-
Notifications
You must be signed in to change notification settings - Fork 129
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Validate rsa private key and certificate content format #971
Changes from 2 commits
4dda146
09b6e3f
0523b4c
f11f463
42f70e8
5caf505
File filter
Filter by extension
Conversations
Jump to
Diff view
Diff view
There are no files selected for viewing
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,14 +1,15 @@ | ||
'use strict'; | ||
|
||
const fs = require('fs-extra'); | ||
const path = require('path'); | ||
const ram = require('../ram'); | ||
const debug = require('debug')('fun:deploy'); | ||
const promiseRetry = require('../retry'); | ||
const getProfile = require('../profile').getProfile; | ||
|
||
const { green, red } = require('colors'); | ||
const { processApiParameters } = require('./deploy-support-api'); | ||
const { getCloudApiClient, getSlsClient, getMnsClient } = require('../client'); | ||
const {green, red} = require('colors'); | ||
const {processApiParameters} = require('./deploy-support-api'); | ||
const {getCloudApiClient, getSlsClient, getMnsClient} = require('../client'); | ||
|
||
const { | ||
getOtsClient, | ||
|
@@ -75,7 +76,9 @@ async function makeLogstore({ | |
console.log(red(`\t\tretry ${times} times`)); | ||
|
||
retry(ex); | ||
} else { exists = false; } | ||
} else { | ||
exists = false; | ||
} | ||
} | ||
}); | ||
|
||
|
@@ -153,7 +156,9 @@ async function slsProjectExist(slsClient, projectName) { | |
|
||
console.log(red(`\tretry ${times} times`)); | ||
retry(ex); | ||
} else { projectExist = false; } | ||
} else { | ||
projectExist = false; | ||
} | ||
} | ||
}); | ||
return projectExist; | ||
|
@@ -250,12 +255,50 @@ async function makeCustomDomain({ | |
let privateKey = certConfig.PrivateKey; | ||
let certificate = certConfig.Certificate; | ||
|
||
if (privateKey && privateKey.endsWith('.pem')) { | ||
certConfig.PrivateKey = await fs.readFile(privateKey, 'utf-8'); | ||
} | ||
if (certificate && certificate.endsWith('.pem')) { | ||
certConfig.Certificate = await fs.readFile(certificate, 'utf-8'); | ||
} | ||
if (privateKey) { | ||
//region resolve RSA private key content | ||
let p = path.resolve(__dirname, privateKey); | ||
// private key is provided by local file | ||
if (fs.pathExistsSync(p)) { | ||
certConfig.PrivateKey = fs.readFileSync(p, 'utf-8'); | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more.
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. Done |
||
} // or it is hardcoded | ||
//endregion | ||
|
||
//region validate RSA private key content | ||
let expectedPrefix = '-----BEGIN RSA PRIVATE KEY-----', expectedSuffix = '-----END RSA PRIVATE KEY-----'; | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. 写在 const 里面吧 There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. Done |
||
if (!certConfig.PrivateKey.startsWith(expectedPrefix) || !certConfig.PrivateKey.endsWith(expectedSuffix)) { | ||
throw new Error(red(` | ||
Please provide a valid PEM encoded RSA private key for ${domainName}. | ||
It's content MUST start with "${expectedPrefix}" AND end with "${expectedSuffix}". | ||
|
||
See: | ||
http://fileformats.archiveteam.org/wiki/PEM_encoded_RSA_private_key`)); | ||
} | ||
//endregion | ||
} // private key is not provided | ||
|
||
if (certificate) { | ||
//region resolve certificate content | ||
let p = path.resolve(__dirname, certificate); | ||
// certificate is provided by local file | ||
if (fs.pathExistsSync(p)) { | ||
certConfig.Certificate = fs.readFileSync(p, 'utf-8'); | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more.
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. Done |
||
} // or it is hardcoded | ||
//endregion | ||
|
||
//region validate certificate content | ||
let expectedPrefix = '-----BEGIN CERTIFICATE-----', expectedSuffix = '-----END CERTIFICATE-----'; | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. 写在 const 里面吧 There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. Done |
||
if (!certConfig.Certificate.startsWith(expectedPrefix) || !certConfig.Certificate.endsWith(expectedSuffix)) { | ||
throw new Error(red(` | ||
Please provide a valid PEM encoded certificate for ${domainName}. | ||
It's content MUST start with "${expectedPrefix}" AND end with "${expectedSuffix}". | ||
|
||
See: | ||
http://fileformats.archiveteam.org/wiki/PEM_encoded_certificate`)); | ||
} | ||
//endregion | ||
} // certificate is not provided | ||
|
||
Object.assign(options, { | ||
certConfig | ||
}); | ||
|
@@ -373,9 +416,11 @@ async function makeApi(group, { | |
'requestPath': requestPath | ||
}, requestConfig); | ||
|
||
const { apiRequestParameters, | ||
const { | ||
apiRequestParameters, | ||
apiServiceParameters, | ||
apiServiceParametersMap } = processApiParameters(requestParameters, serviceParameters, serviceParametersMap); | ||
apiServiceParametersMap | ||
} = processApiParameters(requestParameters, serviceParameters, serviceParametersMap); | ||
|
||
const profile = await getProfile(); | ||
|
||
|
@@ -676,4 +721,4 @@ module.exports = { | |
makeApiTrigger, makeSlsProject, makeOtsInstance, | ||
makeCustomDomain, makeLogstoreIndex, makeSlsAuto, | ||
listCustomDomains | ||
}; | ||
}; |
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,17 @@ | ||
let fs = require('fs-extra'); | ||
let path = require('path'); | ||
const expect = require('expect.js'); | ||
|
||
describe('fs-extra module Tests', function () { | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. 这个测试集的作用是? There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more.
需要添加注释? 还是删掉这个测试集? |
||
|
||
it('should exists', function () { | ||
let p = path.resolve(__dirname, '../LICENSE'); | ||
expect(fs.pathExistsSync(p)).to.be(true); | ||
console.log(fs.readFileSync(p, 'utf-8')); | ||
}); | ||
|
||
it('should not exists', function () { | ||
let p = path.resolve(__dirname, '-----BEGIN RSA PRIVATE KEY-----'); | ||
expect(fs.pathExistsSync(p)).to.be(false); | ||
}); | ||
}); |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
eslint 是如何配置的?
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
我是在 Windows 上用 WebStorm 开发的, 未修改 .eslintrc 的内容, 对 deploy-support.js 使用 .eslintrc 的格式进行格式化的.
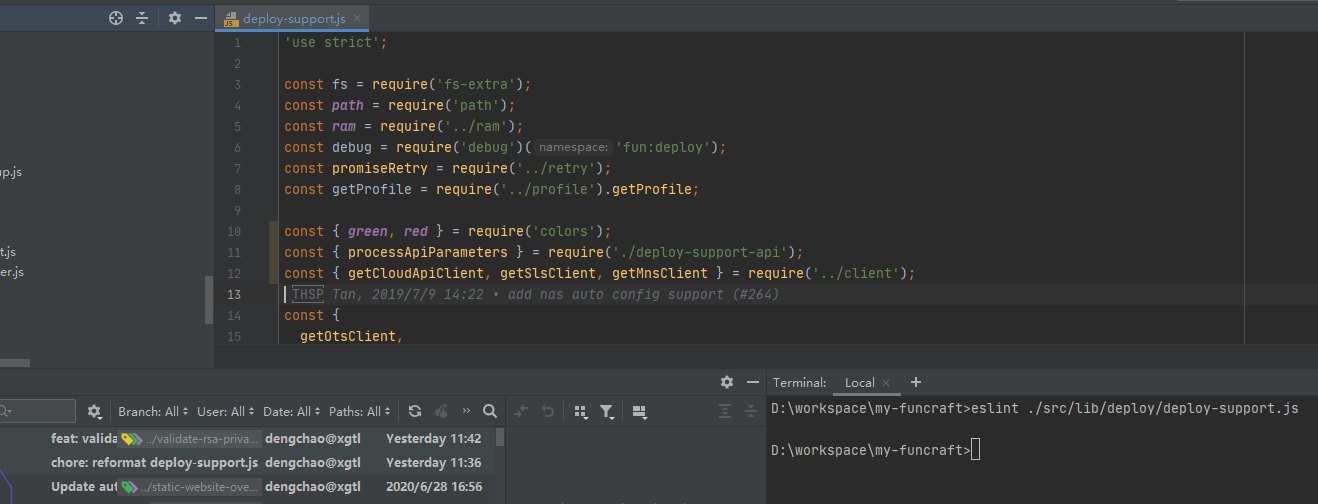
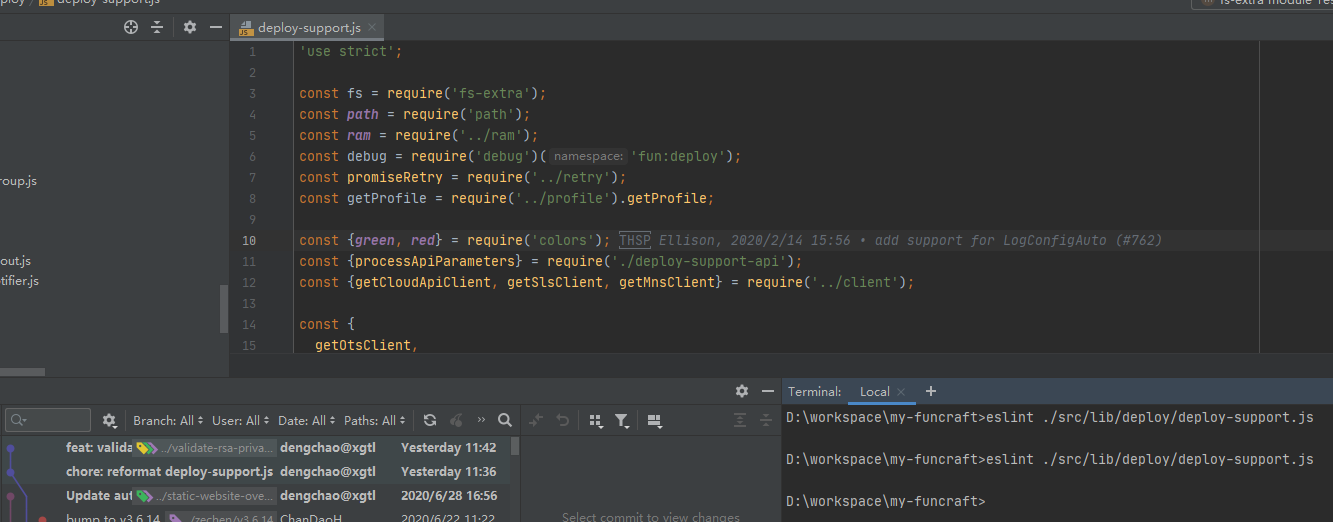
测试后发现有没有空格 eslint 都没有报错.
需要我回滚格式化代码的 commit 么?