-
Notifications
You must be signed in to change notification settings - Fork 33
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
assignment 2 #31
base: master
Are you sure you want to change the base?
assignment 2 #31
Changes from 1 commit
File filter
Filter by extension
Conversations
Jump to
Diff view
Diff view
There are no files selected for viewing
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -62,10 +62,35 @@ | |
|
||
===================== */ | ||
|
||
var jsonToCsv = function(json) { console.log(json); }; | ||
|
||
|
||
var addMarkers = function(map) {}; | ||
var header = [Object.keys(healthCenters[0])]; | ||
var TempArr = [Object.values(healthCenters)]; | ||
healthCentersArr = header.push(TempArr); | ||
for (i=0; i < healthCentersArr.length; i++){console.log(healthCentersArr)}; | ||
|
||
var addIcon1 = L.icon({ | ||
iconUrl: 'https://upload.wikimedia.org/wikipedia/commons/3/3d/Blue_dot.png', | ||
iconSize: [38, 95], | ||
iconAnchor: [22, 94], | ||
popupAnchor: [-3, -76], | ||
}); | ||
var addIcon2 = L.icon({ | ||
iconUrl: 'https://www.pngarts.com/files/4/Dot-PNG-Pic.png', | ||
iconSize: [38, 95], | ||
iconAnchor: [22, 94], | ||
popupAnchor: [-3, -76], | ||
}); | ||
|
||
var addMarkers1 = (map) => {return L.marker([obj[1],obj[0]]).bindPopuup(obj[3],{icon: addIcon1})}; | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. I think you very nearly have it! It is very likely one line that needs to be fixed. But just try to run it again and see if you can find it. There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. Hi Ross, |
||
var addMarkers2 = (map) => {return L.marker([obj[1],obj[0]]).bindPopuup(obj[3],{icon: addIcon2})}; | ||
for (var i = 0; i < healthCentersArr.length; i++) { | ||
if(19140 <= healthCentersArr[i][5] <= 19149){ | ||
if (healthCentersArr[8] === "N/A"){ | ||
addMarkers1(healthCentersArr).addTo(map).bindPopup(healthCentersArr[i][3]); | ||
} else { | ||
addMarkers2(healthCentersArr).addTo(map).bindPopup(healthCentersArr[i][3]); | ||
} | ||
} | ||
}; | ||
|
||
/* ===================== | ||
|
||
|
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,98 @@ | ||
<!DOCTYPE html> | ||
<html> | ||
<head> | ||
<!--stylesheet imports--> | ||
<link rel="stylesheet" href="leaflet.css" /> | ||
</head> | ||
<body> | ||
|
||
<!--left panel--> | ||
<div style="position: absolute; left: 0px; width: 400px; top: 0; bottom: 0;"></div> | ||
<!--map--> | ||
<div id="map" style="position: absolute; right: 0; left: 400px; top: 0; bottom: 0;"></div> | ||
|
||
<!--javascript imports--> | ||
<script src="leaflet.js"></script> | ||
|
||
<script> | ||
|
||
/* ===================== | ||
|
||
# Lab 1, Part 1 — Array Access | ||
|
||
## Introduction | ||
|
||
Refactor your week 1 homework assignment to leverage some of the Javascript | ||
functionality we have been learning about. We will start by improving the | ||
way we store data and the amount of times we call the L.marker method. | ||
|
||
## Task 1: Data Storage | ||
|
||
Let's consider a marker for a single restaurant point location. We could | ||
store information about that marker as an array | ||
([element1, element2, element3...]). Your point location array should | ||
contain the following three elements in this order: 1. lat, 2. lng, 3. label. | ||
|
||
Create an array of arrays (an array that contains multiple arrays) to | ||
represent every restaurant in your restaurant data. Save this as variable | ||
"restaurantData". | ||
|
||
## Task 2: Process Data | ||
|
||
Create a marker for each element in "restaurantData". Your final code should | ||
only include "L.marker" one time. | ||
|
||
## Task 3: Add Two Additional Markers | ||
|
||
Add two additional restaurants to your data. You should only need to modify | ||
"restaurantData" to do this. Confirm that these new markers are added to | ||
your application. | ||
|
||
## Task 4: Add Popups to Markers | ||
|
||
Add popups to your markers. The popup should contain the label for your | ||
restaurant. Refer to the Leaflet documentation for instructions on adding | ||
a popup: http://leafletjs.com/reference.html#popup | ||
|
||
===================== */ | ||
|
||
var map = L.map('map', { | ||
center: [39.9522, -75.1639], | ||
zoom: 14 | ||
}); | ||
|
||
var Stamen_TonerLite = L.tileLayer('http://stamen-tiles-{s}.a.ssl.fastly.net/toner-lite/{z}/{x}/{y}.{ext}', { | ||
attribution: 'Map tiles by <a href="http://stamen.com">Stamen Design</a>, <a href="http://creativecommons.org/licenses/by/3.0">CC BY 3.0</a> — Map data © <a href="http://www.openstreetmap.org/copyright">OpenStreetMap</a>', | ||
subdomains: 'abcd', | ||
minZoom: 0, | ||
maxZoom: 20, | ||
ext: 'png' | ||
}).addTo(map); | ||
|
||
/* ===================== | ||
|
||
Start code | ||
|
||
===================== */ | ||
|
||
L.marker([0, 0]).addTo(map); | ||
L.marker([0, 0]).addTo(map); | ||
L.marker([0, 0]).addTo(map); | ||
|
||
var header = ['lat','lng','label']; | ||
var TempArr = [[39.952238, -75.201702,'Top1'],[39.952927, -75.217711,'Top2'],[39.944143, -75.174881,'Top3']]; | ||
TempArr.unshift(header); | ||
for (i=0; i < TempArr.length; i++){console.log(TempArr[i])}; | ||
var makeAMarker = (obj) => {return L.marker([obj[0], obj[1]]).bindPopup(obj[2])} | ||
for (var i=1; i <= TempArr.length; i++){ makeAMarker(TempArr[i]).addTo(map) } | ||
|
||
|
||
/* ===================== | ||
|
||
End code | ||
|
||
===================== */ | ||
|
||
</script> | ||
</body> | ||
</html> |
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,98 @@ | ||
/* ===================== | ||
# Lab 1, Part 2 — Function Review | ||
===================== */ | ||
|
||
/* ===================== | ||
Instructions: "Write a function that adds one to the number provided" | ||
Example: "plusOne(2) should return 3" | ||
===================== */ | ||
|
||
var plusOne = function(num) { | ||
return num + 1; | ||
}; | ||
|
||
console.log('plusOne success:', plusOne(99) === 100); | ||
|
||
/* ===================== | ||
Instructions: "Write a function that adds two to the number provided" | ||
Example: "plusTwo(2) should return 3" | ||
NOTE: Try using the `plusOne` function in the body of your `plusTwo` function | ||
===================== */ | ||
|
||
var plusTwo = function(num) { | ||
return num + 2; | ||
}; | ||
|
||
console.log('plusTwo success:', plusTwo(99) === 101); | ||
|
||
|
||
/* ===================== | ||
Instructions: "Write a function that checks to see if a number is an integer, | ||
if so, it returns even or odd depending on the number, otherwise it returns "error" | ||
|
||
===================== */ | ||
|
||
var oddOrEven = function(num) { | ||
|
||
}; | ||
|
||
console.log('oddOrEven success:', oddOrEven(100) === 'even' && oddOrEven(201) === 'odd'); | ||
|
||
|
||
|
||
/* ===================== | ||
Instructions: "Write a function, age, that takes a birth year and returns an age in years." | ||
(Let's just assume this person was born January 1 at 12:01 AM) | ||
Example: "age(2000) should return 17" | ||
===================== */ | ||
|
||
var age = function(num) { | ||
return 2019 - num; | ||
}; | ||
|
||
console.log('age success:', age(1971) === 48); | ||
|
||
/* ===================== | ||
Instructions: "Write a function that returns true for numbers over 9000 and false otherwise" | ||
Example: "over9000(22) should return false" | ||
===================== */ | ||
|
||
var over9000 = function(num) { | ||
if (num>9000){ | ||
return 'true'; | ||
} else { | ||
return 'false'; | ||
} | ||
}; | ||
|
||
console.log('over9000 success:', over9000(9001) === true && over9000(12) === false); | ||
|
||
|
||
/* ===================== | ||
Instructions: "Write a function, trump, that checks to see if the input is a string | ||
and if it is, returns the text in all upper case | ||
and if it is not, it prints to the console, "TRY WITH STRINGS" | ||
===================== */ | ||
|
||
|
||
var trump = function(text) { | ||
if(typeof text === string){ | ||
text.toUpperCase(); | ||
} else { | ||
console.log('TRY WITH STRINGS'); | ||
} | ||
}; | ||
|
||
console.log('trump success:', trump(12) === "TRY WITH STRINGS" && trump('hi') === 'HI'); | ||
|
||
|
||
|
||
/* ===================== | ||
Instructions: "Write a function which returns the y coordinate of a line given m, x, and b" | ||
Example: "y(0, 0, 0) should return 0; y(1, 1, 1) should return 2" | ||
===================== */ | ||
|
||
|
||
var y = function(m,x,b) {}; | ||
|
||
console.log('y success:', y(12, 1, 12) === 24); |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
You are extremely close. But haven't quite gotten it.
header
is an array that contains two sub arrays. The first is correct, but the secondTempArr
is an array with a collection of objects.Do you see how these results looks different in the console:
versus
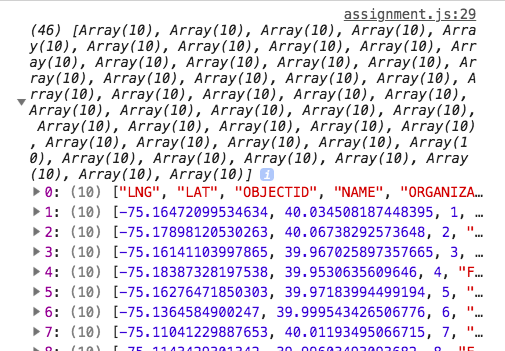