc-linux-x64-gnu/-/swc-linux-x64-gnu-11.1.3.tgz#40030577e6ee272afb0080b45468bea73208f46d"
- integrity sha512-PrTBN0iZudAuj4jSbtXcdBdmfpaDCPIneG4Oms4zcs93KwMgLhivYW082Mvlgx9QVEiRm7+RkFpIVtG/i7JitA==
-
-"@next/swc-win32-x64-msvc@11.1.3":
- version "11.1.3"
- resolved "https://registry.yarnpkg.com/@next/swc-win32-x64-msvc/-/swc-win32-x64-msvc-11.1.3.tgz#2951cbc127f6ea57032a241fb94439cddb5d2482"
- integrity sha512-mRwbscVjRoHk+tDY7XbkT5d9FCwujFIQJpGp0XNb1i5OHCSDO8WW/C9cLEWS4LxKRbIZlTLYg1MTXqLQkvva8w==
-
-"@node-rs/helper@1.2.1":
- version "1.2.1"
- resolved "https://registry.npmjs.org/@node-rs/helper/-/helper-1.2.1.tgz"
- integrity sha512-R5wEmm8nbuQU0YGGmYVjEc0OHtYsuXdpRG+Ut/3wZ9XAvQWyThN08bTh2cBJgoZxHQUPtvRfeQuxcAgLuiBISg==
- dependencies:
- "@napi-rs/triples" "^1.0.3"
+"@next/swc-android-arm64@12.3.4":
+ version "12.3.4"
+ resolved "https://registry.yarnpkg.com/@next/swc-android-arm64/-/swc-android-arm64-12.3.4.tgz#11a146dae7b8bca007239b21c616e83f77b19ed4"
+ integrity sha512-5jf0dTBjL+rabWjGj3eghpLUxCukRhBcEJgwLedewEA/LJk2HyqCvGIwj5rH+iwmq1llCWbOky2dO3pVljrapg==
+
+"@next/swc-darwin-arm64@12.3.4":
+ version "12.3.4"
+ resolved "https://registry.yarnpkg.com/@next/swc-darwin-arm64/-/swc-darwin-arm64-12.3.4.tgz#14ac8357010c95e67327f47082af9c9d75d5be79"
+ integrity sha512-DqsSTd3FRjQUR6ao0E1e2OlOcrF5br+uegcEGPVonKYJpcr0MJrtYmPxd4v5T6UCJZ+XzydF7eQo5wdGvSZAyA==
+
+"@next/swc-darwin-x64@12.3.4":
+ version "12.3.4"
+ resolved "https://registry.yarnpkg.com/@next/swc-darwin-x64/-/swc-darwin-x64-12.3.4.tgz#e7dc63cd2ac26d15fb84d4d2997207fb9ba7da0f"
+ integrity sha512-PPF7tbWD4k0dJ2EcUSnOsaOJ5rhT3rlEt/3LhZUGiYNL8KvoqczFrETlUx0cUYaXe11dRA3F80Hpt727QIwByQ==
+
+"@next/swc-freebsd-x64@12.3.4":
+ version "12.3.4"
+ resolved "https://registry.yarnpkg.com/@next/swc-freebsd-x64/-/swc-freebsd-x64-12.3.4.tgz#fe7ceec58746fdf03f1fcb37ec1331c28e76af93"
+ integrity sha512-KM9JXRXi/U2PUM928z7l4tnfQ9u8bTco/jb939pdFUHqc28V43Ohd31MmZD1QzEK4aFlMRaIBQOWQZh4D/E5lQ==
+
+"@next/swc-linux-arm-gnueabihf@12.3.4":
+ version "12.3.4"
+ resolved "https://registry.yarnpkg.com/@next/swc-linux-arm-gnueabihf/-/swc-linux-arm-gnueabihf-12.3.4.tgz#d7016934d02bfc8bd69818ffb0ae364b77b17af7"
+ integrity sha512-3zqD3pO+z5CZyxtKDTnOJ2XgFFRUBciOox6EWkoZvJfc9zcidNAQxuwonUeNts6Xbm8Wtm5YGIRC0x+12YH7kw==
+
+"@next/swc-linux-arm64-gnu@12.3.4":
+ version "12.3.4"
+ resolved "https://registry.yarnpkg.com/@next/swc-linux-arm64-gnu/-/swc-linux-arm64-gnu-12.3.4.tgz#43a7bc409b03487bff5beb99479cacdc7bd29af5"
+ integrity sha512-kiX0vgJGMZVv+oo1QuObaYulXNvdH/IINmvdZnVzMO/jic/B8EEIGlZ8Bgvw8LCjH3zNVPO3mGrdMvnEEPEhKA==
+
+"@next/swc-linux-arm64-musl@12.3.4":
+ version "12.3.4"
+ resolved "https://registry.yarnpkg.com/@next/swc-linux-arm64-musl/-/swc-linux-arm64-musl-12.3.4.tgz#4d1db6de6dc982b974cd1c52937111e3e4a34bd3"
+ integrity sha512-EETZPa1juczrKLWk5okoW2hv7D7WvonU+Cf2CgsSoxgsYbUCZ1voOpL4JZTOb6IbKMDo6ja+SbY0vzXZBUMvkQ==
+
+"@next/swc-linux-x64-gnu@12.3.4":
+ version "12.3.4"
+ resolved "https://registry.yarnpkg.com/@next/swc-linux-x64-gnu/-/swc-linux-x64-gnu-12.3.4.tgz#c3b414d77bab08b35f7dd8943d5586f0adb15e38"
+ integrity sha512-4csPbRbfZbuWOk3ATyWcvVFdD9/Rsdq5YHKvRuEni68OCLkfy4f+4I9OBpyK1SKJ00Cih16NJbHE+k+ljPPpag==
+
+"@next/swc-linux-x64-musl@12.3.4":
+ version "12.3.4"
+ resolved "https://registry.yarnpkg.com/@next/swc-linux-x64-musl/-/swc-linux-x64-musl-12.3.4.tgz#187a883ec09eb2442a5ebf126826e19037313c61"
+ integrity sha512-YeBmI+63Ro75SUiL/QXEVXQ19T++58aI/IINOyhpsRL1LKdyfK/35iilraZEFz9bLQrwy1LYAR5lK200A9Gjbg==
+
+"@next/swc-win32-arm64-msvc@12.3.4":
+ version "12.3.4"
+ resolved "https://registry.yarnpkg.com/@next/swc-win32-arm64-msvc/-/swc-win32-arm64-msvc-12.3.4.tgz#89befa84e453ed2ef9a888f375eba565a0fde80b"
+ integrity sha512-Sd0qFUJv8Tj0PukAYbCCDbmXcMkbIuhnTeHm9m4ZGjCf6kt7E/RMs55Pd3R5ePjOkN7dJEuxYBehawTR/aPDSQ==
+
+"@next/swc-win32-ia32-msvc@12.3.4":
+ version "12.3.4"
+ resolved "https://registry.yarnpkg.com/@next/swc-win32-ia32-msvc/-/swc-win32-ia32-msvc-12.3.4.tgz#cb50c08f0e40ead63642a7f269f0c8254261f17c"
+ integrity sha512-rt/vv/vg/ZGGkrkKcuJ0LyliRdbskQU+91bje+PgoYmxTZf/tYs6IfbmgudBJk6gH3QnjHWbkphDdRQrseRefQ==
+
+"@next/swc-win32-x64-msvc@12.3.4":
+ version "12.3.4"
+ resolved "https://registry.yarnpkg.com/@next/swc-win32-x64-msvc/-/swc-win32-x64-msvc-12.3.4.tgz#d28ea15a72cdcf96201c60a43e9630cd7fda168f"
+ integrity sha512-DQ20JEfTBZAgF8QCjYfJhv2/279M6onxFjdG/+5B0Cyj00/EdBxiWb2eGGFgQhrBbNv/lsvzFbbi0Ptf8Vw/bg==
"@nodelib/fs.scandir@2.1.4":
version "2.1.4"
@@ -956,6 +927,13 @@
dependencies:
"@sinonjs/commons" "^1.7.0"
+"@swc/helpers@0.4.11":
+ version "0.4.11"
+ resolved "https://registry.yarnpkg.com/@swc/helpers/-/helpers-0.4.11.tgz#db23a376761b3d31c26502122f349a21b592c8de"
+ integrity sha512-rEUrBSGIoSFuYxwBYtlUFMlE2CwGhmW+w9355/5oduSw8e5h2+Tj4UrAGNNgP9915++wj5vkQo0UuOBqOAq4nw==
+ dependencies:
+ tslib "^2.4.0"
+
"@testing-library/dom@^8.0.0":
version "8.11.1"
resolved "https://registry.npmjs.org/@testing-library/dom/-/dom-8.11.1.tgz"
@@ -1256,11 +1234,6 @@ ajv@^8.0.1:
require-from-string "^2.0.2"
uri-js "^4.2.2"
-anser@1.4.9:
- version "1.4.9"
- resolved "https://registry.npmjs.org/anser/-/anser-1.4.9.tgz"
- integrity sha512-AI+BjTeGt2+WFk4eWcqbQ7snZpDBt8SaLlj0RT2h5xfdWaiy51OjYvqwMrNzJLGy8iOAL6nKDITWO+rd4MkYEA==
-
ansi-colors@^4.1.1:
version "4.1.1"
resolved "https://registry.npmjs.org/ansi-colors/-/ansi-colors-4.1.1.tgz"
@@ -1273,7 +1246,7 @@ ansi-escapes@^4.2.1, ansi-escapes@^4.3.0, ansi-escapes@^4.3.1:
dependencies:
type-fest "^0.21.3"
-ansi-regex@^5.0.0, ansi-regex@^5.0.1:
+ansi-regex@^5.0.1:
version "5.0.1"
resolved "https://registry.npmjs.org/ansi-regex/-/ansi-regex-5.0.1.tgz"
integrity sha512-quJQXlTSUGL2LH9SUXo8VwsY4soanhgo6LNSm84E1LBcE8s3O0wpdiRzyR9z/ZZJMlMWv37qOOb9pdJlMUEKFQ==
@@ -1307,7 +1280,7 @@ ansi-styles@^6.0.0:
resolved "https://registry.npmjs.org/ansi-styles/-/ansi-styles-6.1.0.tgz"
integrity sha512-VbqNsoz55SYGczauuup0MFUyXNQviSpFTj1RQtFzmQLk18qbVSpTFFGMT293rmDaQuKCT6InmbuEyUne4mTuxQ==
-anymatch@^3.0.3, anymatch@~3.1.1:
+anymatch@^3.0.3:
version "3.1.2"
resolved "https://registry.npmjs.org/anymatch/-/anymatch-3.1.2.tgz"
integrity sha512-P43ePfOAIupkguHUycrc4qJ9kz8ZiuOUijaETwX7THt0Y/GNK7v0aa8rY816xWjZ7rJdA5XdMcpVFTKMq+RvWg==
@@ -1327,11 +1300,6 @@ aria-query@^5.0.0:
resolved "https://registry.npmjs.org/aria-query/-/aria-query-5.0.0.tgz"
integrity sha512-V+SM7AbUwJ+EBnB8+DXs0hPZHO0W6pqBcc0dW90OwtVG02PswOu/teuARoLQjdDOH+t9pJgGnW5/Qmouf3gPJg==
-array-filter@^1.0.0:
- version "1.0.0"
- resolved "https://registry.npmjs.org/array-filter/-/array-filter-1.0.0.tgz"
- integrity sha1-uveeYubvTCpMC4MSMtr/7CUfnYM=
-
array-includes@^3.1.2, array-includes@^3.1.4:
version "3.1.4"
resolved "https://registry.npmjs.org/array-includes/-/array-includes-3.1.4.tgz"
@@ -1366,39 +1334,6 @@ array.prototype.flatmap@^1.2.5:
define-properties "^1.1.3"
es-abstract "^1.19.0"
-asn1.js@^5.2.0:
- version "5.4.1"
- resolved "https://registry.npmjs.org/asn1.js/-/asn1.js-5.4.1.tgz"
- integrity sha512-+I//4cYPccV8LdmBLiX8CYvf9Sp3vQsrqu2QNXRcrbiWvcx/UdlFiqUJJzxRQxgsZmvhXhn4cSKeSmoFjVdupA==
- dependencies:
- bn.js "^4.0.0"
- inherits "^2.0.1"
- minimalistic-assert "^1.0.0"
- safer-buffer "^2.1.0"
-
-assert@2.0.0:
- version "2.0.0"
- resolved "https://registry.npmjs.org/assert/-/assert-2.0.0.tgz"
- integrity sha512-se5Cd+js9dXJnu6Ag2JFc00t+HmHOen+8Q+L7O9zI0PqQXr20uk2J0XQqMxZEeo5U50o8Nvmmx7dZrl+Ufr35A==
- dependencies:
- es6-object-assign "^1.1.0"
- is-nan "^1.2.1"
- object-is "^1.0.1"
- util "^0.12.0"
-
-assert@^1.1.1:
- version "1.5.0"
- resolved "https://registry.npmjs.org/assert/-/assert-1.5.0.tgz"
- integrity sha512-EDsgawzwoun2CZkCgtxJbv392v4nbk9XDD06zI+kQYoBM/3RBWLlEyJARDOmhAAosBjWACEkKL6S+lIZtcAubA==
- dependencies:
- object-assign "^4.1.1"
- util "0.10.3"
-
-ast-types@0.13.2:
- version "0.13.2"
- resolved "https://registry.npmjs.org/ast-types/-/ast-types-0.13.2.tgz"
- integrity sha512-uWMHxJxtfj/1oZClOxDEV1sQ1HCDkA4MG8Gr69KKeBjEVH0R84WlejZ0y2DcwyBlpAEMltmVYkVgqfLFb2oyiA==
-
astral-regex@^2.0.0:
version "2.0.0"
resolved "https://registry.npmjs.org/astral-regex/-/astral-regex-2.0.0.tgz"
@@ -1409,13 +1344,6 @@ asynckit@^0.4.0:
resolved "https://registry.npmjs.org/asynckit/-/asynckit-0.4.0.tgz"
integrity sha1-x57Zf380y48robyXkLzDZkdLS3k=
-available-typed-arrays@^1.0.2:
- version "1.0.2"
- resolved "https://registry.npmjs.org/available-typed-arrays/-/available-typed-arrays-1.0.2.tgz"
- integrity sha512-XWX3OX8Onv97LMk/ftVyBibpGwY5a8SmuxZPzeOxqmuEqUCOM9ZE+uIaD1VNJ5QnvU2UQusvmKbuM1FR8QWGfQ==
- dependencies:
- array-filter "^1.0.0"
-
babel-jest@^27.4.6:
version "27.4.6"
resolved "https://registry.yarnpkg.com/babel-jest/-/babel-jest-27.4.6.tgz#4d024e69e241cdf4f396e453a07100f44f7ce314"
@@ -1482,36 +1410,11 @@ balanced-match@^1.0.0:
resolved "https://registry.npmjs.org/balanced-match/-/balanced-match-1.0.2.tgz"
integrity sha512-3oSeUO0TMV67hN1AmbXsK4yaqU7tjiHlbxRDZOpH0KW9+CeX4bRAaX0Anxt0tx2MrpRpWwQaPwIlISEJhYU5Pw==
-base64-js@^1.0.2:
- version "1.5.1"
- resolved "https://registry.npmjs.org/base64-js/-/base64-js-1.5.1.tgz"
- integrity sha512-AKpaYlHn8t4SVbOHCy+b5+KKgvR4vrsD8vbvrbiQJps7fKDTkjkDry6ji0rUJjC0kzbNePLwzxq8iypo41qeWA==
-
before-after-hook@^2.2.0:
version "2.2.2"
resolved "https://registry.npmjs.org/before-after-hook/-/before-after-hook-2.2.2.tgz"
integrity sha512-3pZEU3NT5BFUo/AD5ERPWOgQOCZITni6iavr5AUw5AUwQjMlI0kzu5btnyD39AF0gUEsDPwJT+oY1ORBJijPjQ==
-big.js@^5.2.2:
- version "5.2.2"
- resolved "https://registry.npmjs.org/big.js/-/big.js-5.2.2.tgz"
- integrity sha512-vyL2OymJxmarO8gxMr0mhChsO9QGwhynfuu4+MHTAW6czfq9humCB7rKpUjDd9YUiDPU4mzpyupFSvOClAwbmQ==
-
-binary-extensions@^2.0.0:
- version "2.2.0"
- resolved "https://registry.npmjs.org/binary-extensions/-/binary-extensions-2.2.0.tgz"
- integrity sha512-jDctJ/IVQbZoJykoeHbhXpOlNBqGNcwXJKJog42E5HDPUwQTSdjCHdihjj0DlnheQ7blbT6dHOafNAiS8ooQKA==
-
-bn.js@^4.0.0, bn.js@^4.1.0, bn.js@^4.11.9:
- version "4.12.0"
- resolved "https://registry.npmjs.org/bn.js/-/bn.js-4.12.0.tgz"
- integrity sha512-c98Bf3tPniI+scsdk237ku1Dc3ujXQTSgyiPUDEOe7tRkhrqridvh8klBv0HCEso1OLOYcHuCv/cS6DNxKH+ZA==
-
-bn.js@^5.0.0, bn.js@^5.1.1:
- version "5.2.0"
- resolved "https://registry.npmjs.org/bn.js/-/bn.js-5.2.0.tgz"
- integrity sha512-D7iWRBvnZE8ecXiLj/9wbxH7Tk79fAh8IHaTNq1RWRixsS02W+5qS+iE9yq6RYl0asXx5tw0bLhmT5pIfbSquw==
-
brace-expansion@^1.1.7:
version "1.1.11"
resolved "https://registry.npmjs.org/brace-expansion/-/brace-expansion-1.1.11.tgz"
@@ -1520,95 +1423,18 @@ brace-expansion@^1.1.7:
balanced-match "^1.0.0"
concat-map "0.0.1"
-braces@^3.0.1, braces@~3.0.2:
+braces@^3.0.1:
version "3.0.2"
resolved "https://registry.npmjs.org/braces/-/braces-3.0.2.tgz"
integrity sha512-b8um+L1RzM3WDSzvhm6gIz1yfTbBt6YTlcEKAvsmqCZZFw46z626lVj9j1yEPW33H5H+lBQpZMP1k8l+78Ha0A==
dependencies:
fill-range "^7.0.1"
-brorand@^1.0.1, brorand@^1.1.0:
- version "1.1.0"
- resolved "https://registry.npmjs.org/brorand/-/brorand-1.1.0.tgz"
- integrity sha1-EsJe/kCkXjwyPrhnWgoM5XsiNx8=
-
browser-process-hrtime@^1.0.0:
version "1.0.0"
resolved "https://registry.npmjs.org/browser-process-hrtime/-/browser-process-hrtime-1.0.0.tgz"
integrity sha512-9o5UecI3GhkpM6DrXr69PblIuWxPKk9Y0jHBRhdocZ2y7YECBFCsHm79Pr3OyR2AvjhDkabFJaDJMYRazHgsow==
-browserify-aes@^1.0.0, browserify-aes@^1.0.4:
- version "1.2.0"
- resolved "https://registry.npmjs.org/browserify-aes/-/browserify-aes-1.2.0.tgz"
- integrity sha512-+7CHXqGuspUn/Sl5aO7Ea0xWGAtETPXNSAjHo48JfLdPWcMng33Xe4znFvQweqc/uzk5zSOI3H52CYnjCfb5hA==
- dependencies:
- buffer-xor "^1.0.3"
- cipher-base "^1.0.0"
- create-hash "^1.1.0"
- evp_bytestokey "^1.0.3"
- inherits "^2.0.1"
- safe-buffer "^5.0.1"
-
-browserify-cipher@^1.0.0:
- version "1.0.1"
- resolved "https://registry.npmjs.org/browserify-cipher/-/browserify-cipher-1.0.1.tgz"
- integrity sha512-sPhkz0ARKbf4rRQt2hTpAHqn47X3llLkUGn+xEJzLjwY8LRs2p0v7ljvI5EyoRO/mexrNunNECisZs+gw2zz1w==
- dependencies:
- browserify-aes "^1.0.4"
- browserify-des "^1.0.0"
- evp_bytestokey "^1.0.0"
-
-browserify-des@^1.0.0:
- version "1.0.2"
- resolved "https://registry.npmjs.org/browserify-des/-/browserify-des-1.0.2.tgz"
- integrity sha512-BioO1xf3hFwz4kc6iBhI3ieDFompMhrMlnDFC4/0/vd5MokpuAc3R+LYbwTA9A5Yc9pq9UYPqffKpW2ObuwX5A==
- dependencies:
- cipher-base "^1.0.1"
- des.js "^1.0.0"
- inherits "^2.0.1"
- safe-buffer "^5.1.2"
-
-browserify-rsa@^4.0.0, browserify-rsa@^4.0.1:
- version "4.1.0"
- resolved "https://registry.npmjs.org/browserify-rsa/-/browserify-rsa-4.1.0.tgz"
- integrity sha512-AdEER0Hkspgno2aR97SAf6vi0y0k8NuOpGnVH3O99rcA5Q6sh8QxcngtHuJ6uXwnfAXNM4Gn1Gb7/MV1+Ymbog==
- dependencies:
- bn.js "^5.0.0"
- randombytes "^2.0.1"
-
-browserify-sign@^4.0.0:
- version "4.2.1"
- resolved "https://registry.npmjs.org/browserify-sign/-/browserify-sign-4.2.1.tgz"
- integrity sha512-/vrA5fguVAKKAVTNJjgSm1tRQDHUU6DbwO9IROu/0WAzC8PKhucDSh18J0RMvVeHAn5puMd+QHC2erPRNf8lmg==
- dependencies:
- bn.js "^5.1.1"
- browserify-rsa "^4.0.1"
- create-hash "^1.2.0"
- create-hmac "^1.1.7"
- elliptic "^6.5.3"
- inherits "^2.0.4"
- parse-asn1 "^5.1.5"
- readable-stream "^3.6.0"
- safe-buffer "^5.2.0"
-
-browserify-zlib@0.2.0, browserify-zlib@^0.2.0:
- version "0.2.0"
- resolved "https://registry.npmjs.org/browserify-zlib/-/browserify-zlib-0.2.0.tgz"
- integrity sha512-Z942RysHXmJrhqk88FmKBVq/v5tqmSkDz7p54G/MGyjMnCFFnC79XWNbg+Vta8W6Wb2qtSZTSxIGkJrRpCFEiA==
- dependencies:
- pako "~1.0.5"
-
-browserslist@4.16.6:
- version "4.16.6"
- resolved "https://registry.npmjs.org/browserslist/-/browserslist-4.16.6.tgz"
- integrity sha512-Wspk/PqO+4W9qp5iUTJsa1B/QrYn1keNCcEP5OvP7WBwT4KaDly0uONYmC6Xa3Z5IqnUgS0KcgLYu1l74x0ZXQ==
- dependencies:
- caniuse-lite "^1.0.30001219"
- colorette "^1.2.2"
- electron-to-chromium "^1.3.723"
- escalade "^3.1.1"
- node-releases "^1.1.71"
-
browserslist@^4.17.5:
version "4.19.1"
resolved "https://registry.npmjs.org/browserslist/-/browserslist-4.19.1.tgz"
@@ -1632,38 +1458,6 @@ buffer-from@^1.0.0:
resolved "https://registry.npmjs.org/buffer-from/-/buffer-from-1.1.1.tgz"
integrity sha512-MQcXEUbCKtEo7bhqEs6560Hyd4XaovZlO/k9V3hjVUF/zwW7KBVdSK4gIt/bzwS9MbR5qob+F5jusZsb0YQK2A==
-buffer-xor@^1.0.3:
- version "1.0.3"
- resolved "https://registry.npmjs.org/buffer-xor/-/buffer-xor-1.0.3.tgz"
- integrity sha1-JuYe0UIvtw3ULm42cp7VHYVf6Nk=
-
-buffer@5.6.0:
- version "5.6.0"
- resolved "https://registry.npmjs.org/buffer/-/buffer-5.6.0.tgz"
- integrity sha512-/gDYp/UtU0eA1ys8bOs9J6a+E/KWIY+DZ+Q2WESNUA0jFRsJOc0SNUO6xJ5SGA1xueg3NL65W6s+NY5l9cunuw==
- dependencies:
- base64-js "^1.0.2"
- ieee754 "^1.1.4"
-
-buffer@^4.3.0:
- version "4.9.2"
- resolved "https://registry.npmjs.org/buffer/-/buffer-4.9.2.tgz"
- integrity sha512-xq+q3SRMOxGivLhBNaUdC64hDTQwejJ+H0T/NB1XMtTVEwNTrfFF3gAxiyW0Bu/xWEGhjVKgUcMhCrUy2+uCWg==
- dependencies:
- base64-js "^1.0.2"
- ieee754 "^1.1.4"
- isarray "^1.0.0"
-
-builtin-status-codes@^3.0.0:
- version "3.0.0"
- resolved "https://registry.npmjs.org/builtin-status-codes/-/builtin-status-codes-3.0.0.tgz"
- integrity sha1-hZgoeOIbmOHGZCXgPQF0eI9Wnug=
-
-bytes@3.1.0:
- version "3.1.0"
- resolved "https://registry.npmjs.org/bytes/-/bytes-3.1.0.tgz"
- integrity sha512-zauLjrfCG+xvoyaqLoV8bLVXXNGC4JqlxFCutSDWA6fJrTo2ZuvLYTqZ7aHBLZSMOopbzwv8f+wZcVzfVTI2Dg==
-
call-bind@^1.0.0, call-bind@^1.0.2:
version "1.0.2"
resolved "https://registry.npmjs.org/call-bind/-/call-bind-1.0.2.tgz"
@@ -1687,12 +1481,17 @@ camelcase@^6.2.0:
resolved "https://registry.npmjs.org/camelcase/-/camelcase-6.3.0.tgz"
integrity sha512-Gmy6FhYlCY7uOElZUSbxo2UCDH8owEk996gkbrpsgGtrJLM3J7jGxl9Ic7Qwwj4ivOE5AWZWRMecDdF7hqGjFA==
-caniuse-lite@^1.0.30001202, caniuse-lite@^1.0.30001219, caniuse-lite@^1.0.30001228, caniuse-lite@^1.0.30001286:
+caniuse-lite@^1.0.30001286:
version "1.0.30001295"
resolved "https://registry.npmjs.org/caniuse-lite/-/caniuse-lite-1.0.30001295.tgz"
integrity sha512-lSP16vcyC0FEy0R4ECc9duSPoKoZy+YkpGkue9G4D81OfPnliopaZrU10+qtPdT8PbGXad/PNx43TIQrOmJZSQ==
-chalk@2.4.2, chalk@^2.0.0:
+caniuse-lite@^1.0.30001406:
+ version "1.0.30001589"
+ resolved "https://registry.yarnpkg.com/caniuse-lite/-/caniuse-lite-1.0.30001589.tgz#7ad6dba4c9bf6561aec8291976402339dc157dfb"
+ integrity sha512-vNQWS6kI+q6sBlHbh71IIeC+sRwK2N3EDySc/updIGhIee2x5z00J4c1242/5/d6EpEMdOnk/m+6tuk4/tcsqg==
+
+chalk@^2.0.0:
version "2.4.2"
resolved "https://registry.npmjs.org/chalk/-/chalk-2.4.2.tgz"
integrity sha512-Mti+f9lpJNcwF4tWV8/OrTTtF1gZi+f8FqlyAdouralcFWFQWF2+NgCHShjkCb+IFBLq9buZwE1xckQU4peSuQ==
@@ -1701,14 +1500,6 @@ chalk@2.4.2, chalk@^2.0.0:
escape-string-regexp "^1.0.5"
supports-color "^5.3.0"
-chalk@4.0.0:
- version "4.0.0"
- resolved "https://registry.npmjs.org/chalk/-/chalk-4.0.0.tgz"
- integrity sha512-N9oWFcegS0sFr9oh1oz2d7Npos6vNoWW9HvtCg5N1KRFpUhaAhvTv5Y58g880fZaEYSNm3qDz8SU1UrGvp+n7A==
- dependencies:
- ansi-styles "^4.1.0"
- supports-color "^7.1.0"
-
chalk@^4.0.0, chalk@^4.1.0:
version "4.1.2"
resolved "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz"
@@ -1727,44 +1518,16 @@ char-regex@^2.0.0:
resolved "https://registry.yarnpkg.com/char-regex/-/char-regex-2.0.0.tgz#16f98f3f874edceddd300fda5d58df380a7641a6"
integrity sha512-oGu2QekBMXgyQNWPDRQ001bjvDnZe4/zBTz37TMbiKz1NbNiyiH5hRkobe7npRN6GfbGbxMYFck/vQ1r9c1VMA==
-chokidar@3.5.1:
- version "3.5.1"
- resolved "https://registry.npmjs.org/chokidar/-/chokidar-3.5.1.tgz"
- integrity sha512-9+s+Od+W0VJJzawDma/gvBNQqkTiqYTWLuZoyAsivsI4AaWTCzHG06/TMjsf1cYe9Cb97UCEhjz7HvnPk2p/tw==
- dependencies:
- anymatch "~3.1.1"
- braces "~3.0.2"
- glob-parent "~5.1.0"
- is-binary-path "~2.1.0"
- is-glob "~4.0.1"
- normalize-path "~3.0.0"
- readdirp "~3.5.0"
- optionalDependencies:
- fsevents "~2.3.1"
-
ci-info@^3.2.0:
version "3.3.0"
resolved "https://registry.npmjs.org/ci-info/-/ci-info-3.3.0.tgz"
integrity sha512-riT/3vI5YpVH6/qomlDnJow6TBee2PBKSEpx3O32EGPYbWGIRsIlGRms3Sm74wYE1JMo8RnO04Hb12+v1J5ICw==
-cipher-base@^1.0.0, cipher-base@^1.0.1, cipher-base@^1.0.3:
- version "1.0.4"
- resolved "https://registry.npmjs.org/cipher-base/-/cipher-base-1.0.4.tgz"
- integrity sha512-Kkht5ye6ZGmwv40uUDZztayT2ThLQGfnj/T71N/XzeZeo3nf8foyW7zGTsPYkEya3m5f3cAypH+qe7YOrM1U2Q==
- dependencies:
- inherits "^2.0.1"
- safe-buffer "^5.0.1"
-
cjs-module-lexer@^1.0.0:
version "1.2.2"
resolved "https://registry.npmjs.org/cjs-module-lexer/-/cjs-module-lexer-1.2.2.tgz"
integrity sha512-cOU9usZw8/dXIXKtwa8pM0OTJQuJkxMN6w30csNRUerHfeQ5R6U3kkU/FtJeIf3M202OHfY2U8ccInBG7/xogA==
-classnames@2.2.6:
- version "2.2.6"
- resolved "https://registry.npmjs.org/classnames/-/classnames-2.2.6.tgz"
- integrity sha512-JR/iSQOSt+LQIWwrwEzJ9uk0xfN3mTVYMwt1Ir5mUcSN6pU+V4zQFFaJsclJbPuAUQH+yfWef6tm7l1quW3C8Q==
-
clean-stack@^2.0.0:
version "2.2.0"
resolved "https://registry.npmjs.org/clean-stack/-/clean-stack-2.2.0.tgz"
@@ -1836,11 +1599,6 @@ color-name@~1.1.4:
resolved "https://registry.npmjs.org/color-name/-/color-name-1.1.4.tgz"
integrity sha512-dOy+3AuW3a2wNbZHIuMZpTcgjGuLU/uBL/ubcZF9OXbDo8ff4O8yVp5Bf0efS8uEoYo5q4Fx7dY9OgQGXgAsQA==
-colorette@^1.2.2:
- version "1.2.2"
- resolved "https://registry.npmjs.org/colorette/-/colorette-1.2.2.tgz"
- integrity sha512-MKGMzyfeuutC/ZJ1cba9NqcNpfeqMUcYmyF1ZFY6/Cn7CNSAKx6a+s48sqLqyAiZuaP2TcqMhoo+dlwFnVxT9w==
-
colorette@^2.0.16:
version "2.0.16"
resolved "https://registry.npmjs.org/colorette/-/colorette-2.0.16.tgz"
@@ -1858,69 +1616,18 @@ commander@^8.3.0:
resolved "https://registry.npmjs.org/commander/-/commander-8.3.0.tgz"
integrity sha512-OkTL9umf+He2DZkUq8f8J9of7yL6RJKI24dVITBmNfZBmri9zYZQrKkuXiKhyfPSu8tUhnVBB1iKXevvnlR4Ww==
-commondir@^1.0.1:
- version "1.0.1"
- resolved "https://registry.npmjs.org/commondir/-/commondir-1.0.1.tgz"
- integrity sha1-3dgA2gxmEnOTzKWVDqloo6rxJTs=
-
concat-map@0.0.1:
version "0.0.1"
resolved "https://registry.npmjs.org/concat-map/-/concat-map-0.0.1.tgz"
integrity sha1-2Klr13/Wjfd5OnMDajug1UBdR3s=
-console-browserify@^1.1.0:
- version "1.2.0"
- resolved "https://registry.npmjs.org/console-browserify/-/console-browserify-1.2.0.tgz"
- integrity sha512-ZMkYO/LkF17QvCPqM0gxw8yUzigAOZOSWSHg91FH6orS7vcEj5dVZTidN2fQ14yBSdg97RqhSNwLUXInd52OTA==
-
-constants-browserify@1.0.0, constants-browserify@^1.0.0:
- version "1.0.0"
- resolved "https://registry.npmjs.org/constants-browserify/-/constants-browserify-1.0.0.tgz"
- integrity sha1-wguW2MYXdIqvHBYCF2DNJ/y4y3U=
-
-convert-source-map@1.7.0, convert-source-map@^1.4.0, convert-source-map@^1.6.0, convert-source-map@^1.7.0:
+convert-source-map@^1.4.0, convert-source-map@^1.6.0, convert-source-map@^1.7.0:
version "1.7.0"
resolved "https://registry.npmjs.org/convert-source-map/-/convert-source-map-1.7.0.tgz"
integrity sha512-4FJkXzKXEDB1snCFZlLP4gpC3JILicCpGbzG9f9G7tGqGCzETQ2hWPrcinA9oU4wtf2biUaEH5065UnMeR33oA==
dependencies:
safe-buffer "~5.1.1"
-core-util-is@~1.0.0:
- version "1.0.3"
- resolved "https://registry.npmjs.org/core-util-is/-/core-util-is-1.0.3.tgz"
- integrity sha512-ZQBvi1DcpJ4GDqanjucZ2Hj3wEO5pZDS89BWbkcrvdxksJorwUDDZamX9ldFkp9aw2lmBDLgkObEA4DWNJ9FYQ==
-
-create-ecdh@^4.0.0:
- version "4.0.4"
- resolved "https://registry.npmjs.org/create-ecdh/-/create-ecdh-4.0.4.tgz"
- integrity sha512-mf+TCx8wWc9VpuxfP2ht0iSISLZnt0JgWlrOKZiNqyUZWnjIaCIVNQArMHnCZKfEYRg6IM7A+NeJoN8gf/Ws0A==
- dependencies:
- bn.js "^4.1.0"
- elliptic "^6.5.3"
-
-create-hash@^1.1.0, create-hash@^1.1.2, create-hash@^1.2.0:
- version "1.2.0"
- resolved "https://registry.npmjs.org/create-hash/-/create-hash-1.2.0.tgz"
- integrity sha512-z00bCGNHDG8mHAkP7CtT1qVu+bFQUPjYq/4Iv3C3kWjTFV10zIjfSoeqXo9Asws8gwSHDGj/hl2u4OGIjapeCg==
- dependencies:
- cipher-base "^1.0.1"
- inherits "^2.0.1"
- md5.js "^1.3.4"
- ripemd160 "^2.0.1"
- sha.js "^2.4.0"
-
-create-hmac@^1.1.0, create-hmac@^1.1.4, create-hmac@^1.1.7:
- version "1.1.7"
- resolved "https://registry.npmjs.org/create-hmac/-/create-hmac-1.1.7.tgz"
- integrity sha512-MJG9liiZ+ogc4TzUwuvbER1JRdgvUFSB5+VR/g5h82fGaIRWMWddtKBHi7/sVhfjQZ6SehlyhvQYrcYkaUIpLg==
- dependencies:
- cipher-base "^1.0.3"
- create-hash "^1.1.0"
- inherits "^2.0.1"
- ripemd160 "^2.0.0"
- safe-buffer "^5.0.1"
- sha.js "^2.4.8"
-
cross-spawn@^7.0.2, cross-spawn@^7.0.3:
version "7.0.3"
resolved "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz"
@@ -1930,42 +1637,6 @@ cross-spawn@^7.0.2, cross-spawn@^7.0.3:
shebang-command "^2.0.0"
which "^2.0.1"
-crypto-browserify@3.12.0, crypto-browserify@^3.11.0:
- version "3.12.0"
- resolved "https://registry.npmjs.org/crypto-browserify/-/crypto-browserify-3.12.0.tgz"
- integrity sha512-fz4spIh+znjO2VjL+IdhEpRJ3YN6sMzITSBijk6FK2UvTqruSQW+/cCZTSNsMiZNvUeq0CqurF+dAbyiGOY6Wg==
- dependencies:
- browserify-cipher "^1.0.0"
- browserify-sign "^4.0.0"
- create-ecdh "^4.0.0"
- create-hash "^1.1.0"
- create-hmac "^1.1.0"
- diffie-hellman "^5.0.0"
- inherits "^2.0.1"
- pbkdf2 "^3.0.3"
- public-encrypt "^4.0.0"
- randombytes "^2.0.0"
- randomfill "^1.0.3"
-
-css.escape@1.5.1:
- version "1.5.1"
- resolved "https://registry.npmjs.org/css.escape/-/css.escape-1.5.1.tgz"
- integrity sha1-QuJ9T6BK4y+TGktNQZH6nN3ul8s=
-
-cssnano-preset-simple@^3.0.0:
- version "3.0.0"
- resolved "https://registry.npmjs.org/cssnano-preset-simple/-/cssnano-preset-simple-3.0.0.tgz"
- integrity sha512-vxQPeoMRqUT3c/9f0vWeVa2nKQIHFpogtoBvFdW4GQ3IvEJ6uauCP6p3Y5zQDLFcI7/+40FTgX12o7XUL0Ko+w==
- dependencies:
- caniuse-lite "^1.0.30001202"
-
-cssnano-simple@3.0.0:
- version "3.0.0"
- resolved "https://registry.npmjs.org/cssnano-simple/-/cssnano-simple-3.0.0.tgz"
- integrity sha512-oU3ueli5Dtwgh0DyeohcIEE00QVfbPR3HzyXdAl89SfnQG3y0/qcpfLVW+jPIh3/rgMZGwuW96rejZGaYE9eUg==
- dependencies:
- cssnano-preset-simple "^3.0.0"
-
cssom@^0.4.4:
version "0.4.4"
resolved "https://registry.npmjs.org/cssom/-/cssom-0.4.4.tgz"
@@ -1988,11 +1659,6 @@ csstype@^3.0.2:
resolved "https://registry.npmjs.org/csstype/-/csstype-3.0.8.tgz"
integrity sha512-jXKhWqXPmlUeoQnF/EhTtTl4C9SnrxSH/jZUih3jmO6lBKr99rP3/+FmrMj4EFpOXzMtXHAZkd3x0E6h6Fgflw==
-data-uri-to-buffer@3.0.1:
- version "3.0.1"
- resolved "https://registry.npmjs.org/data-uri-to-buffer/-/data-uri-to-buffer-3.0.1.tgz"
- integrity sha512-WboRycPNsVw3B3TL559F7kuBUM4d8CgMEvk6xEJlOp7OBPjt6G7z8WMWlD2rOFZLk6OYfFIUGsCOWzcQH9K2og==
-
data-urls@^2.0.0:
version "2.0.0"
resolved "https://registry.npmjs.org/data-urls/-/data-urls-2.0.0.tgz"
@@ -2002,13 +1668,6 @@ data-urls@^2.0.0:
whatwg-mimetype "^2.3.0"
whatwg-url "^8.0.0"
-debug@2, debug@^2.6.9:
- version "2.6.9"
- resolved "https://registry.npmjs.org/debug/-/debug-2.6.9.tgz"
- integrity sha512-bC7ElrdJaJnPbAP+1EotYvqZsb3ecl5wi6Bfi6BJTUcNowp6cvspg0jXznRTKDjm/E7AdgFBVeAPVMNcKGsHMA==
- dependencies:
- ms "2.0.0"
-
debug@4, debug@^4.0.1, debug@^4.1.0, debug@^4.1.1, debug@^4.3.1, debug@^4.3.3:
version "4.3.3"
resolved "https://registry.npmjs.org/debug/-/debug-4.3.3.tgz"
@@ -2016,6 +1675,13 @@ debug@4, debug@^4.0.1, debug@^4.1.0, debug@^4.1.1, debug@^4.3.1, debug@^4.3.3:
dependencies:
ms "2.1.2"
+debug@^2.6.9:
+ version "2.6.9"
+ resolved "https://registry.npmjs.org/debug/-/debug-2.6.9.tgz"
+ integrity sha512-bC7ElrdJaJnPbAP+1EotYvqZsb3ecl5wi6Bfi6BJTUcNowp6cvspg0jXznRTKDjm/E7AdgFBVeAPVMNcKGsHMA==
+ dependencies:
+ ms "2.0.0"
+
debug@^3.2.7:
version "3.2.7"
resolved "https://registry.npmjs.org/debug/-/debug-3.2.7.tgz"
@@ -2055,24 +1721,11 @@ delayed-stream@~1.0.0:
resolved "https://registry.npmjs.org/delayed-stream/-/delayed-stream-1.0.0.tgz"
integrity sha1-3zrhmayt+31ECqrgsp4icrJOxhk=
-depd@~1.1.2:
- version "1.1.2"
- resolved "https://registry.npmjs.org/depd/-/depd-1.1.2.tgz"
- integrity sha1-m81S4UwJd2PnSbJ0xDRu0uVgtak=
-
deprecation@^2.0.0, deprecation@^2.3.1:
version "2.3.1"
resolved "https://registry.npmjs.org/deprecation/-/deprecation-2.3.1.tgz"
integrity sha512-xmHIy4F3scKVwMsQ4WnVaS8bHOx0DmVwRywosKhaILI0ywMDWPtBSku2HNxRvF7jtwDRsoEwYQSfbxj8b7RlJQ==
-des.js@^1.0.0:
- version "1.0.1"
- resolved "https://registry.npmjs.org/des.js/-/des.js-1.0.1.tgz"
- integrity sha512-Q0I4pfFrv2VPd34/vfLrFOoRmlYj3OV50i7fskps1jZWK1kApMWWT9G6RRUeYedLcBDIhnSDaUvJMb3AhUlaEA==
- dependencies:
- inherits "^2.0.1"
- minimalistic-assert "^1.0.0"
-
detect-newline@^3.0.0:
version "3.1.0"
resolved "https://registry.npmjs.org/detect-newline/-/detect-newline-3.1.0.tgz"
@@ -2088,15 +1741,6 @@ diff-sequences@^27.5.1:
resolved "https://registry.yarnpkg.com/diff-sequences/-/diff-sequences-27.5.1.tgz#eaecc0d327fd68c8d9672a1e64ab8dccb2ef5327"
integrity sha512-k1gCAXAsNgLwEL+Y8Wvl+M6oEFj5bgazfZULpS5CneoPPXRaCCW7dm+q21Ky2VEE5X+VeRDBVg1Pcvvsr4TtNQ==
-diffie-hellman@^5.0.0:
- version "5.0.3"
- resolved "https://registry.npmjs.org/diffie-hellman/-/diffie-hellman-5.0.3.tgz"
- integrity sha512-kqag/Nl+f3GwyK25fhUMYj81BUOrZ9IuJsjIcDE5icNM9FJHAVm3VcUDxdLPoQtTuUylWm6ZIknYJwwaPxsUzg==
- dependencies:
- bn.js "^4.1.0"
- miller-rabin "^4.0.0"
- randombytes "^2.0.0"
-
dir-glob@^3.0.1:
version "3.0.1"
resolved "https://registry.npmjs.org/dir-glob/-/dir-glob-3.0.1.tgz"
@@ -2123,16 +1767,6 @@ dom-accessibility-api@^0.5.9:
resolved "https://registry.npmjs.org/dom-accessibility-api/-/dom-accessibility-api-0.5.10.tgz"
integrity sha512-Xu9mD0UjrJisTmv7lmVSDMagQcU9R5hwAbxsaAE/35XPnPLJobbuREfV/rraiSaEj/UOvgrzQs66zyTWTlyd+g==
-domain-browser@4.19.0:
- version "4.19.0"
- resolved "https://registry.npmjs.org/domain-browser/-/domain-browser-4.19.0.tgz"
- integrity sha512-fRA+BaAWOR/yr/t7T9E9GJztHPeFjj8U35ajyAjCDtAAnTn1Rc1f6W6VGPJrO1tkQv9zWu+JRof7z6oQtiYVFQ==
-
-domain-browser@^1.1.1:
- version "1.2.0"
- resolved "https://registry.npmjs.org/domain-browser/-/domain-browser-1.2.0.tgz"
- integrity sha512-jnjyiM6eRyZl2H+W8Q/zLMA481hzi0eszAaBUzIVnmYVDBbnLxVNnfu1HgEBvCbL+71FrxMl3E6lpKH7Ge3OXA==
-
domexception@^2.0.1:
version "2.0.1"
resolved "https://registry.npmjs.org/domexception/-/domexception-2.0.1.tgz"
@@ -2140,24 +1774,11 @@ domexception@^2.0.1:
dependencies:
webidl-conversions "^5.0.0"
-electron-to-chromium@^1.3.723, electron-to-chromium@^1.4.17:
+electron-to-chromium@^1.4.17:
version "1.4.31"
resolved "https://registry.npmjs.org/electron-to-chromium/-/electron-to-chromium-1.4.31.tgz"
integrity sha512-t3XVQtk+Frkv6aTD4RRk0OqosU+VLe1dQFW83MDer78ZD6a52frgXuYOIsLYTQiH2Lm+JB2OKYcn7zrX+YGAiQ==
-elliptic@^6.5.3:
- version "6.5.4"
- resolved "https://registry.npmjs.org/elliptic/-/elliptic-6.5.4.tgz"
- integrity sha512-iLhC6ULemrljPZb+QutR5TQGB+pdW6KGD5RSegS+8sorOZT+rdQFbsQFJgvN3eRqNALqJer4oQ16YvJHlU8hzQ==
- dependencies:
- bn.js "^4.11.9"
- brorand "^1.1.0"
- hash.js "^1.0.0"
- hmac-drbg "^1.0.1"
- inherits "^2.0.4"
- minimalistic-assert "^1.0.1"
- minimalistic-crypto-utils "^1.0.1"
-
emittery@^0.10.2:
version "0.10.2"
resolved "https://registry.yarnpkg.com/emittery/-/emittery-0.10.2.tgz#902eec8aedb8c41938c46e9385e9db7e03182933"
@@ -2178,18 +1799,6 @@ emoji-regex@^9.2.2:
resolved "https://registry.npmjs.org/emoji-regex/-/emoji-regex-9.2.2.tgz"
integrity sha512-L18DaJsXSUk2+42pv8mLs5jJT2hqFkFE4j21wOmgbUqsZ2hL72NsUU785g9RXgo3s0ZNgVl42TiHp3ZtOv/Vyg==
-emojis-list@^2.0.0:
- version "2.1.0"
- resolved "https://registry.npmjs.org/emojis-list/-/emojis-list-2.1.0.tgz"
- integrity sha1-TapNnbAPmBmIDHn6RXrlsJof04k=
-
-encoding@0.1.13:
- version "0.1.13"
- resolved "https://registry.npmjs.org/encoding/-/encoding-0.1.13.tgz"
- integrity sha512-ETBauow1T35Y/WZMkio9jiM0Z5xjHHmJ4XmjZOq1l/dXz3lr2sRn87nJy20RupqSh1F2m3HHPSp8ShIPQJrJ3A==
- dependencies:
- iconv-lite "^0.6.2"
-
enquirer@^2.3.5:
version "2.3.6"
resolved "https://registry.npmjs.org/enquirer/-/enquirer-2.3.6.tgz"
@@ -2197,7 +1806,7 @@ enquirer@^2.3.5:
dependencies:
ansi-colors "^4.1.1"
-es-abstract@^1.18.0-next.1, es-abstract@^1.18.0-next.2, es-abstract@^1.19.0, es-abstract@^1.19.1:
+es-abstract@^1.19.0, es-abstract@^1.19.1:
version "1.19.1"
resolved "https://registry.npmjs.org/es-abstract/-/es-abstract-1.19.1.tgz"
integrity sha512-2vJ6tjA/UfqLm2MPs7jxVybLoB8i1t1Jd9R3kISld20sIxPcTbLuggQOUxeWeAvIUkduv/CfMjuh4WmiXr2v9w==
@@ -2232,11 +1841,6 @@ es-to-primitive@^1.2.1:
is-date-object "^1.0.1"
is-symbol "^1.0.2"
-es6-object-assign@^1.1.0:
- version "1.1.0"
- resolved "https://registry.npmjs.org/es6-object-assign/-/es6-object-assign-1.1.0.tgz"
- integrity sha1-wsNYJlYkfDnqEHyx5mUrb58kUjw=
-
escalade@^3.1.1:
version "3.1.1"
resolved "https://registry.npmjs.org/escalade/-/escalade-3.1.1.tgz"
@@ -2445,24 +2049,6 @@ esutils@^2.0.2:
resolved "https://registry.npmjs.org/esutils/-/esutils-2.0.3.tgz"
integrity sha512-kVscqXk4OCp68SZ0dkgEKVi6/8ij300KBWTJq32P/dYeWTSwK41WyTxalN1eRmA5Z9UU/LX9D7FWSmV9SAYx6g==
-etag@1.8.1:
- version "1.8.1"
- resolved "https://registry.npmjs.org/etag/-/etag-1.8.1.tgz"
- integrity sha1-Qa4u62XvpiJorr/qg6x9eSmbCIc=
-
-events@^3.0.0:
- version "3.3.0"
- resolved "https://registry.npmjs.org/events/-/events-3.3.0.tgz"
- integrity sha512-mQw+2fkQbALzQ7V0MY0IqdnXNOeTtP4r0lN9z7AAawCXgqea7bDii20AYrIBrFd/Hx0M2Ocz6S111CaFkUcb0Q==
-
-evp_bytestokey@^1.0.0, evp_bytestokey@^1.0.3:
- version "1.0.3"
- resolved "https://registry.npmjs.org/evp_bytestokey/-/evp_bytestokey-1.0.3.tgz"
- integrity sha512-/f2Go4TognH/KvCISP7OUsHn85hT9nUkxxA9BEWxFn+Oj9o8ZNLm/40hdlgSLyuOimsrTKLUMEorQexp/aPQeA==
- dependencies:
- md5.js "^1.3.4"
- safe-buffer "^5.1.1"
-
execa@^5.0.0, execa@^5.1.1:
version "5.1.1"
resolved "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz"
@@ -2548,15 +2134,6 @@ fill-range@^7.0.1:
dependencies:
to-regex-range "^5.0.1"
-find-cache-dir@3.3.1:
- version "3.3.1"
- resolved "https://registry.npmjs.org/find-cache-dir/-/find-cache-dir-3.3.1.tgz"
- integrity sha512-t2GDMt3oGC/v+BMwzmllWDuJF/xcDtE5j/fCGbqDD7OLuJkj0cfh1YSA5VKPvwMeLFLNDBkwOKZ2X85jGLVftQ==
- dependencies:
- commondir "^1.0.1"
- make-dir "^3.0.2"
- pkg-dir "^4.1.0"
-
find-up@^2.1.0:
version "2.1.0"
resolved "https://registry.npmjs.org/find-up/-/find-up-2.1.0.tgz"
@@ -2585,11 +2162,6 @@ flatted@^3.1.0:
resolved "https://registry.npmjs.org/flatted/-/flatted-3.1.1.tgz"
integrity sha512-zAoAQiudy+r5SvnSw3KJy5os/oRJYHzrzja/tBDqrZtNhUw8bt6y8OBzMWcjWr+8liV8Eb6yOhw8WZ7VFZ5ZzA==
-foreach@^2.0.5:
- version "2.0.5"
- resolved "https://registry.npmjs.org/foreach/-/foreach-2.0.5.tgz"
- integrity sha1-C+4AUBiusmDQo6865ljdATbsG5k=
-
form-data@^3.0.0:
version "3.0.1"
resolved "https://registry.npmjs.org/form-data/-/form-data-3.0.1.tgz"
@@ -2604,7 +2176,7 @@ fs.realpath@^1.0.0:
resolved "https://registry.npmjs.org/fs.realpath/-/fs.realpath-1.0.0.tgz"
integrity sha1-FQStJSMVjKpA20onh8sBQRmU6k8=
-fsevents@^2.3.2, fsevents@~2.3.1:
+fsevents@^2.3.2:
version "2.3.2"
resolved "https://registry.npmjs.org/fsevents/-/fsevents-2.3.2.tgz"
integrity sha512-xiqMQR4xAeHTuB9uWm+fFRcIOgKBMiOBP+eXiyT7jsgVCq1bkVygt00oASowB7EdtpOHaaPgKt812P9ab+DDKA==
@@ -2638,13 +2210,6 @@ get-intrinsic@^1.0.2, get-intrinsic@^1.1.0, get-intrinsic@^1.1.1:
has "^1.0.3"
has-symbols "^1.0.1"
-get-orientation@1.1.2:
- version "1.1.2"
- resolved "https://registry.npmjs.org/get-orientation/-/get-orientation-1.1.2.tgz"
- integrity sha512-/pViTfifW+gBbh/RnlFYHINvELT9Znt+SYyDKAUL6uV6By019AK/s+i9XP4jSwq7lwP38Fd8HVeTxym3+hkwmQ==
- dependencies:
- stream-parser "^0.3.1"
-
get-package-type@^0.1.0:
version "0.1.0"
resolved "https://registry.npmjs.org/get-package-type/-/get-package-type-0.1.0.tgz"
@@ -2663,18 +2228,13 @@ get-symbol-description@^1.0.0:
call-bind "^1.0.2"
get-intrinsic "^1.1.1"
-glob-parent@^5.1.0, glob-parent@^5.1.2, glob-parent@~5.1.0:
+glob-parent@^5.1.0, glob-parent@^5.1.2:
version "5.1.2"
resolved "https://registry.npmjs.org/glob-parent/-/glob-parent-5.1.2.tgz"
integrity sha512-AOIgSQCepiJYwP3ARnGx+5VnTu2HBYdzbGP45eLw1vr3zB3vZLeyed1sC9hnbcOc9/SrMyM5RPQrkGz4aS9Zow==
dependencies:
is-glob "^4.0.1"
-glob-to-regexp@^0.4.1:
- version "0.4.1"
- resolved "https://registry.npmjs.org/glob-to-regexp/-/glob-to-regexp-0.4.1.tgz"
- integrity sha512-lkX1HJXwyMcprw/5YUZc2s7DrpAiHB21/V+E1rHUrVNokkvB6bqMzT0VfV6/86ZNabt1k14YOIaT7nDvOX3Iiw==
-
glob@7.1.7, glob@^7.1.1, glob@^7.1.2, glob@^7.1.3, glob@^7.1.4:
version "7.1.7"
resolved "https://registry.npmjs.org/glob/-/glob-7.1.7.tgz"
@@ -2711,7 +2271,7 @@ globby@^11.0.3:
merge2 "^1.3.0"
slash "^3.0.0"
-graceful-fs@^4.1.2, graceful-fs@^4.2.4:
+graceful-fs@^4.2.4:
version "4.2.6"
resolved "https://registry.npmjs.org/graceful-fs/-/graceful-fs-4.2.6.tgz"
integrity sha512-nTnJ528pbqxYanhpDYsi4Rd8MAeaBA67+RZ10CM1m3bTAVFEDcd5AuA4a6W5YkGZ1iNXHzZz8T6TBKLeBuNriQ==
@@ -2760,37 +2320,6 @@ has@^1.0.3:
dependencies:
function-bind "^1.1.1"
-hash-base@^3.0.0:
- version "3.1.0"
- resolved "https://registry.npmjs.org/hash-base/-/hash-base-3.1.0.tgz"
- integrity sha512-1nmYp/rhMDiE7AYkDw+lLwlAzz0AntGIe51F3RfFfEqyQ3feY2eI/NcwC6umIQVOASPMsWJLJScWKSSvzL9IVA==
- dependencies:
- inherits "^2.0.4"
- readable-stream "^3.6.0"
- safe-buffer "^5.2.0"
-
-hash.js@^1.0.0, hash.js@^1.0.3:
- version "1.1.7"
- resolved "https://registry.npmjs.org/hash.js/-/hash.js-1.1.7.tgz"
- integrity sha512-taOaskGt4z4SOANNseOviYDvjEJinIkRgmp7LbKP2YTTmVxWBl87s/uzK9r+44BclBSp2X7K1hqeNfz9JbBeXA==
- dependencies:
- inherits "^2.0.3"
- minimalistic-assert "^1.0.1"
-
-he@1.2.0:
- version "1.2.0"
- resolved "https://registry.npmjs.org/he/-/he-1.2.0.tgz"
- integrity sha512-F/1DnUGPopORZi0ni+CvrCgHQ5FyEAHRLSApuYWMmrbSwoN2Mn/7k+Gl38gJnR7yyDZk6WLXwiGod1JOWNDKGw==
-
-hmac-drbg@^1.0.1:
- version "1.0.1"
- resolved "https://registry.npmjs.org/hmac-drbg/-/hmac-drbg-1.0.1.tgz"
- integrity sha1-0nRXAQJabHdabFRXk+1QL8DGSaE=
- dependencies:
- hash.js "^1.0.3"
- minimalistic-assert "^1.0.0"
- minimalistic-crypto-utils "^1.0.1"
-
html-encoding-sniffer@^2.0.1:
version "2.0.1"
resolved "https://registry.npmjs.org/html-encoding-sniffer/-/html-encoding-sniffer-2.0.1.tgz"
@@ -2803,17 +2332,6 @@ html-escaper@^2.0.0:
resolved "https://registry.npmjs.org/html-escaper/-/html-escaper-2.0.2.tgz"
integrity sha512-H2iMtd0I4Mt5eYiapRdIDjp+XzelXQ0tFE4JS7YFwFevXXMmOp9myNrUvCg0D6ws8iqkRPBfKHgbwig1SmlLfg==
-http-errors@1.7.3:
- version "1.7.3"
- resolved "https://registry.npmjs.org/http-errors/-/http-errors-1.7.3.tgz"
- integrity sha512-ZTTX0MWrsQ2ZAhA1cejAwDLycFsd7I7nVtnkT3Ol0aqodaKW+0CTZDQ1uBv5whptCnc8e8HeRRJxRs0kmm/Qfw==
- dependencies:
- depd "~1.1.2"
- inherits "2.0.4"
- setprototypeof "1.1.1"
- statuses ">= 1.5.0 < 2"
- toidentifier "1.0.0"
-
http-proxy-agent@^4.0.1:
version "4.0.1"
resolved "https://registry.npmjs.org/http-proxy-agent/-/http-proxy-agent-4.0.1.tgz"
@@ -2823,11 +2341,6 @@ http-proxy-agent@^4.0.1:
agent-base "6"
debug "4"
-https-browserify@1.0.0, https-browserify@^1.0.0:
- version "1.0.0"
- resolved "https://registry.npmjs.org/https-browserify/-/https-browserify-1.0.0.tgz"
- integrity sha1-7AbBDgo0wPL68Zn3/X/Hj//QPHM=
-
https-proxy-agent@^5.0.0:
version "5.0.0"
resolved "https://registry.npmjs.org/https-proxy-agent/-/https-proxy-agent-5.0.0.tgz"
@@ -2853,13 +2366,6 @@ iconv-lite@0.4.24:
dependencies:
safer-buffer ">= 2.1.2 < 3"
-iconv-lite@^0.6.2:
- version "0.6.2"
- resolved "https://registry.npmjs.org/iconv-lite/-/iconv-lite-0.6.2.tgz"
- integrity sha512-2y91h5OpQlolefMPmUlivelittSWy0rP+oYVpn6A7GwVHNE8AWzoYOBNmlwks3LobaJxgHCYZAnyNo2GgpNRNQ==
- dependencies:
- safer-buffer ">= 2.1.2 < 3.0.0"
-
identity-obj-proxy@^3.0.0:
version "3.0.0"
resolved "https://registry.npmjs.org/identity-obj-proxy/-/identity-obj-proxy-3.0.0.tgz"
@@ -2867,11 +2373,6 @@ identity-obj-proxy@^3.0.0:
dependencies:
harmony-reflect "^1.4.6"
-ieee754@^1.1.4:
- version "1.2.1"
- resolved "https://registry.npmjs.org/ieee754/-/ieee754-1.2.1.tgz"
- integrity sha512-dcyqhDvX1C46lXZcVqCpK+FtMRQVdIMN6/Df5js2zouUsqG7I6sFxitIC+7KYK29KdXOLHdu9zL4sFnoVQnqaA==
-
ignore@^4.0.6:
version "4.0.6"
resolved "https://registry.npmjs.org/ignore/-/ignore-4.0.6.tgz"
@@ -2882,13 +2383,6 @@ ignore@^5.1.4, ignore@^5.1.8:
resolved "https://registry.npmjs.org/ignore/-/ignore-5.2.0.tgz"
integrity sha512-CmxgYGiEPCLhfLnpPp1MoRmifwEIOgjcHXxOBjv7mY96c+eWScsOP9c112ZyLdWHi0FxHjI+4uVhKYp/gcdRmQ==
-image-size@1.0.0:
- version "1.0.0"
- resolved "https://registry.npmjs.org/image-size/-/image-size-1.0.0.tgz"
- integrity sha512-JLJ6OwBfO1KcA+TvJT+v8gbE6iWbj24LyDNFgFEN0lzegn6cC6a/p3NIDaepMsJjQjlUWqIC7wJv8lBFxPNjcw==
- dependencies:
- queue "6.0.2"
-
import-fresh@^3.0.0, import-fresh@^3.2.1:
version "3.3.0"
resolved "https://registry.npmjs.org/import-fresh/-/import-fresh-3.3.0.tgz"
@@ -2923,21 +2417,11 @@ inflight@^1.0.4:
once "^1.3.0"
wrappy "1"
-inherits@2, inherits@2.0.4, inherits@^2.0.1, inherits@^2.0.3, inherits@^2.0.4, inherits@~2.0.3, inherits@~2.0.4:
+inherits@2:
version "2.0.4"
resolved "https://registry.npmjs.org/inherits/-/inherits-2.0.4.tgz"
integrity sha512-k/vGaX4/Yla3WzyMCvTQOXYeIHvqOKtnqBduzTHpzpQZzAskKMhZ2K+EnBiSM9zGSoIFeMpXKxa4dYeZIQqewQ==
-inherits@2.0.1:
- version "2.0.1"
- resolved "https://registry.npmjs.org/inherits/-/inherits-2.0.1.tgz"
- integrity sha1-sX0I0ya0Qj5Wjv9xn5GwscvfafE=
-
-inherits@2.0.3, inherits@~2.0.1:
- version "2.0.3"
- resolved "https://registry.npmjs.org/inherits/-/inherits-2.0.3.tgz"
- integrity sha1-Yzwsg+PaQqUC9SRmAiSA9CCCYd4=
-
internal-slot@^1.0.3:
version "1.0.3"
resolved "https://registry.npmjs.org/internal-slot/-/internal-slot-1.0.3.tgz"
@@ -2947,25 +2431,11 @@ internal-slot@^1.0.3:
has "^1.0.3"
side-channel "^1.0.4"
-is-arguments@^1.0.4:
- version "1.1.0"
- resolved "https://registry.npmjs.org/is-arguments/-/is-arguments-1.1.0.tgz"
- integrity sha512-1Ij4lOMPl/xB5kBDn7I+b2ttPMKa8szhEIrXDuXQD/oe3HJLTLhqhgGspwgyGd6MOywBUqVvYicF72lkgDnIHg==
- dependencies:
- call-bind "^1.0.0"
-
is-bigint@^1.0.1:
version "1.0.2"
resolved "https://registry.npmjs.org/is-bigint/-/is-bigint-1.0.2.tgz"
integrity sha512-0JV5+SOCQkIdzjBK9buARcV804Ddu7A0Qet6sHi3FimE9ne6m4BGQZfRn+NZiXbBk4F4XmHfDZIipLj9pX8dSA==
-is-binary-path@~2.1.0:
- version "2.1.0"
- resolved "https://registry.npmjs.org/is-binary-path/-/is-binary-path-2.1.0.tgz"
- integrity sha512-ZMERYes6pDydyuGidse7OsHxtbI7WVeUEozgR/g7rd0xUimYNlvZRE/K2MgZTjWy725IfelLeVcEM97mmtRGXw==
- dependencies:
- binary-extensions "^2.0.0"
-
is-boolean-object@^1.1.0:
version "1.1.1"
resolved "https://registry.npmjs.org/is-boolean-object/-/is-boolean-object-1.1.1.tgz"
@@ -3010,26 +2480,13 @@ is-generator-fn@^2.0.0:
resolved "https://registry.npmjs.org/is-generator-fn/-/is-generator-fn-2.1.0.tgz"
integrity sha512-cTIB4yPYL/Grw0EaSzASzg6bBy9gqCofvWN8okThAYIxKJZC+udlRAmGbM0XLeniEJSs8uEgHPGuHSe1XsOLSQ==
-is-generator-function@^1.0.7:
- version "1.0.9"
- resolved "https://registry.npmjs.org/is-generator-function/-/is-generator-function-1.0.9.tgz"
- integrity sha512-ZJ34p1uvIfptHCN7sFTjGibB9/oBg17sHqzDLfuwhvmN/qLVvIQXRQ8licZQ35WJ8KuEQt/etnnzQFI9C9Ue/A==
-
-is-glob@^4.0.0, is-glob@^4.0.1, is-glob@^4.0.3, is-glob@~4.0.1:
+is-glob@^4.0.0, is-glob@^4.0.1, is-glob@^4.0.3:
version "4.0.3"
resolved "https://registry.npmjs.org/is-glob/-/is-glob-4.0.3.tgz"
integrity sha512-xelSayHH36ZgE7ZWhli7pW34hNbNl8Ojv5KVmkJD4hBdD3th8Tfk9vYasLM+mXWOZhFkgZfxhLSnrwRr4elSSg==
dependencies:
is-extglob "^2.1.1"
-is-nan@^1.2.1:
- version "1.3.2"
- resolved "https://registry.npmjs.org/is-nan/-/is-nan-1.3.2.tgz"
- integrity sha512-E+zBKpQ2t6MEo1VsonYmluk9NxGrbzpeeLC2xIViuO2EjU2xsXsBPwTr3Ykv9l08UYEVEdWeRZNouaZqF6RN0w==
- dependencies:
- call-bind "^1.0.0"
- define-properties "^1.1.3"
-
is-negative-zero@^2.0.1:
version "2.0.1"
resolved "https://registry.npmjs.org/is-negative-zero/-/is-negative-zero-2.0.1.tgz"
@@ -3087,17 +2544,6 @@ is-symbol@^1.0.2, is-symbol@^1.0.3:
dependencies:
has-symbols "^1.0.2"
-is-typed-array@^1.1.3:
- version "1.1.5"
- resolved "https://registry.npmjs.org/is-typed-array/-/is-typed-array-1.1.5.tgz"
- integrity sha512-S+GRDgJlR3PyEbsX/Fobd9cqpZBuvUS+8asRqYDMLCb2qMzt1oz5m5oxQCxOgUDxiWsOVNi4yaF+/uvdlHlYug==
- dependencies:
- available-typed-arrays "^1.0.2"
- call-bind "^1.0.2"
- es-abstract "^1.18.0-next.2"
- foreach "^2.0.5"
- has-symbols "^1.0.1"
-
is-typedarray@^1.0.0:
version "1.0.0"
resolved "https://registry.npmjs.org/is-typedarray/-/is-typedarray-1.0.0.tgz"
@@ -3110,11 +2556,6 @@ is-weakref@^1.0.1:
dependencies:
call-bind "^1.0.0"
-isarray@^1.0.0, isarray@~1.0.0:
- version "1.0.0"
- resolved "https://registry.npmjs.org/isarray/-/isarray-1.0.0.tgz"
- integrity sha1-u5NdSFgsuhaMBoNJV6VKPgcSTxE=
-
isexe@^2.0.0:
version "2.0.0"
resolved "https://registry.npmjs.org/isexe/-/isexe-2.0.0.tgz"
@@ -3627,15 +3068,6 @@ jest-watcher@^28.0.0:
jest-util "^28.1.0"
string-length "^4.0.1"
-jest-worker@27.0.0-next.5:
- version "27.0.0-next.5"
- resolved "https://registry.npmjs.org/jest-worker/-/jest-worker-27.0.0-next.5.tgz"
- integrity sha512-mk0umAQ5lT+CaOJ+Qp01N6kz48sJG2kr2n1rX0koqKf6FIygQV0qLOdN9SCYID4IVeSigDOcPeGLozdMLYfb5g==
- dependencies:
- "@types/node" "*"
- merge-stream "^2.0.0"
- supports-color "^8.0.0"
-
jest-worker@^27.4.6:
version "27.4.6"
resolved "https://registry.yarnpkg.com/jest-worker/-/jest-worker-27.4.6.tgz#5d2d93db419566cb680752ca0792780e71b3273e"
@@ -3806,15 +3238,6 @@ listr2@^4.0.1:
through "^2.3.8"
wrap-ansi "^7.0.0"
-loader-utils@1.2.3:
- version "1.2.3"
- resolved "https://registry.npmjs.org/loader-utils/-/loader-utils-1.2.3.tgz"
- integrity sha512-fkpz8ejdnEMG3s37wGL07iSBDg99O9D5yflE9RGNH3hRdx9SOwYfnGYdZOUIZitN8E+E2vkq3MUMYMvPYl5ZZA==
- dependencies:
- big.js "^5.2.2"
- emojis-list "^2.0.0"
- json5 "^1.0.1"
-
locate-path@^2.0.0:
version "2.0.0"
resolved "https://registry.npmjs.org/locate-path/-/locate-path-2.0.0.tgz"
@@ -3835,11 +3258,6 @@ lodash.merge@^4.6.2:
resolved "https://registry.npmjs.org/lodash.merge/-/lodash.merge-4.6.2.tgz"
integrity sha512-0KpjqXRVvrYyCsX1swR/XTK0va6VQkQM6MNo7PqW77ByjAhoARA8EfrP1N4+KlKj8YS0ZUCtRT/YUuhyYDujIQ==
-lodash.sortby@^4.7.0:
- version "4.7.0"
- resolved "https://registry.npmjs.org/lodash.sortby/-/lodash.sortby-4.7.0.tgz"
- integrity sha1-7dFMgk4sycHgsKG0K7UhBRakJDg=
-
lodash.truncate@^4.4.2:
version "4.4.2"
resolved "https://registry.npmjs.org/lodash.truncate/-/lodash.truncate-4.4.2.tgz"
@@ -3879,7 +3297,7 @@ lz-string@^1.4.4:
resolved "https://registry.npmjs.org/lz-string/-/lz-string-1.4.4.tgz"
integrity sha1-wNjq82BZ9wV5bh40SBHPTEmNOiY=
-make-dir@^3.0.0, make-dir@^3.0.2:
+make-dir@^3.0.0:
version "3.1.0"
resolved "https://registry.npmjs.org/make-dir/-/make-dir-3.1.0.tgz"
integrity sha512-g3FeP20LNwhALb/6Cz6Dd4F2ngze0jz7tbzrD2wAV+o9FeNHe4rL+yK2md0J/fiSf1sa1ADhXqi5+oVwOM/eGw==
@@ -3893,15 +3311,6 @@ makeerror@1.0.x:
dependencies:
tmpl "1.0.x"
-md5.js@^1.3.4:
- version "1.3.5"
- resolved "https://registry.npmjs.org/md5.js/-/md5.js-1.3.5.tgz"
- integrity sha512-xitP+WxNPcTTOgnTJcrhM0xvdPepipPSf3I8EIpGKeFLjt3PlJLIDG3u8EX53ZIubkb+5U2+3rELYpEhHhzdkg==
- dependencies:
- hash-base "^3.0.0"
- inherits "^2.0.1"
- safe-buffer "^5.1.2"
-
merge-stream@^2.0.0:
version "2.0.0"
resolved "https://registry.npmjs.org/merge-stream/-/merge-stream-2.0.0.tgz"
@@ -3920,14 +3329,6 @@ micromatch@^4.0.2, micromatch@^4.0.4:
braces "^3.0.1"
picomatch "^2.2.3"
-miller-rabin@^4.0.0:
- version "4.0.1"
- resolved "https://registry.npmjs.org/miller-rabin/-/miller-rabin-4.0.1.tgz"
- integrity sha512-115fLhvZVqWwHPbClyntxEVfVDfl9DLLTuJvq3g2O/Oxi8AiNouAHvDSzHS0viUJc+V5vm3eq91Xwqn9dp4jRA==
- dependencies:
- bn.js "^4.0.0"
- brorand "^1.0.1"
-
mime-db@1.47.0:
version "1.47.0"
resolved "https://registry.npmjs.org/mime-db/-/mime-db-1.47.0.tgz"
@@ -3945,16 +3346,6 @@ mimic-fn@^2.1.0:
resolved "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz"
integrity sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==
-minimalistic-assert@^1.0.0, minimalistic-assert@^1.0.1:
- version "1.0.1"
- resolved "https://registry.npmjs.org/minimalistic-assert/-/minimalistic-assert-1.0.1.tgz"
- integrity sha512-UtJcAD4yEaGtjPezWuO9wC4nwUnVH/8/Im3yEHQP4b67cXlD/Qr9hdITCU1xDbSEXg2XKNaP8jsReV7vQd00/A==
-
-minimalistic-crypto-utils@^1.0.1:
- version "1.0.1"
- resolved "https://registry.npmjs.org/minimalistic-crypto-utils/-/minimalistic-crypto-utils-1.0.1.tgz"
- integrity sha1-9sAMHAsIIkblxNmd+4x8CDsrWCo=
-
minimatch@^3.0.4, minimatch@^3.1.2:
version "3.1.2"
resolved "https://registry.yarnpkg.com/minimatch/-/minimatch-3.1.2.tgz#19cd194bfd3e428f049a70817c038d89ab4be35b"
@@ -3982,17 +3373,10 @@ ms@2.1.2, ms@^2.1.1:
resolved "https://registry.npmjs.org/ms/-/ms-2.1.2.tgz"
integrity sha512-sGkPx+VjMtmA6MX27oA4FBFELFCZZ4S4XqeGOXCv68tT+jb3vk/RyaKWP0PTKyWtmLSM0b+adUTEvbs1PEaH2w==
-nanoid@^3.1.23:
- version "3.2.0"
- resolved "https://registry.yarnpkg.com/nanoid/-/nanoid-3.2.0.tgz#62667522da6673971cca916a6d3eff3f415ff80c"
- integrity sha512-fmsZYa9lpn69Ad5eDn7FMcnnSR+8R34W9qJEijxYhTbfOWzr22n1QxCMzXLK+ODyW2973V3Fux959iQoUxzUIA==
-
-native-url@0.3.4:
- version "0.3.4"
- resolved "https://registry.npmjs.org/native-url/-/native-url-0.3.4.tgz"
- integrity sha512-6iM8R99ze45ivyH8vybJ7X0yekIcPf5GgLV5K0ENCbmRcaRIDoj37BC8iLEmaaBfqqb8enuZ5p0uhY+lVAbAcA==
- dependencies:
- querystring "^0.2.0"
+nanoid@^3.3.4:
+ version "3.3.7"
+ resolved "https://registry.yarnpkg.com/nanoid/-/nanoid-3.3.7.tgz#d0c301a691bc8d54efa0a2226ccf3fe2fd656bd8"
+ integrity sha512-eSRppjcPIatRIMC1U6UngP8XFcz8MQWGQdt1MTBQ7NaAmvXDfvNxbvWV3x2y6CdEUciCSsDHDQZbhYaB8QEo2g==
natural-compare@^1.4.0:
version "1.4.0"
@@ -4004,71 +3388,31 @@ next-seo@^5.1.0:
resolved "https://registry.yarnpkg.com/next-seo/-/next-seo-5.1.0.tgz#aa9fd6249a11bf93e6da06fa2a6bc89268936edf"
integrity sha512-ampuQfNTOi1x+xtRIb6CZGunIo6rQXtMo2Tyu861d5GjJFIwfOXsA4lzCa4+e2rLkyXDyVpavNNUZWa3US9ELw==
-next@^11.1.3:
- version "11.1.3"
- resolved "https://registry.npmjs.org/next/-/next-11.1.3.tgz"
- integrity sha512-ud/gKmnKQ8wtHC+pd1ZiqPRa7DdgulPkAk94MbpsspfNliwZkYs9SIYWhlLSyg+c661LzdUI2nZshvrtggSYWA==
- dependencies:
- "@babel/runtime" "7.15.3"
- "@hapi/accept" "5.0.2"
- "@next/env" "11.1.3"
- "@next/polyfill-module" "11.1.3"
- "@next/react-dev-overlay" "11.1.3"
- "@next/react-refresh-utils" "11.1.3"
- "@node-rs/helper" "1.2.1"
- assert "2.0.0"
- ast-types "0.13.2"
- browserify-zlib "0.2.0"
- browserslist "4.16.6"
- buffer "5.6.0"
- caniuse-lite "^1.0.30001228"
- chalk "2.4.2"
- chokidar "3.5.1"
- constants-browserify "1.0.0"
- crypto-browserify "3.12.0"
- cssnano-simple "3.0.0"
- domain-browser "4.19.0"
- encoding "0.1.13"
- etag "1.8.1"
- find-cache-dir "3.3.1"
- get-orientation "1.1.2"
- https-browserify "1.0.0"
- image-size "1.0.0"
- jest-worker "27.0.0-next.5"
- native-url "0.3.4"
- node-fetch "2.6.1"
- node-html-parser "1.4.9"
- node-libs-browser "^2.2.1"
- os-browserify "0.3.0"
- p-limit "3.1.0"
- path-browserify "1.0.1"
- pnp-webpack-plugin "1.6.4"
- postcss "8.2.15"
- process "0.11.10"
- querystring-es3 "0.2.1"
- raw-body "2.4.1"
- react-is "17.0.2"
- react-refresh "0.8.3"
- stream-browserify "3.0.0"
- stream-http "3.1.1"
- string_decoder "1.3.0"
- styled-jsx "4.0.1"
- timers-browserify "2.0.12"
- tty-browserify "0.0.1"
- use-subscription "1.5.1"
- util "0.12.4"
- vm-browserify "1.1.2"
- watchpack "2.1.1"
+next@12:
+ version "12.3.4"
+ resolved "https://registry.yarnpkg.com/next/-/next-12.3.4.tgz#f2780a6ebbf367e071ce67e24bd8a6e05de2fcb1"
+ integrity sha512-VcyMJUtLZBGzLKo3oMxrEF0stxh8HwuW976pAzlHhI3t8qJ4SROjCrSh1T24bhrbjw55wfZXAbXPGwPt5FLRfQ==
+ dependencies:
+ "@next/env" "12.3.4"
+ "@swc/helpers" "0.4.11"
+ caniuse-lite "^1.0.30001406"
+ postcss "8.4.14"
+ styled-jsx "5.0.7"
+ use-sync-external-store "1.2.0"
optionalDependencies:
- "@next/swc-darwin-arm64" "11.1.3"
- "@next/swc-darwin-x64" "11.1.3"
- "@next/swc-linux-x64-gnu" "11.1.3"
- "@next/swc-win32-x64-msvc" "11.1.3"
-
-node-fetch@2.6.1:
- version "2.6.1"
- resolved "https://registry.npmjs.org/node-fetch/-/node-fetch-2.6.1.tgz"
- integrity sha512-V4aYg89jEoVRxRb2fJdAg8FHvI7cEyYdVAh94HH0UIK8oJxUfkjlDQN9RbMx+bEjP7+ggMiFRprSti032Oipxw==
+ "@next/swc-android-arm-eabi" "12.3.4"
+ "@next/swc-android-arm64" "12.3.4"
+ "@next/swc-darwin-arm64" "12.3.4"
+ "@next/swc-darwin-x64" "12.3.4"
+ "@next/swc-freebsd-x64" "12.3.4"
+ "@next/swc-linux-arm-gnueabihf" "12.3.4"
+ "@next/swc-linux-arm64-gnu" "12.3.4"
+ "@next/swc-linux-arm64-musl" "12.3.4"
+ "@next/swc-linux-x64-gnu" "12.3.4"
+ "@next/swc-linux-x64-musl" "12.3.4"
+ "@next/swc-win32-arm64-msvc" "12.3.4"
+ "@next/swc-win32-ia32-msvc" "12.3.4"
+ "@next/swc-win32-x64-msvc" "12.3.4"
node-fetch@^2.6.1:
version "2.6.6"
@@ -4077,58 +3421,17 @@ node-fetch@^2.6.1:
dependencies:
whatwg-url "^5.0.0"
-node-html-parser@1.4.9:
- version "1.4.9"
- resolved "https://registry.npmjs.org/node-html-parser/-/node-html-parser-1.4.9.tgz"
- integrity sha512-UVcirFD1Bn0O+TSmloHeHqZZCxHjvtIeGdVdGMhyZ8/PWlEiZaZ5iJzR189yKZr8p0FXN58BUeC7RHRkf/KYGw==
- dependencies:
- he "1.2.0"
-
node-int64@^0.4.0:
version "0.4.0"
resolved "https://registry.npmjs.org/node-int64/-/node-int64-0.4.0.tgz"
integrity sha1-h6kGXNs1XTGC2PlM4RGIuCXGijs=
-node-libs-browser@^2.2.1:
- version "2.2.1"
- resolved "https://registry.npmjs.org/node-libs-browser/-/node-libs-browser-2.2.1.tgz"
- integrity sha512-h/zcD8H9kaDZ9ALUWwlBUDo6TKF8a7qBSCSEGfjTVIYeqsioSKaAX+BN7NgiMGp6iSIXZ3PxgCu8KS3b71YK5Q==
- dependencies:
- assert "^1.1.1"
- browserify-zlib "^0.2.0"
- buffer "^4.3.0"
- console-browserify "^1.1.0"
- constants-browserify "^1.0.0"
- crypto-browserify "^3.11.0"
- domain-browser "^1.1.1"
- events "^3.0.0"
- https-browserify "^1.0.0"
- os-browserify "^0.3.0"
- path-browserify "0.0.1"
- process "^0.11.10"
- punycode "^1.2.4"
- querystring-es3 "^0.2.0"
- readable-stream "^2.3.3"
- stream-browserify "^2.0.1"
- stream-http "^2.7.2"
- string_decoder "^1.0.0"
- timers-browserify "^2.0.4"
- tty-browserify "0.0.0"
- url "^0.11.0"
- util "^0.11.0"
- vm-browserify "^1.0.1"
-
-node-releases@^1.1.71:
- version "1.1.71"
- resolved "https://registry.npmjs.org/node-releases/-/node-releases-1.1.71.tgz"
- integrity sha512-zR6HoT6LrLCRBwukmrVbHv0EpEQjksO6GmFcZQQuCAy139BEsoVKPYnf3jongYW83fAa1torLGYwxxky/p28sg==
-
node-releases@^2.0.1:
version "2.0.1"
resolved "https://registry.npmjs.org/node-releases/-/node-releases-2.0.1.tgz"
integrity sha512-CqyzN6z7Q6aMeF/ktcMVTzhAHCEpf8SOarwpzpf8pNBY2k5/oM34UHldUwp8VKI7uxct2HxSRdJjBaZeESzcxA==
-normalize-path@^3.0.0, normalize-path@~3.0.0:
+normalize-path@^3.0.0:
version "3.0.0"
resolved "https://registry.npmjs.org/normalize-path/-/normalize-path-3.0.0.tgz"
integrity sha512-6eZs5Ls3WtCisHWp9S2GUy8dqkpGi4BVSz3GaqiE6ezub0512ESztXUwUB6C6IKbQkY2Pnb/mD4WYojCRwcwLA==
@@ -4155,14 +3458,6 @@ object-inspect@^1.11.0, object-inspect@^1.12.0, object-inspect@^1.9.0:
resolved "https://registry.npmjs.org/object-inspect/-/object-inspect-1.12.0.tgz"
integrity sha512-Ho2z80bVIvJloH+YzRmpZVQe87+qASmBUKZDWgx9cu+KDrX2ZDH/3tMy+gXbZETVGs2M8YdxObOh7XAtim9Y0g==
-object-is@^1.0.1:
- version "1.1.5"
- resolved "https://registry.npmjs.org/object-is/-/object-is-1.1.5.tgz"
- integrity sha512-3cyDsyHgtmi7I7DfSSI2LDp6SK2lwvtbg0p0R1e0RvTqF5ceGx+K2dfSjm1bKDMVCFEDAQvy+o8c6a7VujOddw==
- dependencies:
- call-bind "^1.0.2"
- define-properties "^1.1.3"
-
object-keys@^1.0.12, object-keys@^1.1.1:
version "1.1.1"
resolved "https://registry.npmjs.org/object-keys/-/object-keys-1.1.1.tgz"
@@ -4251,18 +3546,6 @@ optionator@^0.9.1:
type-check "^0.4.0"
word-wrap "^1.2.3"
-os-browserify@0.3.0, os-browserify@^0.3.0:
- version "0.3.0"
- resolved "https://registry.npmjs.org/os-browserify/-/os-browserify-0.3.0.tgz"
- integrity sha1-hUNzx/XCMVkU/Jv8a9gjj92h7Cc=
-
-p-limit@3.1.0:
- version "3.1.0"
- resolved "https://registry.npmjs.org/p-limit/-/p-limit-3.1.0.tgz"
- integrity sha512-TYOanM3wGwNGsZN2cVTYPArw454xnXj5qmWF1bEoAc4+cU/ol7GVh7odevjp1FNHduHc3KZMcFduxU5Xc6uJRQ==
- dependencies:
- yocto-queue "^0.1.0"
-
p-limit@^1.1.0:
version "1.3.0"
resolved "https://registry.npmjs.org/p-limit/-/p-limit-1.3.0.tgz"
@@ -4308,11 +3591,6 @@ p-try@^2.0.0:
resolved "https://registry.npmjs.org/p-try/-/p-try-2.2.0.tgz"
integrity sha512-R4nPAVTAU0B9D35/Gk3uJf/7XYbQcyohSKdvAxIRSNghFl4e71hVoGnBNQz9cWaXxO2I10KTC+3jMdvvoKw6dQ==
-pako@~1.0.5:
- version "1.0.11"
- resolved "https://registry.npmjs.org/pako/-/pako-1.0.11.tgz"
- integrity sha512-4hLB8Py4zZce5s4yd9XzopqwVv/yGNhV1Bl8NTmCq1763HeK2+EwVTv+leGeL13Dnh2wfbqowVPXCIO0z4taYw==
-
parent-module@^1.0.0:
version "1.0.1"
resolved "https://registry.npmjs.org/parent-module/-/parent-module-1.0.1.tgz"
@@ -4320,32 +3598,11 @@ parent-module@^1.0.0:
dependencies:
callsites "^3.0.0"
-parse-asn1@^5.0.0, parse-asn1@^5.1.5:
- version "5.1.6"
- resolved "https://registry.npmjs.org/parse-asn1/-/parse-asn1-5.1.6.tgz"
- integrity sha512-RnZRo1EPU6JBnra2vGHj0yhp6ebyjBZpmUCLHWiFhxlzvBCCpAuZ7elsBp1PVAbQN0/04VD/19rfzlBSwLstMw==
- dependencies:
- asn1.js "^5.2.0"
- browserify-aes "^1.0.0"
- evp_bytestokey "^1.0.0"
- pbkdf2 "^3.0.3"
- safe-buffer "^5.1.1"
-
parse5@6.0.1:
version "6.0.1"
resolved "https://registry.npmjs.org/parse5/-/parse5-6.0.1.tgz"
integrity sha512-Ofn/CTFzRGTTxwpNEs9PP93gXShHcTq255nzRYSKe8AkVpZY7e1fpmTfOyoIvjP5HG7Z2ZM7VS9PPhQGW2pOpw==
-path-browserify@0.0.1:
- version "0.0.1"
- resolved "https://registry.npmjs.org/path-browserify/-/path-browserify-0.0.1.tgz"
- integrity sha512-BapA40NHICOS+USX9SN4tyhq+A2RrN/Ws5F0Z5aMHDp98Fl86lX8Oti8B7uN93L4Ifv4fHOEA+pQw87gmMO/lQ==
-
-path-browserify@1.0.1:
- version "1.0.1"
- resolved "https://registry.npmjs.org/path-browserify/-/path-browserify-1.0.1.tgz"
- integrity sha512-b7uo2UCUOYZcnF/3ID0lulOJi/bafxa1xPe7ZPsammBSpjSWQkjNxlt635YGS2MiR9GjvuXCtz2emr3jbsz98g==
-
path-exists@^3.0.0:
version "3.0.0"
resolved "https://registry.npmjs.org/path-exists/-/path-exists-3.0.0.tgz"
@@ -4376,17 +3633,6 @@ path-type@^4.0.0:
resolved "https://registry.npmjs.org/path-type/-/path-type-4.0.0.tgz"
integrity sha512-gDKb8aZMDeD/tZWs9P6+q0J9Mwkdl6xMV8TjnGP3qJVJ06bdMgkbBlLU8IdfOsIsFz2BW1rNVT3XuNEl8zPAvw==
-pbkdf2@^3.0.3:
- version "3.1.2"
- resolved "https://registry.npmjs.org/pbkdf2/-/pbkdf2-3.1.2.tgz"
- integrity sha512-iuh7L6jA7JEGu2WxDwtQP1ddOpaJNC4KlDEFfdQajSGgGPNi4OyDc2R7QnbY2bR9QjBVGwgvTdNJZoE7RaxUMA==
- dependencies:
- create-hash "^1.1.2"
- create-hmac "^1.1.4"
- ripemd160 "^2.0.1"
- safe-buffer "^5.0.1"
- sha.js "^2.4.8"
-
picocolors@^1.0.0:
version "1.0.0"
resolved "https://registry.npmjs.org/picocolors/-/picocolors-1.0.0.tgz"
@@ -4402,33 +3648,21 @@ pirates@^4.0.4:
resolved "https://registry.yarnpkg.com/pirates/-/pirates-4.0.5.tgz#feec352ea5c3268fb23a37c702ab1699f35a5f3b"
integrity sha512-8V9+HQPupnaXMA23c5hvl69zXvTwTzyAYasnkb0Tts4XvO4CliqONMOnvlq26rkhLC3nWDFBJf73LU1e1VZLaQ==
-pkg-dir@^4.1.0, pkg-dir@^4.2.0:
+pkg-dir@^4.2.0:
version "4.2.0"
resolved "https://registry.npmjs.org/pkg-dir/-/pkg-dir-4.2.0.tgz"
integrity sha512-HRDzbaKjC+AOWVXxAU/x54COGeIv9eb+6CkDSQoNTt4XyWoIJvuPsXizxu/Fr23EiekbtZwmh1IcIG/l/a10GQ==
dependencies:
find-up "^4.0.0"
-platform@1.3.6:
- version "1.3.6"
- resolved "https://registry.npmjs.org/platform/-/platform-1.3.6.tgz"
- integrity sha512-fnWVljUchTro6RiCFvCXBbNhJc2NijN7oIQxbwsyL0buWJPG85v81ehlHI9fXrJsMNgTofEoWIQeClKpgxFLrg==
-
-pnp-webpack-plugin@1.6.4:
- version "1.6.4"
- resolved "https://registry.npmjs.org/pnp-webpack-plugin/-/pnp-webpack-plugin-1.6.4.tgz"
- integrity sha512-7Wjy+9E3WwLOEL30D+m8TSTF7qJJUJLONBnwQp0518siuMxUQUbgZwssaFX+QKlZkjHZcw/IpZCt/H0srrntSg==
- dependencies:
- ts-pnp "^1.1.6"
-
-postcss@8.2.15:
- version "8.2.15"
- resolved "https://registry.npmjs.org/postcss/-/postcss-8.2.15.tgz"
- integrity sha512-2zO3b26eJD/8rb106Qu2o7Qgg52ND5HPjcyQiK2B98O388h43A448LCslC0dI2P97wCAQRJsFvwTRcXxTKds+Q==
+postcss@8.4.14:
+ version "8.4.14"
+ resolved "https://registry.yarnpkg.com/postcss/-/postcss-8.4.14.tgz#ee9274d5622b4858c1007a74d76e42e56fd21caf"
+ integrity sha512-E398TUmfAYFPBSdzgeieK2Y1+1cpdxJx8yXbK/m57nRhKSmk1GB2tO4lbLBtlkfPQTDKfe4Xqv1ASWPpayPEig==
dependencies:
- colorette "^1.2.2"
- nanoid "^3.1.23"
- source-map "^0.6.1"
+ nanoid "^3.3.4"
+ picocolors "^1.0.0"
+ source-map-js "^1.0.2"
prelude-ls@^1.2.1:
version "1.2.1"
@@ -4459,16 +3693,6 @@ pretty-format@^28.1.0:
ansi-styles "^5.0.0"
react-is "^18.0.0"
-process-nextick-args@~2.0.0:
- version "2.0.1"
- resolved "https://registry.npmjs.org/process-nextick-args/-/process-nextick-args-2.0.1.tgz"
- integrity sha512-3ouUOpQhtgrbOa17J7+uxOTpITYWaGP7/AhoR3+A+/1e9skrzelGi/dXzEYyvbxubEF6Wn2ypscTKiKJFFn1ag==
-
-process@0.11.10, process@^0.11.10:
- version "0.11.10"
- resolved "https://registry.npmjs.org/process/-/process-0.11.10.tgz"
- integrity sha1-czIwDoQBYb2j5podHZGn1LwW8YI=
-
progress@^2.0.0:
version "2.0.3"
resolved "https://registry.npmjs.org/progress/-/progress-2.0.3.tgz"
@@ -4496,85 +3720,16 @@ psl@^1.1.33:
resolved "https://registry.npmjs.org/psl/-/psl-1.8.0.tgz"
integrity sha512-RIdOzyoavK+hA18OGGWDqUTsCLhtA7IcZ/6NCs4fFJaHBDab+pDDmDIByWFRQJq2Cd7r1OoQxBGKOaztq+hjIQ==
-public-encrypt@^4.0.0:
- version "4.0.3"
- resolved "https://registry.npmjs.org/public-encrypt/-/public-encrypt-4.0.3.tgz"
- integrity sha512-zVpa8oKZSz5bTMTFClc1fQOnyyEzpl5ozpi1B5YcvBrdohMjH2rfsBtyXcuNuwjsDIXmBYlF2N5FlJYhR29t8Q==
- dependencies:
- bn.js "^4.1.0"
- browserify-rsa "^4.0.0"
- create-hash "^1.1.0"
- parse-asn1 "^5.0.0"
- randombytes "^2.0.1"
- safe-buffer "^5.1.2"
-
-punycode@1.3.2:
- version "1.3.2"
- resolved "https://registry.npmjs.org/punycode/-/punycode-1.3.2.tgz"
- integrity sha1-llOgNvt8HuQjQvIyXM7v6jkmxI0=
-
-punycode@^1.2.4:
- version "1.4.1"
- resolved "https://registry.npmjs.org/punycode/-/punycode-1.4.1.tgz"
- integrity sha1-wNWmOycYgArY4esPpSachN1BhF4=
-
punycode@^2.1.0, punycode@^2.1.1:
version "2.1.1"
resolved "https://registry.npmjs.org/punycode/-/punycode-2.1.1.tgz"
integrity sha512-XRsRjdf+j5ml+y/6GKHPZbrF/8p2Yga0JPtdqTIY2Xe5ohJPD9saDJJLPvp9+NSBprVvevdXZybnj2cv8OEd0A==
-querystring-es3@0.2.1, querystring-es3@^0.2.0:
- version "0.2.1"
- resolved "https://registry.npmjs.org/querystring-es3/-/querystring-es3-0.2.1.tgz"
- integrity sha1-nsYfeQSYdXB9aUFFlv2Qek1xHnM=
-
-querystring@0.2.0:
- version "0.2.0"
- resolved "https://registry.npmjs.org/querystring/-/querystring-0.2.0.tgz"
- integrity sha1-sgmEkgO7Jd+CDadW50cAWHhSFiA=
-
-querystring@^0.2.0:
- version "0.2.1"
- resolved "https://registry.npmjs.org/querystring/-/querystring-0.2.1.tgz"
- integrity sha512-wkvS7mL/JMugcup3/rMitHmd9ecIGd2lhFhK9N3UUQ450h66d1r3Y9nvXzQAW1Lq+wyx61k/1pfKS5KuKiyEbg==
-
queue-microtask@^1.2.2:
version "1.2.3"
resolved "https://registry.npmjs.org/queue-microtask/-/queue-microtask-1.2.3.tgz"
integrity sha512-NuaNSa6flKT5JaSYQzJok04JzTL1CA6aGhv5rfLW3PgqA+M2ChpZQnAC8h8i4ZFkBS8X5RqkDBHA7r4hej3K9A==
-queue@6.0.2:
- version "6.0.2"
- resolved "https://registry.npmjs.org/queue/-/queue-6.0.2.tgz"
- integrity sha512-iHZWu+q3IdFZFX36ro/lKBkSvfkztY5Y7HMiPlOUjhupPcG2JMfst2KKEpu5XndviX/3UhFbRngUPNKtgvtZiA==
- dependencies:
- inherits "~2.0.3"
-
-randombytes@^2.0.0, randombytes@^2.0.1, randombytes@^2.0.5:
- version "2.1.0"
- resolved "https://registry.npmjs.org/randombytes/-/randombytes-2.1.0.tgz"
- integrity sha512-vYl3iOX+4CKUWuxGi9Ukhie6fsqXqS9FE2Zaic4tNFD2N2QQaXOMFbuKK4QmDHC0JO6B1Zp41J0LpT0oR68amQ==
- dependencies:
- safe-buffer "^5.1.0"
-
-randomfill@^1.0.3:
- version "1.0.4"
- resolved "https://registry.npmjs.org/randomfill/-/randomfill-1.0.4.tgz"
- integrity sha512-87lcbR8+MhcWcUiQ+9e+Rwx8MyR2P7qnt15ynUlbm3TU/fjbgz4GsvfSUDTemtCCtVCqb4ZcEFlyPNTh9bBTLw==
- dependencies:
- randombytes "^2.0.5"
- safe-buffer "^5.1.0"
-
-raw-body@2.4.1:
- version "2.4.1"
- resolved "https://registry.npmjs.org/raw-body/-/raw-body-2.4.1.tgz"
- integrity sha512-9WmIKF6mkvA0SLmA2Knm9+qj89e+j1zqgyn8aXGd7+nAduPoqgI9lO57SAZNn/Byzo5P7JhXTyg9PzaJbH73bA==
- dependencies:
- bytes "3.1.0"
- http-errors "1.7.3"
- iconv-lite "0.4.24"
- unpipe "1.0.0"
-
react-dom@^17.0.1:
version "17.0.2"
resolved "https://registry.npmjs.org/react-dom/-/react-dom-17.0.2.tgz"
@@ -4584,26 +3739,21 @@ react-dom@^17.0.1:
object-assign "^4.1.1"
scheduler "^0.20.2"
-react-is@17.0.2, react-is@^17.0.1:
- version "17.0.2"
- resolved "https://registry.npmjs.org/react-is/-/react-is-17.0.2.tgz"
- integrity sha512-w2GsyukL62IJnlaff/nRegPQR94C/XXamvMWmSHRJ4y7Ts/4ocGRmTHvOs8PSE6pB3dWOrD/nueuU5sduBsQ4w==
-
react-is@^16.8.1:
version "16.13.1"
resolved "https://registry.npmjs.org/react-is/-/react-is-16.13.1.tgz"
integrity sha512-24e6ynE2H+OKt4kqsOvNd8kBpV65zoxbA4BVsEOB3ARVWQki/DHzaUoC5KuON/BiccDaCCTZBuOcfZs70kR8bQ==
+react-is@^17.0.1:
+ version "17.0.2"
+ resolved "https://registry.npmjs.org/react-is/-/react-is-17.0.2.tgz"
+ integrity sha512-w2GsyukL62IJnlaff/nRegPQR94C/XXamvMWmSHRJ4y7Ts/4ocGRmTHvOs8PSE6pB3dWOrD/nueuU5sduBsQ4w==
+
react-is@^18.0.0:
version "18.1.0"
resolved "https://registry.yarnpkg.com/react-is/-/react-is-18.1.0.tgz#61aaed3096d30eacf2a2127118b5b41387d32a67"
integrity sha512-Fl7FuabXsJnV5Q1qIOQwx/sagGF18kogb4gpfcG4gjLBWO0WDiiz1ko/ExayuxE7InyQkBLkxRFG5oxY6Uu3Kg==
-react-refresh@0.8.3:
- version "0.8.3"
- resolved "https://registry.npmjs.org/react-refresh/-/react-refresh-0.8.3.tgz"
- integrity sha512-X8jZHc7nCMjaCqoU+V2I0cOhNW+QMBwSUkeXnTi8IPe6zaRWfn60ZzvFDZqWPfmSJfjub7dDW1SP0jaHWLu/hg==
-
react@^17.0.2:
version "17.0.2"
resolved "https://registry.npmjs.org/react/-/react-17.0.2.tgz"
@@ -4612,35 +3762,6 @@ react@^17.0.2:
loose-envify "^1.1.0"
object-assign "^4.1.1"
-readable-stream@^2.0.2, readable-stream@^2.3.3, readable-stream@^2.3.6:
- version "2.3.7"
- resolved "https://registry.npmjs.org/readable-stream/-/readable-stream-2.3.7.tgz"
- integrity sha512-Ebho8K4jIbHAxnuxi7o42OrZgF/ZTNcsZj6nRKyUmkhLFq8CHItp/fy6hQZuZmP/n3yZ9VBUbp4zz/mX8hmYPw==
- dependencies:
- core-util-is "~1.0.0"
- inherits "~2.0.3"
- isarray "~1.0.0"
- process-nextick-args "~2.0.0"
- safe-buffer "~5.1.1"
- string_decoder "~1.1.1"
- util-deprecate "~1.0.1"
-
-readable-stream@^3.5.0, readable-stream@^3.6.0:
- version "3.6.0"
- resolved "https://registry.npmjs.org/readable-stream/-/readable-stream-3.6.0.tgz"
- integrity sha512-BViHy7LKeTz4oNnkcLJ+lVSL6vpiFeX6/d3oSH8zCW7UxP2onchk+vTGB143xuFjHS3deTgkKoXXymXqymiIdA==
- dependencies:
- inherits "^2.0.3"
- string_decoder "^1.1.1"
- util-deprecate "^1.0.1"
-
-readdirp@~3.5.0:
- version "3.5.0"
- resolved "https://registry.npmjs.org/readdirp/-/readdirp-3.5.0.tgz"
- integrity sha512-cMhu7c/8rdhkHXWsY+osBhfSy0JikwpHK/5+imo+LpeasTF8ouErHrlYkwT0++njiyuDvc7OFY5T3ukvZ8qmFQ==
- dependencies:
- picomatch "^2.2.1"
-
regenerator-runtime@^0.13.4:
version "0.13.7"
resolved "https://registry.npmjs.org/regenerator-runtime/-/regenerator-runtime-0.13.7.tgz"
@@ -4733,14 +3854,6 @@ rimraf@^3.0.0, rimraf@^3.0.2:
dependencies:
glob "^7.1.3"
-ripemd160@^2.0.0, ripemd160@^2.0.1:
- version "2.0.2"
- resolved "https://registry.npmjs.org/ripemd160/-/ripemd160-2.0.2.tgz"
- integrity sha512-ii4iagi25WusVoiC4B4lq7pbXfAp3D9v5CwfkY33vffw2+pkDjY1D8GaN7spsxvCSx8dkPqOZCEZyfxcmJG2IA==
- dependencies:
- hash-base "^3.0.0"
- inherits "^2.0.1"
-
run-parallel@^1.1.9:
version "1.2.0"
resolved "https://registry.npmjs.org/run-parallel/-/run-parallel-1.2.0.tgz"
@@ -4755,17 +3868,12 @@ rxjs@^7.5.4:
dependencies:
tslib "^2.1.0"
-safe-buffer@^5.0.1, safe-buffer@^5.1.0, safe-buffer@^5.1.1, safe-buffer@^5.1.2, safe-buffer@~5.1.0, safe-buffer@~5.1.1:
+safe-buffer@~5.1.1:
version "5.1.2"
resolved "https://registry.npmjs.org/safe-buffer/-/safe-buffer-5.1.2.tgz"
integrity sha512-Gd2UZBJDkXlY7GbJxfsE8/nvKkUEU1G38c1siN6QP6a9PT9MmHB8GnpscSmMJSoF8LOIrt8ud/wPtojys4G6+g==
-safe-buffer@^5.2.0, safe-buffer@~5.2.0:
- version "5.2.1"
- resolved "https://registry.npmjs.org/safe-buffer/-/safe-buffer-5.2.1.tgz"
- integrity sha512-rp3So07KcdmmKbGvgaNxQSJr7bGVSVk5S9Eq1F+ppbRo70+YeaDxkw5Dd8NPN+GD6bjnYm2VuPuCXmpuYvmCXQ==
-
-"safer-buffer@>= 2.1.2 < 3", "safer-buffer@>= 2.1.2 < 3.0.0", safer-buffer@^2.1.0:
+"safer-buffer@>= 2.1.2 < 3":
version "2.1.2"
resolved "https://registry.npmjs.org/safer-buffer/-/safer-buffer-2.1.2.tgz"
integrity sha512-YZo3K82SD7Riyi0E1EQPojLz7kpepnSQI9IyPbHHg1XXXevb5dJI7tpyN2ADxGcQbHG7vcyRHk0cbwqcQriUtg==
@@ -4797,24 +3905,6 @@ semver@^7.2.1, semver@^7.3.2, semver@^7.3.5:
dependencies:
lru-cache "^6.0.0"
-setimmediate@^1.0.4:
- version "1.0.5"
- resolved "https://registry.npmjs.org/setimmediate/-/setimmediate-1.0.5.tgz"
- integrity sha1-KQy7Iy4waULX1+qbg3Mqt4VvgoU=
-
-setprototypeof@1.1.1:
- version "1.1.1"
- resolved "https://registry.npmjs.org/setprototypeof/-/setprototypeof-1.1.1.tgz"
- integrity sha512-JvdAWfbXeIGaZ9cILp38HntZSFSo3mWg6xGcJJsd+d4aRMOqauag1C63dJfDw7OaMYwEbHMOxEZ1lqVRYP2OAw==
-
-sha.js@^2.4.0, sha.js@^2.4.8:
- version "2.4.11"
- resolved "https://registry.npmjs.org/sha.js/-/sha.js-2.4.11.tgz"
- integrity sha512-QMEp5B7cftE7APOjk5Y6xgrbWu+WkLVQwk8JNjZ8nKRciZaByEW6MubieAiToS7+dwvrjGhH8jRXz3MVd0AYqQ==
- dependencies:
- inherits "^2.0.1"
- safe-buffer "^5.0.1"
-
shebang-command@^2.0.0:
version "2.0.0"
resolved "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz"
@@ -4827,11 +3917,6 @@ shebang-regex@^3.0.0:
resolved "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz"
integrity sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==
-shell-quote@1.7.2:
- version "1.7.2"
- resolved "https://registry.npmjs.org/shell-quote/-/shell-quote-1.7.2.tgz"
- integrity sha512-mRz/m/JVscCrkMyPqHc/bczi3OQHkLTqXHEFu0zDhK/qfv3UcOA4SVmRCLmos4bhjr9ekVQubj/R7waKapmiQg==
-
side-channel@^1.0.4:
version "1.0.4"
resolved "https://registry.npmjs.org/side-channel/-/side-channel-1.0.4.tgz"
@@ -4887,6 +3972,11 @@ slice-ansi@^5.0.0:
ansi-styles "^6.0.0"
is-fullwidth-code-point "^4.0.0"
+source-map-js@^1.0.2:
+ version "1.0.2"
+ resolved "https://registry.yarnpkg.com/source-map-js/-/source-map-js-1.0.2.tgz#adbc361d9c62df380125e7f161f71c826f1e490c"
+ integrity sha512-R0XvVJ9WusLiqTCEiGCmICCMplcCkIwwR11mOSD9CR5u+IXYdiseeEuXCVAjS54zqwkLcPNnmU4OeJ6tUrWhDw==
+
source-map-support@^0.5.6:
version "0.5.19"
resolved "https://registry.npmjs.org/source-map-support/-/source-map-support-0.5.19.tgz"
@@ -4895,18 +3985,6 @@ source-map-support@^0.5.6:
buffer-from "^1.0.0"
source-map "^0.6.0"
-source-map@0.7.3, source-map@^0.7.3:
- version "0.7.3"
- resolved "https://registry.npmjs.org/source-map/-/source-map-0.7.3.tgz"
- integrity sha512-CkCj6giN3S+n9qrYiBTX5gystlENnRW5jZeNLHpe6aue+SrHcG5VYwujhW9s4dY31mEGsxBDrHR6oI69fTXsaQ==
-
-source-map@0.8.0-beta.0:
- version "0.8.0-beta.0"
- resolved "https://registry.npmjs.org/source-map/-/source-map-0.8.0-beta.0.tgz"
- integrity sha512-2ymg6oRBpebeZi9UUNsgQ89bhx01TcTkmNTGnNO88imTmbSgy4nfujrgVEFKWpMTEGA11EDkTt7mqObTPdigIA==
- dependencies:
- whatwg-url "^7.0.0"
-
source-map@^0.5.0:
version "0.5.7"
resolved "https://registry.npmjs.org/source-map/-/source-map-0.5.7.tgz"
@@ -4917,6 +3995,11 @@ source-map@^0.6.0, source-map@^0.6.1, source-map@~0.6.1:
resolved "https://registry.npmjs.org/source-map/-/source-map-0.6.1.tgz"
integrity sha512-UjgapumWlbMhkBgzT7Ykc5YXUT46F0iKu8SGXq0bcwP5dz/h0Plj6enJqjz1Zbq2l5WaqYnrVbwWOWMyF3F47g==
+source-map@^0.7.3:
+ version "0.7.3"
+ resolved "https://registry.npmjs.org/source-map/-/source-map-0.7.3.tgz"
+ integrity sha512-CkCj6giN3S+n9qrYiBTX5gystlENnRW5jZeNLHpe6aue+SrHcG5VYwujhW9s4dY31mEGsxBDrHR6oI69fTXsaQ==
+
sourcemap-codec@1.4.8:
version "1.4.8"
resolved "https://registry.yarnpkg.com/sourcemap-codec/-/sourcemap-codec-1.4.8.tgz#ea804bd94857402e6992d05a38ef1ae35a9ab4c4"
@@ -4934,72 +4017,11 @@ stack-utils@^2.0.3:
dependencies:
escape-string-regexp "^2.0.0"
-stacktrace-parser@0.1.10:
- version "0.1.10"
- resolved "https://registry.npmjs.org/stacktrace-parser/-/stacktrace-parser-0.1.10.tgz"
- integrity sha512-KJP1OCML99+8fhOHxwwzyWrlUuVX5GQ0ZpJTd1DFXhdkrvg1szxfHhawXUZ3g9TkXORQd4/WG68jMlQZ2p8wlg==
- dependencies:
- type-fest "^0.7.1"
-
-"statuses@>= 1.5.0 < 2":
- version "1.5.0"
- resolved "https://registry.npmjs.org/statuses/-/statuses-1.5.0.tgz"
- integrity sha1-Fhx9rBd2Wf2YEfQ3cfqZOBR4Yow=
-
-stream-browserify@3.0.0:
- version "3.0.0"
- resolved "https://registry.npmjs.org/stream-browserify/-/stream-browserify-3.0.0.tgz"
- integrity sha512-H73RAHsVBapbim0tU2JwwOiXUj+fikfiaoYAKHF3VJfA0pe2BCzkhAHBlLG6REzE+2WNZcxOXjK7lkso+9euLA==
- dependencies:
- inherits "~2.0.4"
- readable-stream "^3.5.0"
-
-stream-browserify@^2.0.1:
- version "2.0.2"
- resolved "https://registry.npmjs.org/stream-browserify/-/stream-browserify-2.0.2.tgz"
- integrity sha512-nX6hmklHs/gr2FuxYDltq8fJA1GDlxKQCz8O/IM4atRqBH8OORmBNgfvW5gG10GT/qQ9u0CzIvr2X5Pkt6ntqg==
- dependencies:
- inherits "~2.0.1"
- readable-stream "^2.0.2"
-
-stream-http@3.1.1:
- version "3.1.1"
- resolved "https://registry.npmjs.org/stream-http/-/stream-http-3.1.1.tgz"
- integrity sha512-S7OqaYu0EkFpgeGFb/NPOoPLxFko7TPqtEeFg5DXPB4v/KETHG0Ln6fRFrNezoelpaDKmycEmmZ81cC9DAwgYg==
- dependencies:
- builtin-status-codes "^3.0.0"
- inherits "^2.0.4"
- readable-stream "^3.6.0"
- xtend "^4.0.2"
-
-stream-http@^2.7.2:
- version "2.8.3"
- resolved "https://registry.npmjs.org/stream-http/-/stream-http-2.8.3.tgz"
- integrity sha512-+TSkfINHDo4J+ZobQLWiMouQYB+UVYFttRA94FpEzzJ7ZdqcL4uUUQ7WkdkI4DSozGmgBUE/a47L+38PenXhUw==
- dependencies:
- builtin-status-codes "^3.0.0"
- inherits "^2.0.1"
- readable-stream "^2.3.6"
- to-arraybuffer "^1.0.0"
- xtend "^4.0.0"
-
-stream-parser@^0.3.1:
- version "0.3.1"
- resolved "https://registry.npmjs.org/stream-parser/-/stream-parser-0.3.1.tgz"
- integrity sha1-FhhUhpRCACGhGC/wrxkRwSl2F3M=
- dependencies:
- debug "2"
-
string-argv@^0.3.1:
version "0.3.1"
resolved "https://registry.npmjs.org/string-argv/-/string-argv-0.3.1.tgz"
integrity sha512-a1uQGz7IyVy9YwhqjZIZu1c8JO8dNIe20xBmSS6qu9kv++k3JGzCVmprbNN5Kn+BgzD5E7YYwg1CcjuJMRNsvg==
-string-hash@1.1.3:
- version "1.1.3"
- resolved "https://registry.npmjs.org/string-hash/-/string-hash-1.1.3.tgz"
- integrity sha1-6Kr8CsGFW0Zmkp7X3RJ1311sgRs=
-
string-length@^4.0.1:
version "4.0.2"
resolved "https://registry.npmjs.org/string-length/-/string-length-4.0.2.tgz"
@@ -5073,27 +4095,6 @@ string.prototype.trimstart@^1.0.4:
call-bind "^1.0.2"
define-properties "^1.1.3"
-string_decoder@1.3.0, string_decoder@^1.1.1:
- version "1.3.0"
- resolved "https://registry.npmjs.org/string_decoder/-/string_decoder-1.3.0.tgz"
- integrity sha512-hkRX8U1WjJFd8LsDJ2yQ/wWWxaopEsABU1XfkM8A+j0+85JAGppt16cr1Whg6KIbb4okU6Mql6BOj+uup/wKeA==
- dependencies:
- safe-buffer "~5.2.0"
-
-string_decoder@^1.0.0, string_decoder@~1.1.1:
- version "1.1.1"
- resolved "https://registry.npmjs.org/string_decoder/-/string_decoder-1.1.1.tgz"
- integrity sha512-n/ShnvDi6FHbbVfviro+WojiFzv+s8MPMHBczVePfUpDJLwoLT0ht1l4YwBCbi8pJAveEEdnkHyPyTP/mzRfwg==
- dependencies:
- safe-buffer "~5.1.0"
-
-strip-ansi@6.0.0:
- version "6.0.0"
- resolved "https://registry.npmjs.org/strip-ansi/-/strip-ansi-6.0.0.tgz"
- integrity sha512-AuvKTrTfQNYNIctbR1K/YGTR1756GycPsg7b9bdV9Duqur4gv6aKqHXah67Z8ImS7WEz5QVcOtlfW2rZEugt6w==
- dependencies:
- ansi-regex "^5.0.0"
-
strip-ansi@^6.0.0, strip-ansi@^6.0.1:
version "6.0.1"
resolved "https://registry.npmjs.org/strip-ansi/-/strip-ansi-6.0.1.tgz"
@@ -5128,29 +4129,10 @@ strip-json-comments@^3.1.0, strip-json-comments@^3.1.1:
resolved "https://registry.npmjs.org/strip-json-comments/-/strip-json-comments-3.1.1.tgz"
integrity sha512-6fPc+R4ihwqP6N/aIv2f1gMH8lOVtWQHoqC4yK6oSDVVocumAsfCqjkXnqiYMhmMwS/mEHLp7Vehlt3ql6lEig==
-styled-jsx@4.0.1:
- version "4.0.1"
- resolved "https://registry.npmjs.org/styled-jsx/-/styled-jsx-4.0.1.tgz"
- integrity sha512-Gcb49/dRB1k8B4hdK8vhW27Rlb2zujCk1fISrizCcToIs+55B4vmUM0N9Gi4nnVfFZWe55jRdWpAqH1ldAKWvQ==
- dependencies:
- "@babel/plugin-syntax-jsx" "7.14.5"
- "@babel/types" "7.15.0"
- convert-source-map "1.7.0"
- loader-utils "1.2.3"
- source-map "0.7.3"
- string-hash "1.1.3"
- stylis "3.5.4"
- stylis-rule-sheet "0.0.10"
-
-stylis-rule-sheet@0.0.10:
- version "0.0.10"
- resolved "https://registry.npmjs.org/stylis-rule-sheet/-/stylis-rule-sheet-0.0.10.tgz"
- integrity sha512-nTbZoaqoBnmK+ptANthb10ZRZOGC+EmTLLUxeYIuHNkEKcmKgXX1XWKkUBT2Ac4es3NybooPe0SmvKdhKJZAuw==
-
-stylis@3.5.4:
- version "3.5.4"
- resolved "https://registry.npmjs.org/stylis/-/stylis-3.5.4.tgz"
- integrity sha512-8/3pSmthWM7lsPBKv7NXkzn2Uc9W7NotcwGNpJaa3k7WMM1XDCA4MgT5k/8BIexd5ydZdboXtU90XH9Ec4Bv/Q==
+styled-jsx@5.0.7:
+ version "5.0.7"
+ resolved "https://registry.yarnpkg.com/styled-jsx/-/styled-jsx-5.0.7.tgz#be44afc53771b983769ac654d355ca8d019dff48"
+ integrity sha512-b3sUzamS086YLRuvnaDigdAewz1/EFYlHpYBP5mZovKEdQQOIIYq8lApylub3HHZ6xFjV051kkGU7cudJmrXEA==
supports-color@^5.3.0:
version "5.5.0"
@@ -5239,23 +4221,11 @@ through@^2.3.8:
resolved "https://registry.npmjs.org/through/-/through-2.3.8.tgz"
integrity sha1-DdTJ/6q8NXlgsbckEV1+Doai4fU=
-timers-browserify@2.0.12, timers-browserify@^2.0.4:
- version "2.0.12"
- resolved "https://registry.npmjs.org/timers-browserify/-/timers-browserify-2.0.12.tgz"
- integrity sha512-9phl76Cqm6FhSX9Xe1ZUAMLtm1BLkKj2Qd5ApyWkXzsMRaA7dgr81kf4wJmQf/hAvg8EEyJxDo3du/0KlhPiKQ==
- dependencies:
- setimmediate "^1.0.4"
-
tmpl@1.0.x:
version "1.0.5"
resolved "https://registry.npmjs.org/tmpl/-/tmpl-1.0.5.tgz"
integrity sha512-3f0uOEAQwIqGuWW2MVzYg8fV/QNnc/IpuJNG837rLuczAaLVHslWHZQj4IGiEl5Hs3kkbhwL9Ab7Hrsmuj+Smw==
-to-arraybuffer@^1.0.0:
- version "1.0.1"
- resolved "https://registry.npmjs.org/to-arraybuffer/-/to-arraybuffer-1.0.1.tgz"
- integrity sha1-fSKbH8xjfkZsoIEYCDanqr/4P0M=
-
to-fast-properties@^2.0.0:
version "2.0.0"
resolved "https://registry.npmjs.org/to-fast-properties/-/to-fast-properties-2.0.0.tgz"
@@ -5268,11 +4238,6 @@ to-regex-range@^5.0.1:
dependencies:
is-number "^7.0.0"
-toidentifier@1.0.0:
- version "1.0.0"
- resolved "https://registry.npmjs.org/toidentifier/-/toidentifier-1.0.0.tgz"
- integrity sha512-yaOH/Pk/VEhBWWTlhI+qXxDFXlejDGcQipMlyxda9nthulaxLZUNcUqFxokp0vcYnvteJln5FNQDRrxj3YcbVw==
-
tough-cookie@^4.0.0:
version "4.0.0"
resolved "https://registry.npmjs.org/tough-cookie/-/tough-cookie-4.0.0.tgz"
@@ -5282,13 +4247,6 @@ tough-cookie@^4.0.0:
punycode "^2.1.1"
universalify "^0.1.2"
-tr46@^1.0.1:
- version "1.0.1"
- resolved "https://registry.npmjs.org/tr46/-/tr46-1.0.1.tgz"
- integrity sha1-qLE/1r/SSJUZZ0zN5VujaTtwbQk=
- dependencies:
- punycode "^2.1.0"
-
tr46@^2.0.2:
version "2.0.2"
resolved "https://registry.npmjs.org/tr46/-/tr46-2.0.2.tgz"
@@ -5301,11 +4259,6 @@ tr46@~0.0.3:
resolved "https://registry.npmjs.org/tr46/-/tr46-0.0.3.tgz"
integrity sha1-gYT9NH2snNwYWZLzpmIuFLnZq2o=
-ts-pnp@^1.1.6:
- version "1.2.0"
- resolved "https://registry.npmjs.org/ts-pnp/-/ts-pnp-1.2.0.tgz"
- integrity sha512-csd+vJOb/gkzvcCHgTGSChYpy5f1/XKNsmvBGO4JXS+z1v2HobugDz4s1IeFXM3wZB44uczs+eazB5Q/ccdhQw==
-
tsconfig-paths@^3.14.1:
version "3.14.1"
resolved "https://registry.yarnpkg.com/tsconfig-paths/-/tsconfig-paths-3.14.1.tgz#ba0734599e8ea36c862798e920bcf163277b137a"
@@ -5326,6 +4279,11 @@ tslib@^2.1.0:
resolved "https://registry.yarnpkg.com/tslib/-/tslib-2.3.1.tgz#e8a335add5ceae51aa261d32a490158ef042ef01"
integrity sha512-77EbyPPpMz+FRFRuAFlWMtmgUWGe9UOG2Z25NqCwiIjRhOf5iKGuzSe5P2w1laq+FkRy4p+PCuVkJSGkzTEKVw==
+tslib@^2.4.0:
+ version "2.6.2"
+ resolved "https://registry.yarnpkg.com/tslib/-/tslib-2.6.2.tgz#703ac29425e7b37cd6fd456e92404d46d1f3e4ae"
+ integrity sha512-AEYxH93jGFPn/a2iVAwW87VuUIkR1FVUKB77NwMF7nBTDkDrrT/Hpt/IrCJ0QXhW27jTBDcf5ZY7w6RiqTMw2Q==
+
tsutils@^3.21.0:
version "3.21.0"
resolved "https://registry.npmjs.org/tsutils/-/tsutils-3.21.0.tgz"
@@ -5333,16 +4291,6 @@ tsutils@^3.21.0:
dependencies:
tslib "^1.8.1"
-tty-browserify@0.0.0:
- version "0.0.0"
- resolved "https://registry.npmjs.org/tty-browserify/-/tty-browserify-0.0.0.tgz"
- integrity sha1-oVe6QC2iTpv5V/mqadUk7tQpAaY=
-
-tty-browserify@0.0.1:
- version "0.0.1"
- resolved "https://registry.npmjs.org/tty-browserify/-/tty-browserify-0.0.1.tgz"
- integrity sha512-C3TaO7K81YvjCgQH9Q1S3R3P3BtN3RIM8n+OvX4il1K1zgE8ZhI0op7kClgkxtutIE8hQrcrHBXvIheqKUUCxw==
-
type-check@^0.4.0, type-check@~0.4.0:
version "0.4.0"
resolved "https://registry.npmjs.org/type-check/-/type-check-0.4.0.tgz"
@@ -5372,11 +4320,6 @@ type-fest@^0.21.3:
resolved "https://registry.npmjs.org/type-fest/-/type-fest-0.21.3.tgz"
integrity sha512-t0rzBq87m3fVcduHDUFhKmyyX+9eo6WQjZvf51Ea/M0Q7+T374Jp1aUiyUl0GKxp8M/OETVHSDvmkyPgvX+X2w==
-type-fest@^0.7.1:
- version "0.7.1"
- resolved "https://registry.npmjs.org/type-fest/-/type-fest-0.7.1.tgz"
- integrity sha512-Ne2YiiGN8bmrmJJEuTWTLJR32nh/JdL1+PSicowtNb0WFpn59GK8/lfD61bVtzguz7b3PBt74nxpv/Pw5po5Rg==
-
typedarray-to-buffer@^3.1.5:
version "3.1.5"
resolved "https://registry.npmjs.org/typedarray-to-buffer/-/typedarray-to-buffer-3.1.5.tgz"
@@ -5409,11 +4352,6 @@ universalify@^0.1.2:
resolved "https://registry.npmjs.org/universalify/-/universalify-0.1.2.tgz"
integrity sha512-rBJeI5CXAlmy1pV+617WB9J63U6XcazHHF2f2dbJix4XzpUF0RS3Zbj0FGIOCAva5P/d/GBOYaACQ1w+0azUkg==
-unpipe@1.0.0:
- version "1.0.0"
- resolved "https://registry.npmjs.org/unpipe/-/unpipe-1.0.0.tgz"
- integrity sha1-sr9O6FFKrmFltIF4KdIbLvSZBOw=
-
uri-js@^4.2.2:
version "4.4.1"
resolved "https://registry.npmjs.org/uri-js/-/uri-js-4.4.1.tgz"
@@ -5421,51 +4359,10 @@ uri-js@^4.2.2:
dependencies:
punycode "^2.1.0"
-url@^0.11.0:
- version "0.11.0"
- resolved "https://registry.npmjs.org/url/-/url-0.11.0.tgz"
- integrity sha1-ODjpfPxgUh63PFJajlW/3Z4uKPE=
- dependencies:
- punycode "1.3.2"
- querystring "0.2.0"
-
-use-subscription@1.5.1:
- version "1.5.1"
- resolved "https://registry.npmjs.org/use-subscription/-/use-subscription-1.5.1.tgz"
- integrity sha512-Xv2a1P/yReAjAbhylMfFplFKj9GssgTwN7RlcTxBujFQcloStWNDQdc4g4NRWH9xS4i/FDk04vQBptAXoF3VcA==
- dependencies:
- object-assign "^4.1.1"
-
-util-deprecate@^1.0.1, util-deprecate@~1.0.1:
- version "1.0.2"
- resolved "https://registry.npmjs.org/util-deprecate/-/util-deprecate-1.0.2.tgz"
- integrity sha1-RQ1Nyfpw3nMnYvvS1KKJgUGaDM8=
-
-util@0.10.3:
- version "0.10.3"
- resolved "https://registry.npmjs.org/util/-/util-0.10.3.tgz"
- integrity sha1-evsa/lCAUkZInj23/g7TeTNqwPk=
- dependencies:
- inherits "2.0.1"
-
-util@0.12.4, util@^0.12.0:
- version "0.12.4"
- resolved "https://registry.npmjs.org/util/-/util-0.12.4.tgz"
- integrity sha512-bxZ9qtSlGUWSOy9Qa9Xgk11kSslpuZwaxCg4sNIDj6FLucDab2JxnHwyNTCpHMtK1MjoQiWQ6DiUMZYbSrO+Sw==
- dependencies:
- inherits "^2.0.3"
- is-arguments "^1.0.4"
- is-generator-function "^1.0.7"
- is-typed-array "^1.1.3"
- safe-buffer "^5.1.2"
- which-typed-array "^1.1.2"
-
-util@^0.11.0:
- version "0.11.1"
- resolved "https://registry.npmjs.org/util/-/util-0.11.1.tgz"
- integrity sha512-HShAsny+zS2TZfaXxD9tYj4HQGlBezXZMZuM/S5PKLLoZkShZiGk9o5CzukI1LVHZvjdvZ2Sj1aW/Ndn2NB/HQ==
- dependencies:
- inherits "2.0.3"
+use-sync-external-store@1.2.0:
+ version "1.2.0"
+ resolved "https://registry.yarnpkg.com/use-sync-external-store/-/use-sync-external-store-1.2.0.tgz#7dbefd6ef3fe4e767a0cf5d7287aacfb5846928a"
+ integrity sha512-eEgnFxGQ1Ife9bzYs6VLi8/4X6CObHMw9Qr9tPY43iKwsPw8xE8+EFsf/2cFZ5S3esXgpWgtSCtLNS41F+sKPA==
v8-compile-cache@^2.0.3:
version "2.3.0"
@@ -5481,11 +4378,6 @@ v8-to-istanbul@^8.1.0:
convert-source-map "^1.6.0"
source-map "^0.7.3"
-vm-browserify@1.1.2, vm-browserify@^1.0.1:
- version "1.1.2"
- resolved "https://registry.npmjs.org/vm-browserify/-/vm-browserify-1.1.2.tgz"
- integrity sha512-2ham8XPWTONajOR0ohOKOHXkm3+gaBmGut3SRuu75xLd/RRaY6vqgh8NBYYk7+RW3u5AtzPQZG8F10LHkl0lAQ==
-
w3c-hr-time@^1.0.2:
version "1.0.2"
resolved "https://registry.npmjs.org/w3c-hr-time/-/w3c-hr-time-1.0.2.tgz"
@@ -5507,24 +4399,11 @@ walker@^1.0.7:
dependencies:
makeerror "1.0.x"
-watchpack@2.1.1:
- version "2.1.1"
- resolved "https://registry.npmjs.org/watchpack/-/watchpack-2.1.1.tgz"
- integrity sha512-Oo7LXCmc1eE1AjyuSBmtC3+Wy4HcV8PxWh2kP6fOl8yTlNS7r0K9l1ao2lrrUza7V39Y3D/BbJgY8VeSlc5JKw==
- dependencies:
- glob-to-regexp "^0.4.1"
- graceful-fs "^4.1.2"
-
webidl-conversions@^3.0.0:
version "3.0.1"
resolved "https://registry.npmjs.org/webidl-conversions/-/webidl-conversions-3.0.1.tgz"
integrity sha1-JFNCdeKnvGvnvIZhHMFq4KVlSHE=
-webidl-conversions@^4.0.2:
- version "4.0.2"
- resolved "https://registry.npmjs.org/webidl-conversions/-/webidl-conversions-4.0.2.tgz"
- integrity sha512-YQ+BmxuTgd6UXZW3+ICGfyqRyHXVlD5GtQr5+qjiNW7bF0cqrzX500HVXPBOvgXb5YnzDd+h0zqyv61KUD7+Sg==
-
webidl-conversions@^5.0.0:
version "5.0.0"
resolved "https://registry.npmjs.org/webidl-conversions/-/webidl-conversions-5.0.0.tgz"
@@ -5555,15 +4434,6 @@ whatwg-url@^5.0.0:
tr46 "~0.0.3"
webidl-conversions "^3.0.0"
-whatwg-url@^7.0.0:
- version "7.1.0"
- resolved "https://registry.npmjs.org/whatwg-url/-/whatwg-url-7.1.0.tgz"
- integrity sha512-WUu7Rg1DroM7oQvGWfOiAK21n74Gg+T4elXEQYkOhtyLeWiJFoOGLXPKI/9gzIie9CtwVLm8wtw6YJdKyxSjeg==
- dependencies:
- lodash.sortby "^4.7.0"
- tr46 "^1.0.1"
- webidl-conversions "^4.0.2"
-
whatwg-url@^8.0.0, whatwg-url@^8.5.0:
version "8.5.0"
resolved "https://registry.npmjs.org/whatwg-url/-/whatwg-url-8.5.0.tgz"
@@ -5584,19 +4454,6 @@ which-boxed-primitive@^1.0.2:
is-string "^1.0.5"
is-symbol "^1.0.3"
-which-typed-array@^1.1.2:
- version "1.1.4"
- resolved "https://registry.npmjs.org/which-typed-array/-/which-typed-array-1.1.4.tgz"
- integrity sha512-49E0SpUe90cjpoc7BOJwyPHRqSAd12c10Qm2amdEZrJPCY2NDxaW01zHITrem+rnETY3dwrbH3UUrUwagfCYDA==
- dependencies:
- available-typed-arrays "^1.0.2"
- call-bind "^1.0.0"
- es-abstract "^1.18.0-next.1"
- foreach "^2.0.5"
- function-bind "^1.1.1"
- has-symbols "^1.0.1"
- is-typed-array "^1.1.3"
-
which@^2.0.1:
version "2.0.2"
resolved "https://registry.npmjs.org/which/-/which-2.0.2.tgz"
@@ -5657,11 +4514,6 @@ xmlchars@^2.2.0:
resolved "https://registry.npmjs.org/xmlchars/-/xmlchars-2.2.0.tgz"
integrity sha512-JZnDKK8B0RCDw84FNdDAIpZK+JuJw+s7Lz8nksI7SIuU3UXJJslUthsi+uWBUYOwPFwW7W7PRLRfUKpxjtjFCw==
-xtend@^4.0.0, xtend@^4.0.2:
- version "4.0.2"
- resolved "https://registry.npmjs.org/xtend/-/xtend-4.0.2.tgz"
- integrity sha512-LKYU1iAXJXUgAXn9URjiu+MWhyUXHsvfp7mcuYm9dSUKK0/CjtrUwFAxD82/mCWbtLsGjFIad0wIsod4zrTAEQ==
-
y18n@^5.0.5:
version "5.0.8"
resolved "https://registry.npmjs.org/y18n/-/y18n-5.0.8.tgz"
@@ -5694,8 +4546,3 @@ yargs@^16.2.0:
string-width "^4.2.0"
y18n "^5.0.5"
yargs-parser "^20.2.2"
-
-yocto-queue@^0.1.0:
- version "0.1.0"
- resolved "https://registry.npmjs.org/yocto-queue/-/yocto-queue-0.1.0.tgz"
- integrity sha512-rVksvsnNCdJ/ohGc6xgPwyN8eheCxsiLM8mxuE/t/mOVqJewPuO1miLpTHQiRgTKCLexL4MeAFVagts7HmNZ2Q==