-
Notifications
You must be signed in to change notification settings - Fork 2
/
model_finish_file_data_upload_request.go
196 lines (162 loc) · 10.8 KB
/
model_finish_file_data_upload_request.go
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
/*
VRChat API Documentation
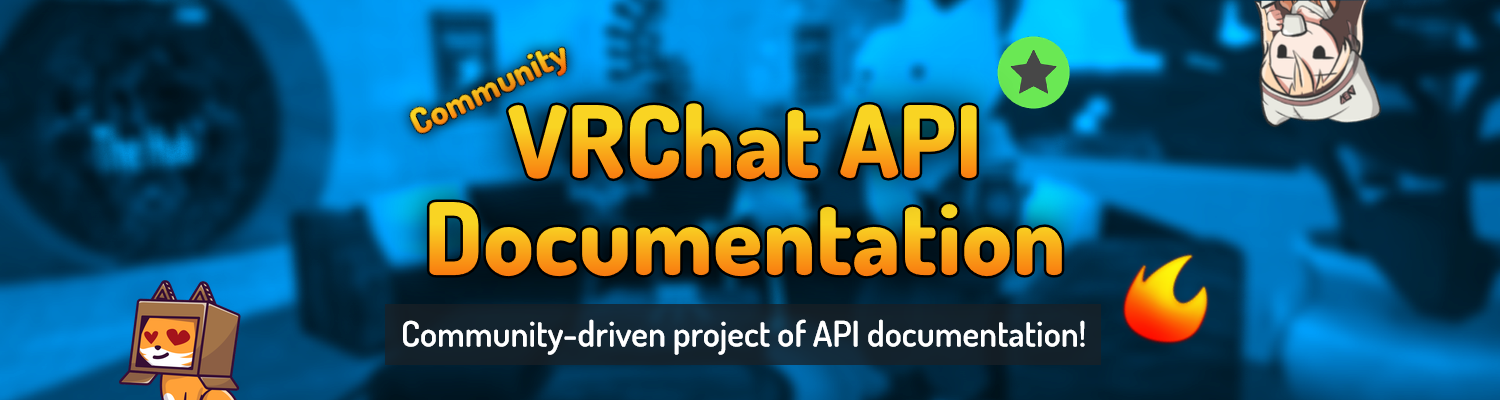 # Welcome to the VRChat API Before we begin, we would like to state this is a **COMMUNITY DRIVEN PROJECT**. This means that everything you read on here was written by the community itself and is **not** officially supported by VRChat. The documentation is provided \"AS IS\", and any action you take towards VRChat is completely your own responsibility. The documentation and additional libraries SHALL ONLY be used for applications interacting with VRChat's API in accordance with their [Terms of Service](https://hello.vrchat.com/legal) and [Community Guidelines](https://hello.vrchat.com/community-guidelines), and MUST NOT be used for modifying the client, \"avatar ripping\", or other illegal activities. Malicious usage or spamming the API may result in account termination. Certain parts of the API are also more sensitive than others, for example moderation, so please tread extra carefully and read the warnings when present.  Finally, use of the API using applications other than the approved methods (website, VRChat application, Unity SDK) is not officially supported. VRChat provides no guarantee or support for external applications using the API. Access to API endpoints may break **at any time, without notice**. Therefore, please **do not ping** VRChat Staff in the VRChat Discord if you are having API problems, as they do not provide API support. We will make a best effort in keeping this documentation and associated language libraries up to date, but things might be outdated or missing. If you find that something is no longer valid, please contact us on Discord or [create an issue](https://github.com/vrchatapi/specification/issues) and tell us so we can fix it. # Getting Started The VRChat API can be used to programmatically retrieve or update information regarding your profile, friends, avatars, worlds and more. The API consists of two parts, \"Photon\" which is only used in-game, and the \"Web API\" which is used by both the game and the website. This documentation focuses only on the Web API. The API is designed around the REST ideology, providing semi-simple and usually predictable URIs to access and modify objects. Requests support standard HTTP methods like GET, PUT, POST, and DELETE and standard status codes. Response bodies are always UTF-8 encoded JSON objects, unless explicitly documented otherwise. <div class=\"callout callout-error\"> <strong>🛑 Warning! Do not touch Photon!</strong><br> Photon is only used by the in-game client and should <b>not</b> be touched. Doing so may result in permanent account termination. </div> <div class=\"callout callout-info\"> <strong>ℹ️ API Key and Authentication</strong><br> The API Key has always been the same and is currently <code>JlE5Jldo5Jibnk5O5hTx6XVqsJu4WJ26</code>. Read <a href=\"#tag--authentication\">Authentication</a> for how to log in. </div> # Using the API For simply exploring what the API can do it is strongly recommended to download [Insomnia](https://insomnia.rest/download), a free and open-source API client that's great for sending requests to the API in an orderly fashion. Insomnia allows you to send data in the format that's required for VRChat's API. It is also possible to try out the API in your browser, by first logging in at [vrchat.com/home](https://vrchat.com/home/) and then going to [vrchat.com/api/1/auth/user](https://vrchat.com/api/1/auth/user), but the information will be much harder to work with. For more permanent operation such as software development it is instead recommended to use one of the existing language SDKs. This community project maintains API libraries in several languages, which allows you to interact with the API with simple function calls rather than having to implement the HTTP protocol yourself. Most of these libraries are automatically generated from the API specification, sometimes with additional helpful wrapper code to make usage easier. This allows them to be almost automatically updated and expanded upon as soon as a new feature is introduced in the specification itself. The libraries can be found on [GitHub](https://github.com/vrchatapi) or following: * [NodeJS (JavaScript)](https://www.npmjs.com/package/vrchat) * [Dart](https://pub.dev/packages/vrchat_dart) * [Rust](https://crates.io/crates/vrchatapi) * [C#](https://github.com/vrchatapi/vrchatapi-csharp) * [Python](https://github.com/vrchatapi/vrchatapi-python) # Pagination Most endpoints enforce pagination, meaning they will only return 10 entries by default, and never more than 100.<br> Using both the limit and offset parameters allows you to easily paginate through a large number of objects. | Query Parameter | Type | Description | | ----------|--|------- | | `n` | integer | The number of objects to return. This value often defaults to 10. Highest limit is always 100.| | `offset` | integer | A zero-based offset from the default object sorting.| If a request returns fewer objects than the `limit` parameter, there are no more items available to return. # Contribution Do you want to get involved in the documentation effort? Do you want to help improve one of the language API libraries? This project is an [OPEN Open Source Project](https://openopensource.org)! This means that individuals making significant and valuable contributions are given commit-access to the project. It also means we are very open and welcoming of new people making contributions, unlike some more guarded open-source projects. [](https://discord.gg/qjZE9C9fkB)
API version: 1.12.0
Contact: [email protected]
*/
// Code generated by OpenAPI Generator (https://openapi-generator.tech); DO NOT EDIT.
package vrchatapi
import (
"encoding/json"
)
// checks if the FinishFileDataUploadRequest type satisfies the MappedNullable interface at compile time
var _ MappedNullable = &FinishFileDataUploadRequest{}
// FinishFileDataUploadRequest
type FinishFileDataUploadRequest struct {
// Array of ETags uploaded.
Etags []string `json:"etags,omitempty"`
// Always a zero in string form, despite how many parts uploaded.
// Deprecated
NextPartNumber string `json:"nextPartNumber"`
// Always a zero in string form, despite how many parts uploaded.
// Deprecated
MaxParts string `json:"maxParts"`
}
// NewFinishFileDataUploadRequest instantiates a new FinishFileDataUploadRequest object
// This constructor will assign default values to properties that have it defined,
// and makes sure properties required by API are set, but the set of arguments
// will change when the set of required properties is changed
func NewFinishFileDataUploadRequest(nextPartNumber string, maxParts string) *FinishFileDataUploadRequest {
this := FinishFileDataUploadRequest{}
this.NextPartNumber = nextPartNumber
this.MaxParts = maxParts
return &this
}
// NewFinishFileDataUploadRequestWithDefaults instantiates a new FinishFileDataUploadRequest object
// This constructor will only assign default values to properties that have it defined,
// but it doesn't guarantee that properties required by API are set
func NewFinishFileDataUploadRequestWithDefaults() *FinishFileDataUploadRequest {
this := FinishFileDataUploadRequest{}
var nextPartNumber string = "0"
this.NextPartNumber = nextPartNumber
var maxParts string = "0"
this.MaxParts = maxParts
return &this
}
// GetEtags returns the Etags field value if set, zero value otherwise.
func (o *FinishFileDataUploadRequest) GetEtags() []string {
if o == nil || IsNil(o.Etags) {
var ret []string
return ret
}
return o.Etags
}
// GetEtagsOk returns a tuple with the Etags field value if set, nil otherwise
// and a boolean to check if the value has been set.
func (o *FinishFileDataUploadRequest) GetEtagsOk() ([]string, bool) {
if o == nil || IsNil(o.Etags) {
return nil, false
}
return o.Etags, true
}
// HasEtags returns a boolean if a field has been set.
func (o *FinishFileDataUploadRequest) HasEtags() bool {
if o != nil && !IsNil(o.Etags) {
return true
}
return false
}
// SetEtags gets a reference to the given []string and assigns it to the Etags field.
func (o *FinishFileDataUploadRequest) SetEtags(v []string) {
o.Etags = v
}
// GetNextPartNumber returns the NextPartNumber field value
// Deprecated
func (o *FinishFileDataUploadRequest) GetNextPartNumber() string {
if o == nil {
var ret string
return ret
}
return o.NextPartNumber
}
// GetNextPartNumberOk returns a tuple with the NextPartNumber field value
// and a boolean to check if the value has been set.
// Deprecated
func (o *FinishFileDataUploadRequest) GetNextPartNumberOk() (*string, bool) {
if o == nil {
return nil, false
}
return &o.NextPartNumber, true
}
// SetNextPartNumber sets field value
// Deprecated
func (o *FinishFileDataUploadRequest) SetNextPartNumber(v string) {
o.NextPartNumber = v
}
// GetMaxParts returns the MaxParts field value
// Deprecated
func (o *FinishFileDataUploadRequest) GetMaxParts() string {
if o == nil {
var ret string
return ret
}
return o.MaxParts
}
// GetMaxPartsOk returns a tuple with the MaxParts field value
// and a boolean to check if the value has been set.
// Deprecated
func (o *FinishFileDataUploadRequest) GetMaxPartsOk() (*string, bool) {
if o == nil {
return nil, false
}
return &o.MaxParts, true
}
// SetMaxParts sets field value
// Deprecated
func (o *FinishFileDataUploadRequest) SetMaxParts(v string) {
o.MaxParts = v
}
func (o FinishFileDataUploadRequest) MarshalJSON() ([]byte, error) {
toSerialize,err := o.ToMap()
if err != nil {
return []byte{}, err
}
return json.Marshal(toSerialize)
}
func (o FinishFileDataUploadRequest) ToMap() (map[string]interface{}, error) {
toSerialize := map[string]interface{}{}
if !IsNil(o.Etags) {
toSerialize["etags"] = o.Etags
}
toSerialize["nextPartNumber"] = o.NextPartNumber
toSerialize["maxParts"] = o.MaxParts
return toSerialize, nil
}
type NullableFinishFileDataUploadRequest struct {
value *FinishFileDataUploadRequest
isSet bool
}
func (v NullableFinishFileDataUploadRequest) Get() *FinishFileDataUploadRequest {
return v.value
}
func (v *NullableFinishFileDataUploadRequest) Set(val *FinishFileDataUploadRequest) {
v.value = val
v.isSet = true
}
func (v NullableFinishFileDataUploadRequest) IsSet() bool {
return v.isSet
}
func (v *NullableFinishFileDataUploadRequest) Unset() {
v.value = nil
v.isSet = false
}
func NewNullableFinishFileDataUploadRequest(val *FinishFileDataUploadRequest) *NullableFinishFileDataUploadRequest {
return &NullableFinishFileDataUploadRequest{value: val, isSet: true}
}
func (v NullableFinishFileDataUploadRequest) MarshalJSON() ([]byte, error) {
return json.Marshal(v.value)
}
func (v *NullableFinishFileDataUploadRequest) UnmarshalJSON(src []byte) error {
v.isSet = true
return json.Unmarshal(src, &v.value)
}