-
Notifications
You must be signed in to change notification settings - Fork 2
Django wiki
This will be the Django wiki where we will post our notes and tutorials for everyone to follow
First make a separate conda environment or if you already have the Dublin bikes environment from SE course use that.Since everyone uses Mac and already have conda use the command.
source activate your_env_name
Now in terminal go to your folder where the project will be cloned and use the command conda install Django
after which proceed to install Django. To see if Django was installed properly check the version by typing in python -m django --version
. you can see that the version will be 3.0.3 congratulations Django is installed.
django-admin
with this we will be able to see all the Django commands with one of them being startproject
to create a Django project so we should type in django-admin startproject project_name
this will create a full Django project with directories in the working directory like this: 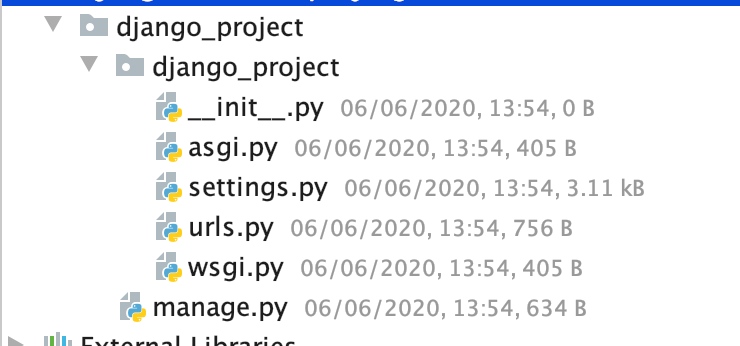
Now we can start working on the project
Now that Django has created all the file structure for us lets look at them the first file is
-
manage.py - This file is used to run all the commands which are executed via the terminal DO NOT MAKE ANY CHANGES TO THIS FILE
-
init.py - Normal python file which tells Python that this directory is a package we in flask have to make our own init file
-
settings.py - Has all the settings for our web app which we can tweak, it also has a secret key which will provide us with some security features when we go into production we must hide it other settings include one for DB. Each section has a link to the documentation
-
urls.py - setup for mapping of URLs for different users.
-
wsgi.py - Django sets up a default wsgi server for us to use.DO NOT MODIFY THIS
In your terminal run command python manage.py runserver
to run our Skeleton app, you will see the port in which Django has been launched usually it will be port :8000
so open localhost:port_number in browser to see our Django app.
If you go to link localhost:port_number/admin
you will see an admin page we won't be able to type in the credentials yet. If you remember there was a file called urls.py
if we go there we see the following code
urlpatterns = [
path('admin/', admin.site.urls),
]
This means if we go to the link called admin/
run the logic contained in admin.site.urls
this is how we do route logic in Django similar to @routes in flask
In Django, we have set up the basic website something that is confusing is that in this application itself we can have multiple apps which are its own things. So we can have a blog site an online store etc so a single project can have multiple apps this can be a good thing as we can use this to separate out features and we can add them to multiple projects example if we really like our blog app we can use it in multiple apps.
python manage.py startapp app_name
Is the command to create an app. After executing the command we will see a new folder structure like this:
from django.shortcuts import render
and we see render is already imported for us we will make another import from django httpresponse from django.http import HttpResponse
And after that we will create a function called home which will handle the traffic from home so the full file will look like this
from django.shortcuts import render
from django.http import HttpResponse
def home(request):
return HttpResponse('<h1>BLOG HOME</h1>')
def about(request):
return HttpResponse('<h1>About</h1>')
so we have a function called home it will take a response
(we will talk about response later) argument and return a response. Show what the user wants to see when he accesses a particular URL and how we want to handle certain routes.
Now make a urls.py file in the blogs and make it similar to urls.py in our django_project folder.
from django.contrib import admin
from django.urls import path
from . import views
urlpatterns = [
path('', views.home,name="blog-home"),
path('about/', views.about,name="blog-about")
]
Note that we imported views using a relative path because it's from the same package. look at the following code
path('', views.home,name="blog-home"),
it means when we get a path called '' execute function 'home' from views. basically we mapped the url to function in views.py. But the thing is if we simply type our address now it won't work because in our django-project directory we have another urls.py file so this file will tell whole website to what pages user must be sent if open the projects url.py file we get something like this
from django.contrib import admin
from django.urls import path,include
urlpatterns = [
path('admin/', admin.site.urls),
path('blog/',include('blog.urls'))
]
so if we type localhost:8000/blog/ this tells our project url.py to check for blog/ and indeed we find it. Now there is a statement called include('blogs.urls'))
which means redirect to the package blog URLs module. Note now the important concept along with passing to blog.urls we pass the additional URL which we type here we typed only localhost:8000/blog/ so it will pass everything after blog/ as an argument to blog.url where it will check and since we did not pass anything the code path('', views.home,name="blog-home"),
will be activated which will redirect to execute views.home and we get hello world website. This was a difficult topic and let's try another example. Let user in browser type localhost:8000/blog/about. Now in project url.py django will look for paths with /blog and indeed it finds one. The path has an include which asks Django to go to blog.url django goes there along with argument which is rest of the ur which is /about. In that urls.py Django looks at path associated with /about and sees that it asks Django to run function about() in view module.
Now what if we wanted Django to redirect us directly to the blog home page in that case simply change the path in project urls.py to path('',include('blog.urls'))
and test it out.
Look at following two routing examples from a link used in navbar
<a class="nav-item nav-link" href="{% url 'blog-home' %}">Home</a>
<a class="nav-item nav-link" href="/about">About</a>
Here we see that in first link href is done by using templates and in the template we used keyword called url
followed by the name
parameter we provided in url patters
urlpatterns = [
path('', views.home,name="blog-home"),
path('about/', views.about,name="blog-about")
]
this is a more dynamic approach which is more maintainable as we don't have to manually change stuff when we do any form of changes.
- Django installation
- Django Project creation
- Package Directories
- Hello,World
- Setting up projects
- Routing
- Templating
- Django templating Engine syntax
- Passing variable to template
- Template inheritance
- Static file linking
- Admin page
- Django ORM
- Making DB
- ORM based queries
- Django Model set
- Use of ORM DB with Django