-
Notifications
You must be signed in to change notification settings - Fork 22
SDK usage examples
Thomas Piller edited this page Dec 15, 2022
·
3 revisions
Create a temporary GeoJSON view in MapX via the SDK
is fairly easy. Once the GeoJSON view is ready, you can interact with it as any other view in the views list : add or remove layer on the map, move it within the views list. If you chose to save it, it will be available the next time the user open this instance of MapX: the data is saved locally, in the browser. Beside view interaction, you can write a custom script to animate the features style on the map.
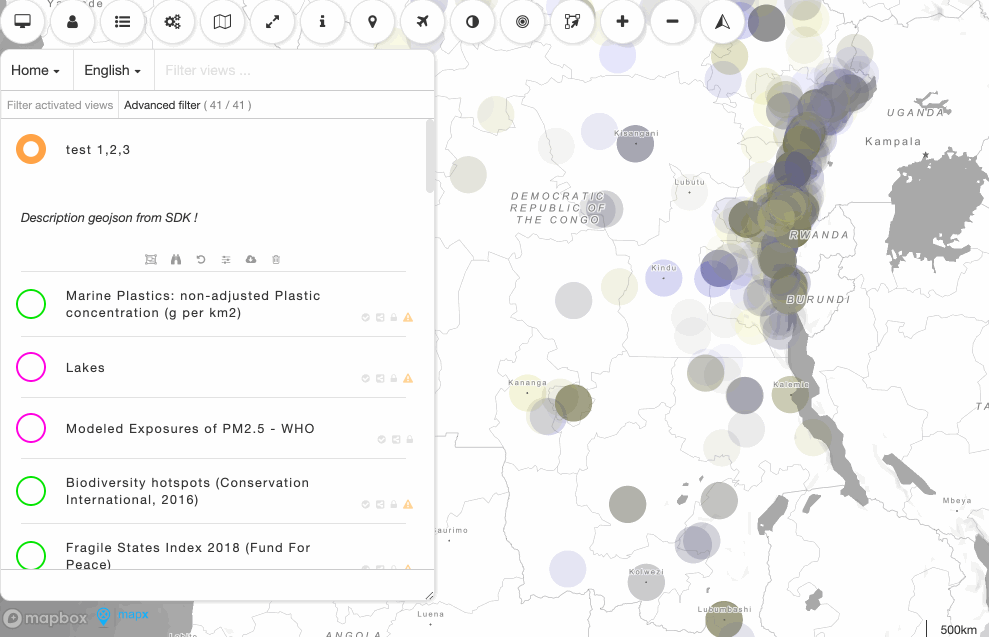
mapx.on('ready', () => {
/**
* Fetch data, geojson
*/
fetch(`< url to geojson >`)
.then((r) => r.json() // or r.text)
.then((gj) => {
/**
* Create temporary view
*/
return mapx.ask('view_geojson_create', {
data: gj,
filetype: 'geojson',
title: 'test 1,2,3',
abstract: 'Description GeoJSON from SDK !',
save: false
});
})
.then((v) => {
/*
* View as object is returned, use the id
*/
setRandomStyle(v.id);
});
});
/*
* Example of random style, to illustrate style change.
* @param {String} idView Any vector idView. In this example, newly created GeoJSON view's id.
*/
var n = 0;
function setRandomStyle(idView) {
/**
* Expression
*/
const rule = ['rgba',['*',['get',f()],2.5], ['*',['get',f()],2.5], ['*',['get',f()],2.5],0.1];
/**
* SDK command
*/
mapx.ask('view_geojson_set_style', {
idView: idView,
paint: {
'circle-radius': Math.random() * 100, // discrete values = transition ✨
'circle-color': col,
'circle-stroke-color':col
}
});
/**
* no more than 3 repeat. If the view is removed before end, this will produce errors.
*/
if(n++>3){
return;
}
setTimeout(() => {
setRandomStyle(idView);
}, 1000);
}
/*
* The data has 5 fields. Choose randomly one
* @return {String} random field name
*/
function f(){
return ['a','b','c','d','e'][Math.ceil(Math.random()*4)];
}
Anything unclear or inaccurate? Please let us know at [email protected]
1. Introduction
- 1.1 MapX highlights
- 1.2 MapX key concepts
- 2.1 Data catalog
- 2.1.1 Project
- 2.1.2 Login
- 2.1.3 Language change
- 2.1.4 Filtering
- 2.1.5 Sorting and organizing
- 2.2 Toolbox (basics)
- 2.3 Search tool
- 2.4 Menu bar
- 2.4.1 Navigation buttons
- 2.4.2 Map composer
- 2.4.3 Draw tool
- 2.4.4 Sharing manager
- 2.4.5 Highlight vector features
- 2.4.6 Others
3. Views
- 3.1 Definition
- 3.2 Badges
- 3.3 Tools
- 3.3.1 Download
- 3.3.2 Code share
- 3.3.3 Attribute table
- 3.3.4 Others
- 3.4 Querying and filtering data
- 3.5 Publication of new views
- 3.6 Dashboards
- 3.7 Sharing views between projects
4. Metadata
- 4.1 Structure
- 4.2 Editing metadata
5. Sources
- 5.1 Publication of new sources
- 5.1.1 Data preparation
- 5.1.2 Supported formats
- 5.1.3 Data publication
- 5.1.4 Tips & tricks
- 5.2 Managing sources
- 5.3 Editing sources
- 5.4 Table join tool
- 5.4.1 SQL joins
- 5.4.2 Create/edit a table join
6. Story maps
- 6.1 How to tell a great story?
- 6.2 Recommendations for story map development
- 6.3 Preparing your story map
- 6.4 Glossary
- 6.5 Creating a story map view
- 6.6 Adding content to a story map
- 6.6.1 Defining the settings of a story map
- 6.6.2 Creating a step
- 6.7 Sharing a story map
- 6.8 Tips & tricks
- 9.1 How to contribute
- 9.2 Upgrade Docker images
- 9.3 Search tool API
- 9.4 URL parameters
- 9.5 SDK documentation
- 9.6 SDK usage examples