-
Notifications
You must be signed in to change notification settings - Fork 2
Ui Module Wiki
This library makes it easy to process your gallery with RecyclerView.
Avoid using 'GalleryRecyclerView' as much as possible because it is the screen that requires custom the most in the app service. Rather, copy the example code and use it according to the app service.🥰
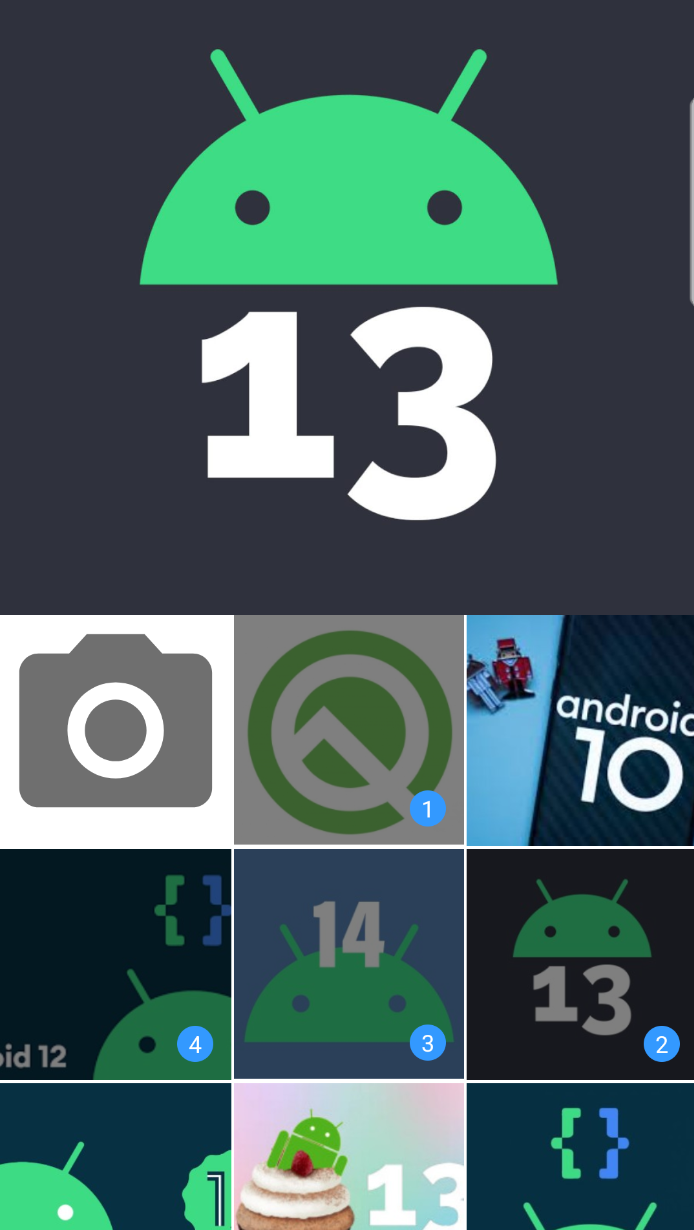
This is a UI class that expresses data imported through Cursor in RecyclerView
A function that must be invoked as required because there is a logic that automatically updates when a picture is added.
A function that imports gallery data and exposes it to RecyclerView.
Automatically process updates when gallery images are added.
First Index CameraViewHolder Visible Flag
First Index CameraViewHolder set Camera Resource Id
A function that sets the maximum number of pictures to be selected.
A function that sets the width/height value of the numeric UI exposed to the selected item in the picture.
The ability to set the background of the numeric UI that is exposed to the selected item in the picture.
The ability to set the color of the letter in the numeric UI that is exposed to the selected item in the picture.
'RequestManager' to be used in 'View Holder'.
ViewHolder Auto Click Function
set GalleryAdapter Click Listener
interface GalleryListener {
fun onCameraOpen()
/**
* Photo Picker Callback
* @param item Current GalleryItem
* @param isAdd true Picker, false No Picker
*/
fun onPhotoPicker(item: GalleryItem, isAdd: Boolean)
/**
* Picker Max Count Callback
*/
fun onMaxPickerCount()
}
<com.gallery.ui.GalleryRecyclerView
android:id="@+id/rvGallery"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:scrollbars="vertical"
app:cursor="@{vm.cursor}"
app:galleryCameraVisible="true"
app:gallerySelectedMaxCount="5"
app:layoutManager="androidx.recyclerview.widget.GridLayoutManager"
app:onGalleryCameraOpen="@{()->vm.onCameraOpen()}"
app:onGalleryMaxPicker="@{()->vm.onMaxPhotoClick()}"
app:onGalleryPhotoClick="@{(item,isAdd) -> vm.onPhotoClick(item,isAdd)}"
app:spanCount="3"
tools:listitem="@layout/vh_child_gallery" />
internal object GalleryBindingAdapter {
interface GalleryCameraOpenListener {
fun callback()
}
interface GalleryPhotoClickListener {
fun callback(item: GalleryItem, isAdd: Boolean)
}
interface GalleryMaxClickListener {
fun callback()
}
/**
* GalleryRecyclerView setListener
* DataBinding Example
*
*/
@JvmStatic
@BindingAdapter(
"onGalleryCameraOpen",
"onGalleryPhotoClick",
"onGalleryMaxPicker", requireAll = false
)
fun GalleryRecyclerView.setGalleryRecyclerViewListener(
cameraOpen: GalleryCameraOpenListener? = null,
photoClick: GalleryPhotoClickListener? = null,
maxClick: GalleryMaxClickListener? = null
) {
setListener(object : GalleryListener {
override fun onCameraOpen() {
cameraOpen?.callback()
}
override fun onPhotoPicker(item: GalleryItem, isAdd: Boolean) {
photoClick?.callback(item, isAdd)
}
override fun onMaxPickerCount() {
maxClick?.callback()
}
})
}
/**
* GalleryRecyclerView Set Cusor
* DataBinding Example
*/
@JvmStatic
@BindingAdapter("cursor")
fun setGalleryCursor(
view: GalleryRecyclerView,
cursor: Cursor?
) {
view.setCursor(cursor)
}
}