forked from dartmouth-cs52/slackattack
-
Notifications
You must be signed in to change notification settings - Fork 1
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
1 changed file
with
78 additions
and
31 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,74 +1,111 @@ | ||
# Make a Slack Bot! | ||
|
||
In this assignment we'll be building a Slack bot. The bot will be able to do things like respond to messages and message users as they join your Slack team. It will be a simple [Node.js](https://nodejs.org/en/) and [Express.js](http://expressjs.com/) app and run on [Heroku](https://www.heroku.com/). Don't worry if you haven't used these technologies before– all will be explained. | ||
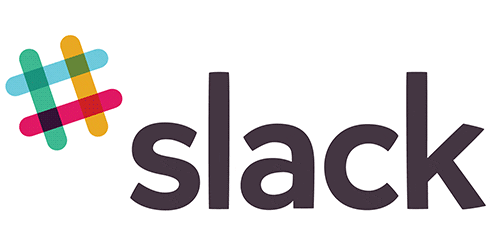 | ||
|
||
Are you ready to invent the next AI? Any self-respecting bot needs to be able to communicate via Slack. The bot will be able to do things like respond to messages and message users as they join your Slack team. It will be a simple [Node.js](https://nodejs.org/en/) and [Express.js](http://expressjs.com/) app and run on [Heroku](https://www.heroku.com/). Don't worry if you haven't used these technologies before — all will be explained! | ||
|
||
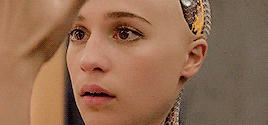 | ||
|
||
As a general introduction to this guide, we'll be using the command line interface (CLI) a lot. Things that require use of the CLI will be formatted as `code`. Furthermore, the bear 🐻 you see here calls attention to action items. Do these things! | ||
|
||
## Slack Bot Basics | ||
Bot users are similar to human users in that they have names, profile pictures, exist as part of the team, can post messages, invited to channels, and more. The main difference is that bots are controlled programmatically via a a user token that accesses one of Slack's APIs. We'll be building a custom bot that listens to certain events, like a message or a new member joining your team, and responds accordingly. | ||
In Slack, bot users are similar to human users in that they have names, profile pictures, exist as part of the team, can post messages, invited to channels, and more. The main difference is that bots are controlled programmatically via an API that Slack provides. We'll be building a custom bot that listens to certain events, like a message or a new member joining your team, and responds accordingly. | ||
|
||
## APIs | ||
|
||
🚩Application Program Interfaces (APIs) are sets of methods and protocols by which programs talk to each other. | ||
|
||
We'll be talking a lot more about this when we start designing our own! But for now we'll use Slack's API to communicate with Slack's servers. | ||
|
||
## Setup | ||
Some of the technologies we'll be using are Slack, Github, Heroku, and Node.js. You're probably already familiar with Slack and Github, but Heroku and Node might be new. Node is runtime environment used for developing server-side web applications and Heroku is a [Platform-as-a-Service](https://en.wikipedia.org/wiki/Platform_as_a_service) which runs our Node application. Let's walk through these basic setup steps together. | ||
Some of the technologies we'll be using are Slack, Github, Heroku, and Node.js. You're already familiar with Slack and Github, but Heroku and Node might be new. Node is runtime environment used for developing server-side web applications and Heroku is a [Platform-as-a-Service](https://en.wikipedia.org/wiki/Platform_as_a_service) which runs our Node application. Let's walk through these basic setup steps together. | ||
|
||
1. **GitHub** | ||
|
||
🐻Fork this repository! | ||
|
||
1. **Slack** | ||
|
||
🐻From the Slack desktop app, click on the team name in the top-left and then go to "Apps & Integrations." Search for "bot" and click the top result, "Bots." Click "Add Configuration" and fill in the details for the bot. Take note of the API Token, we'll use it later. | ||
🐻From the Slack desktop app, click on the team name in the top-left and then go to "Apps & Integrations." Search for "bot" and click the top result, "Bots." Click "Add Configuration". Choose a name for your bot and fill in the details for the bot. Take note of the API Token, we'll use it later. | ||
|
||
1. **Github** | ||
 | ||
|
||
🐻Make a repo on [Github](http://github.com/). | ||
1. **Setup Local Environment** | ||
|
||
1. **Heroku** | ||
🚩Your app will need to know about the API token. This token allows you to talk to Slack's servers in an authenticated way. API keys can be thought of as authentication for programs. | ||
|
||
🐻Head over to [Heroku](https://www.heroku.com/) and login/sign up. Then, make a new app. In the "Deploy" tab, set the deployment method to **Github** and connect to the repo you made. Head over to "Settings" and add a Config Variable `SLACK_BOT_TOKEN` with value set to the API token of the Slack bot you made in step 1. | ||
You'll need to have the API token as a local environment variable. This way you can run and test your Node app locally and you don't have to put your secret API key into your code — which would be a security risk as then potentially it could be used by anybody if your code was public. | ||
|
||
You'll also want to have the API token as a local environment variable. This way you can run and test your Node app locally, which is often faster and more convenient than deploying on Heroku. You do not want to include the key directly in your code, which is under source control and sometimes public.. | ||
You can set an environment variable with `export <name>="XXXX"` and view it with `echo $<name>`. | ||
|
||
🐻Export an environment variable with `export <name>="XXXX"`. View it with `echo $<name>` | ||
💻 | ||
```bash | ||
export SLACK_BOT_TOKEN="TOKEN_YOU_SAVED_EARLIER" | ||
echo $SLACK_BOT_TOKEN | ||
``` | ||
|
||
Then, you'll be able to access this in your Javascript file with `process.env.<name>`. | ||
Note: on Windows try `set SLACK_BOT_TOKEN "TOKEN_YOU_SAVED_EARLIER"`. | ||
|
||
Heroku also requires a `Procfile` which tells Heroku what commands to run in its [dynos](https://devcenter.heroku.com/articles/dynos) | ||
In our app we will be able to access this in javascript with `process.env.SLACK_BOT_TOKEN`. | ||
|
||
🐻Our `Procfile` is just one line, `web: node web.js`, where `web` defines the dyno type (this one receives HTTP traffic), `node` is the command and `web.js` is the name of our main Javascript file. | ||
🚩 The above export only sets the variable for our currently running shell. To save on OS X or Linux something akin to: `echo 'export SLACK_BOT_TOKEN="TOKEN_YOU_SAVED_EARLIER"' >> ~/.profile` should do the trick, but this differs a lot by shell and operating system. | ||
|
||
1. **Node.js and Node Package Manager (npm)** | ||
|
||
First, let's install Node.js with Homebrew. | ||
🐻First, let's install [Node.js](https://nodejs.org/en/). | ||
🐻`brew install node` | ||
macOS: | ||
```bash | ||
brew install node | ||
``` | ||
Node's package manager, npm, is installed automatically with Node. It lets you install packages with | ||
Windows: | ||
download the installer from: [Node.js](https://nodejs.org/en/) | ||
🐻`npm install <package> --save` | ||
Node's package manager, npm, is installed automatically with Node. It lets you install packages with`npm install <package> --save` | ||
|
||
which both installs and **saves** the package as a dependency in the `package.json file`, explained below. | ||
|
||
🐻Setup your `package.json` file, which specifies settings for your Node app– it should be pretty similar to this | ||
🐻Initialize your Node app: | ||
|
||
```bash | ||
cd slackattack | ||
npm init | ||
``` | ||
|
||
npm will ask you bunch of questions to prepopulate your `package.json`. This is a file that tells node things about your app. How to start it, what packages it depends on, who the author is etc. | ||
|
||
🐻 Open your project in Atom `atom .` and massage your `package.json` file to look something like: | ||
|
||
```json | ||
{ | ||
"name": "WelcomeBot", | ||
"version": "0.0.0", | ||
"description": "Team Slack welcome bot", | ||
"main": "web.js", | ||
"name": "jackjack", | ||
"version": "1.0.0", | ||
"description": "", | ||
"main": "app.js", | ||
"scripts": { | ||
}, | ||
"repository": { | ||
"type": "git", | ||
"url": "[email protected]:welcome-bot.git " | ||
"test": "echo \"Error: no test specified\" && exit 1" | ||
}, | ||
"author": "Your Name", | ||
"license": "ISC", | ||
"dependencies": { | ||
} | ||
"license": "ISC" | ||
} | ||
``` | ||
The **url** is based on your Heroku app name and can be found under "Settings." Note that there are no dependencies yet– you can go ahead and try installing Express with `npm install`, described above. | ||
|
||
Note that there are no dependencies yet. Lets add one: | ||
|
||
```bash | ||
npm install --save express | ||
``` | ||
|
||
|
||
1. **Express** | ||
|
||
[Express](http://expressjs.com/) is a web framework for Node. Our main use for it is to let our Node app know which port it should listen to. | ||
|
||
🐻Make a new file, `web.js`, which will be the main file of our app. At the top, include things like your packages (e.g. `var express = require('express');`). Then, add this snippet of code which will let the Node app know which port to listen to. | ||
🐻Make a new file, `app.js`, which will be the main file of our app. This could really be named anything. Later we'll look at best practices for how to structure more complicated Express.js projects. | ||
At the top, include things like your packages (e.g. `var express = require('express');`). Then, add this snippet of code which will let the Node app know which port to listen to. | ||
``` | ||
var app = express(); | ||
|
@@ -125,3 +162,13 @@ At this point you have a bot which has some sort of basic response when it recei | |
|
||
## Make Your Bot Your Own | ||
At this point you've achieved a general understanding of what goes into making a Slack bot have implemented some basic but powerful functionalities. Now, go and see what else you can do with your Slack bot. Brainstorm, read documentation, and experiment. Make your bot the best that it can be! | ||
## Deploy On Heroku | ||
1. 🐻Head over to [Heroku](https://www.heroku.com/) and login/sign up. Then, make a new app. In the "Deploy" tab, set the deployment method to **Github** and connect to the repo you made. Head over to "Settings" and add a Config Variable `SLACK_BOT_TOKEN` with value set to the API token of the Slack bot you made in step 1. | ||
Heroku also requires a `Procfile` which tells Heroku what commands to run in its [dynos](https://devcenter.heroku.com/articles/dynos) | ||
🐻Our `Procfile` is just one line, `web: node web.js`, where `web` defines the dyno type (this one receives HTTP traffic), `node` is the command and `web.js` is the name of our main Javascript file. |