generated from jobindjohn/obsidian-publish-mkdocs
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
fixed some things, time for a revamp
- Loading branch information
1 parent
3ea6b9d
commit 58f6616
Showing
5 changed files
with
103 additions
and
4 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,4 +1,5 @@ | ||
.vscode/settings.json | ||
.obsidian/* | ||
.obsidian/ | ||
|
||
.vscode/settings.json | ||
.obsidian/* | ||
.obsidian/ | ||
template/ | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,43 @@ | ||
# 11. Container With Most Water | ||
|
||
Tags: #array #twopointers #greedy #medium | ||
|
||
### Problem Statement | ||
You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`. | ||
|
||
Find two lines that together with the x-axis form a container, such that the container contains the most water. | ||
|
||
Return _the maximum amount of water a container can store_. | ||
|
||
**Notice** that you may not slant the container. | ||
|
||
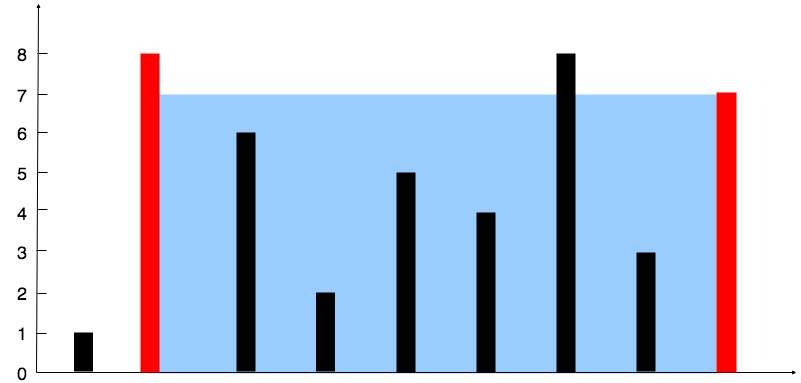 | ||
|
||
|
||
```Example | ||
Input: height = [1,8,6,2,5,4,8,3,7] | ||
Output: 49 | ||
Explanation: The above vertical lines are represented by array [1,8,6,2,5,4,8,3,7]. In this case, the max area of water (blue section) the container can contain is 49. | ||
``` | ||
|
||
### My Approach | ||
```Python | ||
class Solution: | ||
def maxArea(self, height: List[int]) -> int: | ||
i = 0 | ||
j = len(height)-1 | ||
temp = 0 | ||
primary = 0 | ||
while(i<j): | ||
temp = min(height[i], height[j])*(j-i) | ||
if temp > primary: | ||
primary = temp | ||
if height[j]>height[i]: | ||
i+=1 | ||
else: | ||
j-=1 | ||
return primary | ||
``` | ||
|
||
|
||
### Personal Thoughts |
Empty file.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,43 @@ | ||
# 1679. Max Number of K-Sum Pairs | ||
|
||
Tags: #array #twopointers #sorting #hashtable #medium | ||
|
||
### Problem Statement | ||
You are given an integer array `nums` and an integer `k`. | ||
|
||
In one operation, you can pick two numbers from the array whose sum equals `k` and remove them from the array. | ||
|
||
Return _the maximum number of operations you can perform on the array_. | ||
|
||
```Example | ||
Input: nums = [1,2,3,4], k = 5 | ||
Output: 2 | ||
Explanation: Starting with nums = [1,2,3,4]: | ||
- Remove numbers 1 and 4, then nums = [2,3] | ||
- Remove numbers 2 and 3, then nums = [] | ||
There are no more pairs that sum up to 5, hence a total of 2 operations. | ||
``` | ||
|
||
### My Approach | ||
```Python | ||
class Solution: | ||
def maxOperations(self, nums: List[int], k: int) -> int: | ||
i = 0 | ||
j = len(nums)-1 | ||
count = 0 | ||
nums.sort() | ||
|
||
while(i<j): | ||
sum =nums[i]+nums[j] | ||
if sum == k: | ||
count +=1 | ||
i+=1 | ||
j-=1 | ||
elif sum < k: | ||
i+=1 | ||
else: | ||
j-=1 | ||
return count | ||
``` | ||
|
||
### Personal Thoughts |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters