-
Notifications
You must be signed in to change notification settings - Fork 88
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Sea Turtles - Shannon Bellemore #88
base: main
Are you sure you want to change the base?
Changes from all commits
File filter
Filter by extension
Conversations
Jump to
Diff view
Diff view
There are no files selected for viewing
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,146 @@ | ||
"use strict"; | ||
|
||
|
||
const state = { | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. 👍🏽 |
||
tempDegrees: 50, | ||
city: 'Anchorage', | ||
sky: 'snowy', | ||
}; | ||
|
||
// increase temperature | ||
const increaseTemp = () => { | ||
state.tempDegrees += 1; | ||
const tempContainer = document.getElementById('temp-number'); | ||
tempContainer.textContent = state.tempDegrees; | ||
changesByTemp(); | ||
}; | ||
|
||
// decrease temperature | ||
const decreaseTemp = () => { | ||
state.tempDegrees -= 1; | ||
const tempContainer = document.getElementById('temp-number'); | ||
tempContainer.textContent = state.tempDegrees; | ||
changesByTemp(); | ||
}; | ||
|
||
// change temperature display color & ground weather garden by temperature number | ||
const changesByTemp = () => { | ||
const tempContainer = document.getElementById('temp-number'); | ||
const gardenContainer = document.getElementById('ground-garden') | ||
tempContainer.textContent = state.tempDegrees; | ||
if(state.tempDegrees >= 80) { | ||
tempContainer.style.color = '#d04123'; | ||
gardenContainer.textContent = '🌵__🐍_🦂_🌵🌵__🐍_🏜_🦂' | ||
} else if(state.tempDegrees >= 70 && state.tempDegrees <= 79) { | ||
tempContainer.style.color = '#f29f4e'; | ||
gardenContainer.textContent = '🌸🌿🌼__🌷🌻🌿_☘️🌱_🌻🌷' | ||
} else if(state.tempDegrees >= 60 && state.tempDegrees <= 69) { | ||
tempContainer.style.color = '#f1d42d'; | ||
gardenContainer.textContent = '🌾🌾_🍃_🪨__🛤_🌾🌾🌾_🍃' | ||
} else if(state.tempDegrees >= 50 && state.tempDegrees <= 59) { | ||
tempContainer.style.color = '#7caf4e'; | ||
gardenContainer.textContent = '🌲🌲⛄️🌲⛄️🍂🌲🍁🌲🌲⛄️🍂🌲' | ||
} else if(state.tempDegrees <= 49) { | ||
tempContainer.style.color = '#73a3bb'; | ||
gardenContainer.textContent = '🌲🌲⛄️🌲⛄️🍂🌲🍁🌲🌲⛄️🍂🌲' | ||
} | ||
}; | ||
|
||
// changes the sky element | ||
const changeSky = () => { | ||
let currentSky = document.getElementById('skys').value; | ||
let skyContainer = document.getElementById('sky-garden'); | ||
state.sky = currentSky; | ||
if(state.sky === 'cloudy') { | ||
skyContainer.textContent = '☁️☁️ ☁️ ☁️☁️ ☁️ 🌤 ☁️ ☁️☁️' | ||
} else if(state.sky === 'sunny') { | ||
skyContainer.textContent = '☁️ ☁️ ☁️ ☀️ ☁️ ☁️' | ||
} else if(state.sky === 'rainy') { | ||
skyContainer.textContent = '🌧🌈⛈🌧🌧💧⛈🌧🌦🌧💧🌧🌧' | ||
} else if(state.sky === 'snowy') { | ||
skyContainer.textContent = '🌨❄️🌨🌨❄️❄️🌨❄️🌨❄️❄️🌨🌨' | ||
} | ||
}; | ||
|
||
// changes city name according to text input | ||
const changeCityName = () => { | ||
const cityInput = document.getElementById('city-input').value; | ||
const currentCityName = document.getElementById('city-name'); | ||
currentCityName.textContent = cityInput; | ||
state.city = cityInput; | ||
}; | ||
|
||
// reset city name to the default | ||
const resetCityName = () => { | ||
const cityContainer = document.getElementById('city-input'); | ||
const currentCityName = document.getElementById('city-name'); | ||
state.city = 'Anchorage'; | ||
cityContainer.value = state.city; | ||
currentCityName.textContent = state.city; | ||
}; | ||
|
||
// converts given degrees kelvin to fahrenheit | ||
const convertTemp = (degreesKelvin) => { | ||
let degreesFahrenheit = Math.round(1.8*(degreesKelvin -273) + 32); | ||
return degreesFahrenheit; | ||
}; | ||
|
||
// gets latitiude and longitude for current city inputted | ||
const getLatitudeAndLongitude = () => { | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. 👍🏽 |
||
axios | ||
.get('http://127.0.0.1:5000/location', { | ||
params: { | ||
q: state.city | ||
}, | ||
}) | ||
.then((response) => { | ||
let latitude = response.data[0].lat; | ||
let longitude = response.data[0].lon; | ||
getTemperature(latitude, longitude); | ||
}) | ||
.catch((error) => { | ||
console.log('error with getting lat & lon!', error.response.data); | ||
}); | ||
} | ||
|
||
// gets current real time temperature using latitude and longitude | ||
const getTemperature = (latitude, longitude) => { | ||
axios | ||
.get('http://127.0.0.1:5000/weather', { | ||
params: { | ||
lat: latitude, | ||
lon: longitude, | ||
}, | ||
}) | ||
.then((response) => { | ||
const degreesKelvin = response.data.current.temp; | ||
let currentTemp = convertTemp(degreesKelvin); | ||
state.tempDegrees = currentTemp; | ||
changesByTemp(); | ||
}) | ||
.catch((error) => { | ||
console.log('error with getting temp!', error.response.data); | ||
}); | ||
} | ||
|
||
const registerEventHandlers = () => { | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. 👍🏽 |
||
const increaseTempButton = document.querySelector('#increase-temp-button'); | ||
increaseTempButton.addEventListener('click', increaseTemp); | ||
|
||
const decreaseTempButton = document.querySelector('#decrease-temp-button'); | ||
decreaseTempButton.addEventListener('click', decreaseTemp); | ||
|
||
const cityInput = document.getElementById('city-input'); | ||
cityInput.addEventListener('input', changeCityName); | ||
|
||
const getRealTemp = document.getElementById('real-temp-button'); | ||
getRealTemp.addEventListener('click', getLatitudeAndLongitude); | ||
|
||
const changeCurrentSky = document.getElementById('skys'); | ||
changeCurrentSky.addEventListener('change', changeSky); | ||
|
||
const resetCity = document.getElementById('reset-city-button'); | ||
resetCity.addEventListener('click', resetCityName); | ||
}; | ||
|
||
document.addEventListener("DOMContentLoaded", registerEventHandlers); |
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,104 @@ | ||
header{ | ||
/* display: flex; | ||
justify-content: flex-start; */ | ||
Comment on lines
+2
to
+3
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. you can remove this |
||
display: grid; | ||
grid-template-rows: 1fr 1fr; | ||
} | ||
|
||
#city-name { | ||
color: #ae78f0; | ||
font-size: 150%; | ||
font-weight: bold; | ||
} | ||
|
||
body{ | ||
font-family: 'Courier New', Courier, monospace; | ||
color: #1e475b; | ||
background-color: #bad0f1; | ||
|
||
display: flex; | ||
justify-content: center; | ||
align-items: center; | ||
} | ||
|
||
.main-container{ | ||
display: grid; | ||
grid-template-columns: 100px 300px 20px 300px 100px; | ||
grid-template-rows: 50px 150px 20px 200px 50px; | ||
} | ||
|
||
.general-container{ | ||
color: #73a3bb; | ||
background-color: #ffffff; | ||
text-align: center; | ||
border: 2px cornflowerblue; | ||
border-style: dashed; | ||
border-radius: 10px; | ||
} | ||
|
||
.temperature-container{ | ||
width: 300px; | ||
height: 150px; | ||
grid-column-start: 2; | ||
grid-row-start: 2; | ||
} | ||
|
||
.city-container{ | ||
width: 300px; | ||
height: 150px; | ||
grid-row-start: 2; | ||
grid-column-start: 4; | ||
} | ||
|
||
.landscape-container{ | ||
width: 620px; | ||
height: 200px; | ||
grid-row-start: 4; | ||
grid-column-start: 2; | ||
} | ||
|
||
#increase-temp-button{ | ||
padding: 0; | ||
border: 0; | ||
margin: 0; | ||
font-size: 125%; | ||
} | ||
|
||
#increase-temp-button:hover{ | ||
cursor: pointer; | ||
} | ||
|
||
#decrease-temp-button{ | ||
padding: 0; | ||
border: 0; | ||
margin: 0; | ||
font-size: 125%; | ||
} | ||
|
||
#real-temp-button{ | ||
color: whitesmoke; | ||
background-color: #1e475b; | ||
} | ||
|
||
#real-temp-button:hover{ | ||
cursor: pointer; | ||
} | ||
|
||
#decrease-temp-button:hover{ | ||
cursor: pointer; | ||
} | ||
|
||
#temp-number{ | ||
font-size: 200%; | ||
font-weight: bold; | ||
} | ||
|
||
#reset-city-button{ | ||
color: whitesmoke; | ||
background-color: #1e475b; | ||
} | ||
|
||
#reset-city-button:hover{ | ||
cursor: pointer; | ||
} | ||
|
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Your html page is clean and well organized! I see that you are using span and div tags for a few things. I want to share a resource that walks through when to use particular tags:
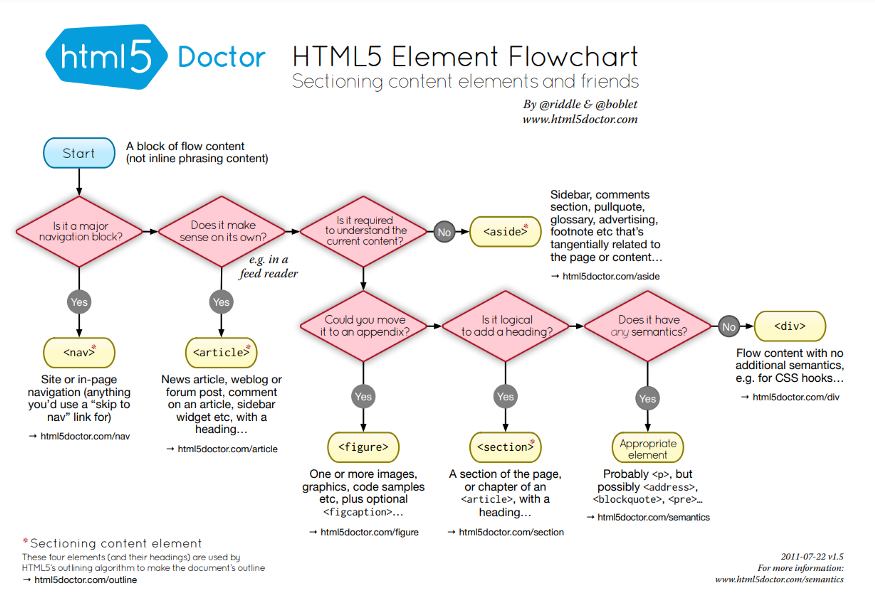