-
Notifications
You must be signed in to change notification settings - Fork 1.2k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
4 changed files
with
165 additions
and
2 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,86 @@ | ||
# [1496. 判断路径是否相交](https://leetcode.cn/problems/path-crossing/) | ||
|
||
- 标签:哈希表、字符串 | ||
- 难度:简单 | ||
|
||
## 题目链接 | ||
|
||
- [1496. 判断路径是否相交 - 力扣](https://leetcode.cn/problems/path-crossing/) | ||
|
||
## 题目大意 | ||
|
||
**描述**:给定一个字符串 $path$,其中 $path[i]$ 的值可以是 `'N'`、`'S'`、`'E'` 或者 `'W'`,分别表示向北、向南、向东、向西移动一个单位。 | ||
|
||
你从二维平面上的原点 $(0, 0)$ 处开始出发,按 $path$ 所指示的路径行走。 | ||
|
||
**要求**:如果路径在任何位置上与自身相交,也就是走到之前已经走过的位置,请返回 $True$;否则,返回 $False$。 | ||
|
||
**说明**: | ||
|
||
- $1 \le path.length \le 10^4$。 | ||
- $path[i]$ 为 `'N'`、`'S'`、`'E'` 或 `'W'`。 | ||
|
||
**示例**: | ||
|
||
- 示例 1: | ||
|
||
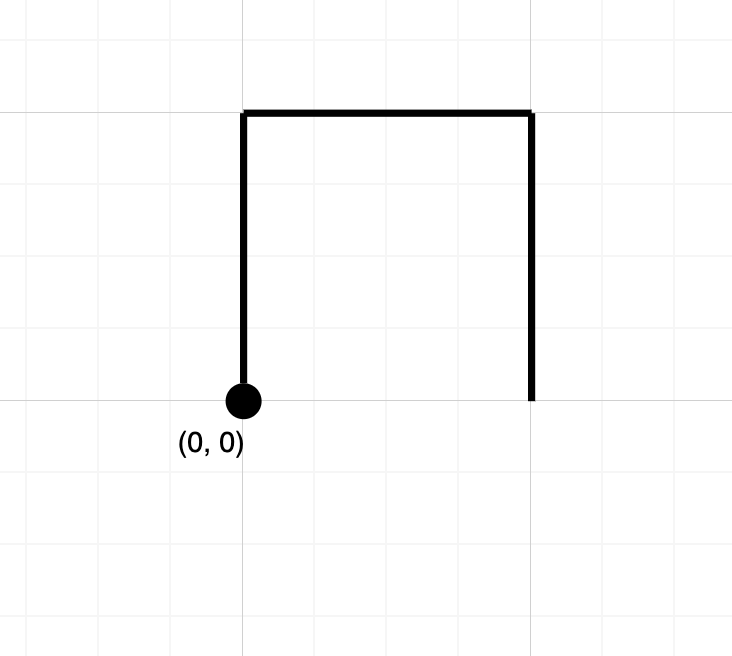 | ||
|
||
```python | ||
输入:path = "NES" | ||
输出:false | ||
解释:该路径没有在任何位置相交。 | ||
``` | ||
|
||
- 示例 2: | ||
|
||
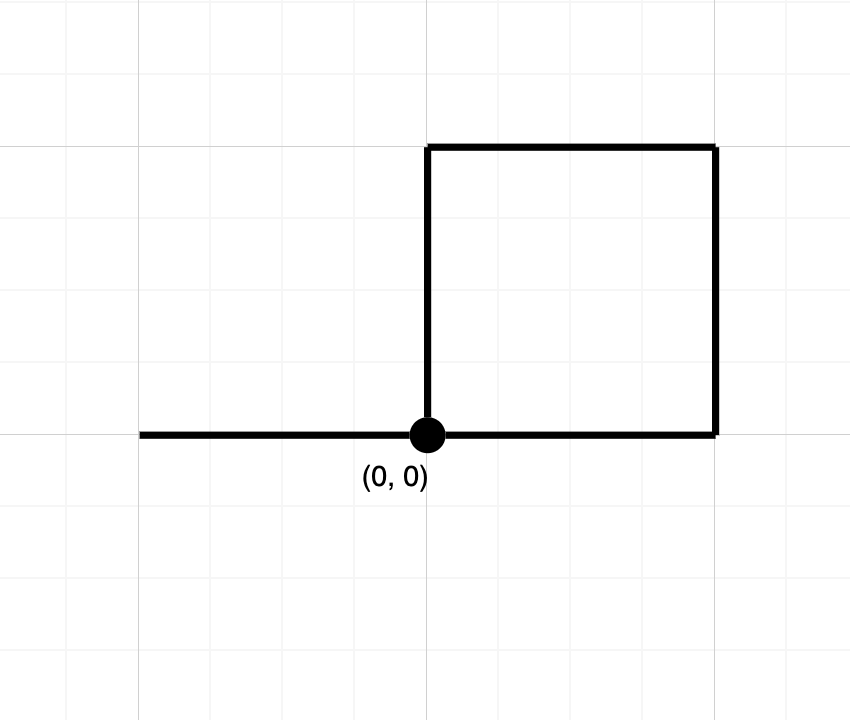 | ||
|
||
```python | ||
输入:path = "NESWW" | ||
输出:true | ||
解释:该路径经过原点两次。 | ||
``` | ||
|
||
## 解题思路 | ||
|
||
### 思路 1:哈希表 + 模拟 | ||
|
||
1. 使用哈希表将 `'N'`、`'S'`、`'E'`、`'W'` 对应横纵坐标轴上的改变表示出来。 | ||
2. 使用集合 $visited$ 存储走过的坐标元组。 | ||
3. 遍历 $path$,按照 $path$ 所指示的路径模拟行走,并将所走过的坐标使用 $visited$ 存储起来。 | ||
4. 如果在 $visited$ 遇到已经走过的坐标,则返回 $True$。 | ||
5. 如果遍历完仍未发现已经走过的坐标,则返回 $False$。 | ||
|
||
### 思路 1:代码 | ||
|
||
```Python | ||
class Solution: | ||
def isPathCrossing(self, path: str) -> bool: | ||
directions = { | ||
"N" : (-1, 0), | ||
"S" : (1, 0), | ||
"W" : (0, -1), | ||
"E" : (0, 1), | ||
} | ||
|
||
x, y = 0, 0 | ||
|
||
visited = set() | ||
visited.add((x, y)) | ||
|
||
for ch in path: | ||
x += directions[ch][0] | ||
y += directions[ch][1] | ||
if (x, y) in visited: | ||
return True | ||
visited.add((x, y)) | ||
|
||
return False | ||
``` | ||
|
||
### 思路 1:复杂度分析 | ||
|
||
- **时间复杂度**:$O(n)$,其中 $n$ 为数组 $path$ 的长度。 | ||
- **空间复杂度**:$O(n)$。 | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,75 @@ | ||
# [1647. 字符频次唯一的最小删除次数](https://leetcode.cn/problems/minimum-deletions-to-make-character-frequencies-unique/) | ||
|
||
- 标签:贪心、哈希表、字符串、排序 | ||
- 难度:中等 | ||
|
||
## 题目链接 | ||
|
||
- [1647. 字符频次唯一的最小删除次数 - 力扣](https://leetcode.cn/problems/minimum-deletions-to-make-character-frequencies-unique/) | ||
|
||
## 题目大意 | ||
|
||
**描述**:给定一个字符串 $s$。 | ||
|
||
**要求**:返回使 $s$ 成为优质字符串需要删除的最小字符数。 | ||
|
||
**说明**: | ||
|
||
- **频次**:指的是该字符在字符串中的出现次数。例如,在字符串 `"aab"` 中,`'a'` 的频次是 $2$,而 `'b'` 的频次是 $1$。 | ||
- **优质字符串**:如果字符串 $s$ 中不存在两个不同字符频次相同的情况,就称 $s$ 是优质字符串。 | ||
- $1 \le s.length \le 10^5$。 | ||
- $s$ 仅含小写英文字母。 | ||
|
||
**示例**: | ||
|
||
- 示例 1: | ||
|
||
```python | ||
输入:s = "aab" | ||
输出:0 | ||
解释:s 已经是优质字符串。 | ||
``` | ||
|
||
- 示例 2: | ||
|
||
```python | ||
输入:s = "aaabbbcc" | ||
输出:2 | ||
解释:可以删除两个 'b' , 得到优质字符串 "aaabcc" 。 | ||
另一种方式是删除一个 'b' 和一个 'c' ,得到优质字符串 "aaabbc"。 | ||
``` | ||
|
||
## 解题思路 | ||
|
||
### 思路 1:贪心算法 + 哈希表 | ||
|
||
1. 使用哈希表 $cnts$ 统计每字符串中每个字符出现次数。 | ||
2. 然后使用集合 $s\underline{}set$ 保存不同的出现次数。 | ||
3. 遍历哈希表中所偶出现次数: | ||
1. 如果当前出现次数不在集合 $s\underline{}set$ 中,则将该次数添加到集合 $s\underline{}set$ 中。 | ||
2. 如果当前出现次数在集合 $s\underline{}set$ 中,则不断减少该次数,直到该次数不在集合 $s\underline{}set$ 中停止,将次数添加到集合 $s\underline{}set$ 中,同时将减少次数累加到答案 $ans$ 中。 | ||
4. 遍历完哈希表后返回答案 $ans$。 | ||
|
||
### 思路 1:代码 | ||
|
||
```Python | ||
class Solution: | ||
def minDeletions(self, s: str) -> int: | ||
cnts = Counter(s) | ||
s_set = set() | ||
|
||
ans = 0 | ||
for key, value in cnts.items(): | ||
while value > 0 and value in s_set: | ||
value -= 1 | ||
ans += 1 | ||
s_set.add(value) | ||
|
||
return ans | ||
``` | ||
|
||
### 思路 1:复杂度分析 | ||
|
||
- **时间复杂度**:$O(n)$。 | ||
- **空间复杂度**:$O(n)$。 | ||
|