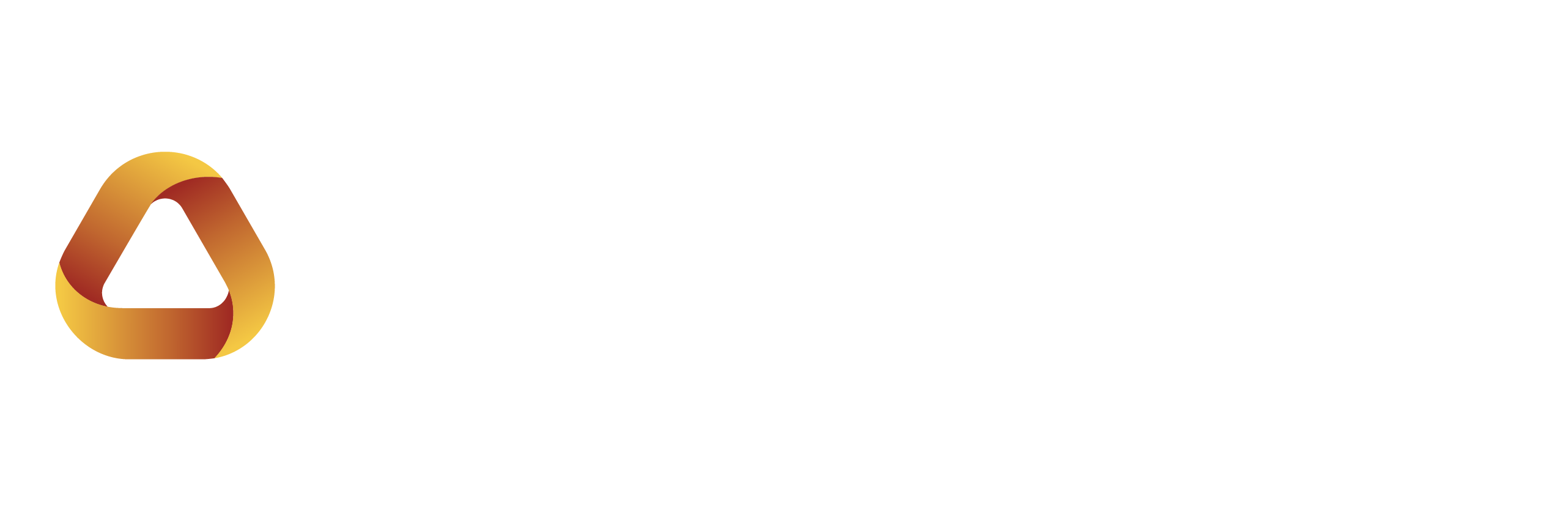
Automata DCAP Attestation consists of three parts:
-
PCCS Router: A central contract to read collaterals from
automata-on-chain-pccs
-
Automata DCAP Attestation: This is the entrypoint contract for users to submit a quote to be verified. This contract parses the Quote header to identify the version, which then forwards the quote to the respective QuoteVerifier contract.
-
Quote Verifier(s): This contract provides the full implementation on verifying a given quote specific to its version. This contract is intended to be called only from the Automata DCAP Attestation contract.
Automata DCAP Attestation contract implements two attestation methods available to users. Here is a quick comparison:
On-Chain | Groth16 Proof Verification with RiscZero | Groth16 Proof Verification with SP1 V3 | Plonk Proof Verification with SP1 V3 | |
---|---|---|---|---|
Quote Verification Time | Instant | Proving takes 2 - 5 minutes, instant verification | Proving takes <2 minutes, instant verification | Proving takes <2 minutes, instant verification |
Gas Cost | ~4-5M gas (varies by collateral size) | 351k gas | 325k gas | 410k gas |
Execution | Runs fully on-chain | Execution proven by remote prover Bonsai | Execution proven by the SP1 Network | Execution proven by the SP1 Network |
To integrate your contract with Automata DCAP Attestation, you need to first install Foundry.
Add to your dependency, by running:
forge install automata-network/automata-dcap-attestation
Then, add the following to your remappings.txt
@automata-network/dcap-attestation/=lib/automata-dcap-attestation/contracts/
import "@automata-network/dcap-attestation/AutomataDcapAttestationFee.sol";
contract ExampleDcapContract {
AutomataDcapAttestationFee attest;
constructor(address _attest) {
attest = AutomataDcapAttestationFee(_attest);
}
// On-Chain Attestation example
function attestOnChain(bytes calldata quote) public {
(bool success, bytes memory output) = attest.verifyAndAttestOnChain(quote);
if (success) {
// ... implementation to handle successful attestations
} else {
string memory errorMessage = string(output);
// ... implementation to handle failed attestations
}
}
// SNARK Attestation example
// ZkCoProcessorType can either be RiscZero or Succinct
function attestWithSnark(
bytes calldata output,
ZkCoProcessorType zkvm,
bytes calldata proofBytes
) public
{
(bool success, bytes memory output) = attest.verifyAndAttestWithZKProof(
output,
zkvm,
proofBytes
);
if (success) {
// ... implementation to handle successful attestations
} else {
string memory errorMessage = string(output);
// ... implementation to handle failed attestations
}
}
}
Clone this repo, by running the following command:
git clone [email protected]:automata-network/automata-dcap-attestation.git --recurse-submodules
Before you begin, make sure to create a copy of the .env
file with the example provided. Then, please provide any remaining variables that are missing.
cp .env.example .env
Compile the contracts:
forge build
Testing the contracts:
forge test
To view gas report, pass the --gas-report
flag.
To provide additional test cases, please include those in the /forge-test
directory.
To provide additional scripts, please include those in the /forge-script
directory.
Deploy the PCCS Router:
forge script DeployRouter --rpc-url $RPC_URL --broadcast -vvvv
Deploy Automata DCAP Attestation Entrypoint:
forge script AttestationScript --rpc-url $RPC_URL --broadcast -vvvv --sig "deployEntrypoint()"
Deploy Quote Verifier(s):
forge script DeployV3 --rpc-url $RPC_URL --broadcast -vvvv
The naming format for the script is simply DeployV{x}
, where x
is the quote version supported by the verifier. Currently, we only support V3 and V4 quotes.
Whitelist QuoteVerifier(s) in the Entrypoint contract:
forge script AttestationScript --rpc-url $RPC_URL --broadcast -vvvv --sig "configVerifier(address)" <verifier-address>
The ImageID currently used for the DCAP RiscZero Guest Program is 83613a8beec226d1f29714530f1df791fa16c2c4dfcf22c50ab7edac59ca637f
.
The VKEY currently used for the DCAP SP1 Program is
0043e4e0c286cf4a2c03472ca2384f35a008558bc5de4e0f39d1d1bc989badca
.
ℹ️ Note:
The deployment addresses shown here are currently based on the latest changes made.
To view deployments on the previous version (will be deprecated soon), you may refer to this branch.