-
Notifications
You must be signed in to change notification settings - Fork 3
Action Dialog
####Action Dialog This dialog was inspired by the iOS UIAlertController class. And its main usage is to present a ListView with custom actions inside a dialog. I made this dialog because I simply needed a dialog that can hold more than 3 buttons, So now I can have as many as I want. Note that even if the list view exceeds the screen's limit, there is the built in ScrollView that comes with the ListView. Another important thing to note is that I made this as dynamic as possible, so other developers can also create their own custom action items.
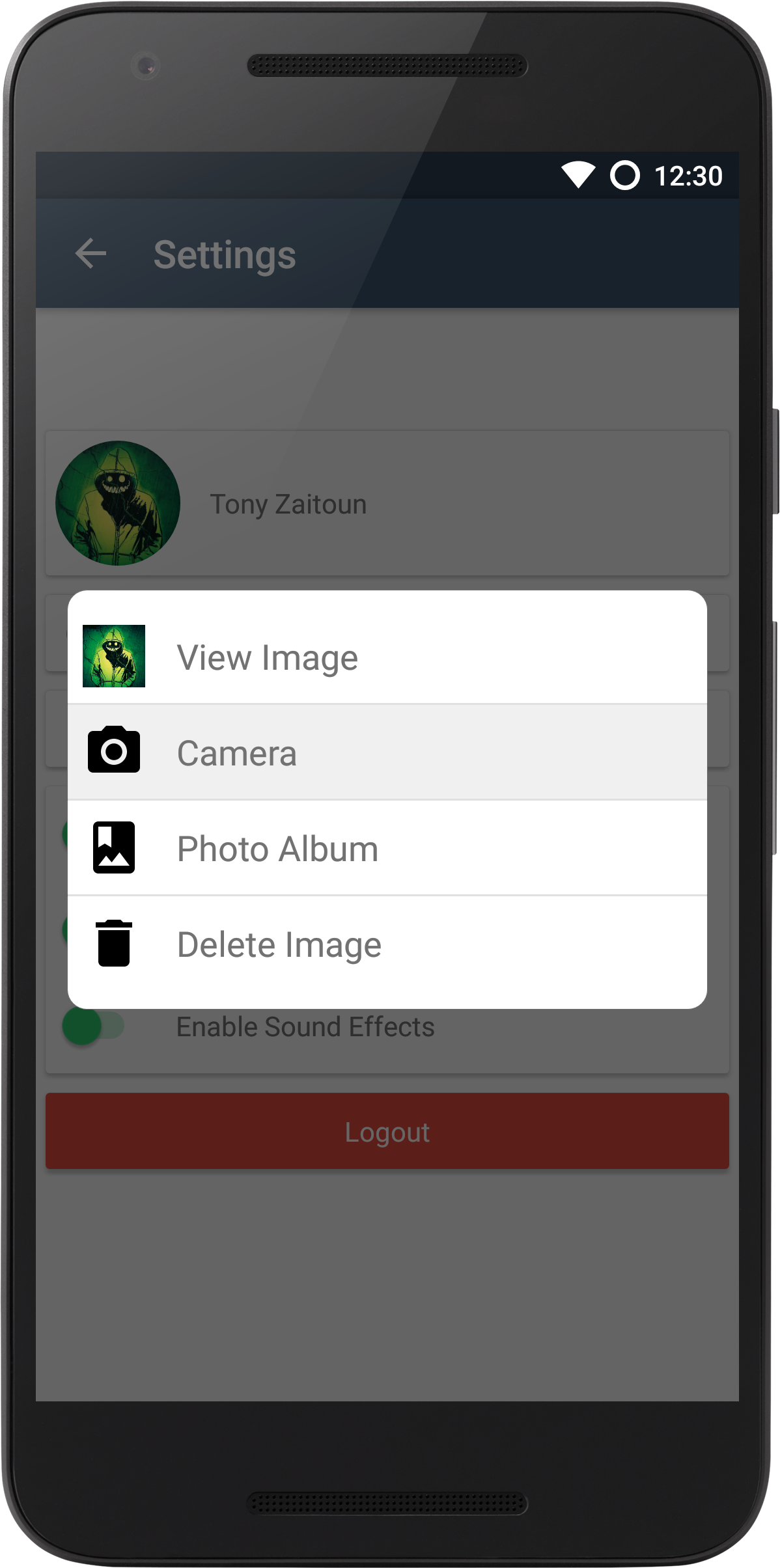
####AlertDialog.java class:
/**
* Created by Tony Zaitoun on 5/14/2016.
*/
public class ActionDialog extends BaseAlertDialog
Class Members:
private Context context;
private TextView title;
private TextView description;
private ListView listView;
private ArrayList<ActionItem> items;
/**The View of the dialog**/
private View dialogView;
/**The Root AlertDialog**/
private AlertDialog dialog;
private boolean allowAnimation = true;
private boolean cancelable = true;
private boolean isLocked = false;
private boolean showActionIcons = true;
Class public constructors:
/**
* Default ActionDialog constructor.
* @param context - Application Context.
* @param items - The action items.
*/
public ActionDialog(Context context, final ArrayList<ActionItem> items){...}
class public methods:
/**
* Show the dialog.
*/
@Override
public void show(){...}
/**
* Dismiss the dialog.
*/
@Override
public void dismiss(){...}
/**
* Sets the text on the dialog's title TextView.
*
* Note that if you do not set a title the title TextView's visibility will be set to to gone.
*
* @param title is the title you wish to set.
*/
public void setDialogTitle(String title){...}
/**
* Sets the text on the dialog's description TextView.
*
* Note that if you do not set a description the description TextView's visibility will be set to to gone.
*
* @param description is the description you wish to set.
*/
public void setDialogDescription(String description){...}
/**
* set the title visibility.
*
* @param show if true visibility will be VISIBLE, if false it will be set to GONE.
*/
public void setTitleVisable(boolean show){...}
/**
* set the description visibility.
*
* @param show if true visibility will be VISIBLE, if false it will be set to GONE.
*/
public void setDescriptionVisable(boolean show){...}
/**
*
* Getters and Setters.
*
*/
public Context getContext(){...}
public void setContext(Context context){...}
public TextView getTitle(){...}
public ListView getListView(){...}
public ArrayList<ActionItem> getItems(){...}
public View getDialogView(){...}
public void setDialogView(View dialogView){...}
public AlertDialog getDialog(){...}
public void setDialog(AlertDialog dialog){...}
public boolean isAllowAnimation(){...}
public void setAllowAnimation(boolean allowAnimation){...}
public boolean isCancelable(){...}
public void setCancelable(boolean cancelable){...}
public boolean isShowActionIcons(){...}
public void setShowActionIcons(boolean showActionIcons){...}
public TextView getDescription(){...}
Usage:
import net.crofis.ui.custom.actionitem.ActionItem;
import net.crofis.ui.custom.actionitem.ActionItemClickListener;
import net.crofis.ui.dialog.ActionDialog;
//First we have to create the list that will contain the action items
List <ActionItem> items = new ArrayList<>();
//Fill the list with our desired actions
Drawable icon = getResources().getDrawable(R.drawable.common_ic_googleplayservices);
for (int i = 0; i < 4; i++) {
final int finalI = i;
//Create the action item with constructor ActionItem(Drawable,String, ActionItemClickListner);
ActionItem item = new ActionItem(icon, "item " + (i + 1), new ActionItemClickListener() {
@Override
public void onActionSelected() {
//This is what will be triggered when the action is selected.
Toast.makeText(MainActivity.this,"item "+(finalI +1)+" selected.",Toast.LENGTH_SHORT).show();
}
});
items.add(item);
}
//Create the ActionDialog object with constuctor ActionDialog(Context,List<ActionItem>)
final ActionDialog dialog = new ActionDialog(this, items);
//Optionl customization - set the gravity of the dialog (TOP or BOTTOM)
//Must import net.crofis.ui.dialog.DialogManager;
DialogManager.setDialogPosition(dialog,dialog.getDialog(), Gravity.BOTTOM);
//Set the text - if not set, the TextViews will disappear.
dialog.setDialogTitle("Select Action");
dialog.setDialogDescription("Select an action to close the dialog.");
//Finall, show the dialog
dialog.show();
Create your own ActionItem class:
Here is an example of a class that extends ActionItem:
public class UIAlertAction extends ActionItem {
@Override
public String getTitle() {
return super.getTitle();
}
/**
* Default constructor.
*
* @param icon - Action Icon
* @param title - Action Title
* @param listener - Action Listener
*/
public UIAlertAction(Drawable icon, String title, ActionItemClickListener listener) {
super(icon, title, listener);
}
/**
* getView() is a method that is used only by sub-classes. It will always return null by the super class.
* Example on how to override:
*
* The following code snippet, will use the default item but it will be designed like iOS.
*/
@Override
public View getView(Context context, boolean showActionIcons) {
View convertView = LayoutInflater.from(context).inflate(R.layout.ui_general_action, null, false);
TextView title = (TextView) convertView.findViewById(R.id.action_title);
title.setText(getTitle());
title.setGravity(Gravity.CENTER);
title.setTextColor(context.getResources().getColor(R.color.blue));
if (false)((ImageView) convertView.findViewById(R.id.action_icon)).setImageDrawable(getIcon());
else convertView.findViewById(R.id.action_icon).setVisibility(View.GONE);
return convertView;
}
}
ui_general_action.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal" android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center_vertical">
<ImageView
android:layout_margin="8dp"
android:layout_width="32dp"
android:layout_height="32dp"
android:id="@+id/action_icon" />
<TextView
android:layout_weight="1"
android:layout_margin="8dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textAppearance="?android:attr/textAppearanceMedium"
android:text="Medium Text"
android:id="@+id/action_title" />
</LinearLayout>
And then simply do:
ArrayList<ActionItem> items = new ArrayList<>();
for (int i = 0; i < 4; i++) {
final int finalI = i;
ActionItem item = new UIAlertAction(null, "item " + (i + 1), new ActionItemClickListener() {
@Override
public void onActionSelected() {
Toast.makeText(this,"item "+(finalI +1)+" selected.",Toast.LENGTH_SHORT).show();
}
});
items.add(item);
}
final ActionDialog dialog = new ActionDialog(this, items);
dialog.getTitle().setGravity(Gravity.CENTER);
dialog.getDescription().setGravity(Gravity.CENTER);
dialog.setDialogTitle("Select Action");
dialog.setDialogDescription("Select an action to close the dialog.");
dialog.show();
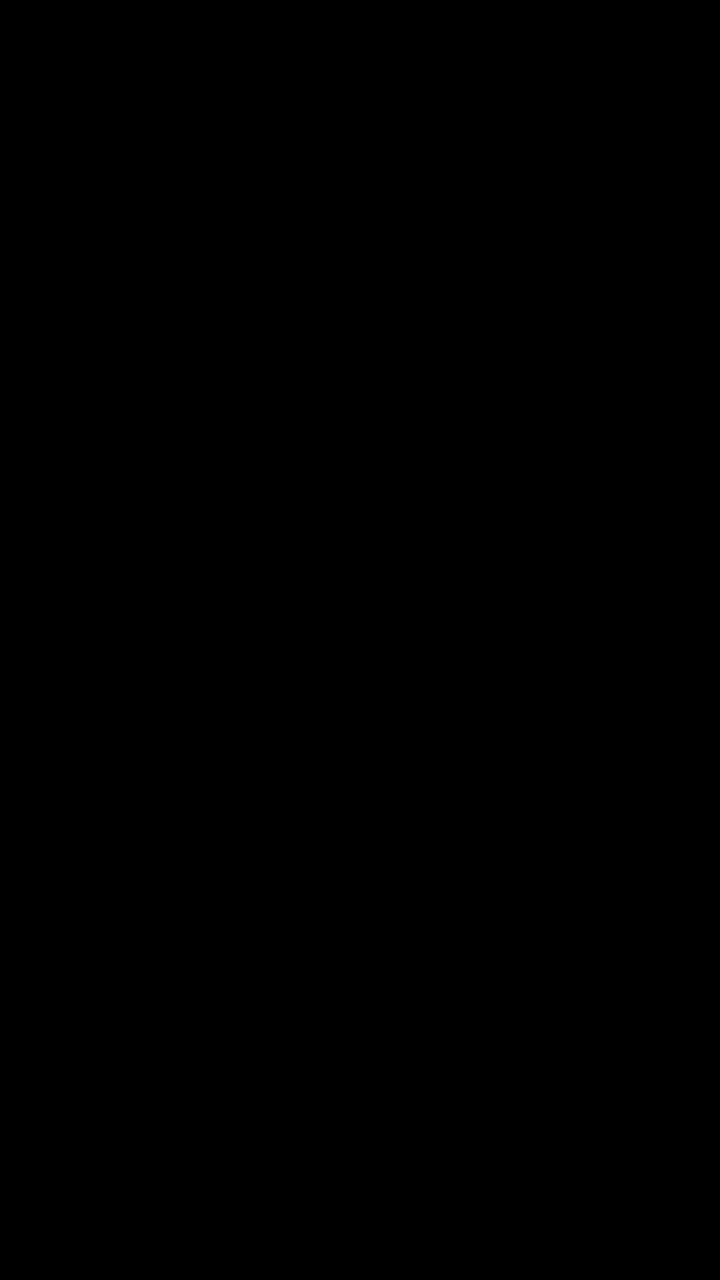